C++ string interpolation allows you to embed variable values directly within a string by using a combination of string concatenation or formatting libraries, such as `std::ostringstream` for a clean and concise output.
Here’s an example using `std::ostringstream`:
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::string name = "Alice";
int age = 30;
std::ostringstream oss;
oss << "Hello, my name is " << name << " and I am " << age << " years old.";
std::cout << oss.str() << std::endl;
return 0;
}
Understanding Strings in C++
What is a String in C++?
In C++, a string is a sequence of characters used to represent text. The most common way to handle strings is through the `std::string` class provided by the Standard Library. It offers multiple member functions and operators that simplify the manipulation of strings compared to traditional C-style strings, which are arrays of characters terminated by a null character (`'\0'`).
C-style strings can be cumbersome to handle, especially when it comes to memory management, whereas `std::string` automatically manages memory allocation and deallocation, making it a preferred choice for modern C++ programming.
Why String Interpolation Matters?
String interpolation is a powerful technique that allows you to construct strings from multiple variables smoothly and efficiently. Its significance lies in improving code readability and reducing the potential for errors. When using string interpolation, you can avoid the tedious and error-prone process of manual string concatenation, leading to clearer and more maintainable code.

Traditional Methods of String Construction
Using `+` Operator for Concatenation
The simplest approach to concatenate strings is by using the `+` operator. This method allows you to concatenate two strings or append a string to another easily. Here’s a straightforward code example:
std::string firstName = "John";
std::string lastName = "Doe";
std::string fullName = firstName + " " + lastName;
In this example, the `fullName` variable is constructed by concatenating `firstName`, a space, and `lastName`. While this approach is simple, it can become unwieldy when dealing with multiple strings or variables, leading to decreased readability.
Using `sprintf()` for Formatted Strings
Another method is using `sprintf()` from the C standard library. This function allows for formatted output similar to how you would use printf in C. Here’s how it can be employed:
char buffer[100];
sprintf(buffer, "Hello, %s %s!", firstName.c_str(), lastName.c_str());
In this code snippet, the `sprintf()` function formats the string and stores it in the `buffer`. While effective, the method is somewhat outdated and requires careful management of buffer sizes to avoid buffer overflow errors.
Using `stringstream`
The `stringstream` class provides a more object-oriented way to handle string construction. It streamlines the process of building strings and can be more efficient than concatenation. Here’s an illustration:
#include <sstream>
std::stringstream ss;
ss << "Hello, " << firstName << " " << lastName << "!";
std::string output = ss.str();
This code leverages `stringstream` to concatenate strings. The `str()` member function retrieves the constructed string, making this method both flexible and efficient for building more complex strings.

Modern C++ Approaches to String Interpolation
Introduction to C++20 Features
With the introduction of C++20, several features were added to enhance string handling, including the `std::format()` function, which simplifies string formatting and interpolation.
Using `std::format()`
The `std::format()` function allows for straightforward string interpolation, handling type conversions implicitly and supporting various format specifiers. It significantly improves readability while maintaining performance. Here’s a quick example:
#include <format>
std::string formattedString = std::format("Hello, {} {}!", firstName, lastName);
In this example, `std::format()` takes care of inserting the values of `firstName` and `lastName` into the string template. This approach allows for cleaner syntax and minimizes the risk of errors associated with manual string construction.
Leveraging String Views
Another modern feature is `std::string_view`, an efficient way to view strings without making copies. It can be particularly useful in string interpolation when performance matters. Here’s how you might use it:
#include <string_view>
#include <format>
std::string_view viewFirstName = firstName;
std::string combined = std::format("Hello, {}!", viewFirstName);
Using `std::string_view` prevents unnecessary allocations and copies, making your string interpolation code more efficient.
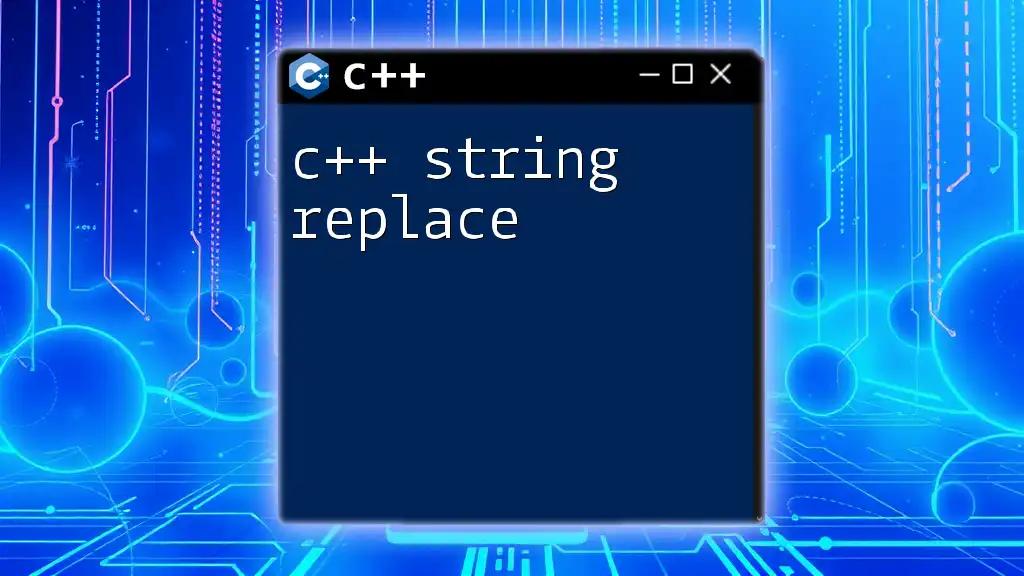
Best Practices for String Interpolation
Choosing the Right Approach
When it comes to string interpolation in C++, you have several options. The choice largely depends on factors like readability, performance, and the complexity of the strings you’re working with. Generally, prefer `std::format()` for modern applications due to its flexibility and safety, especially when dealing with various types of data.
Avoiding Common Pitfalls
While string manipulation is a powerful tool, it can lead to common pitfalls such as:
- Buffer overflows when using functions like `sprintf()`.
- Loss of performance when excessively concatenating temporary strings.
- Increased complexity and decreased readability in large string constructions.
Mitigating these issues involves consistently applying the best practices outlined above.

Conclusion
C++ string interpolation can dramatically enhance how you manage and manipulate text in your programs. By adopting modern techniques and tools, you can boost your code’s readability and maintainability while minimizing the chances of errors. As C++ continues to evolve, embracing its features will lead to more elegant and efficient coding practices.