In C++, the `insert` member function of the `std::string` class allows you to insert a substring or character at a specified position within the original string.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello World!";
str.insert(5, ", C++"); // Inserts ", C++" at index 5
std::cout << str << std::endl; // Output: Hello, C++ World!
return 0;
}
Understanding C++ Strings
In C++, a string is a sequence of characters that represent text. It is essential to comprehend the difference between `std::string` and C-style strings. `std::string`, part of the C++ Standard Library, offers a more flexible and user-friendly interface compared to C-style strings, which are simply an array of characters ending with a null terminator (`'\0'`).
Common operations on strings include concatenation, comparison, and searching. Among these operations, string insertion plays a vital role, allowing developers to manipulate and create dynamic text content effectively.
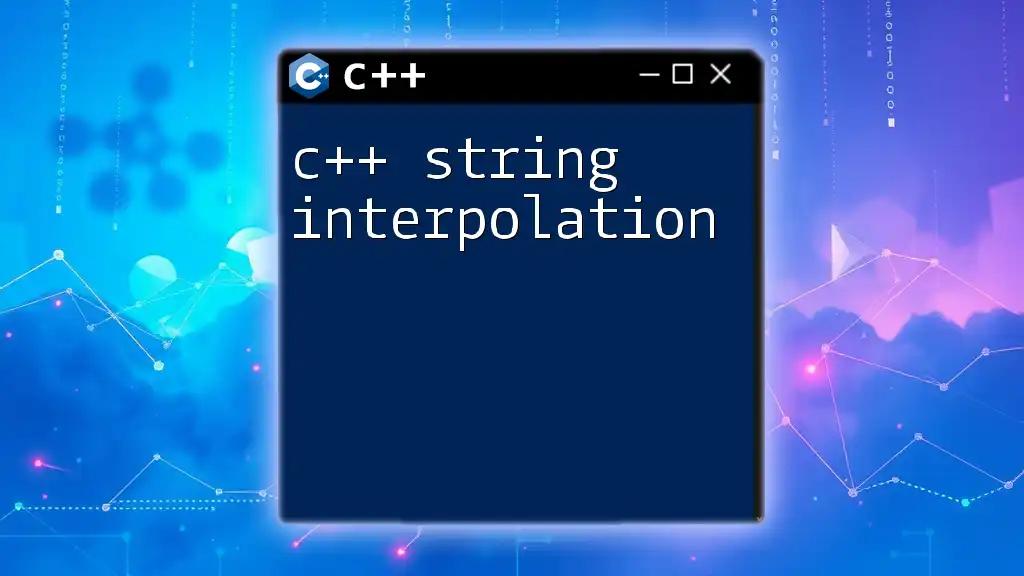
The `insert` Function Overview
The `insert` function in C++ is a powerful utility for inserting content into a string at a specific position. It enhances the way strings can be manipulated, making code cleaner and more intuitive. Understanding how to utilize it can greatly improve string operations in your C++ programs.
Syntax of the `insert` Function
The syntax of the `insert` function can vary depending on what you intend to insert:
-
Basic Syntax for Inserting a String
string& insert(size_t pos, const string& str);
This function inserts the string `str` before the character at position `pos`.
-
Inserting a Character
string& insert(size_t pos, size_t n, char c);
Here, `n` characters of `c` are inserted starting at position `pos`.
-
Inserting a Substring
string& insert(size_t pos, const string& str, size_t subpos, size_t sublen);
This variant allows you to insert a substring from `str` starting at `subpos` with length `sublen`.

Inserting Strings at Specific Positions
Example 1: Basic String Insert
Consider the following example:
std::string str = "Hello, World!";
str.insert(7, "C++ ");
// Result: "Hello, C++ World!"
In this code, `C++ ` is inserted at position 7 of the original string. This effectively transforms it into "Hello, C++ World!" The `insert` function alters the original string by adding the new content seamlessly.
Example 2: Inserting Substring
You can also insert a substring:
std::string str = "Hello, World!";
str.insert(5, " Beautiful");
// Result: "Hello Beautiful, World!"
Here, " Beautiful" is inserted at position 5, demonstrating how the `insert` function facilitates dynamic text alteration.
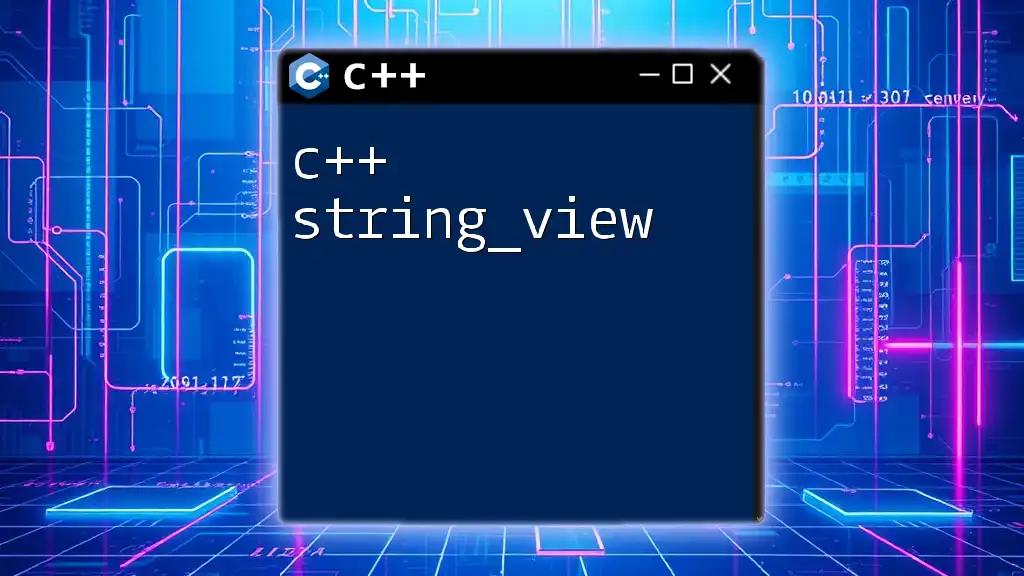
Inserting Characters at Specific Positions
Example 3: Inserting a Single Character
The `insert` function can also be used to insert single characters.
std::string str = "Hell World!";
str.insert(4, 1, 'o');
// Result: "Hello World!"
In this example, we insert a single character `'o'` at index 4. As a result, "Hell World!" becomes "Hello World!". This functionality showcases how the `insert` method can work at a more granular level, providing flexibility in string construction.
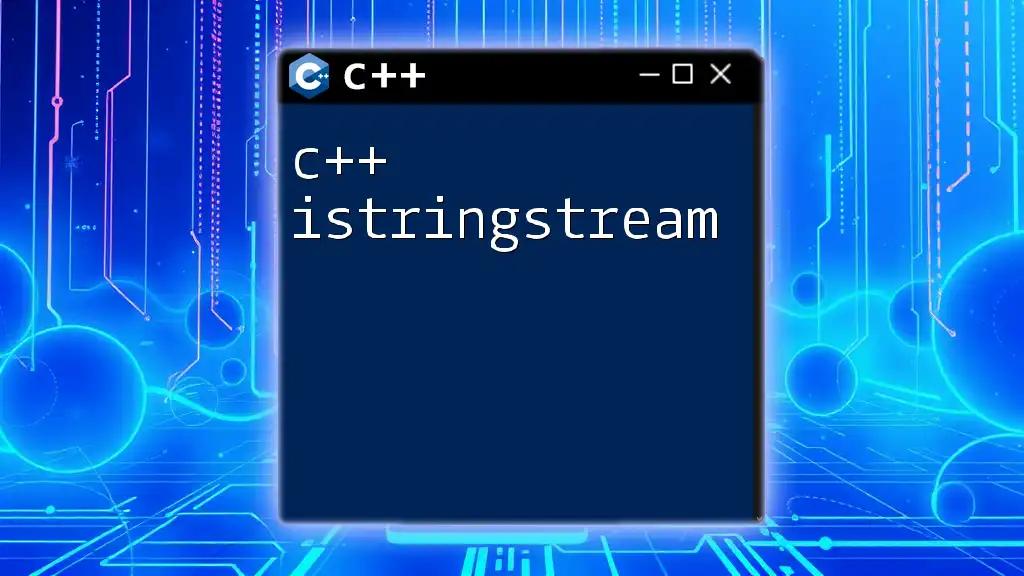
Using Iterators with the Insert Function
C++ allows for even more sophisticated string manipulation through the use of iterators.
Example 4: Inserting using Iterators
This method enables you to insert contents from one string into another.
std::string str1 = "Hello, ";
std::string str2 = "World!";
str1.insert(str1.end(), str2.begin(), str2.end());
// Result: "Hello, World!"
Here, we use `str1.end()` to specify where to insert `str2`. This technique can minimize the need for additional indices and aids in managing larger and more complex strings.
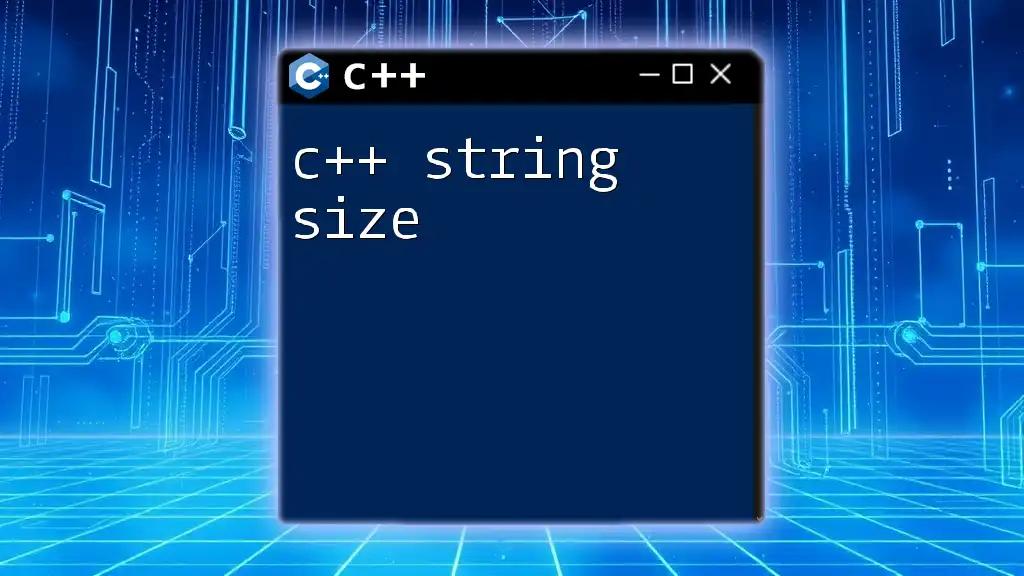
Practical Applications of String Insertion
Real-World Use Cases: Inserting strings can be particularly useful in a variety of scenarios, such as creating dynamic output reports, generating email templates, or even modifying file paths on-the-fly. For instance, when dynamically constructing log messages, correctly inserted strings can ensure clarity and consistency:
std::string logMessage = "Error: ";
logMessage.insert(logMessage.length(), "File not found");
// Result: "Error: File not found"

Handling Errors and Edge Cases
When using the `insert` function, it's crucial to consider potential pitfalls:
- Out-of-Bounds Insertion: Inserting at a position greater than the string length can throw exceptions.
- Performance Issues: Frequent string modifications can lead to inefficient memory usage – always be cautious with dynamic insertion in tight loops.
Implementing checks before an insert operation can safeguard your code against unwanted exceptions.
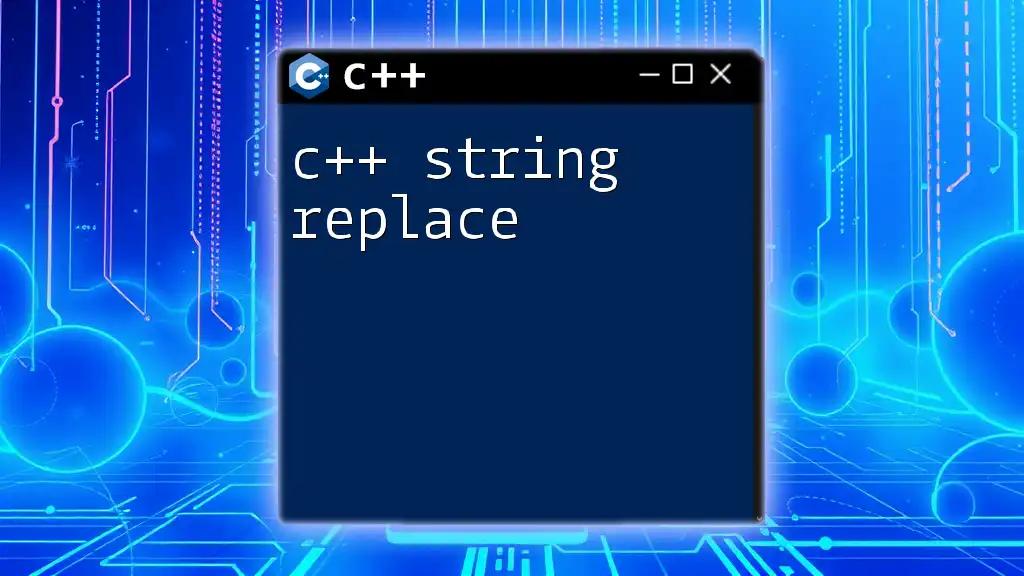
Performance Considerations
The complexity of string insertion depends on the position at which content is added. Inserting at the middle of a string requires shifting elements, which can affect performance. If lots of modifications are needed, consider using alternative data structures like `std::vector<char>` to handle heavy insert operations more efficiently. After all, strings in C++ are immutable in terms of size during insertion.

Conclusion
Understanding C++ string insertation is a fundamental skill for any developer working with text manipulation. The flexibility provided by the `insert` function can significantly enhance how strings are managed in your code. By mastering this functionality, you're well on your way to writing cleaner and more efficient C++ programs.
As you continue your programming journey, remember to practice these examples and explore additional string functions. With time and experience, string manipulation will become an intuitive part of your coding toolkit.

Additional Resources
Consider referring to C++ documentation, online courses, or textbooks focusing on string operations for further insight and examples. Experimenting with `std::string` in a hands-on manner will deepen your understanding and skill in using this versatile language feature effectively.