In C++, the `std::to_string` function converts various data types, such as integers and floating-point numbers, into their string representation.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <string>
int main() {
int number = 42;
std::string strNumber = std::to_string(number);
std::cout << "The string representation is: " << strNumber << std::endl;
return 0;
}
What is Stringification?
Stringification is a process in programming that converts code elements into string literals. In the context of C++, it allows you to take an identifier, expression, or even a macro and turn it into its string representation. This can be incredibly useful, especially for debugging or when you need to generate strings dynamically at compile time.
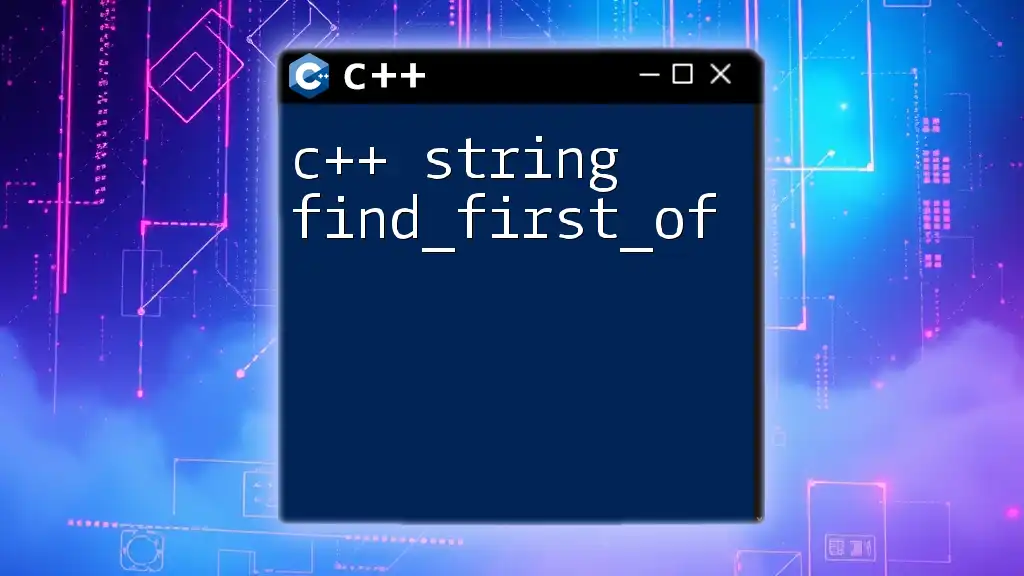
The Basics of Stringification
Understanding Macros and Preprocessor Directives
In C++, the preprocessor is a powerful tool that handles directives before the actual compilation begins. Macros defined using the `#define` directive enable us to create concise and reusable code snippets. When utilized alongside stringification, they can enhance code readability and reduce repetition.
Stringification in C++
In C++, stringification is primarily accomplished using the `#` operator within a macro definition. This operator takes a macro argument and converts its value into a string literal, facilitating various practical applications.
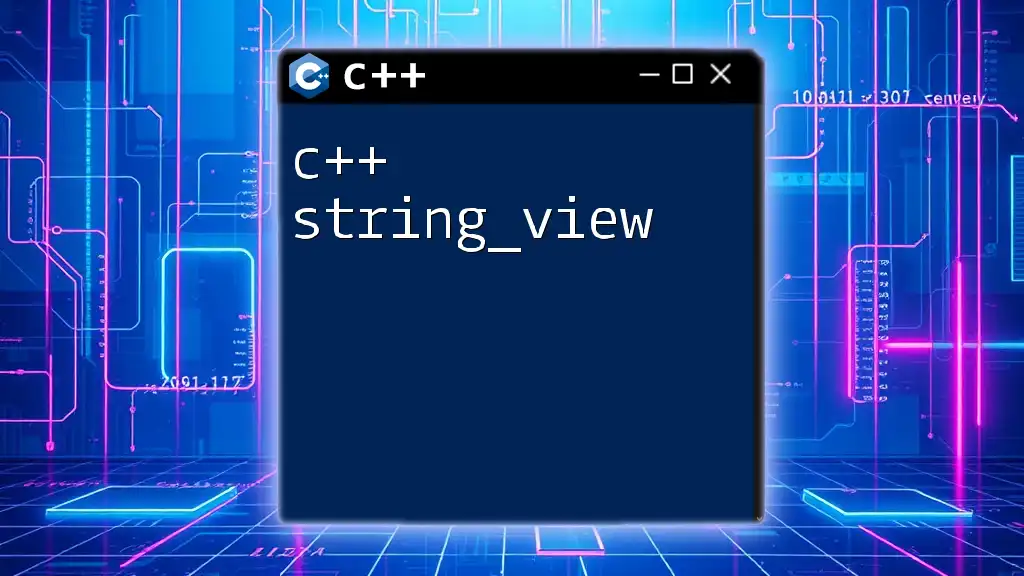
Different Methods to Stringify in C++
Using the Stringify Macro
Creating a Simple Stringify Macro
Creating a stringification macro is straightforward. You can define a macro that takes an argument and produces its string representation:
#define STRINGIFY(x) #x
Explanation: When you call `STRINGIFY(variableName)`, it returns `"variableName"` as a string literal. This can be particularly useful when you want the names of variables or macros to be represented as strings.
Practical Example: Stringifying a Variable Name
Let’s see how the `STRINGIFY` macro works in action:
#include <iostream>
#define STRINGIFY(x) #x
int main() {
std::cout << STRINGIFY(variableName) << std::endl; // Outputs: variableName
return 0;
}
In this example, calling `STRINGIFY(variableName)` will produce the output `variableName`. This allows you to generate string representations dynamically, which is beneficial for logging and error messages.
Stringification with Concatenation
Combining Stringification with Concatenation
You can combine stringification with token pasting to create more complex macros. The `##` operator concatenates tokens together, and when used in tandem with the `#` operator, you can create quite powerful macros.
Here’s how you can do that:
#define MAKE_STRING(name) STRINGIFY(name)
#define CONCAT_NAME(name1, name2) name1##name2
int main() {
int myVariable = 10;
std::cout << MAKE_STRING(myVariable) << std::endl; // Outputs: myVariable
std::cout << CONCAT_NAME(my, Variable) << std::endl; // Outputs the name of the concatenated variable
return 0;
}
In this snippet, `MAKE_STRING(myVariable)` will convert `myVariable` to its string representation, whereas `CONCAT_NAME(my, Variable)` will concatenate `my` and `Variable` at compile time, effectively treating it as a single variable name.
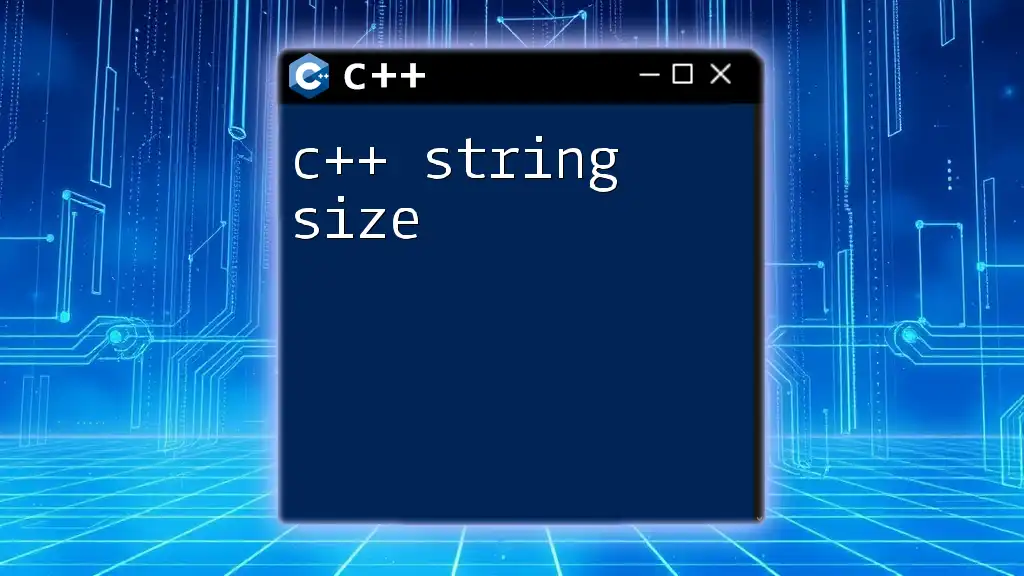
Common Use Cases for Stringification
Debugging
One of the primary uses of stringification is for debugging purposes. By logging variable names and their values, you can gain better insights into your code's behavior. For example:
#define DEBUG_LOG(variable) std::cout << "Debug: " << STRINGIFY(variable) << " = " << variable << std::endl;
int main() {
int x = 42;
DEBUG_LOG(x); // Outputs: Debug: x = 42
return 0;
}
This example shows how stringification can enhance debug output, making tracking variables significantly easier.
Configuration Management
Stringification can also assist in managing configuration settings within your code. By creating string literals for configuration keys, you can standardize how values are referenced throughout your application.
#define CONFIG_KEY(key) STRINGIFY(key)
int main() {
std::cout << "Configuration Key: " << CONFIG_KEY(MAX_CONNECTIONS) << std::endl; // Outputs: Configuration Key: MAX_CONNECTIONS
return 0;
}
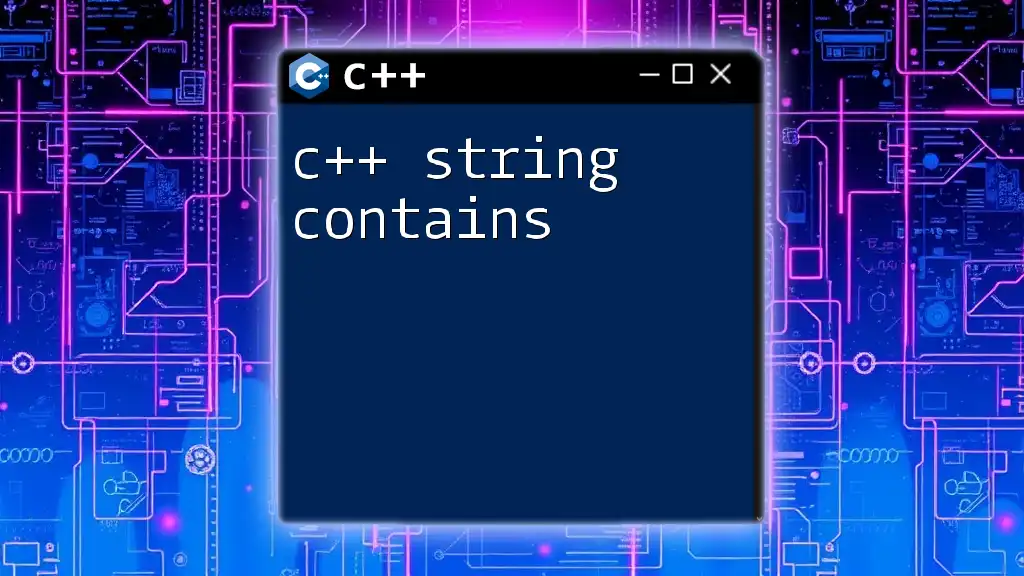
Best Practices for Stringification
Avoiding Common Pitfalls
While stringification is a powerful feature, there are common pitfalls that you should be aware of. For example, the `#` operator can introduce unexpected results if not utilized correctly. Here’s a potential issue:
#define STRINGIFY(x) #x
std::cout << STRINGIFY(1 + 2) << std::endl; // Outputs: "1 + 2" but not "3"!
In this case, the output is not the evaluation of `1 + 2` but the string literal `"1 + 2"`. Ensure that you understand these nuances to avoid confusion.
When to Use Stringification
Stringification is most beneficial in scenarios where dynamic string generation is required, such as logging, debugging, or constructing messages based on variable names. However, it should be used judiciously to maintain code clarity and avoid overcomplication.
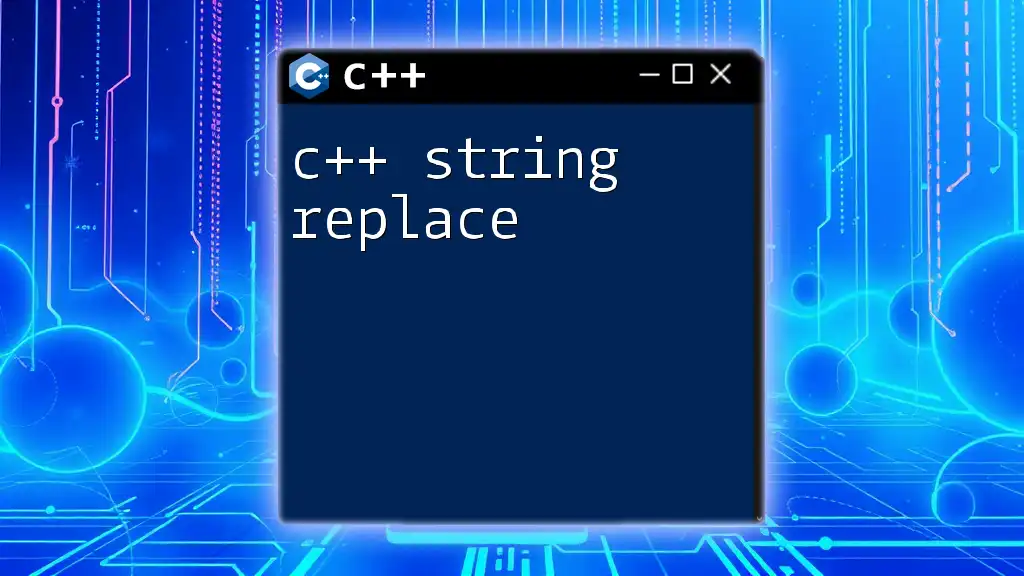
Conclusion
C++ stringify is an essential tool for developers, providing the ability to convert identifiers into string literals easily. By mastering stringification techniques, you can effectively enhance your debugging processes and manage configurations more efficiently. Experiment with the discussed macros and see how they can be integrated into your C++ projects for better performance and readability.
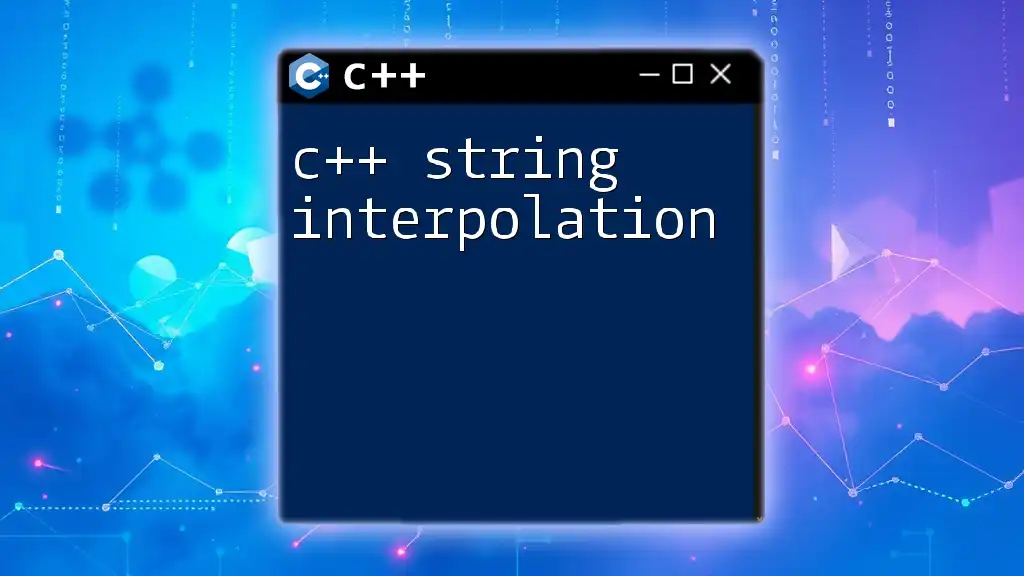
Additional Resources
To deepen your understanding of C++ stringification, consider exploring the following resources:
- Standard C++ documentation on preprocessor directives and macros
- Recommended books on C++ programming to build a strong foundation
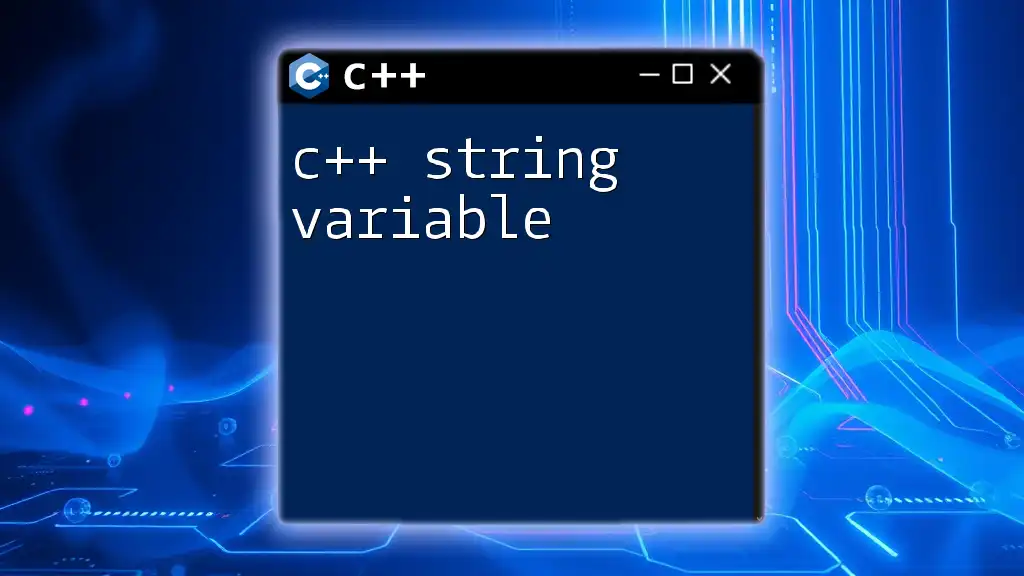
FAQs
What is the difference between stringification and concatenation?
Stringification transforms identifiers into string literals, while concatenation combines multiple tokens or strings into a single string. They can be used together for powerful macro definitions.
Can stringification be used in all versions of C++?
Yes, stringification using the `#` operator is part of the C++ preprocessor and is available in all standard versions of C++.
Where can I find more advanced stringification techniques?
Advanced techniques can be found in specialized programming books and online resources dedicated to macro programming and preprocessor usage in C++. Exploring community forums can also yield valuable insights into real-world applications.