In C++, you can obtain the size of a string using the `size()` or `length()` member functions, which return the number of characters in the string.
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, World!";
std::cout << "Size of the string: " << myString.size() << std::endl;
return 0;
}
Understanding Strings in C++
What is a String in C++?
In C++, a string is a sequence of characters. Unlike C-style strings that are arrays of characters terminated by a null character (`'\0'`), C++ provides a more powerful and flexible `std::string` class. This class enhances simplicity and safety when managing string data.
You can define a string as follows:
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello, World!";
std::cout << greeting << std::endl;
return 0;
}
This snippet demonstrates the straightforward declaration and initialization of a string.
The C++ Standard Library
The `std::string` class belongs to the C++ Standard Library, making it a robust choice for handling strings. It provides various functions to manipulate strings efficiently, leading to better memory management and less complexity for developers compared to raw character arrays.
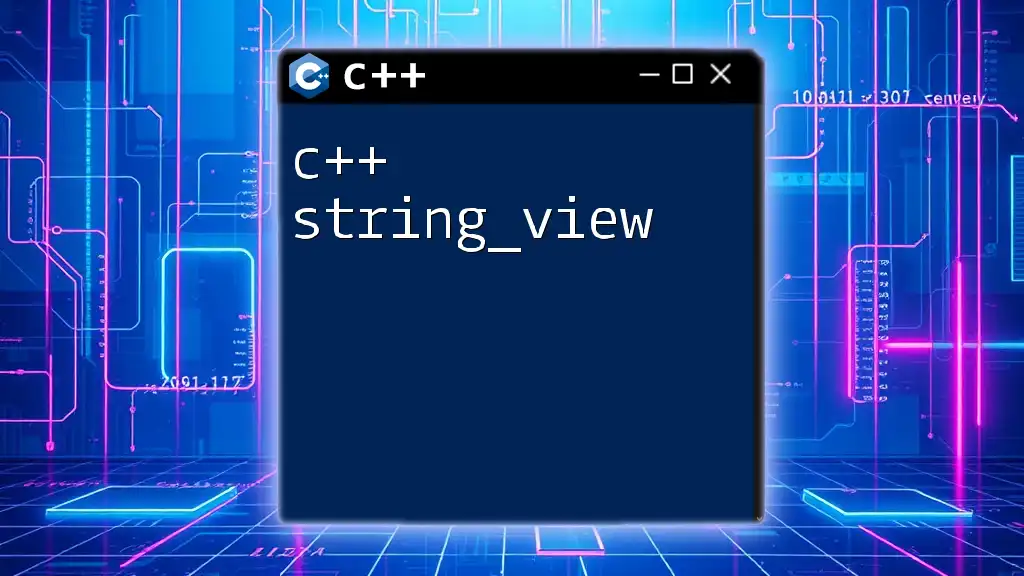
Determining the Size of a String in C++
C++ String Size Function
To find out the size of a C++ string, you can utilize the `.size()` method. This method returns the total number of characters in the string, including spaces but excluding the null character.
The syntax is straightforward:
string_object.size();
Here’s an example:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::cout << "Size of string: " << str.size() << std::endl; // Output: 13
return 0;
}
In this example, the size of the string "Hello, World!" is 13 characters.
Alternative: Using Length Method
C++ also provides a `.length()` method, which serves the same purpose as `.size()`. Both methods will return an integer representing the number of characters in the string. They can be used interchangeably, but `.length()` is a legacy method dating back to earlier versions of the language.
Consider this illustrative example:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello!";
std::cout << "Length of string: " << str.length() << std::endl; // Output: 6
return 0;
}
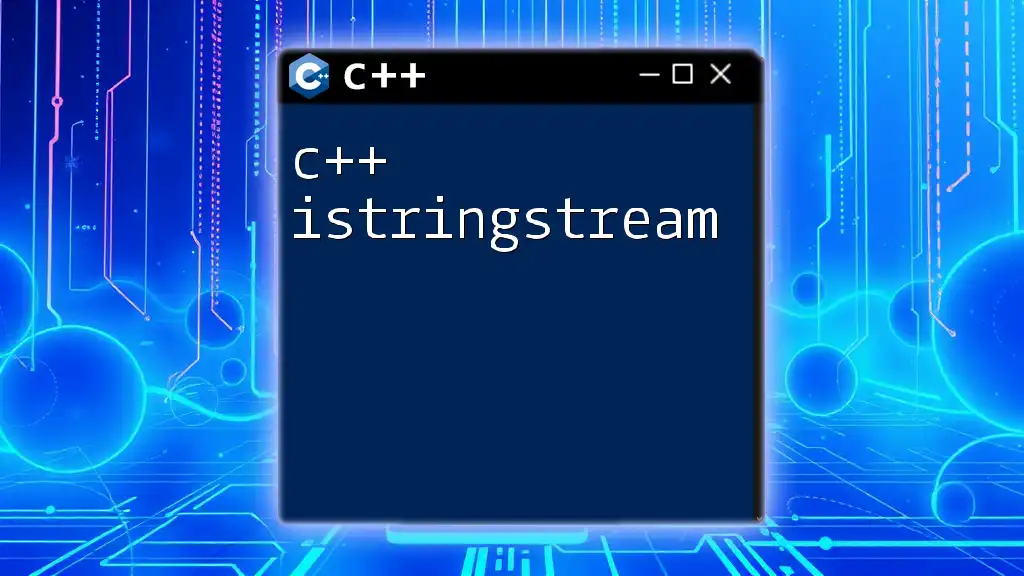
The Size of Empty and Non-Empty Strings
Empty Strings
When you create an empty string, its size will be zero. This can be crucial when working with conditions that rely on string inputs.
#include <iostream>
#include <string>
int main() {
std::string emptyStr;
std::cout << "Size of empty string: " << emptyStr.size() << std::endl; // Output: 0
return 0;
}
An empty string is helpful for initializing variables before usage.
Non-Empty Strings
The size of a non-empty string can include characters, numbers, symbols, and even spaces. For instance:
#include <iostream>
#include <string>
int main() {
std::string str = " "; // Contains spaces
std::cout << "Size of string with spaces: " << str.size() << std::endl; // Output: 3
return 0;
}
In this example, the string contains three spaces, which are counted towards its size.
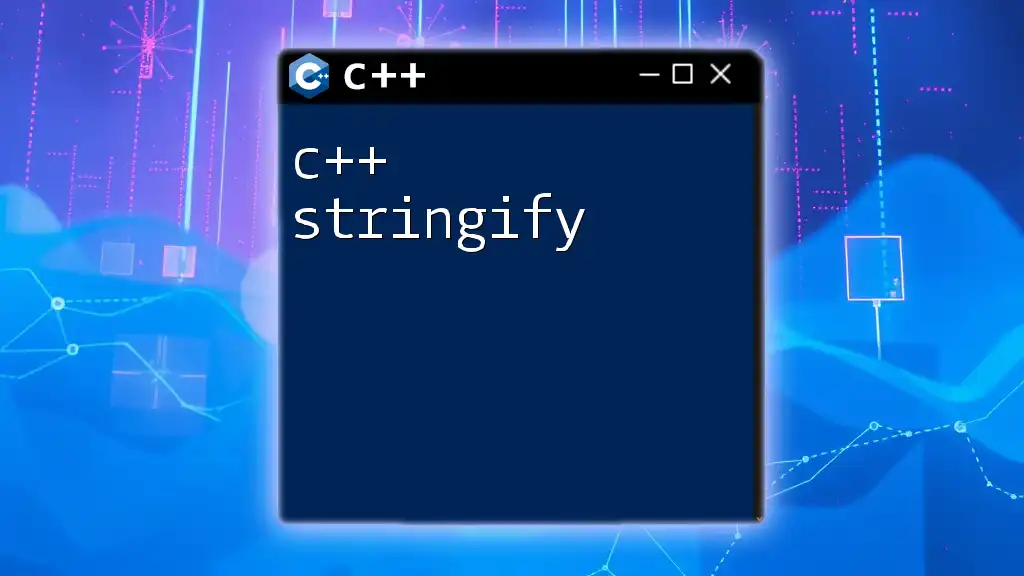
Memory Considerations
Memory Allocation for Strings
`std::string` manages memory dynamically. When you create a string, it allocates memory to hold the characters. If more characters are added than the allocated space, the string reallocates memory to accommodate the change. This process often involves copying the existing string to a new location with more space.
The Impact of String Size on Performance
As strings grow larger, performance can suffer, especially in tight loops or high-frequency calls. Keep in mind that while `std::string` is versatile, excessive use of long strings may lead to increased processing time and memory usage. Being aware of possible performance issues allows developers to write more efficient code.
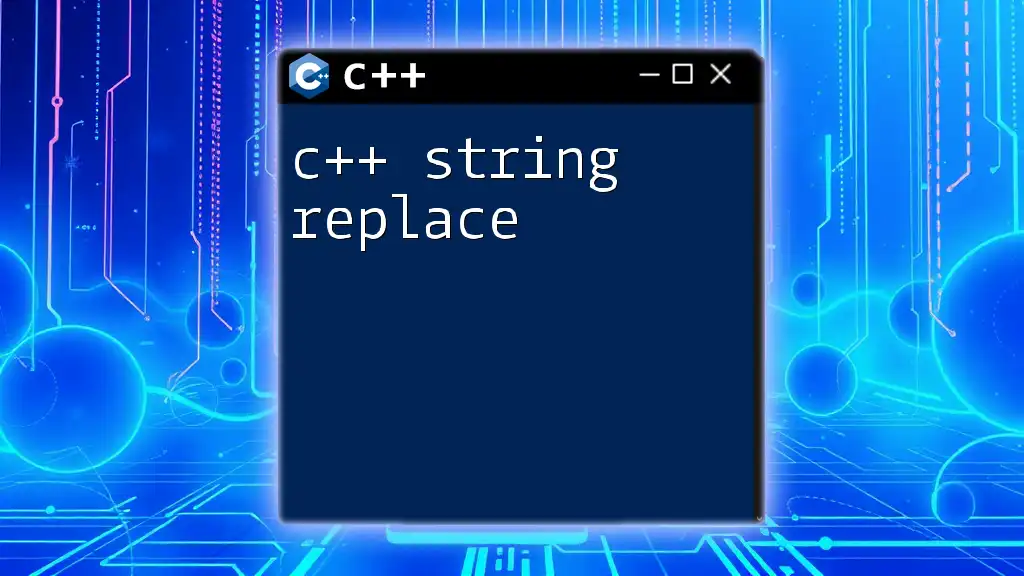
Practical Applications of String Size
Validating Input Data
In real-world scenarios, validating user input is a critical function. By checking the size of input strings, you can ensure that data adheres to specified formats or limits, preventing potential errors or misuse.
#include <iostream>
#include <string>
void validateInput(const std::string &input) {
if (input.size() > 10) {
std::cout << "Input is too long!" << std::endl;
}
}
int main() {
std::string userInput;
std::cout << "Enter a string: ";
std::cin >> userInput;
validateInput(userInput);
return 0;
}
This example demonstrates input validation by flagging strings longer than ten characters.
Managing Dynamic String Resizing
When concatenating strings, it’s crucial to understand how C++ manages string sizes and reallocates as needed.
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello";
std::string str2 = "World!";
str1 += str2; // Concatenation
std::cout << "Concatenated string size: " << str1.size() << std::endl; // Output: 12
return 0;
}
In this case, the two strings combine to form a single string "HelloWorld!", showcasing how `std::string` adjusts its size dynamically.
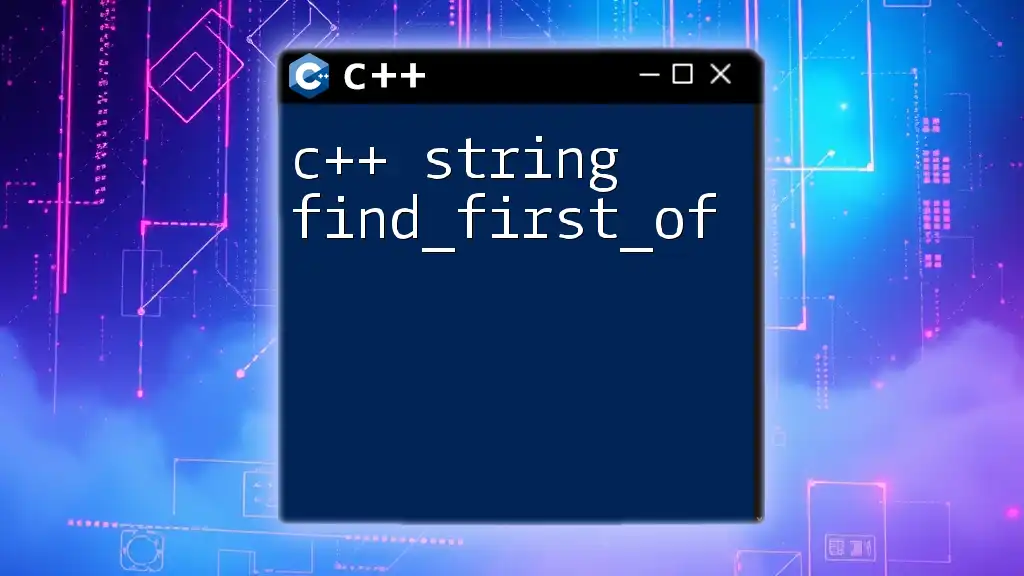
Common Mistakes and Troubleshooting
Misunderstanding String Size vs. Capacity
It’s essential to differentiate between the size and capacity of a string. The size indicates the number of characters, while capacity reflects the allocated memory to hold data. Here’s an example comparing the two:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
std::cout << "Size: " << str.size() << ", Capacity: " << str.capacity() << std::endl;
return 0;
}
Understanding this distinction can help optimize memory usage in applications.
Accessing Size Incorrectly
Sometimes, developers might mistakenly use uninitialized strings or simply ignore the possibility of empty strings, leading to unexpected behavior. Always ensure your string is initialized and valid before accessing its size.
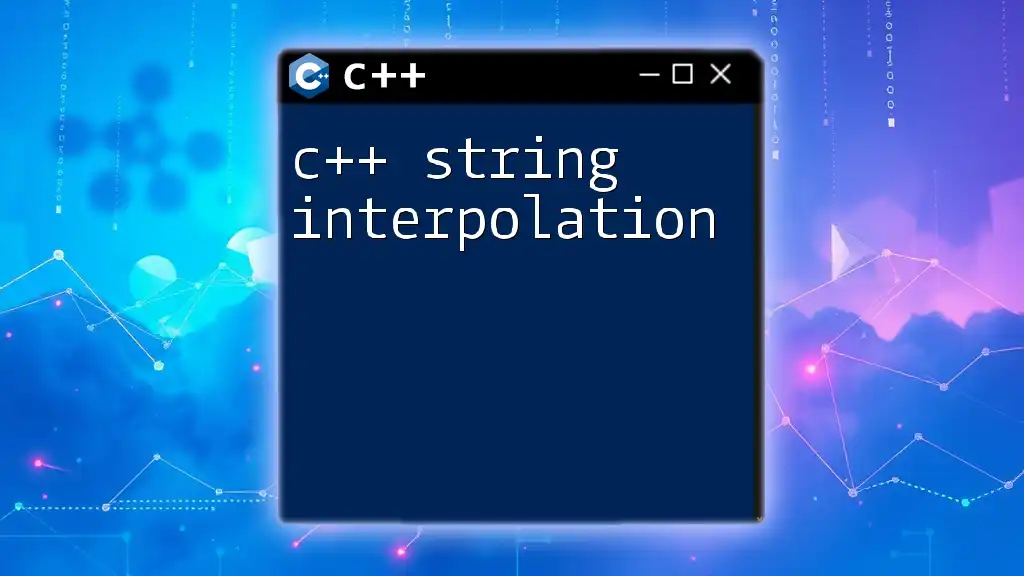
Conclusion
Understanding C++ string size is crucial for effective string manipulation and memory management. By mastering the methods to determine string sizes, validating inputs, and knowing the implications of string capacity, developers can write more robust and efficient code. As you continue to explore string handling, practice using these concepts and examples to deepen your understanding of C++.