`istringstream` is a class in C++ that allows you to read from a string as if it were a stream, making it useful for parsing formatted data.
Here’s a simple example:
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::string data = "42 3.14 Hello";
std::istringstream stream(data);
int intValue;
float floatValue;
std::string stringValue;
stream >> intValue >> floatValue >> stringValue;
std::cout << "Integer: " << intValue << ", Float: " << floatValue << ", String: " << stringValue << std::endl;
return 0;
}
What is istringstream?
`istringstream` is a part of the C++ Standard Library, specifically included in the `<sstream>` header. It allows you to treat strings as streams, enabling formatted input and output operations similar to those provided for file streams. Unlike `ostringstream` (which is used for outputting data to a string), `istringstream` is focused on reading data from strings.
When to use `istringstream`? It is particularly useful in scenarios where you need to parse data from strings—for instance, reading numbers, extracting substrings, or converting between data types. Understanding its functionality can greatly streamline your code when dealing with string manipulations.
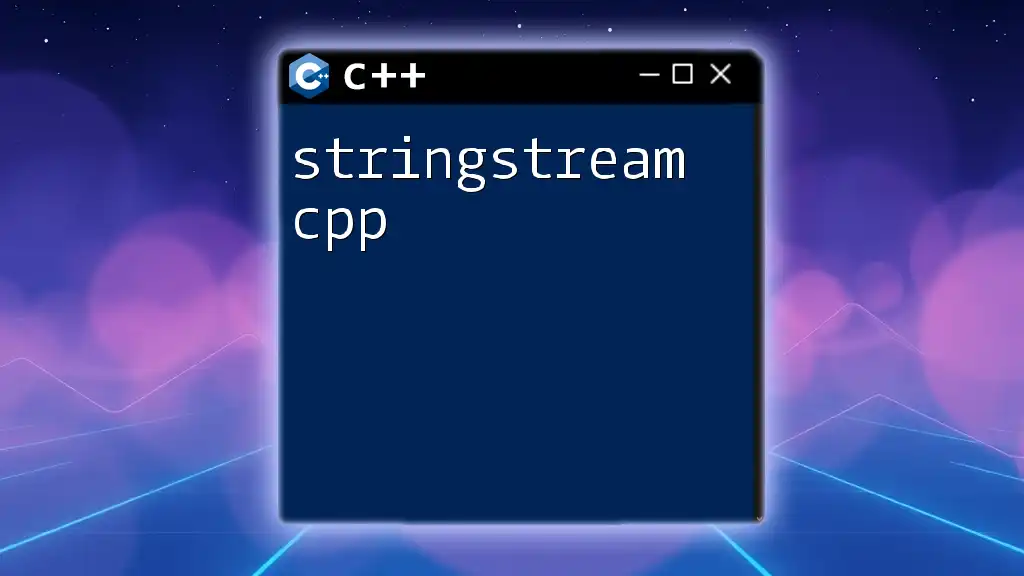
When to Use istringstream?
Here are some common situations in which using `istringstream` can be beneficial:
- Parsing user input: Easily read and interpret user-provided data.
- Reading formatted strings: Extracts values from strings that are formatted in a specific pattern.
- Converting data types: Facilitates converting strings into other data types, like integers or floats, in a safe and elegant manner.
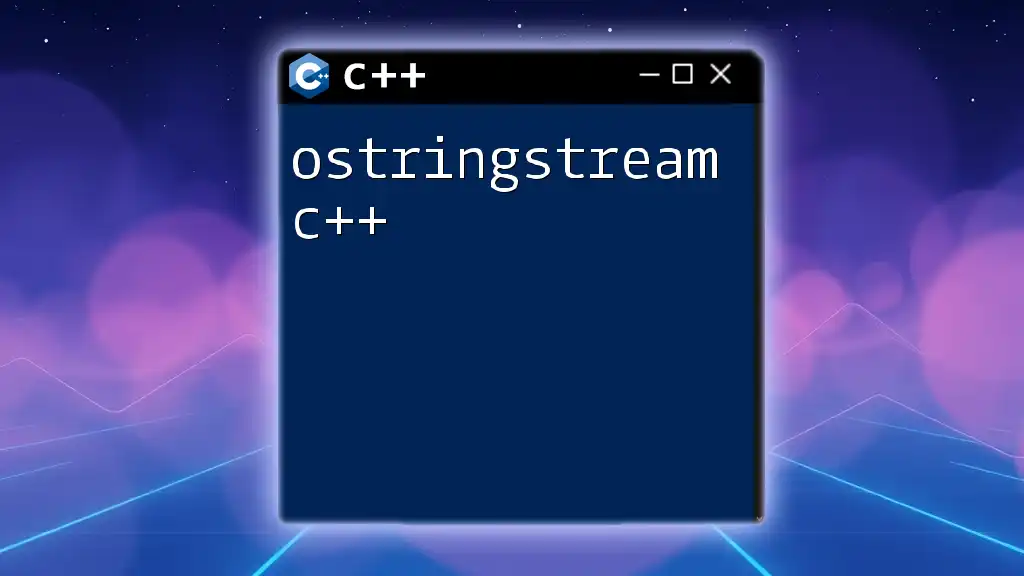
Getting Started with istringstream
Required Header
To utilize `istringstream`, you must include the following header file at the beginning of your code:
#include <sstream>
Creating an istringstream Object
Instantiating an `istringstream` object is straightforward. You can simply create an instance by passing a string to its constructor:
std::istringstream input("Example string for parsing");
Here, the string "Example string for parsing" is ready for parsing with various extraction operations.
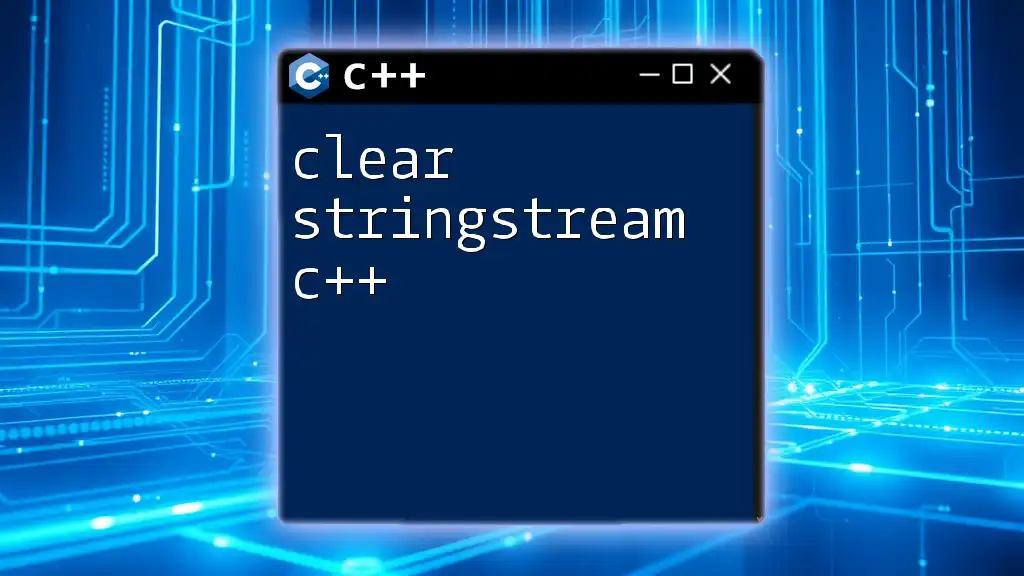
Basic Operations with istringstream
Extraction Operators
The extraction operator (`>>`) is commonly used with `istringstream` for fetching data.
Consider the example below:
std::istringstream input("100 200 300");
int a, b, c;
input >> a >> b >> c; // extracts 100, 200, and 300
In this snippet, we create an input stream initialized with three integers. The extraction operator retrieves the values into the variables `a`, `b`, and `c`. After execution, `a` contains 100, `b` contains 200, and `c` contains 300.
Handling Different Data Types
`istringstream` is versatile, allowing you to extract various data types seamlessly.
The following code demonstrates how to extract different types:
std::istringstream input("John 25 5.9");
std::string name;
int age;
float height;
input >> name >> age >> height; // extracts respective values
In this example, a string containing a name, age, and height is converted into specific data types. After executing this code, `name` holds "John", `age` is set to 25, and `height` is assigned a value of 5.9.
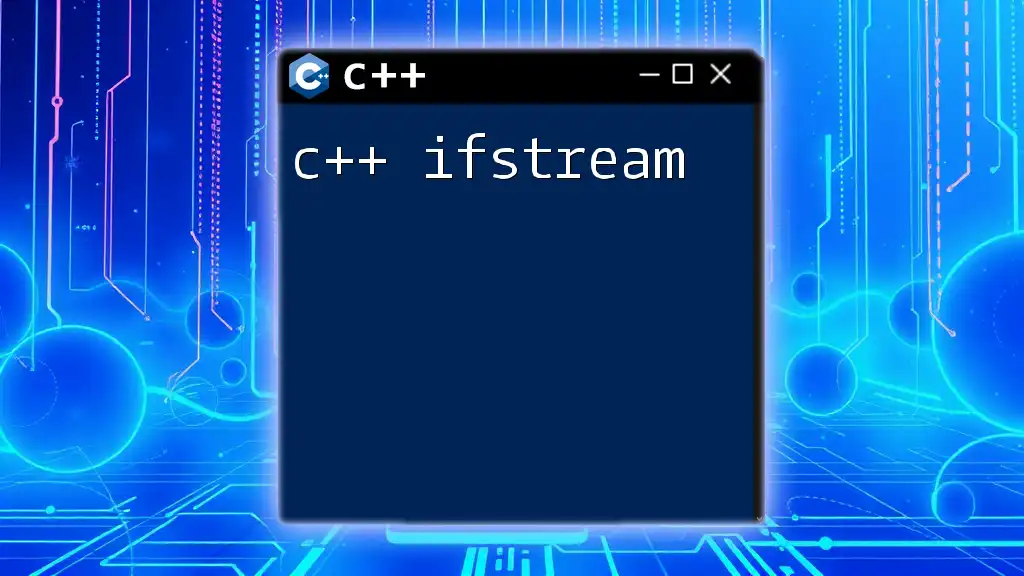
String Manipulation with istringstream
Converting String to Number
One of the most practical uses of `istringstream` is converting string representations of numbers to their respective types. This can be achieved easily:
std::istringstream ss("12345");
int number;
ss >> number; // number is now 12345
Here, a string representing a number is read into an integer variable, thus enabling numeric calculations or comparisons.
Parsing Delimited Data
You can also use `istringstream` to parse strings with specific delimiters, such as commas or spaces. For instance, to read a comma-separated list of fruits, you can use:
std::istringstream input("apple,orange,banana");
std::string fruit;
while (getline(input, fruit, ',')) {
std::cout << fruit << std::endl; // outputs each fruit
}
In this loop, `getline` effectively extracts each fruit from the input string, utilizing a comma as the delimiter. This method allows for flexible parsing of formatted data.
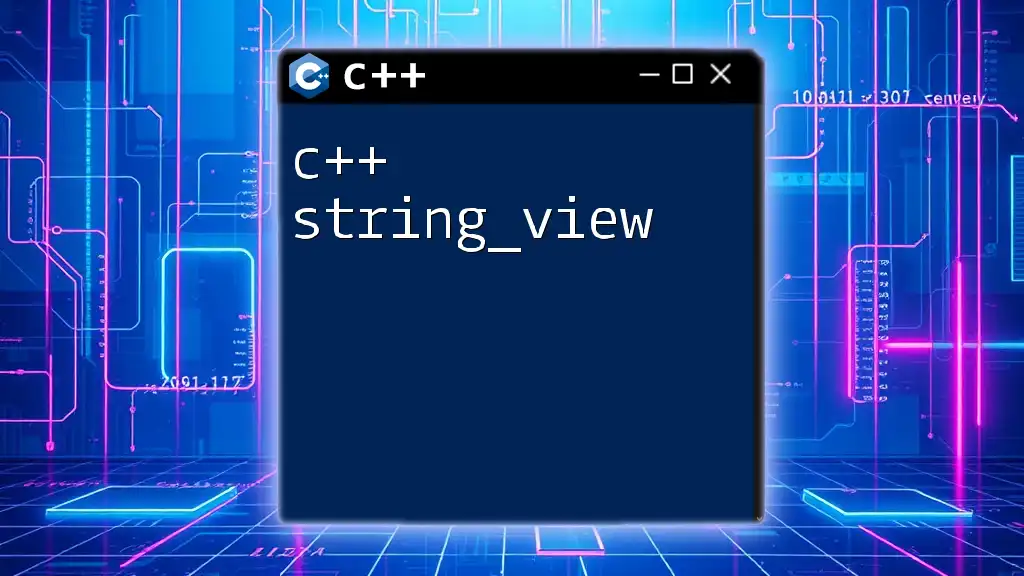
Error Handling with istringstream
Checking for Input Failures
Input operations might not always succeed. It's crucial to check for potential errors using the `fail` state:
if (input.fail()) {
std::cerr << "Input failed" << std::endl;
}
This check ensures that if the extraction fails (e.g., due to type mismatches or format issues), you are notified and can handle the situation gracefully.
Clearing and Resetting istringstream
After performing operations, you may want to reset or clear the state of the `istringstream` object so you can reuse it:
input.clear(); // Clear fail state
input.str("new string"); // Reset the string within the stream
The `clear()` function is essential for resetting the fail state, while `str()` allows you to set a new string for parsing.
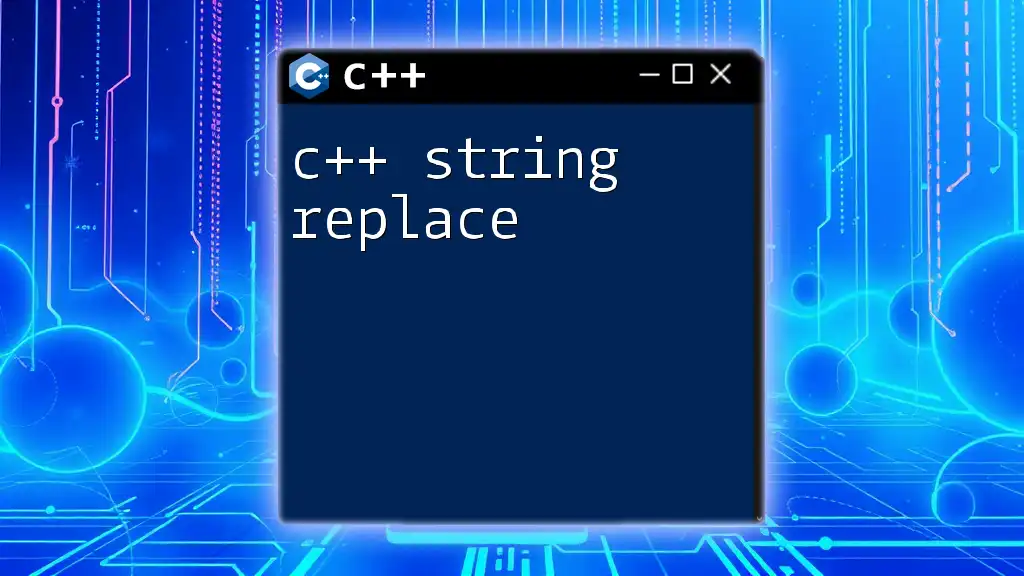
Performance Considerations
While `istringstream` provides a convenient way to handle string parsing, performance may vary compared to other methods. In performance-critical applications, consider alternatives, or use `istringstream` judiciously. However, for most everyday applications, the ease of use and readability it provides make it a solid choice.
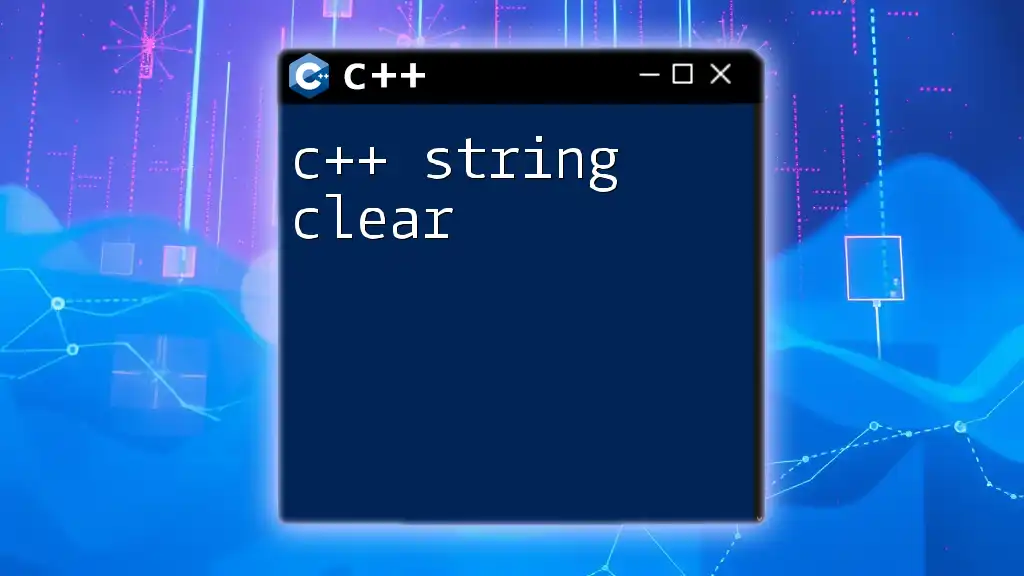
Conclusion
The `istringstream` class in C++ is a powerful tool for string manipulation, enabling you to read data formatted as strings efficiently. With capabilities to convert types, parse delimited data, and handle user inputs, it serves as a versatile resource in the C++ Standard Library. By mastering `istringstream`, you can elevate your coding skills and produce cleaner, more readable code.
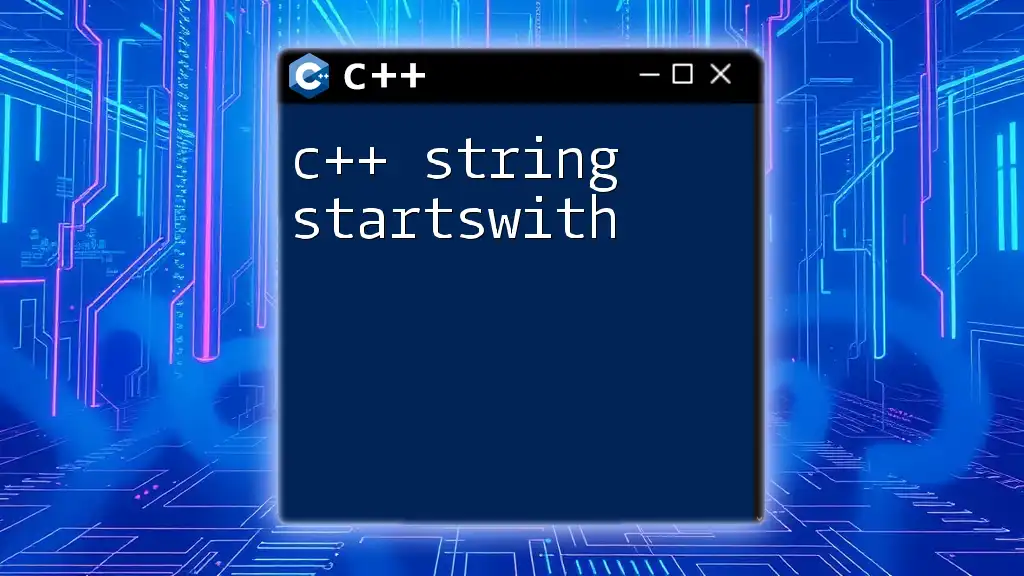
Further Reading and Resources
To dive deeper into the world of `istringstream` and related functionalities, consider consulting the following resources:
- C++ Standard Library documentation
- Tutorials and community forums
- Coding exercise platforms for hands-on practice