To clear a `stringstream` in C++, you can use the `str()` method to reset its content and `clear()` to reset its state flags. Here's a code snippet for demonstration:
#include <sstream>
#include <iostream>
int main() {
std::stringstream ss;
ss << "Hello, World!";
std::cout << "Before clear: " << ss.str() << std::endl; // Output: Hello, World!
ss.str(""); // Clear the content
ss.clear(); // Clear any error flags
std::cout << "After clear: " << ss.str() << std::endl; // Output: (empty)
return 0;
}
What is StringStream in C++?
`std::stringstream` is a class from the C++ Standard Library that allows you to use a string as a stream. This utility is crucial for various programming scenarios where input and output need to be directly manipulated as strings rather than through file I/O or standard input/output streams. By converting between strings and streams, we can easily perform operations such as reading formatted data from strings or storing strings in a dynamic manner.
Why You Might Need to Clear a StringStream
There are several situations where the need to clear stringstream C++ arises. For example, when you intend to reuse a `stringstream` for a new set of data without creating a new object, it is essential to clear its previous content. This helps to avoid unintended results from residual data, ensuring the integrity of your program.
Benefits of managing string stream content include:
- Reuse of memory by clearing existing data
- Avoiding errors from leftover data
- Enhanced performance by limiting unnecessary object creation
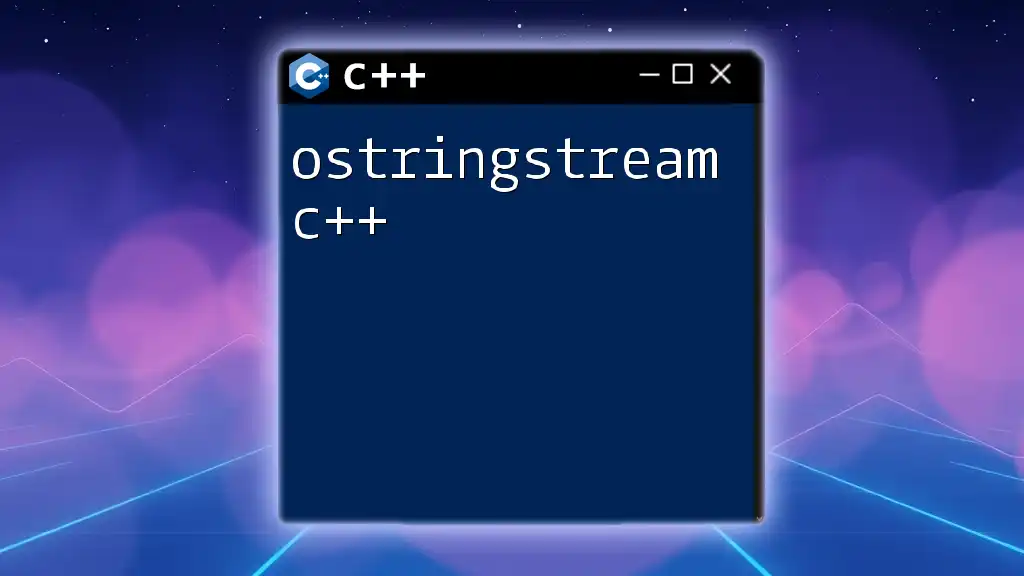
Understanding StringStream in C++
What is std::stringstream?
`std::stringstream` is a part of the `<sstream>` header and serves as an in-memory stream that allows reading from and writing to strings similar to I/O operations performed on regular streams. Distinctively, it provides flexibility in handling strings through specialized manipulation functions.
Basic Usage of StringStream
To start using `std::stringstream`, include the following header in your program:
#include <sstream>
The basic structure of creating a stringstream object is simple:
std::stringstream ss;
You can then insert data into the stringstream or retrieve data from it using standard input/output operations.
Example:
#include <sstream>
#include <iostream>
std::stringstream ss;
ss << "Hello, World!";
std::cout << ss.str() << std::endl; // Outputs: Hello, World!
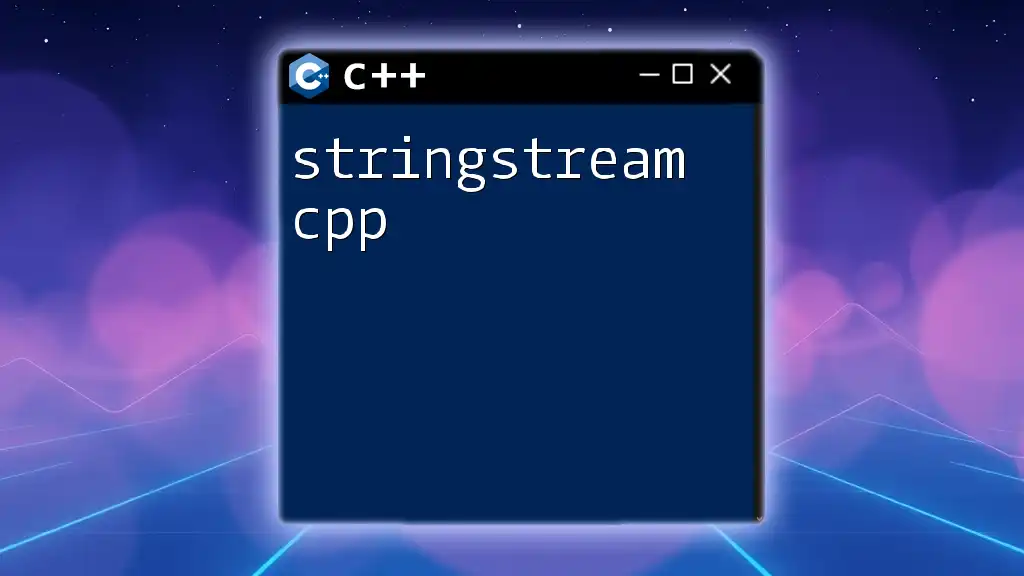
How to Clear a StringStream
Why Clear a StringStream?
Clearing a stringstream is essential for resetting its content for new input, preventing data contamination between multiple data entries. Another key reason is to manage the stream's error state after a failed input operation.
Methods to Clear StringStream
Using the `str()` Method
The `str("")` method effectively clears the contents of a stringstream by assigning an empty string to it. This action not only cleans the data but sets a new state for further input/output.
Example of clearing using `str()`:
ss.str(""); // Clear the contents
Using the `clear()` Method
The `clear()` method is used to reset the error flags of the stringstream. When a stream encounters an error (for instance, trying to extract data of an incompatible type), it enters a fail state. Calling `clear()` restores its operability, allowing you to continue using the same stream without the need for reinitialization.
Example of clearing using `clear()`:
ss.clear(); // Clear the error flags
Example: Clearing a StringStream with str()
std::stringstream ss;
ss << "Input 1";
std::cout << ss.str() << std::endl; // Outputs: Input 1
// Clear for next input
ss.str(""); // Clear the contents
ss << "Input 2";
std::cout << ss.str() << std::endl; // Outputs: Input 2
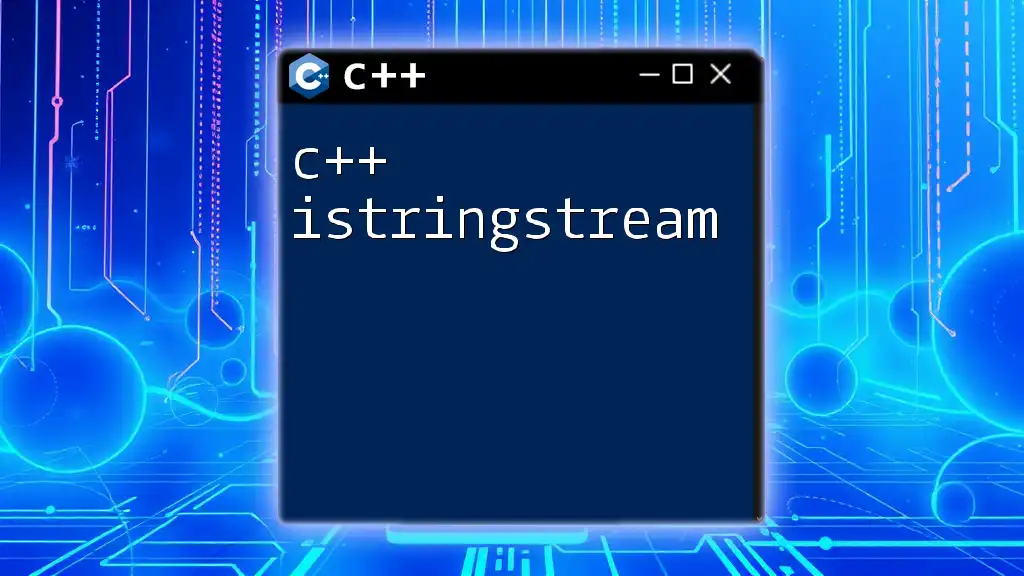
Practical Examples of Clearing StringStream
Resetting StringStream for New Input
Here’s a practical demonstration of how to properly clear and reset a stringstream for reading multiple inputs:
std::stringstream ss;
ss << "Data 1";
std::cout << ss.str() << std::endl; // Outputs: Data 1
// Resetting for new data
ss.str(""); // Clear contents
ss << "Data 2";
std::cout << ss.str() << std::endl; // Outputs: Data 2
This example highlights the utility of clearing stringstream to prevent leftover data from affecting new entries.
Handling Errors When Parsing Data
When parsing data, preventing errors is crucial. If an invalid operation causes a fail state, you can easily clear this state using both `clear()` and `str()`.
Example demonstrating error handling:
int num;
ss << "abc"; // Example input that causes failure
ss >> num; // Attempt to read integer value
if(ss.fail()) {
ss.clear(); // Clear error state
ss.str(""); // Clear contents for reuse
}
In this example, after trying to read an invalid string into an integer, we check if the operation failed. If so, we clear both the error flags and the existing data.
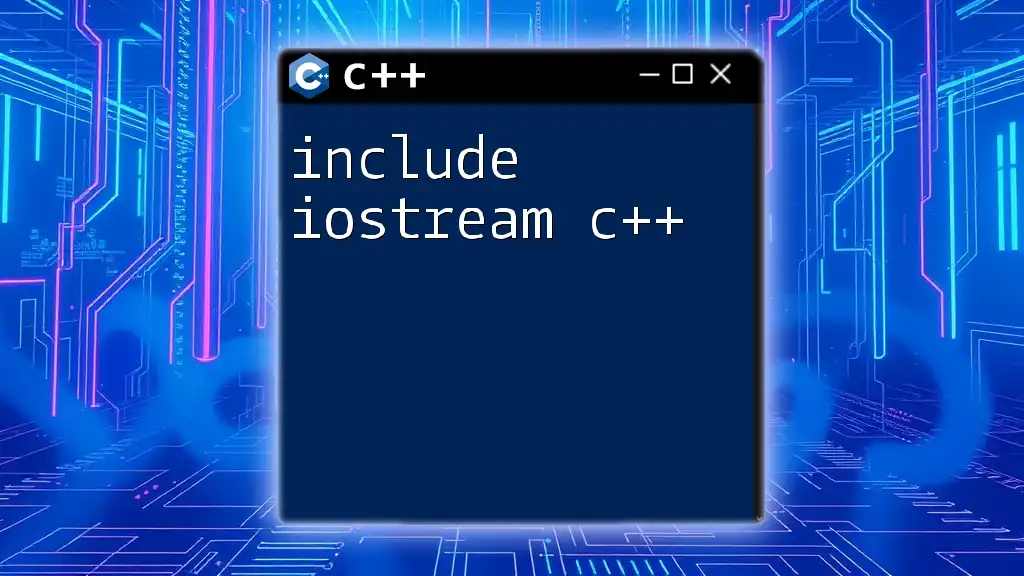
Best Practices for Using StringStream in C++
Avoiding Common Pitfalls
Here are some tips for working with stringstreams effectively.
- Always Clear Before Reuse: Before using a `stringstream` multiple times, ensure to clear the contents to prevent data from previous operations from mixing with new data.
- Check for Fail States: Always validate input operations and handle potential errors gracefully.
Performance Considerations
Repeated clearing and reusing of a single stringstream can be more efficient than creating multiple instances, especially in scenarios where performance matters. However, note that extensive usage of stringstreams can lead to potential performance bottlenecks if not managed carefully.
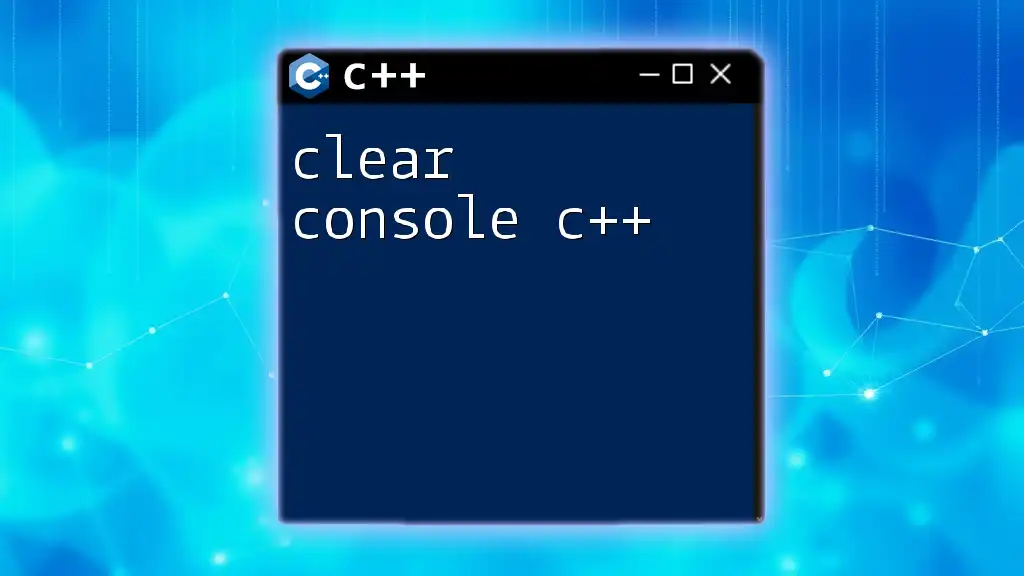
Conclusion
In summary, understanding how to clear stringstream C++ is a fundamental skill for any C++ developer. Whether you are resetting the stream for new input or handling errors efficiently, mastering these techniques will enhance your proficiency in managing string data effectively. By using methods like `str("")` and `clear()`, you can ensure your stringstreams are used efficiently and safely, paving the way for robust and error-free applications.
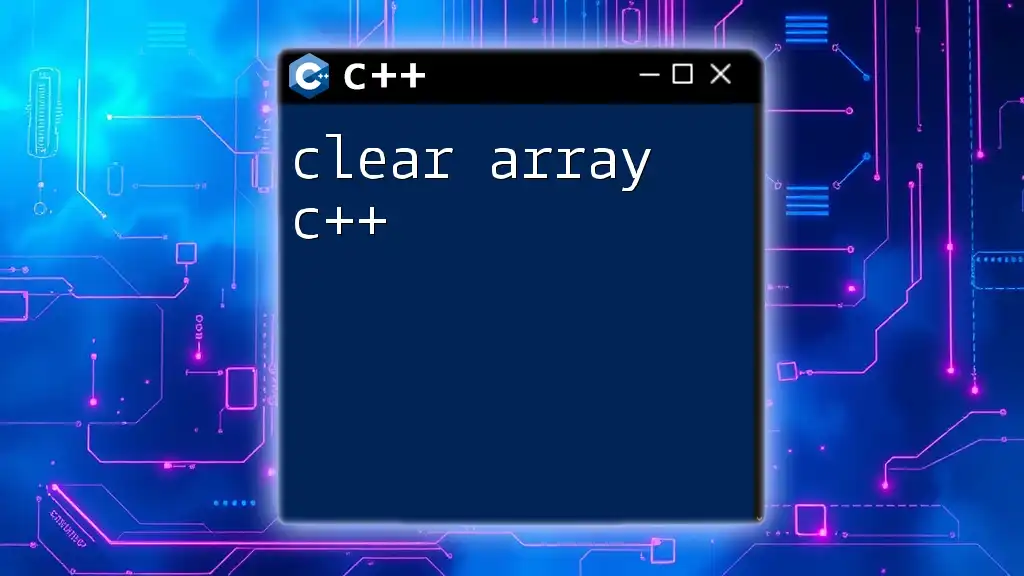
Additional Resources
Further Reading on String Manipulation in C++
To delve deeper into this subject, explore books and articles focused on C++ programming and string manipulation.
Community and Support
Engaging with communities online can offer valuable support. Websites like Stack Overflow or C++ forums can provide quick assistance when you encounter challenges in your coding journey.