A C-string in C++ is an array of characters terminated by a null character ('\0'), typically used to represent text.
Here's a simple example of declaring and initializing a C-string in C++:
#include <iostream>
int main() {
const char* cString = "Hello, World!";
std::cout << cString << std::endl;
return 0;
}
What is a C-String?
A C-string is a sequence of characters stored in contiguous memory locations, terminated by a null character (`'\0'`). This null terminator is essential as it indicates where the string ends in memory, allowing functions to correctly interpret the data.
Memory Representation
Understanding how a C-string is represented in memory is crucial for effective manipulation. A C-string is essentially an array of `char` type. For instance, if you have a C-string like `"Hello"`, the memory representation would look like this:
H e l l o \0
The null terminator is what separates the string from the subsequent memory, ensuring that the functions that operate on C-strings know when to stop reading.
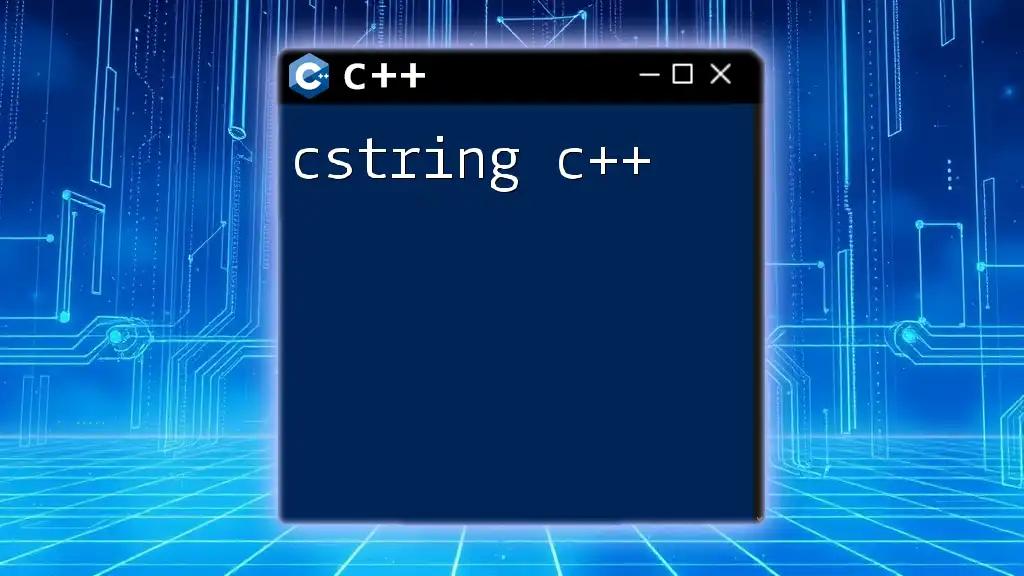
Basic Operations on C-Strings
Creating a C-String
In C++, you typically create a C-string by declaring an array of characters. You can initialize it directly, as seen in the following example:
char greeting[6] = "Hello";
Important Note: Always ensure the size of the array is large enough to hold the characters of the string along with the null terminator.
Accessing C-String Elements
Accessing individual characters of a C-string involves referencing its index. In C++, indices start from zero. For example:
char str[] = "World";
char firstChar = str[0]; // 'W'
This allows you to retrieve or modify specific characters within the string. Manipulating these elements correctly is essential for string operations.
Modifying C-String Values
Unlike the immutable nature of `std::string`, C-strings can be altered freely. Here’s an example of how to change a character within a C-string:
str[0] = 'w'; // Changes "World" to "world"
It’s an easy and direct way to make changes, but be cautious of invalid index accesses.
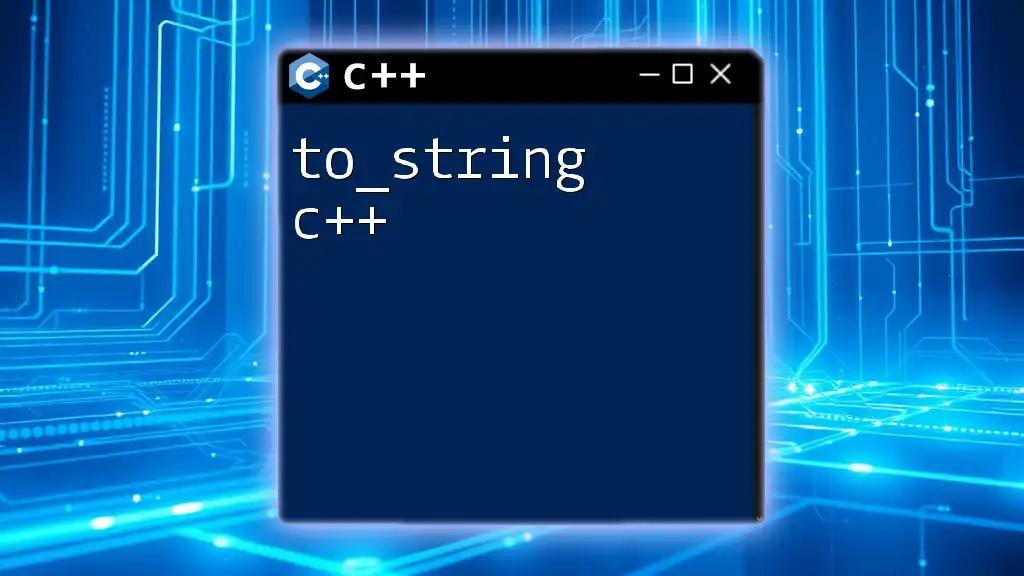
Common C-String Functions
Using `<cstring>` Library
C++ provides a number of functions for string manipulation through the `<cstring>` library (formerly `<string.h>`). Understanding these functions is vital for working with C-strings effectively.
`strlen()`
The `strlen()` function returns the length of a C-string, excluding the null terminator:
#include <cstring>
int length = strlen(str); // Returns the length of str
This is an essential function as it helps you determine how many characters are in the string.
`strcpy()`
To copy one C-string to another, you would use `strcpy()`. It’s important to ensure the destination array is large enough to hold the content you are copying:
char dest[6];
strcpy(dest, greeting); // Copies "Hello" to dest
`strcat()`
To concatenate two C-strings, `strcat()` comes in handy. It appends the second string to the end of the first:
char hello[20] = "Hello, ";
strcat(hello, "World!"); // Results in "Hello, World!"
`strcmp()`
When comparing two C-strings, `strcmp()` is the function you would use. It returns zero if the strings are equal, a negative value if the first string is less than the second, or a positive value if it is greater:
if (strcmp(hello, greeting) == 0) {
// code if strings are equal
}
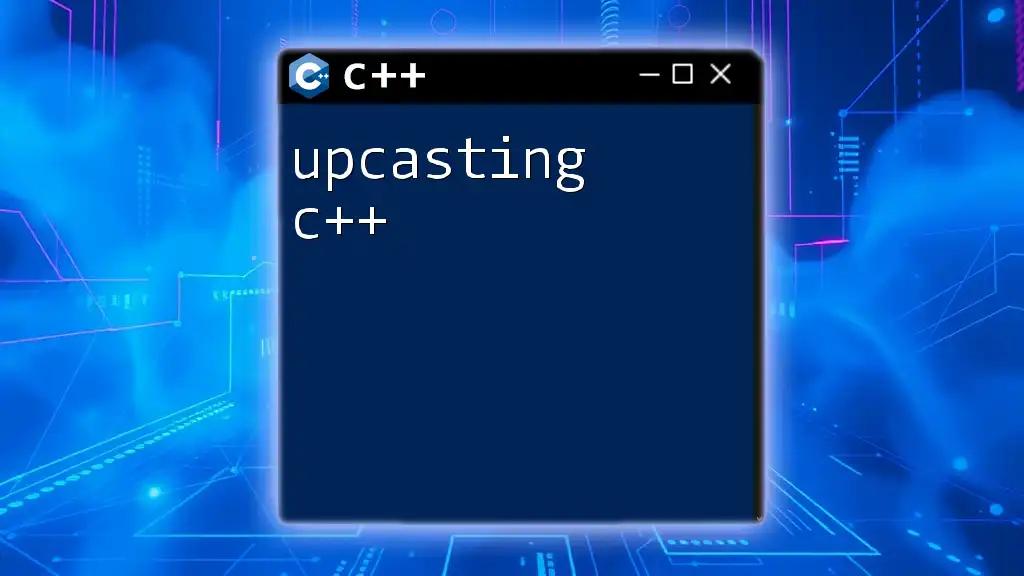
Common Pitfalls with C-Strings
Buffer Overflow
One of the most common pitfalls in manipulating C-strings is buffer overflow. This occurs when you attempt to copy or concatenate strings without ensuring the destination array is sufficiently sized. This can lead to unpredictable behavior or program crashes. Always check your lengths and sizes before performing such operations.
Null Termination Issues
Another critical issue is mishandling the null terminator. If a C-string is not properly null-terminated, functions operating on it may read past the intended end of the string, leading to potential memory access violations. For example, if you write:
char str[5] = "Hell"; // Missing '\0'
If you attempt to use `strlen()` on this string, it may continue reading memory until it finds a `'\0'`, leading to incorrect results or crashes.
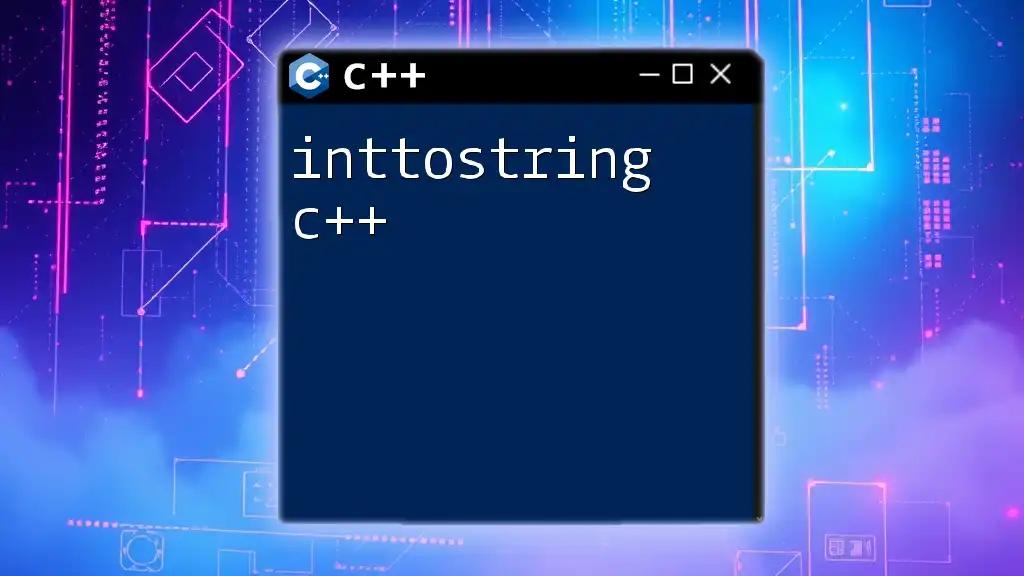
Transitioning from C-Strings to `std::string`
Benefits of Using `std::string`
Using `std::string` has several advantages over C-strings. `std::string` automatically manages memory, resizes as necessary, and simplifies concatenation and comparison. These features significantly reduce the potential for errors such as buffer overflows and null termination problems.
Converting Between C-Strings and `std::string`
Converting between `std::string` and C-strings is a common task. You can easily convert a `std::string` to a C-string using the `c_str()` method:
std::string cppStr = "Hello";
const char* cStr = cppStr.c_str(); // C-string conversion
Conversely, if you need to create a `std::string` from a C-string, you can do so directly:
char cStr[] = "World";
std::string cppStr(cStr); // Converts C-string to std::string

Conclusion
Understanding C-strings in C++ is essential for any programmer, as they are a fundamental part of C-based languages. From basic string manipulation to recognizing the common pitfalls, mastering C-strings will enhance your programming skills significantly. Always remember to practice proper string handling to avoid typical mistakes, and consider transitioning to `std::string` when it fits your needs. The flexibility and robustness of C++ provide various tools for effective string manipulation, and becoming familiar with C-strings will serve as a strong foundation for your coding journey.

Additional Resources
For those looking to deepen their understanding of C-strings and C++, numerous books and online resources are available. Engage with programming communities and forums to ask questions and share knowledge, reinforcing your learning in C++ programming.