In C++, the `sizeof` operator returns the size (in bytes) of the string object, but note that it does not return the length of the string itself; to get the actual length, you should use the `.length()` or `.size()` method instead.
Here's a code snippet to illustrate this:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
std::cout << "Size of std::string object: " << sizeof(text) << " bytes" << std::endl;
std::cout << "Length of string: " << text.length() << " characters" << std::endl;
return 0;
}
Understanding `sizeof` in C++
What is `sizeof`?
The `sizeof` operator in C++ is a vital tool that allows developers to determine the size, in bytes, of a data type or object at compile time. Its significance lies in its utility for managing memory effectively, enabling optimized code that is aware of the exact memory requirements of various data types.
How `sizeof` Works in C++
When you use `sizeof`, it calculates the size based on the type of the variable or the expression passed to it. For fundamental data types (such as `int`, `char`, `float`), `sizeof` returns consistent results across different compilers and platforms. However, the size of user-defined types, like structures or classes, may vary based on their members.
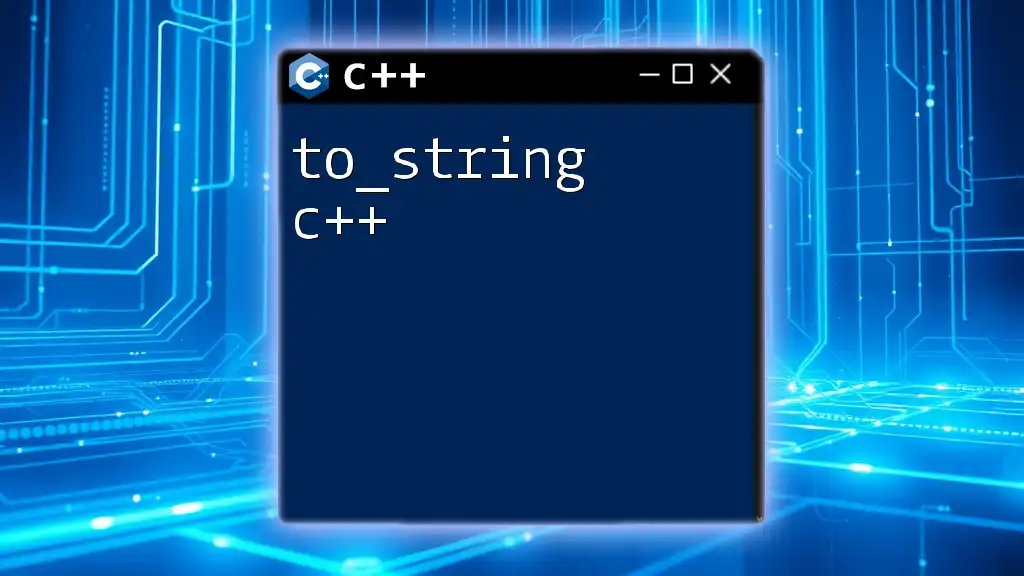
Strings in C++
Introduction to Strings in C++
In C++, strings can be represented in two primary ways: as C-style strings (null-terminated character arrays) or as instances of `std::string`, which is a part of the Standard Library. Understanding these differences is crucial when working with string data, particularly when using memory-related functions like `sizeof`.
C-style Strings vs. C++ `std::string`
C-style strings are simple arrays of characters that end with a null character (`'\0'`). They require manual memory management, including allocation and deallocation, making them prone to common issues such as buffer overflows.
On the other hand, `std::string` is a more robust option, providing dynamic memory management and a rich set of member functions that facilitate string manipulation. The advantages of `std::string` include automatic memory management, easier manipulation, and safety compared to C-style strings.
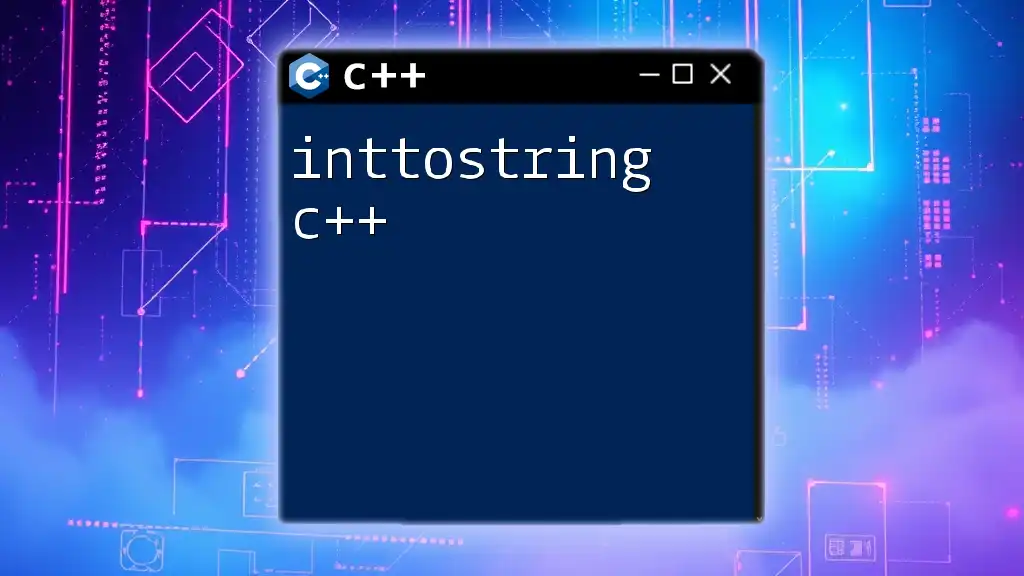
Using `sizeof` with Strings in C++
Measuring C-style Strings
When measuring C-style strings, `sizeof` yields the size of the pointer rather than the length of the actual string content. This can lead to misunderstandings. Consider the following code:
const char* cString = "Hello, World!";
std::cout << "Size of C-style string: " << sizeof(cString) << " bytes" << std::endl;
In this example, `sizeof(cString)` returns the size of the pointer (typically 4 or 8 bytes, depending on the architecture), not the length of the string `"Hello, World!"`. Therefore, using `sizeof` directly on C-style strings can be misleading.
Measuring `std::string` Objects
When it comes to `std::string`, things can be slightly different. If we take a look at this code:
std::string str = "Hello, World!";
std::cout << "Size of std::string object: " << sizeof(str) << " bytes" << std::endl;
You might expect `sizeof(str)` to return the length of the string itself. However, it only returns the size of the `std::string` object, which typically includes internal storage, pointer overhead, and metadata, not the length of the actual string content.
Why `sizeof` May Not Reflect Expected Size
Understanding the behavior of `std::string` is essential. The string's length and capacity can differ, often leading to confusion. For instance, consider the following code snippet:
std::string str = "Hello, World!";
std::cout << "Actual length: " << str.length() << " bytes" << std::endl;
std::cout << "Size of std::string object: " << sizeof(str) << " bytes" << std::endl;
Here, `str.length()` returns the number of characters in the string, while `sizeof(str)` returns the size of the object that contains information about the string, which is generally much smaller than the actual length.
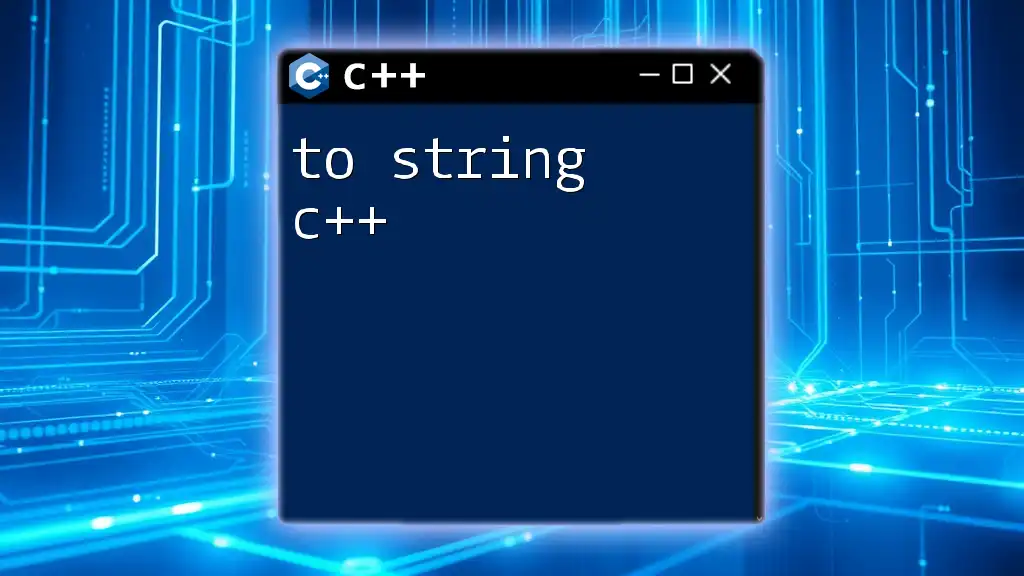
Practical Examples and Use Cases
Use Case 1: Debugging Memory
The `sizeof` operator can be crucial in identifying memory allocation issues. For example, understanding the difference in sizes between pointers and allocated memory can help pinpoint memory leaks or buffer overflows easily.
Use Case 2: Performance Optimization
When choosing between C-style strings and `std::string`, performance is often a key consideration. Owing to its flexibility and built-in functionality, `std::string` can lead to optimized code that eliminates the need for manual memory management, ultimately enhancing performance. Evaluating the impact on memory usage can be easily done with `sizeof`.
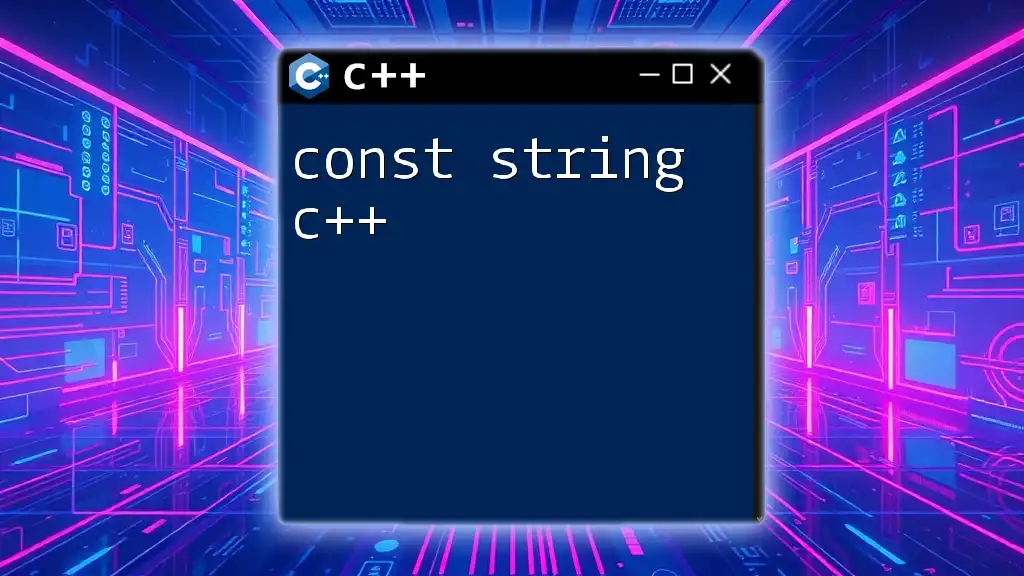
Common Mistakes to Avoid
Misunderstanding Size Calculations
One common pitfall when using `sizeof` is misinterpreting it with pointers. For example, when using `sizeof` with a pointer to an array versus the array itself, `sizeof` will provide sizes that do not correspond to the total data size held by the pointer.
Assuming Sizes are Consistent
Assuming the same size for data types across different platforms can lead to bugs. Since `sizeof` can yield varying sizes based on the compiler or architecture, it's essential to test and verify sizes in a target environment.
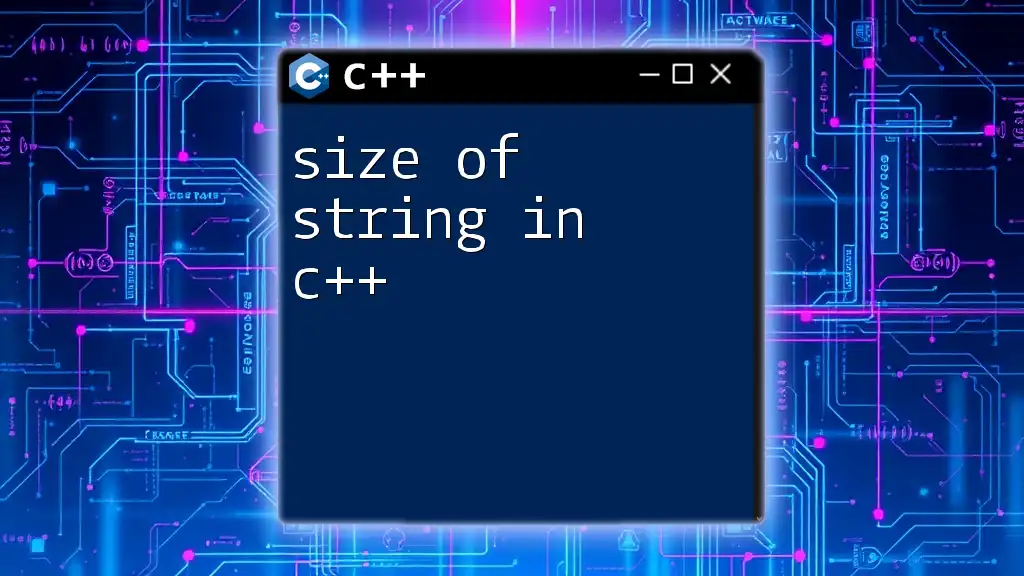
Conclusion
Recap of Key Points
In summary, when exploring the topic of `sizeof string C++`, we observed the differences between measuring C-style strings and `std::string`. Understanding how `sizeof` works in these contexts is important for effective memory management and performance optimization.
Further Learning Resources
For those interested in deepening their understanding of C++ strings and memory management, numerous resources are available, including official documentation, online tutorials, and specialized courses. These materials can enrich your knowledge and improve your programming proficiency.