In C++, a multi-line string can be created using raw string literals, which allow you to include line breaks and special characters without the need for escape sequences.
#include <iostream>
int main() {
const char* multiLineString = R"(
This is a multi-line string.
It can span multiple lines
without using escape characters.
)";
std::cout << multiLineString;
return 0;
}
Understanding C++ Multi-Line Strings
Definition and Syntax
A multi-line string in C++ is a feature that allows you to define string literals that span multiple lines in a clear and readable manner. This is primarily achieved using raw string literals, which enable you to write strings without the need for escape sequences, thereby preserving formatting as-is.
The syntax for a raw string literal is as follows:
R"delimiter(string)delimiter"
Here, `delimiter` is an optional identifier that you can choose to use if your string contains parentheses. The content inside the quotes can include new lines, tabs, and backslashes without any special handling.
Advantages of Using Multi-Line Strings
Multi-line strings in C++ offer several advantages:
-
Improved Readability: Multi-line strings make your code more readable, especially when dealing with long paragraphs or blocks of text. They visually represent the text structure, allowing programmers to discern the content quickly.
-
Easier Maintenance: With everything presented neatly in a multi-line format, maintaining and updating the strings becomes much easier.
-
Handling Complex String Data: When dealing with complex data formats (like JSON or XML), multi-line strings can be very useful, preserving the exact formatting needed without cluttering the code with escape characters.
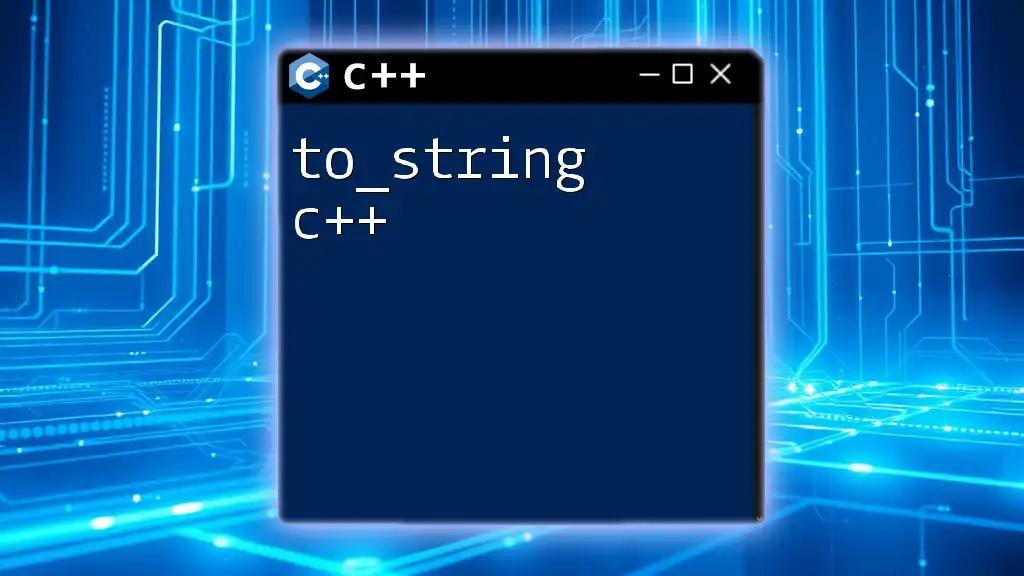
Creating Multi-Line Strings
Using Raw String Literals
Creating a multi-line string using raw string literals is straightforward. For example, you can define a multi-line string as follows:
const char* multiLine = R"(This is a multi-line
string literal that spans
across multiple lines.)";
In this snippet, the string retains its original formatting, making it easier to understand its structure when viewed in your code.
Using Escape Characters
Alternatively, you can create multi-line strings using escape sequences like `\n`. While this method works, it can quickly become hard to read, especially when many lines are involved.
const char* multiLine = "This is a multi-line\n"
"string literal that spans\n"
"across multiple lines.";
Pros and Cons: Using escape characters allows for more flexibility but may lead to reduced readability. Raw strings are cleaner and more straightforward but may feel less intuitive initially for those more accustomed to traditional string syntax.
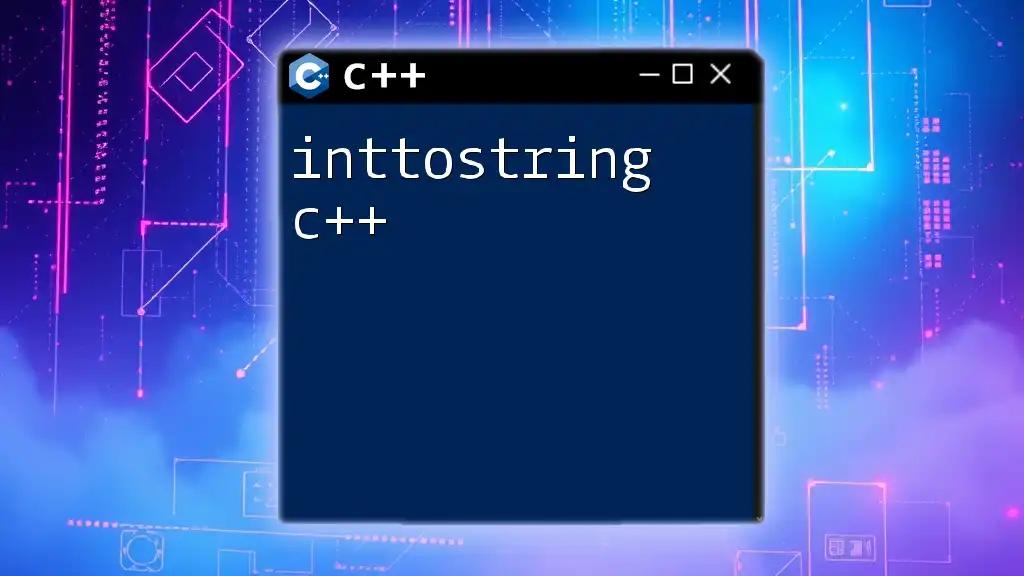
Working With Multi-Line Strings
String Manipulation Functions
C++ provides a plethora of built-in functions to manipulate strings, making it easy to work with multi-line strings. Common functions include:
- Concatenation: You can easily concatenate multi-line strings with other strings.
- Finding Length: Utilize the `.length()` method to determine the length of a multi-line string.
- Substrings: Extract portions of a multi-line string with the `.substr()` method.
Example of Multi-Line String Manipulation
Here’s a snippet that demonstrates a few string operations:
#include <iostream>
#include <string>
int main() {
std::string multiLine = R"(First line
Second line
Third line)";
std::cout << "Length of the string: " << multiLine.length() << std::endl;
std::cout << "Manipulated version: " << multiLine + R"( Fourth line)" << std::endl;
return 0;
}
In this code, we define a multi-line string and print its length. We also demonstrate how to concatenate another string easily. The resulting output illustrates the seamless integration of multi-line strings into existing text.
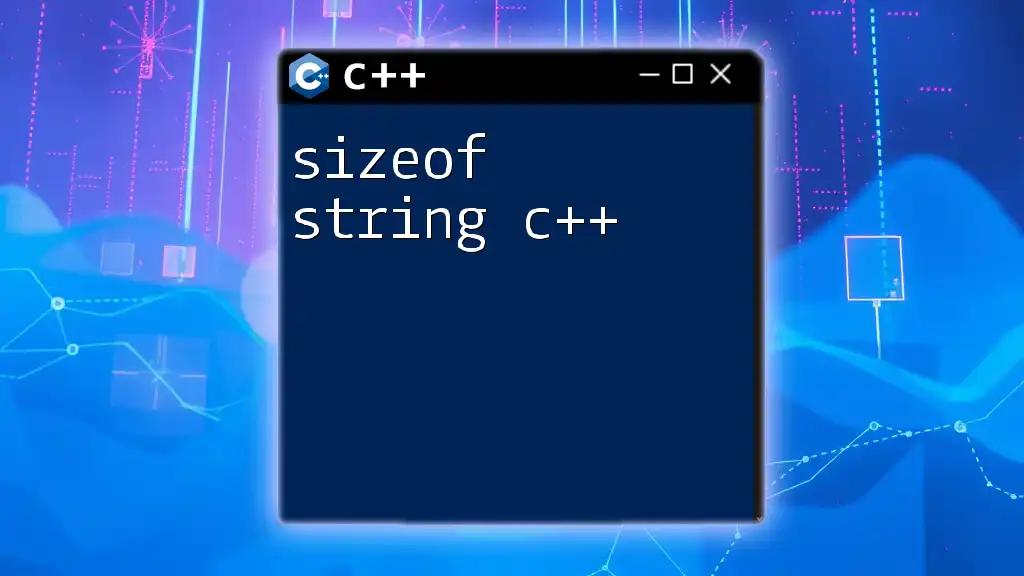
Practical Applications of Multi-Line Strings
Using Multi-Line Strings in File I/O
Multi-line strings can be incredibly useful when dealing with file input/output operations. For instance, when writing a block of text into a file, you could do the following:
#include <fstream>
int main() {
std::ofstream myfile("example.txt");
myfile << R"(Line 1: Hello,
Line 2: Welcome to
Line 3: Multi-line strings in C++.)";
myfile.close();
return 0;
}
This example creates a text file and writes a multi-line string into it, demonstrating how easily text can be formatted and manipulated with multi-line strings.
Multi-Line Strings in Console Output
Displaying messages in a user-friendly way is another practical application for multi-line strings. Here’s how you might present an error message:
#include <iostream>
int main() {
std::cout << R"(Error: Invalid input.
Please check your data and try
again.)" << std::endl;
return 0;
}
This method simplifies the process of organizing output in a manner that’s easy to read, thereby enhancing user experience.

Common Pitfalls and Best Practices
Pitfalls When Working With Multi-Line Strings
While working with multi-line strings, several common pitfalls may arise:
-
Misunderstanding Raw String Syntax: It’s essential to grasp how the delimiters work. Incorrectly placing or omitting them can lead to compilation errors.
-
Forgetting to Escape Backslashes: In strings that do not utilize raw literals, backslashes must be escaped, which can lead to mistakes and confusion.
-
Mixing Raw and Regular Strings Incorrectly: This can lead to unintended behavior and surprises in string handling.
Best Practices
To maximize the utility of multi-line strings, consider the following best practices:
-
When to Use Multi-Line Strings: Generally, opt for multi-line strings when dealing with large blocks of text, as they offer better readability and maintainability.
-
Tips for Formatting Multi-Line Strings: Consistently align your code for better readability. If using raw strings, keep the content organized to reflect the logical structure of your output or text.

Conclusion
In conclusion, understanding and utilizing multi-line strings in C++ is essential for writing clean, maintainable, and readable code. These strings facilitate the handling of complex data structures and enhance code documentation through clear formatting. As you practice working with multi-line strings, you'll discover newfound efficiency and effectiveness in your programming projects.

Call to Action
Share your experiences with multi-line strings and how they've improved your coding practices. If you're interested in advanced techniques, explore related topics such as string manipulation functions and C++ grammar concepts to deepen your understanding.
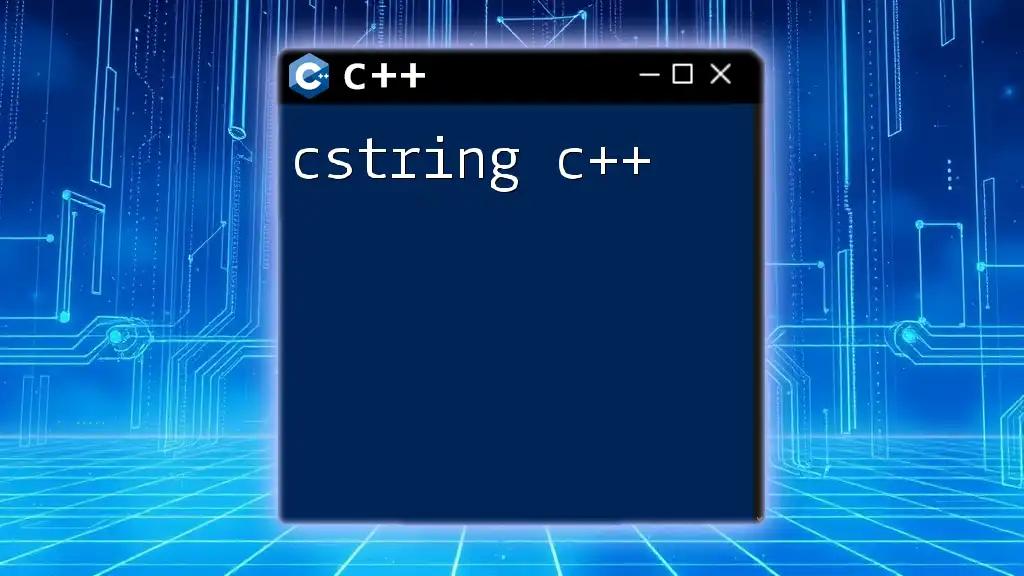
Additional Resources
For further reading, refer to reputable C++ documentation and tutorials on string manipulation to continue enhancing your programming skills.