Multi-threading in C++ allows multiple threads to run concurrently, enabling better resource utilization and improved performance in applications. Here’s a simple example using the C++11 standard:
#include <iostream>
#include <thread>
void sayHello() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
std::thread t(sayHello);
t.join(); // Wait for the thread to finish
return 0;
}
Understanding Threads
What is a Thread?
A thread is the smallest unit of processing that can be scheduled by the operating system. It exists within a process — a running instance of a program — and threads provide a way to perform multiple tasks concurrently. By enabling concurrent operations, multi-threading improves application performance, especially in systems with multiple processors or cores.
Process vs. Thread
To understand the distinction between processes and threads:
- A process is an independent program that contains its own memory space and resources.
- A thread, on the other hand, shares the same memory space with other threads within its process, making it lightweight and more efficient in executing concurrent tasks.
For instance, in a web browser, each tab can be considered a thread of a single process, facilitating tasks like page loading and rendering while sharing resources like memory.
Creating a Thread in C++
In C++, creating a thread is straightforward using the thread library introduced in C++11. Here’s how you can do it:
Include the necessary header files like `<thread>` and `<iostream>`, which provide functions and classes for managing threads.
The following example illustrates a simple way to create and start a thread:
#include <iostream>
#include <thread>
void printHello() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
std::thread t(printHello);
t.join(); // Wait for the thread to finish
return 0;
}
In this example, the `printHello` function runs on the new thread. The `join()` method waits for the thread to finish before continuing the execution of the program.

Thread Management
Joining Threads
When you create threads, it's important to manage their execution. One way to do this is through the `join()` function. This function blocks the calling thread until the thread associated with it has finished execution.
Consider the following example:
void printNumbers(int x) {
for (int i = 1; i <= x; i++)
std::cout << i << " ";
}
int main() {
std::thread t1(printNumbers, 5);
std::thread t2(printNumbers, 3);
t1.join();
t2.join();
return 0;
}
Here, two threads run concurrently, each printing numbers. The main thread waits for both `t1` and `t2` to finish before it exits.
Detaching Threads
Instead of joining threads, you may choose to detach them using the `detach()` function. This allows the thread to run independently. Once detached, you cannot join the thread, and it will continue executing until its function completes.
Here’s an example:
void printHello() {
std::cout << "Hello from detached thread!" << std::endl;
}
int main() {
std::thread t(printHello);
t.detach(); // Now the thread runs independently
// Note: t cannot be joined now
return 0;
}
Important Note: Be cautious while using detached threads, as it can lead to resource management challenges if not handled properly.

Synchronization in Multi-threading
What is Synchronization?
In a multi-threaded environment, multiple threads may attempt to access and modify shared data simultaneously. This can lead to data races — situations where the timing of threads affects the correctness of the program. To prevent these issues, synchronization is crucial.
Mutex in C++
A mutex (short for mutual exclusion) helps manage access to shared data by allowing only one thread to access a piece of data at a time. The C++ Standard Library provides `std::mutex` for this purpose.
Here’s an example of how to use a mutex to protect shared data:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
void safePrint(int x) {
mtx.lock(); // Lock the mutex before accessing shared resource
std::cout << x << std::endl;
mtx.unlock(); // Unlock the mutex after accessing
}
int main() {
std::thread t1(safePrint, 1);
std::thread t2(safePrint, 2);
t1.join();
t2.join();
return 0;
}
By locking and unlocking the mutex, we ensure that the `safePrint` function can only be accessed by one thread at a time, preventing data races.
Lock Guards
To make mutex management easier and less error-prone, C++ offers `std::lock_guard`, which automatically locks the mutex upon instantiation and releases it when the `lock_guard` goes out of scope.
Here’s an example of using `lock_guard`:
void safePrint(int x) {
std::lock_guard<std::mutex> lock(mtx); // Automatically locks the mutex
std::cout << x << std::endl;
}
Using `lock_guard` helps avoid forgetting to unlock the mutex, thereby minimizing the risk of deadlocks.

Advanced Multi-threading Concepts
Condition Variables
Condition variables enable threads to communicate about the occurrence of events, allowing threads to wait until a particular condition is met.
Here's how you might use a condition variable in C++:
#include <iostream>
#include <thread>
#include <condition_variable>
#include <mutex>
std::condition_variable cv;
std::mutex m;
bool ready = false;
void waitForWork() {
std::unique_lock<std::mutex> lck(m);
cv.wait(lck, [] { return ready; }); // Wait until ready is true
// Process work
}
void workReady() {
std::unique_lock<std::mutex> lck(m);
ready = true;
cv.notify_one(); // Notify one waiting thread
}
In this snippet, `waitForWork()` waits until `ready` becomes true, while `workReady()` notifies the waiting thread, allowing it to proceed.
Thread Pools
Creating and destroying threads has overhead. A common approach to address this is by using a thread pool, which is a collection of pre-instantiated threads ready for execution. Thread pools manage a queue of tasks and distribute these tasks among the threads in the pool.
Implementing a basic thread pool can enhance performance by reusing threads instead of creating new ones for every task.

Best Practices for Multi-threading in C++
Avoiding Data Races
To ensure thread safety:
- Always protect shared resources with mutexes or other synchronization primitives.
- Minimize the sharing of mutable state whenever possible.
Deadlock Prevention
Deadlocks can occur when two or more threads are blocked forever, waiting for each other to release resources. To prevent deadlock:
- Always acquire locks in the same order across different threads.
- Use timed locks that attempt to acquire a lock for a given period before giving up.
Performance Considerations
When implementing multi-threading, measure and optimize performance. Some key considerations include:
- Profiling multi-threaded applications to identify bottlenecks.
- Considering the overhead of locking mechanisms. Aim for low lock contention.
- Evaluate if the complexity of multi-threading outweighs its benefits for your application.
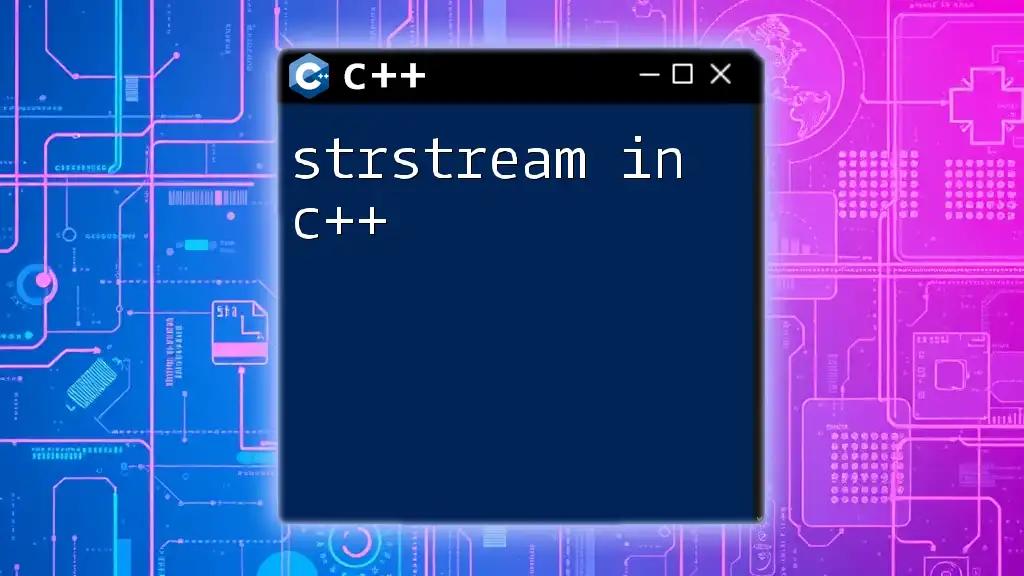
Conclusion
Multi-threading is a powerful feature in C++ that enhances application performance by allowing concurrent execution. With the right understanding of threads, synchronization, and management techniques, you can develop efficient and safe applications. Make sure to practice and explore different scenarios in multi-threading to leverage its full potential.