The `std::thread` in C++ allows you to run tasks concurrently by creating a new thread of execution, enabling efficient use of multi-core processors.
Here’s a simple code snippet demonstrating how to create and run a thread:
#include <iostream>
#include <thread>
void hello() {
std::cout << "Hello from the thread!" << std::endl;
}
int main() {
std::thread t(hello); // Create and run the thread
t.join(); // Wait for the thread to finish
return 0;
}
What is `std::thread`?
`std::thread` is a C++ Standard Library class that enables the creation and management of threads. A thread represents an independent sequence of execution within a program, allowing multiple operations to occur simultaneously. This is crucial in modern applications where performance and responsiveness are key, particularly in scenarios involving user interfaces or tasks that can run concurrently.
Introduced in C++11, `std::thread` provides a portable and straightforward interface for creating threads and executing functions concurrently.
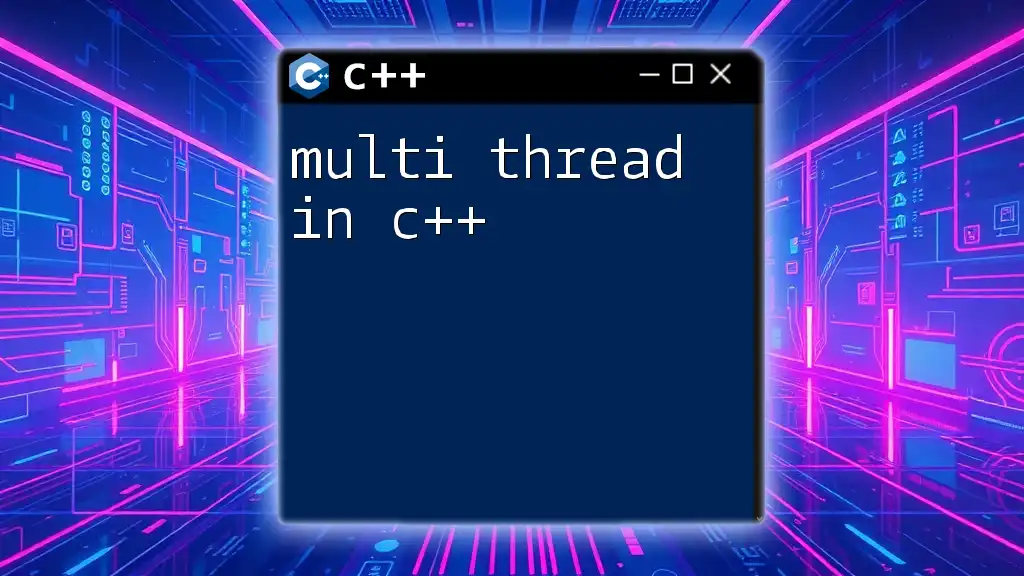
Benefits of Using `std::thread`
Utilizing `std::thread` brings numerous advantages to application development:
- Improved Performance: By executing tasks in parallel, applications can significantly reduce execution time, especially for CPU-bound operations.
- Better Resource Utilization: It allows for better utilization of multi-core processors, leveraging concurrent execution to make the most of hardware capabilities.
- Responsive User Interfaces: For applications with GUIs, separating the user interface thread from computational work enables smooth operation and responsiveness.
- Real-world Applications: Think of web servers, gaming applications, and data processing tasks. They all benefit from the concurrent execution capabilities that `std::thread` provides.
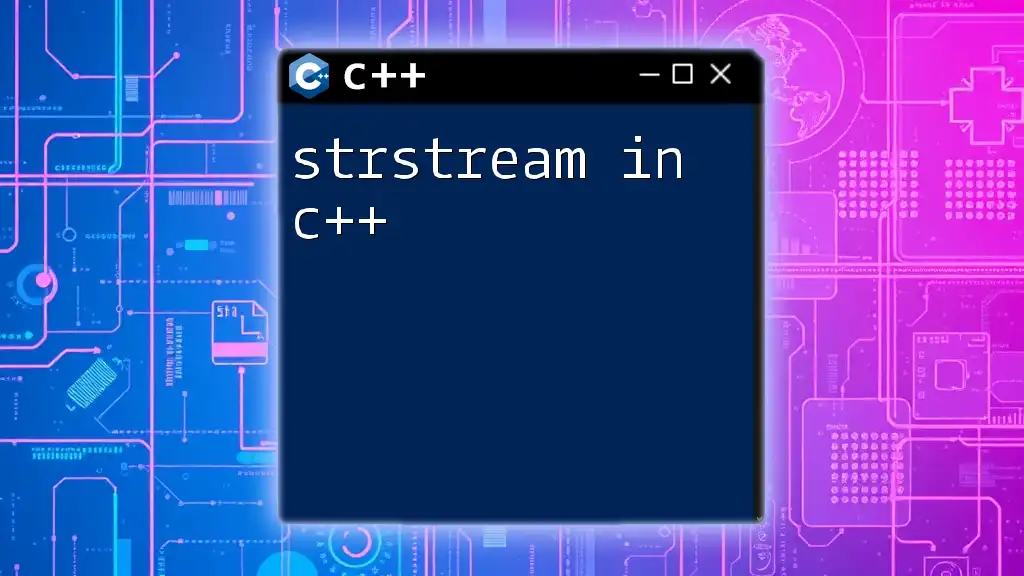
Setting Up Your Environment
To get started with using `std::thread`, ensure you meet the following requirements:
- You need to use C++11 or later.
- Ensure your compiler supports `std::thread`. Popular choices include GCC, Clang, and MSVC.
Once your environment is set up, you can create a sample project that incorporates threading to understand its functionalities better.
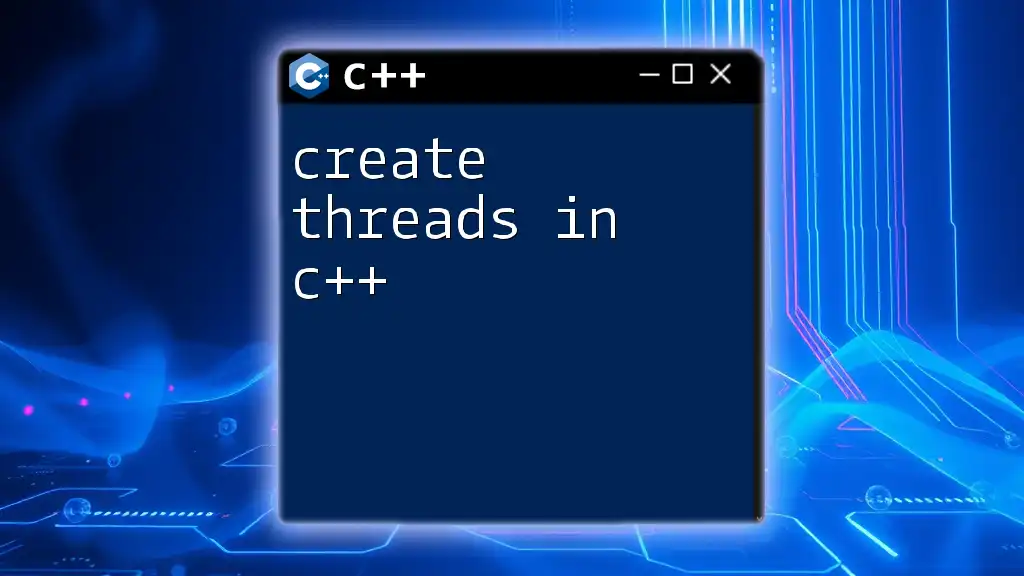
Creating a Basic Thread
Basic Syntax of `std::thread`
Creating a thread in C++ with `std::thread` is straightforward. The primary step involves instantiating a `std::thread` object and passing the function to be executed.
Here’s a simple code snippet demonstrating basic thread creation:
#include <iostream>
#include <thread>
void hello() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
std::thread t(hello); // Create a thread
t.join(); // Wait for the thread to finish
return 0;
}
In this example, the `hello` function runs on a separate thread. The `join()` method is crucial here; it ensures the main thread waits for the spawned thread to complete its execution before proceeding.
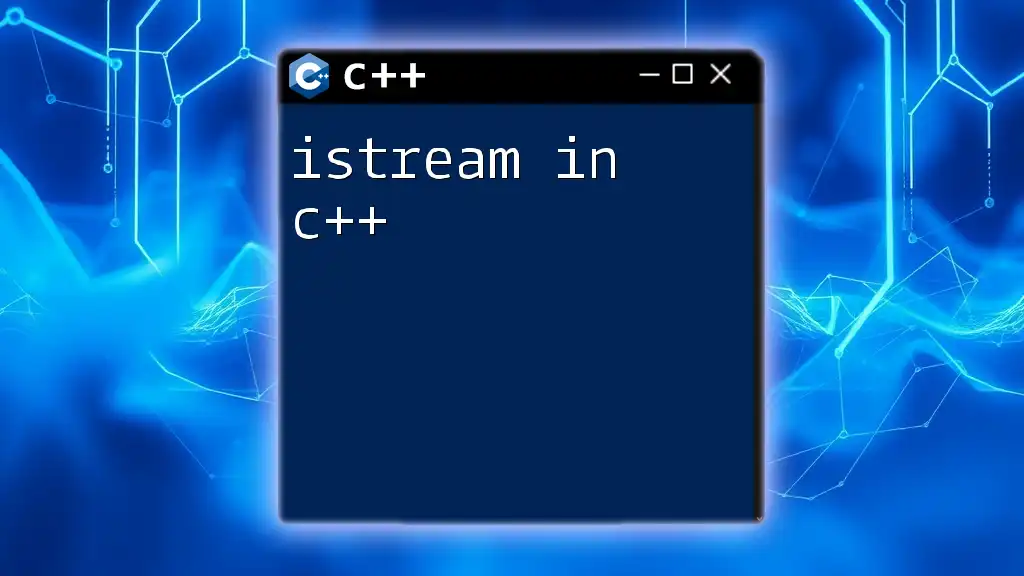
Managing Threads
Starting and Joining Threads
When you create a thread, it immediately starts executing the provided function. However, managing its lifecycle—particularly waiting for it to finish—is essential. This is where the `join()` method comes into play.
If you call `join()`, the main thread will block until the thread represented by the `std::thread` object completes. On the other hand, if you want the thread to continue running independently, you can use `detach()`.
Detaching Threads
Detaching a thread allows it to run freely in the background, independent of the main thread. While this can be useful, beware that you lose control over the detached thread, including its lifetime.
Here’s an example of using `detach()`:
void detached_function() {
std::cout << "This will run in the background!" << std::endl;
}
int main() {
std::thread d_thread(detached_function);
d_thread.detach(); // The thread continues independently
// Main thread execution
std::this_thread::sleep_for(std::chrono::seconds(1)); // Ensure the background thread has time to run.
return 0;
}
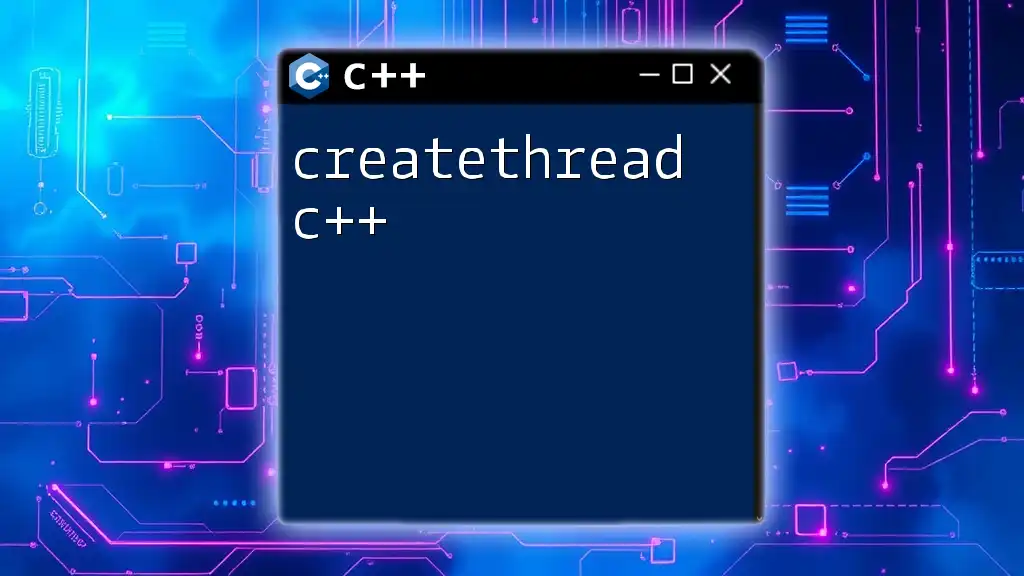
Passing Arguments to Threads
When creating threads, you might want to pass arguments to the functions they will execute.
Passing Basic Types
Passing simple types like int or char is direct. You can simply provide them as additional arguments to the `std::thread` constructor:
void print_number(int n) {
std::cout << "Number: " << n << std::endl;
}
int main() {
std::thread t(print_number, 5);
t.join();
return 0;
}
Passing Complex Data Types
You can also pass complex data structures, such as structs or classes. Here’s an example:
struct Data {
int x;
};
void process_data(Data data) {
std::cout << "Processing: " << data.x << std::endl;
}
int main() {
Data data = {10};
std::thread t(process_data, data);
t.join();
return 0;
}
When passing complex types, be cautious of copying overhead and side effects. In many cases, it may be better to use references or pointers.
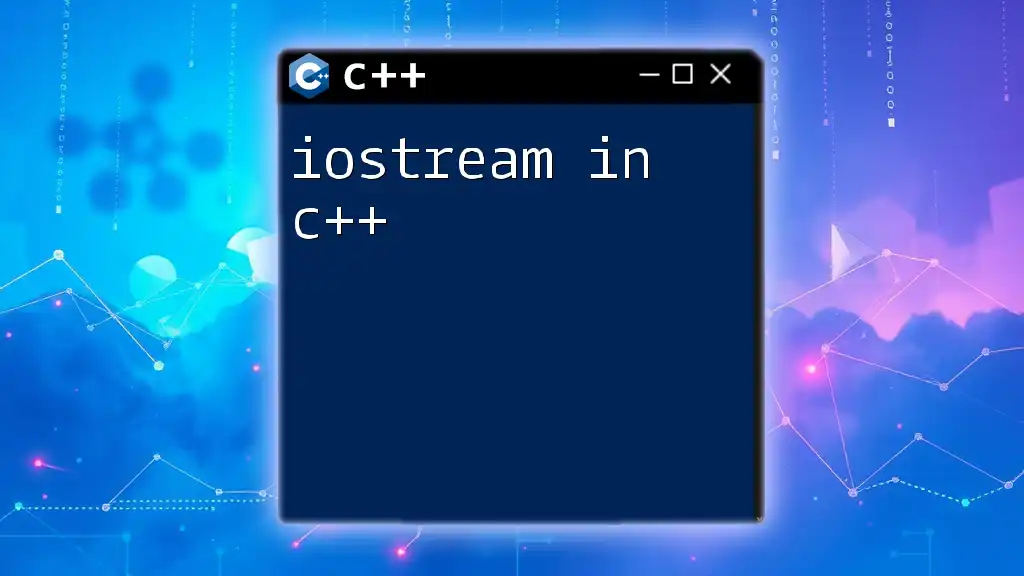
Thread Safety and Synchronization
Understanding Race Conditions
A race condition occurs when two or more threads attempt to access shared data simultaneously, leading to unpredictable results. This can cause your program to behave in ways you might not expect.
Using Mutexes for Protection
To prevent race conditions, you can use `std::mutex`, which provides a way to protect shared data. Here’s how:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
void safe_increment(int &n) {
std::lock_guard<std::mutex> lock(mtx); // Locks the mutex
++n;
}
int main() {
int counter = 0;
std::thread t1(safe_increment, std::ref(counter));
std::thread t2(safe_increment, std::ref(counter));
t1.join();
t2.join();
std::cout << "Final Counter: " << counter << std::endl; // Safe increment
return 0;
}
In this example, `std::lock_guard` automatically locks the mutex when it goes out of scope, ensuring that increments to `counter` are thread-safe.
Condition Variables
To handle scenarios where threads need to wait for certain conditions before proceeding, you can use `std::condition_variable`. This can help with managing thread states effectively.
For example, a producer-consumer scenario can utilize a condition variable to notify consumers when data is available. You can find plenty of resources explaining this pattern in detail.
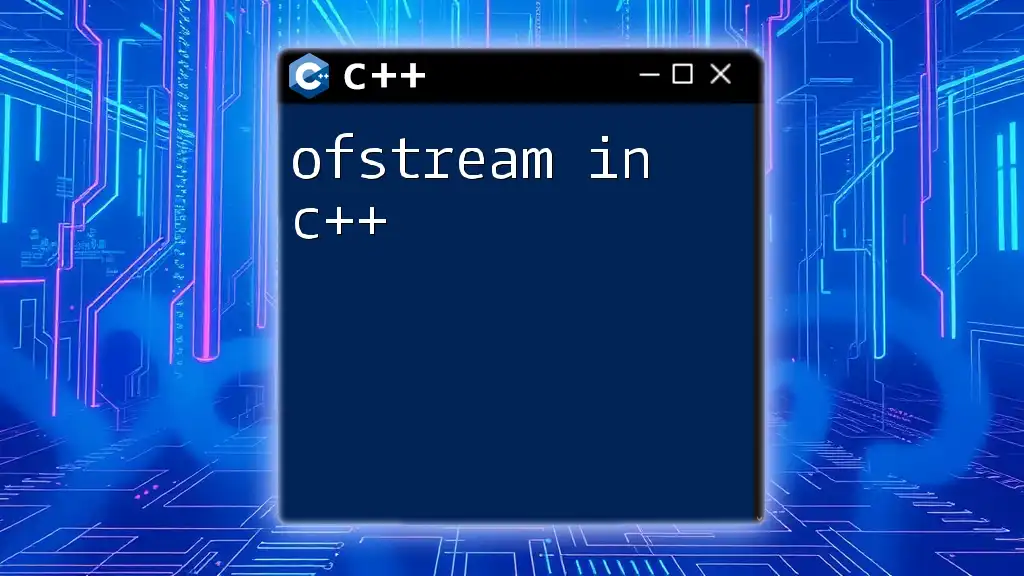
Advanced Thread Management
Thread Pools
Managing individual threads can become cumbersome, particularly if your application spawns a large number of them. To mitigate this, implement a thread pool—a group of pre-instantiated threads that can be reused for executing tasks.
Creating a simple thread pool requires careful consideration of task handling and the lifecycle of threads.
Future and Promises
C++ offers a powerful mechanism to interact with concurrent tasks through futures and promises. A promise provides a way to set a value or an exception that a future can eventually retrieve. This is very useful for obtaining results from threads.
Here’s a quick example of using `std::promise` and `std::future`:
#include <iostream>
#include <thread>
#include <future>
void compute_sum(std::promise<int> &&p) {
int sum = 0;
for(int i = 1; i <= 10; ++i) sum += i;
p.set_value(sum); // Setting the result
}
int main() {
std::promise<int> prom;
std::future<int> fut = prom.get_future();
std::thread t(compute_sum, std::move(prom));
t.detach();
std::cout << "Sum: " << fut.get() << std::endl; // Wait and get the result
return 0;
}
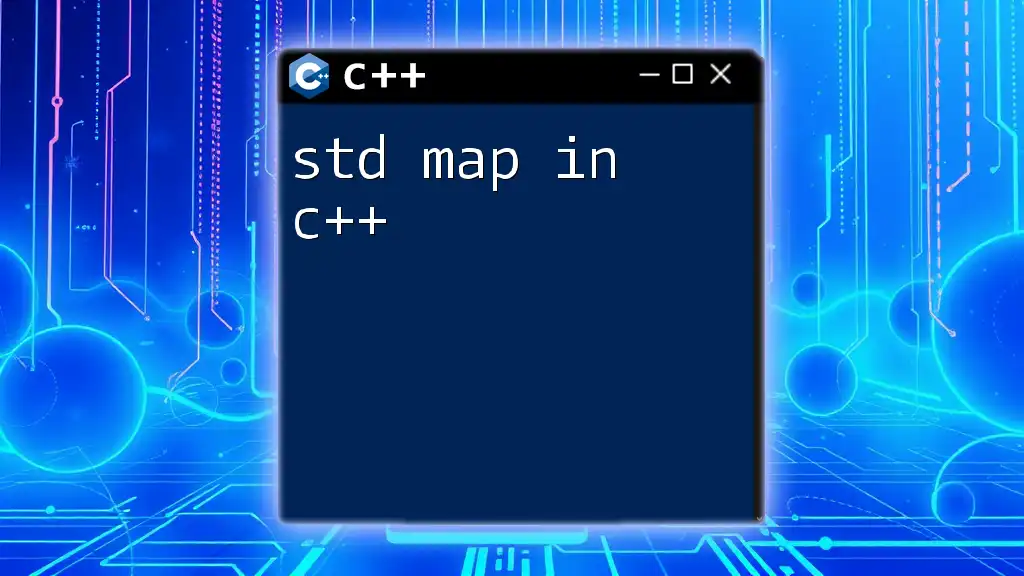
Best Practices for Using `std::thread`
When working with `std::thread`, keep these best practices in mind:
- Avoid busy-waiting: Use synchronization primitives like mutexes, condition variables, or other concurrent data structures to prevent CPU hogging.
- Manage resources properly: Always ensure that every thread is either joined or detached to avoid resource leaks.
- Limit shared data access: Reduce the shared data as much as possible, and always protect access to shared entities.
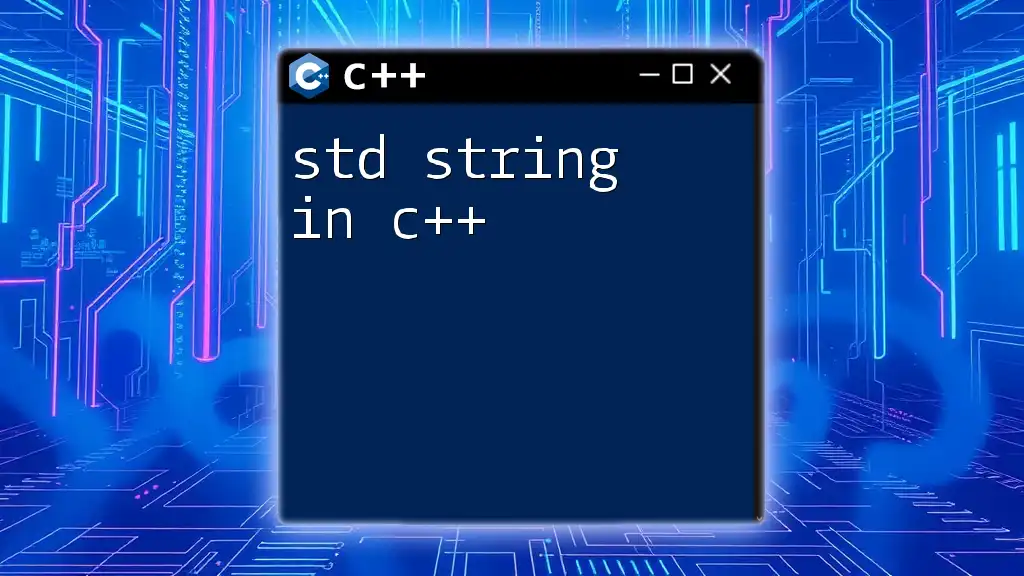
Conclusion
Understanding and effectively using `std::thread in C++` can significantly enhance your ability to develop efficient, responsive, and high-performance applications. By grasping the basic and advanced techniques associated with threading, you position yourself to tackle complex problems that require concurrent processing. As you continue your journey with multithreading, explore and experiment with various patterns and practices to deepen your expertise.
Feel free to explore additional resources or community forums to further enrich your knowledge and skills in using `std::thread`. With practice, you will become proficient in creating robust applications that fully utilize the power of modern hardware.