`std::cout` in C++ is used to output data to the standard console, allowing programmers to display information to the user.
Here's a simple example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is the Standard Output Stream?
The standard output is the destination where a program writes its output data, typically the console or terminal window. In C++, using streams makes it easier to handle input and output operations by allowing you to work with data as a series of bytes. This approach allows for flexibility and powerful features like chaining commands.

Introducing `std::cout`
`std::cout` stands for "standard character output" and is an instance of the `ostream` class. The use of the prefix `std::` signifies that this function is part of the Standard Library namespace, which helps prevent conflicts with similarly-named objects in other libraries or user-defined code.
By default, `std::cout` outputs to the console, which is crucial for displaying information to the user during a program's execution.
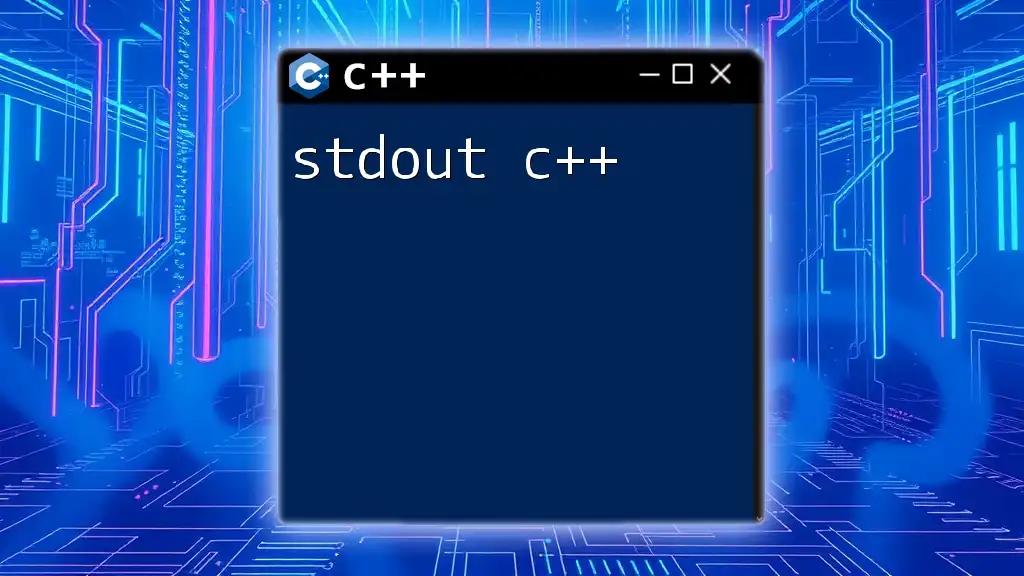
How to Use `std::cout`
Basic Syntax and Structure
The basic syntax for using `std::cout` involves the insertion operator (`<<`), which sends data to the output stream. Here is a simple example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, `"Hello, World!"` gets printed to the console followed by a line break. The `std::endl` manipulator also flushes the output buffer, ensuring all data is displayed immediately.
Printing Different Data Types
Integer and Floating-Point Numbers
You can use `std::cout` to print various data types. For example, printing an integer and a floating-point number can be done as follows:
int num = 42;
float pi = 3.14f;
std::cout << "Integer: " << num << ", Float: " << pi << std::endl;
Here, `num` and `pi` are displayed along with accompanying text. This simple usage highlights how easy it is to concatenate different data types in a single output command.
Strings and Characters
`std::cout` can also handle string and character data types seamlessly. Here’s an illustrative example:
std::string message = "Welcome to C++!";
char initial = 'C';
std::cout << "Message: " << message << ", Initial: " << initial << std::endl;
Notice how we can add variety to our output by combining different data types gracefully.
Boolean Values
For boolean values, the output can be enhanced using the `std::boolalpha` manipulator, which converts boolean values to their textual representations (true/false). Here’s how you can print a boolean value:
bool isAvailable = true;
std::cout << "Available: " << std::boolalpha << isAvailable << std::endl;
Without `std::boolalpha`, the output would be `1` for true and `0` for false, which might not be self-explanatory.

Formatting Output with `std::cout`
Using Manipulators for Output Formatting
Manipulators are functions that modify the formatting of the output. `std::endl` is the most commonly used manipulator that outputs a newline and flushes the output buffer. Although both `std::endl` and `'\n'` can create new lines, `std::endl` ensures immediate display of output, which may not be necessary in all scenarios.
`std::setw()`, `std::setprecision()`, and Others
To control the display width and precision for floating-point numbers, use the following manipulators:
#include <iomanip>
std::cout << std::setw(10) << "Number" << " "
<< std::setprecision(2) << std::fixed
<< pi << std::endl;
Here, `std::setw(10)` sets the width of the output to 10 characters, and `std::setprecision(2)` limits the output to two decimal points, enhancing readability for numeric outputs.
Controlling Floating-point Output
In some cases, you may need to control how floating-point numbers are displayed. You can use scientific notation for large or small numbers using:
std::cout << std::scientific << 123456.789 << std::endl;
This results in `1.234568e+05`, a clearer representation for certain applications.
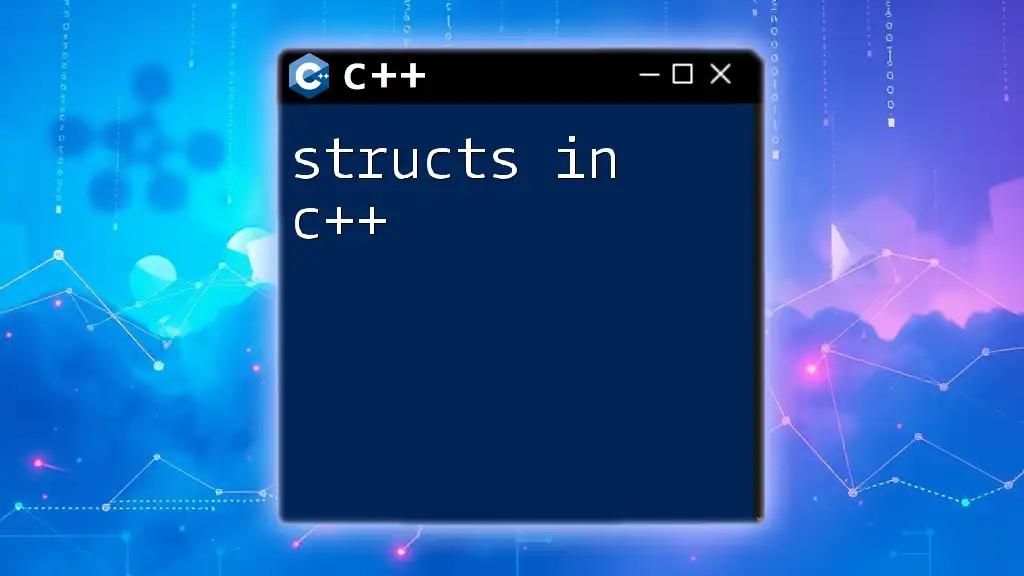
Best Practices for Using `std::cout`
Clarity and Readability
Avoid clutter and enhance user experience by ensuring outputs are intuitive and clear. For instance, instead of this confusing output:
std::cout << num << " " << pi << " " << message << std::endl;
Consider a more descriptive approach:
std::cout << "The answer is: " << num << ", Pi is approximately: " << pi << ", Message: " << message << std::endl;
Performance Considerations
While `std::cout` is convenient for debugging, it may not be suitable for large-scale applications where performance is critical. Using alternative output methods, such as writing to files, can improve performance and efficiency, especially in high-volume operations.

Common Mistakes When Using `std::cout`
Forgetting `#include <iostream>`
One frequent mistake is neglecting to include the `<iostream>` header file, which results in errors. Make sure you start your code with:
#include <iostream>
Using Incorrect Data Types
Mismanaging data types while attempting to print them can cause issues. For instance, trying to print a custom struct without defining how `std::cout` handles it will lead to an error. Here’s a problematic piece of code:
struct Point {
int x, y;
};
Point pt = {10, 20};
// std::cout << pt; // Error: no overloaded operator for Point
To fix this, you need to overload the `<<` operator.

Advanced Topics
Overloading the `<<` Operator
By overloading the `<<` operator, you can customize how objects of a class are printed. Here’s how to achieve that for a `Point` struct:
struct Point {
int x, y;
friend std::ostream& operator<<(std::ostream& os, const Point& pt) {
return os << "(" << pt.x << ", " << pt.y << ")";
}
};
Point pt = {10, 20};
std::cout << "Point: " << pt << std::endl;
This code outputs `Point: (10, 20)`, showcasing how overloading enhances the usability of `std::cout`.
Multithreading with `std::cout`
In a multithreaded context, outputting data to `std::cout` can lead to race conditions, potentially causing mixed or lost output. To handle this challenge, you can use mutexes to synchronize access to `std::cout`, ensuring threads do not interfere with one another while outputting data.
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
void safePrint(const std::string& message) {
std::lock_guard<std::mutex> guard(mtx);
std::cout << message << std::endl;
}
// Example of use in threads

Conclusion
Mastering `std::cout` in C++ allows you to effectively communicate information to users and can dramatically enhance the clarity of your programs. From printing basic data types to formatting output for better readability, `std::cout` is a fundamental component of C++ programming. Familiarizing yourself with its capabilities, best practices, and potential pitfalls will aid greatly in developing robust applications.