In C++, `cin` is used for input from the user, while `cout` is used for output to the console, allowing for easy interaction with the user.
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "You entered: " << number << endl;
return 0;
}
Understanding cout in C++
What is cout?
cout stands for "character output" and is used to display output to the console in C++. The cout object is part of the standard C++ library, specifically in the `<iostream>` header file, and is utilized for printing text, variables, and formatted information.
Syntax of cout
Using cout is straightforward and primarily follows a basic syntax:
cout << value;
Here, the `<<` operator, known as the insertion operator, pushes the data (e.g., strings, numbers) to the output stream.
Example Code:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this example, cout prints "Hello, World!" to the console. The `endl` flushes the output buffer, ensuring the text appears immediately.
Formatting Output
Using std::endl
`std::endl` is a manipulator that denotes the end of a line. It also flushes the output buffer, which can be particularly important in interactive applications. However, frequent use can slow down performance due to constant flushing.
Difference between `std::endl` and `\n`:
- `std::endl` flushes the buffer, while `\n` does not.
Example Code:
cout << "Hello, World!" << endl; // New line with flushing the output stream
cout << "Hello again!" << "\n"; // New line without flushing
Manipulators
Manipulators help control the formatting of output. Some common manipulators include setw for setting the width of the output and setprecision for controlling the number of decimal digits.
Example Code:
#include <iomanip>
cout << fixed << setprecision(2) << 3.14159 << endl; // Output: 3.14
In this example, the output is formatted to display two decimal places.

Understanding cin in C++
What is cin?
cin, short for "character input," is used to accept user inputs from the console. Like cout, it is also part of the `<iostream>` header and plays a crucial role in interacting with users.
Syntax of cin
The syntax for cin is similar to cout, relying on the extraction operator (`>>`):
cin >> variable;
This operator pulls the input data from the standard input stream into the specified variable.
Handling Different Data Types
Validating Input
It's important to ensure that the data type of the input matches the expected type to avoid runtime errors. This can be accomplished through input validation.
Example Code:
int age;
while (true) {
cout << "Enter your age: ";
if (!(cin >> age)) {
cout << "Invalid input. Please enter a number." << endl;
cin.clear(); // Clear the error flag
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Ignore the bad input
} else {
break; // Valid input received
}
}
In the above code, if the user enters a non-integer value, cin sets an error state, which is then cleared, and the input buffer is ignored until valid input is given.

Combining cin and cout
Reading and Writing in the Same Program
Combining cin and cout allows for seamless interaction within a program.
Example Code:
string name;
cout << "Enter your name: ";
cin >> name;
cout << "Welcome, " << name << "!" << endl;
This simple program prompts the user for their name and greets them using the input received.
Common Pitfalls
Input Buffer Issues
Sometimes, input operations can lead to unintended behavior, especially when clearing input streams. A common pitfall occurs when expecting a string input after a numeric input, as remnants in the buffer may cause issues.
Example Code:
int age;
string name;
cout << "Enter your age: ";
cin >> age;
cout << "Enter your name: ";
cin >> name; // Issues if you expect to input a full name
To properly handle full names, you should consider using `getline()` after reading the integer.

Additional Features of cin and cout
Error Handling with cin
It's critical to handle errors with cin effectively. If an invalid operation occurs, checking the state of cin can help gracefully handle such errors.
Example Code:
if (cin.fail()) {
cout << "Input error occurred." << endl;
}
This checks if cin entered an error state after input attempts, allowing for corrective actions.
Customizing cin and cout
You can further customize output and input behavior using various manipulators and member functions, like `setf()` to adjust the floating-point style.
Example Code:
cout.setf(ios::fixed);
cout.precision(2);
cout << "Pi is approximately: " << 3.14159 << endl;
This code sets the output to a fixed-point notation with two decimal places.

Conclusion
In summary, cin and cout form the backbone of standard input and output in C++. Mastery of these commands is essential for effective programming and interaction with users. By understanding both their basic usage and advanced features, you can develop more robust and user-friendly applications.
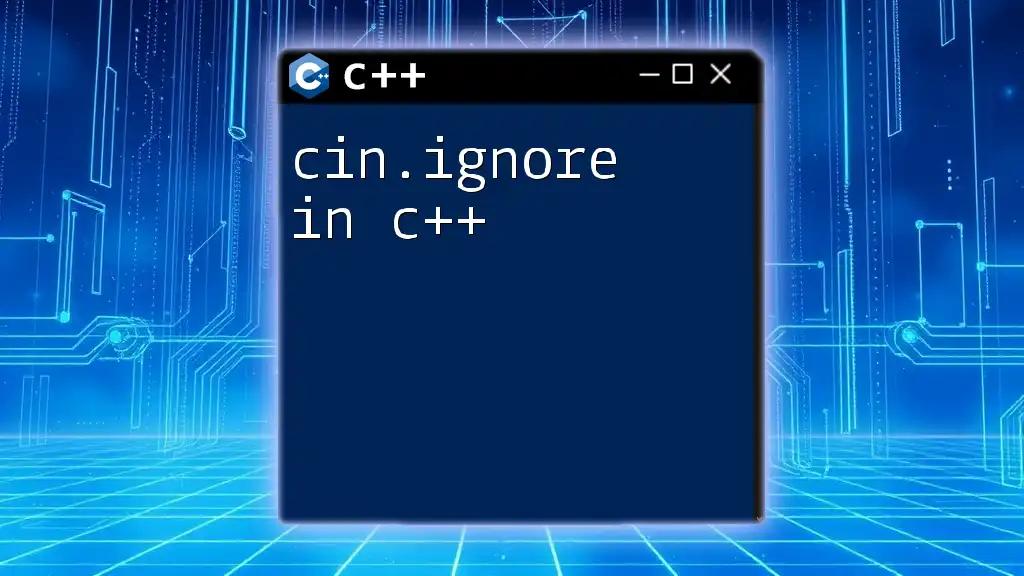
Call to Action
Stay tuned for more concise tutorials on C++ commands to enhance your programming skill set! Keep practicing and experimenting with cin and cout in C++ to solidify your understanding.