In C++, the `rand()` function is used to generate pseudo-random numbers, and the `srand()` function initializes the random number generator with a seed value to ensure different sequences of random numbers.
Here’s a simple example:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
srand(static_cast<unsigned int>(time(0))); // Seed the random number generator
int randomNum = rand() % 100; // Generate a random number between 0 and 99
std::cout << "Random Number: " << randomNum << std::endl;
return 0;
}
Understanding Randomness in C++
What is Randomness?
Randomness plays a crucial role in various programming contexts, including simulations, gaming, statistical analyses, and cryptography. Randomness refers to the lack of pattern or predictability in events, enabling more dynamic and realistic outcomes in applications. When working with random numbers, it's vital to understand how they are generated and their implications in the context of your program.
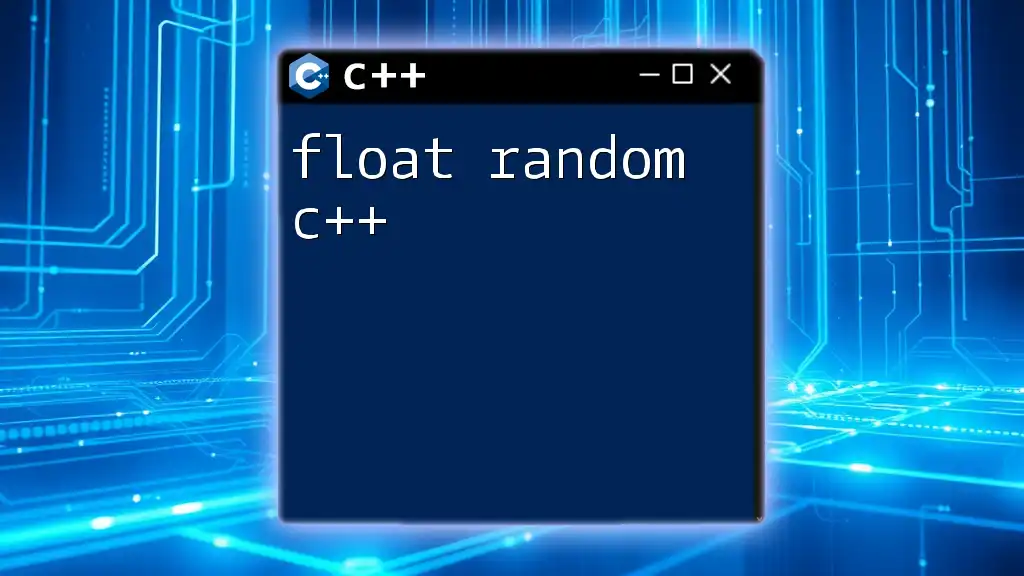
Generating Random Numbers in C++
Using `rand()` Function
What is `rand()`?
The `rand()` function is a standard tool provided by C++ for generating pseudo-random integers. It produces a random integer within the range of 0 to `RAND_MAX`, generally defined as at least 32767. This simplicity makes `rand()` a popular choice for straightforward random number generation tasks.
Code Example: Basic Random Integer Generation
To generate a random integer, you can use the simple code snippet below:
#include <iostream>
#include <cstdlib> // For rand and RAND_MAX
int main() {
std::cout << "Random Integer: " << rand() << std::endl;
return 0;
}
In this example, the program prints a random integer each time it is executed. However, using `rand()` alone has limitations that may affect your application's randomness.
Understanding `RAND_MAX`
`RAND_MAX` is a constant defined in `<cstdlib>` that indicates the maximum value returned by the `rand()` function. While useful, relying solely on `rand()` can lead to predictability, especially in critical applications like gaming or cryptography.
Seeding the Random Number Generator
Importance of Seeding
Seeding is essential to ensure that your sequence of random numbers is unpredictable. Without seeding, `rand()` generates the same numbers on every run, which is not ideal for most applications.
Using `srand()`
To seed the random number generator, the `srand()` function should be called before using `rand()`. This function takes an integer as an argument, typically derived from the current time, to ensure variability in output.
Code Example: How to Seed the Random Number Generator
Here's an example that seeds the random number generator to produce different outputs:
#include <iostream>
#include <cstdlib> // For rand and srand
#include <ctime> // For time
int main() {
srand(time(0)); // Seed the RNG with the current time
std::cout << "Random Integer: " << rand() << std::endl;
return 0;
}
In this code, `srand(time(0));` seeds the generator with the current time, ensuring a new sequence of random numbers each time the program runs.
Best Practices for Seeding
- Always seed the random number generator to improve randomness.
- Consider using a different seeding strategy if you need specific patterns or repeatability, such as seeding with a fixed value during testing or debugging.
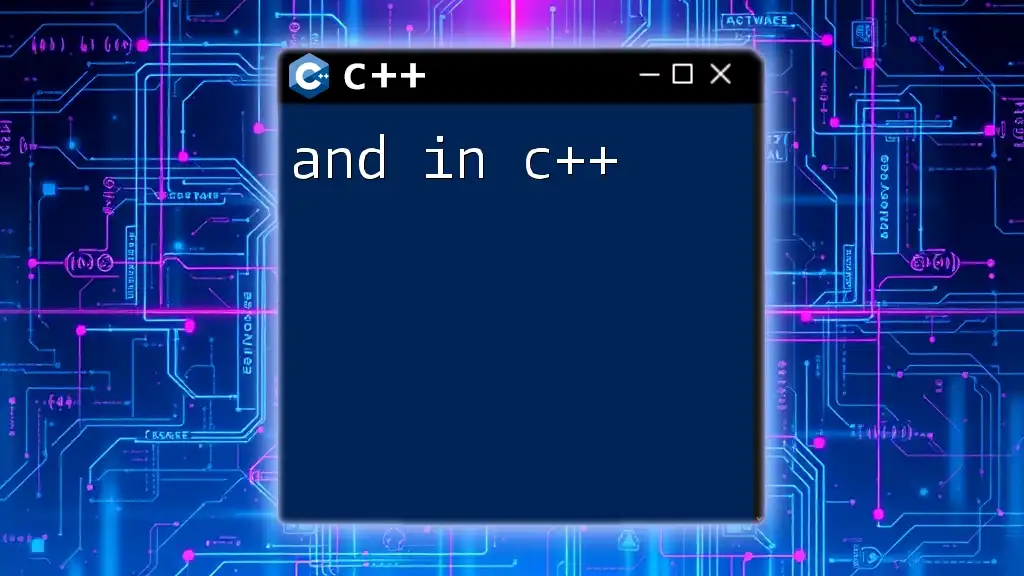
Advanced Random Number Generation with `<random>`
Introduction to `<random>`
While `rand()` provides basic functionality, the C++ Standard Library offers the `<random>` header, which introduces modern, robust ways to generate random numbers. Unlike `rand()`, the `<random>` library implements true random distribution mechanics, offering better randomness and control.
Random Number Distribution
Uniform Distribution
Uniform distribution ensures that each number within a specific range is equally likely to be generated. This type of distribution is crucial in scenarios where every outcome should occur with equal probability.
Code Example: Generating Uniformly Distributed Integers
Here’s an example of generating uniformly distributed integers:
#include <iostream>
#include <random>
int main() {
std::default_random_engine generator;
std::uniform_int_distribution<int> distribution(1, 10);
std::cout << "Random Integer: " << distribution(generator) << std::endl;
return 0;
}
In this snippet, `distribution(generator)` produces a random integer between 1 and 10, ensuring uniformity across the range.
Normal Distribution
Understanding normal distribution (or Gaussian distribution) is essential for applications that require values around a mean with a specific variability.
Code Example: Generating Normally Distributed Values
The following code illustrates how to generate values that follow a normal distribution:
#include <iostream>
#include <random>
int main() {
std::default_random_engine generator;
std::normal_distribution<double> distribution(0.0, 1.0); // mean 0, stddev 1
std::cout << "Random Value: " << distribution(generator) << std::endl;
return 0;
}
This code will produce a value centered around 0 with a standard deviation of 1, typical for many statistical applications.
Other Distribution Types
Beyond uniform and normal distributions, C++ `<random>` provides several other distribution types:
- Binomial Distribution: Useful for representing success/failure outcomes.
- Poisson Distribution: Ideal for modeling random events in a fixed interval.
- Exponential Distribution: Frequently employed in reliability analysis.
Each of these distributions offers unique features and applications, emphasizing the flexibility of the `<random>` library in C++.
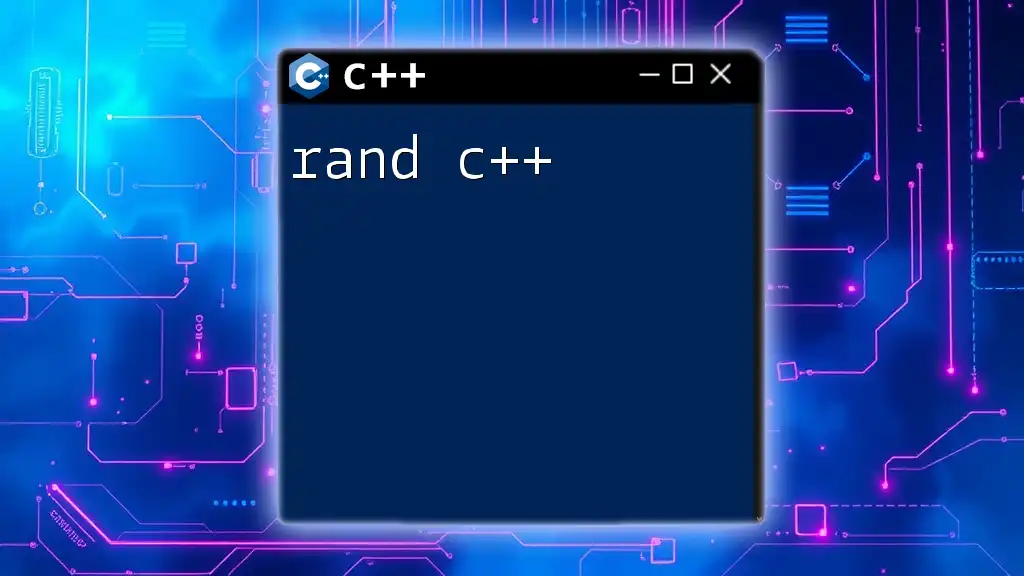
Common Use Cases for Random Numbers
Random Sampling
Random sampling is vital in statistical analysis, surveys, and simulations. This method allows you to select a subset of items from a larger dataset randomly.
Code Example: Random Sampling Technique Using `<random>`
Here's a simple way to select a random sample from a fixed array:
#include <iostream>
#include <random>
#include <array>
int main() {
std::array<int, 5> data = {1, 2, 3, 4, 5};
std::default_random_engine generator;
std::uniform_int_distribution<int> distribution(0, data.size() - 1);
std::cout << "Random Sample: " << data[distribution(generator)] << std::endl;
return 0;
}
Games and Simulations
In gaming, random numbers define actions, outcomes, and behaviors, making gameplay more dynamic and unpredictable. From rolling dice to generating random terrain, randomness adds a layer of excitement.
Code Example: Simulating Dice Rolls
A simple dice-rolling simulation can effectively demonstrate C++ random functionalities:
#include <iostream>
#include <random>
int main() {
std::default_random_engine generator;
std::uniform_int_distribution<int> distribution(1, 6); // Dice faces
std::cout << "Dice Roll: " << distribution(generator) << std::endl;
return 0;
}
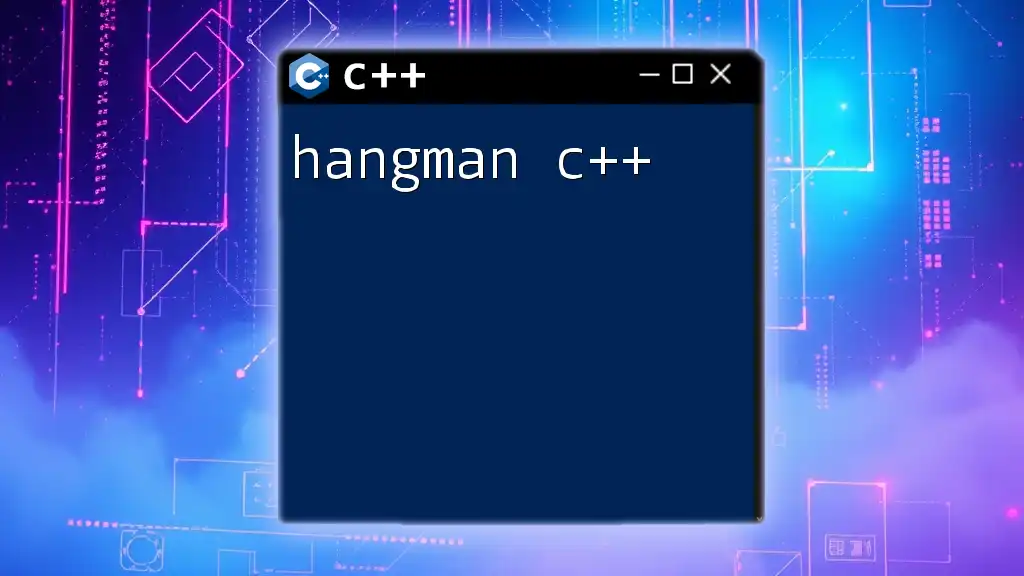
Best Practices for Using Random Numbers in C++
Avoiding Common Pitfalls
When using random numbers, avoid common pitfalls like:
- Relying solely on `rand()`, which can produce predictable outputs.
- Forgetting to seed the random number generator results in repeated sequences.
Ensuring Repeatability
In some cases, repeatability is crucial, such as in testing scenarios. You can achieve repeatable results by seeding with a constant value, allowing for consistent outputs.
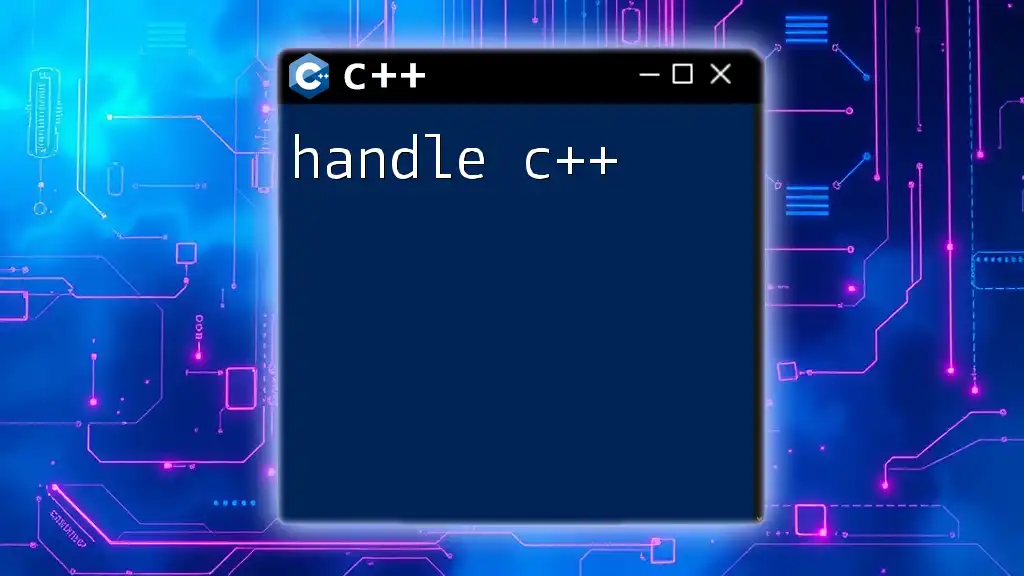
Conclusion
Randomness in C++ is a powerful tool that can significantly enhance your programs, from gaming to simulations. Understanding the fundamentals of random number generation and embracing the more advanced `<random>` library can lead to better practices and outcomes in your coding projects.
By delving into the nuances of randomness and leveraging C++ functionalities, you can create dynamic and engaging applications tailored to various purposes.
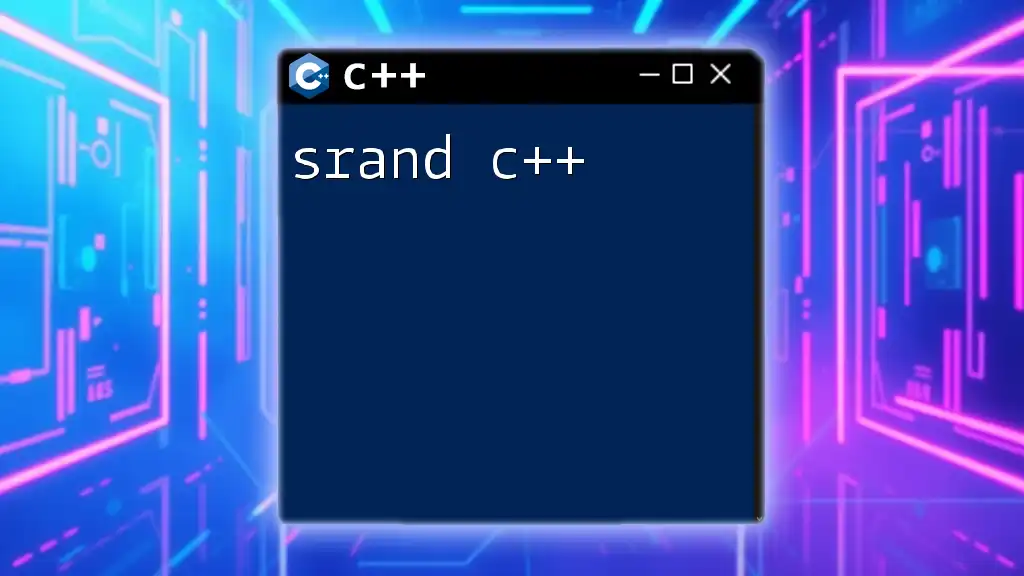
Additional Resources
To deepen your understanding of randomness in C++, consider exploring the official C++ documentation and recommended books on the subject. These resources can provide you with further insights and practical guidance.
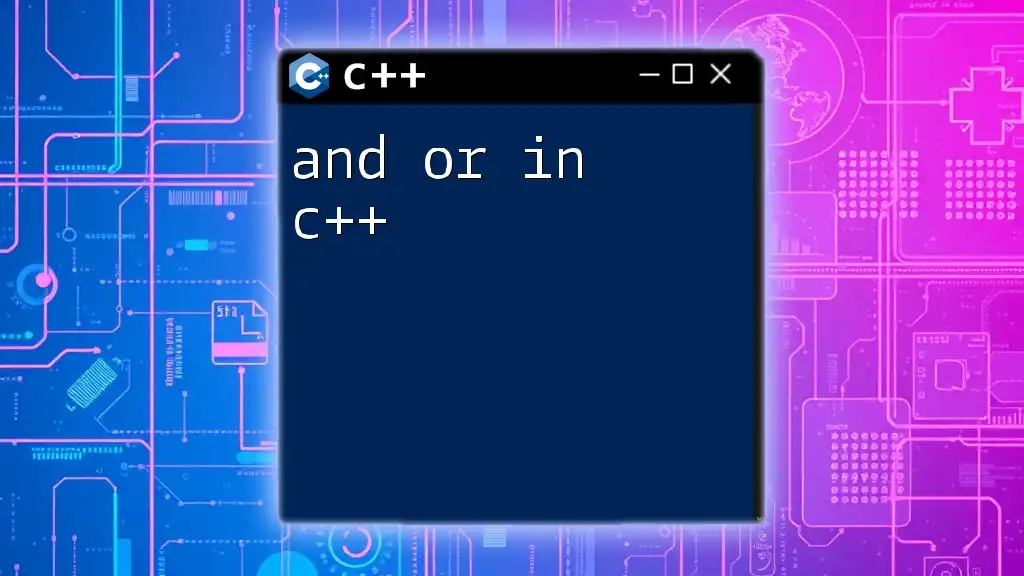
Call to Action
Have you implemented random number generation in your projects? Share your experiences or examples of using random numbers in C++. Subscribe to stay updated on more tips and tricks related to C++ programming!