The `auto` keyword in C++ enables automatic type deduction, allowing the compiler to determine the variable's type based on the initializer's type.
Here's a code snippet demonstrating its use:
#include <iostream>
#include <vector>
int main() {
auto number = 42; // auto deduces int
auto name = "Hello, C++"; // auto deduces const char*
auto numbers = std::vector<int>{1, 2, 3}; // auto deduces std::vector<int>
std::cout << number << std::endl;
std::cout << name << std::endl;
for (auto n : numbers) {
std::cout << n << " ";
}
return 0;
}
What is the Auto Keyword in C++?
Definition of Auto
The auto keyword in C++ is a powerful feature introduced in C++11 that enables automatic type deduction for variables. With auto, you can define a variable without explicitly specifying its type. Instead, the compiler infers the type based on the initializer you provide. This simplifies the process of variable declaration and makes your code cleaner and easier to maintain.
Significance of Auto in C++
Using auto significantly impacts the way developers write C++ code. It reduces verbosity in variable declarations and enhances code maintainability by making it easier to adapt to changes. If a type changes due to updates in the codebase or library, using auto minimizes the need for simultaneous changes across multiple declaration statements.
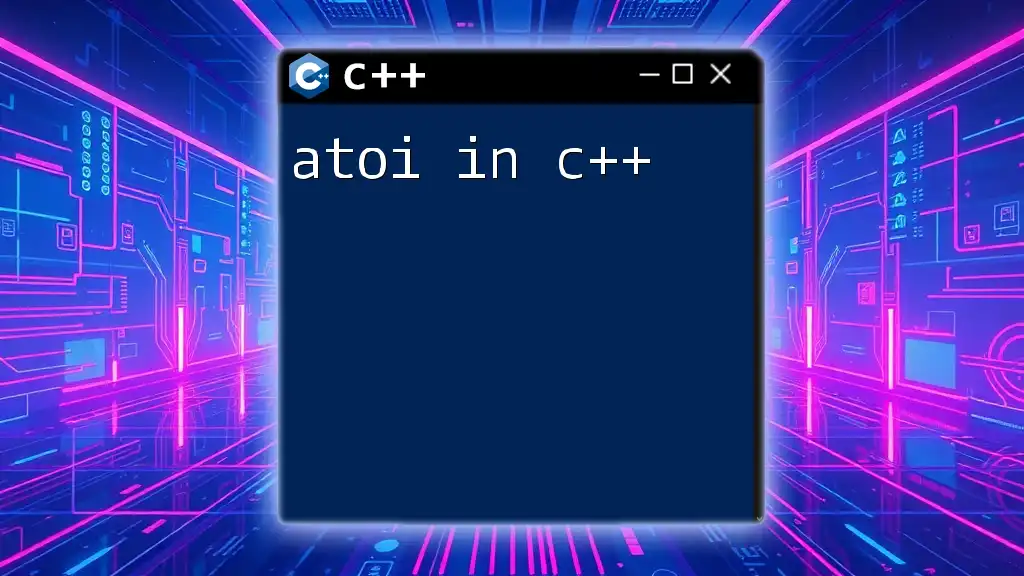
How Does the Auto Keyword Work?
Type Deduction Mechanism
The auto keyword utilizes a type deduction mechanism that assigns the correct type based on the right-hand side expression of the declaration. The following example illustrates this:
auto x = 5; // x is deduced as int
auto y = 3.14; // y is deduced as double
auto message = "Hello, World!"; // message is deduced as const char*
In this example, `x` is deduced as an `int`, `y` as a `double`, and `message` is inferred as a pointer to a `const char`.
Basic Rules of Using Auto
To successfully use the auto keyword, there are several essential rules to follow:
-
Initialization: A variable declared with auto must be initialized at the point of declaration. The compiler needs this information to deduce the variable's type.
-
Cannot be used for function return types: Prior to C++14, you could not use auto in function return types without a trailing return type.
-
Ambiguity: If the initializer leads to ambiguity in type deduction, such as with `nullptr`, the B compiler will throw an error.
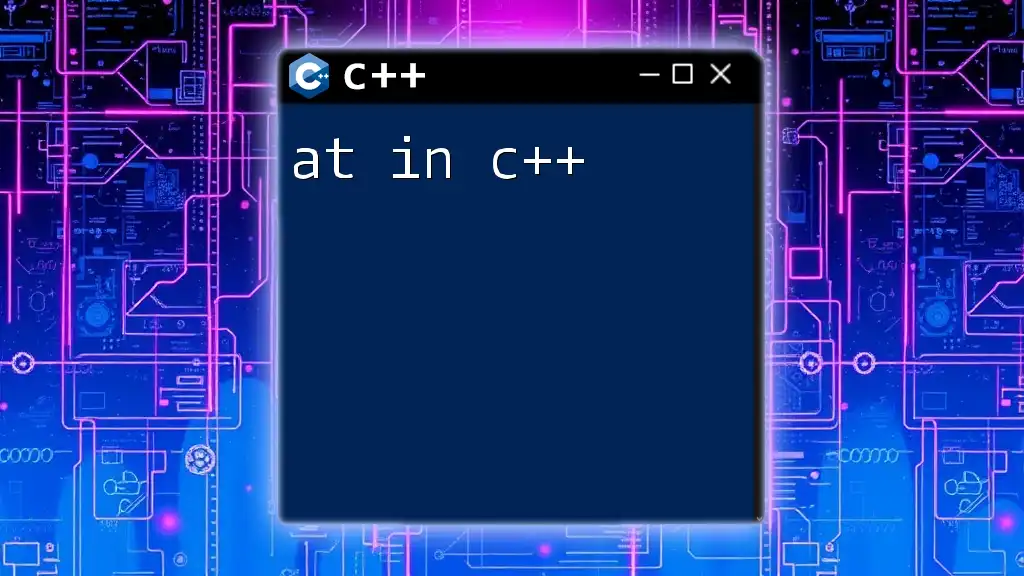
Benefits of Using C++ Auto
Code Clarity and Readability
One of the most significant advantages of using auto is the improvement in code clarity and readability, especially when dealing with complex types. For instance, when working with iterators from the Standard Template Library (STL), using auto can drastically simplify the code, as shown below:
std::vector<int>::iterator it = vec.begin(); // Traditional
auto it = vec.begin(); // Using auto, clearer and more concise
The second line is far simpler, clearly demonstrating the intent without needing to unravel complex type specifications.
Flexibility in Type Changes
Using auto provides flexibility when types change. Imagine if you start with a float and later want to use a double; auto makes this transition seamless. Here’s an example:
auto pi = 3.14f; // Initially a float
pi = 3.14; // Automatically adapts to double without any modifications
This adaptability helps maintain your code with minimal cognitive overhead, as you avoid unnecessary type declarations.
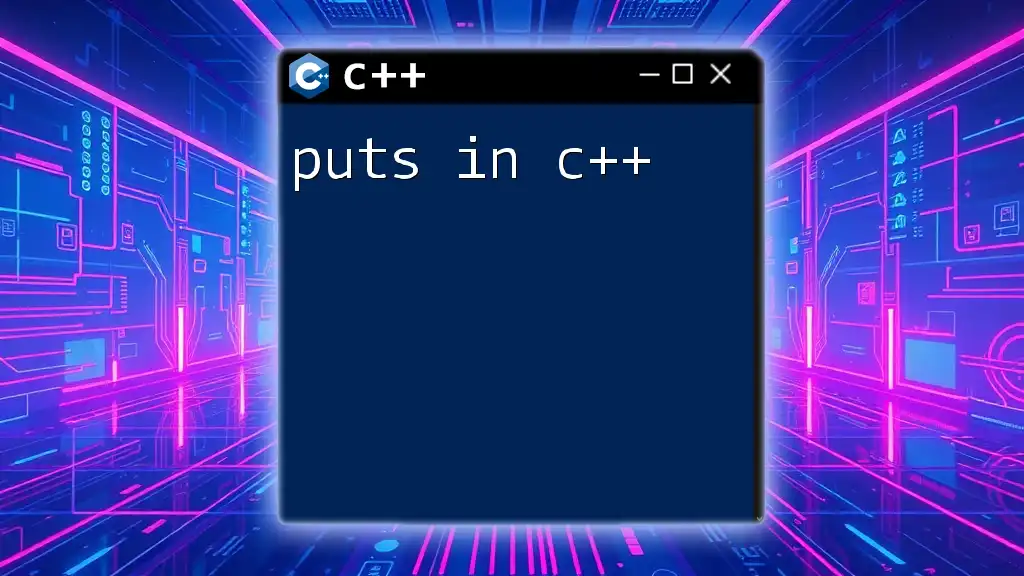
Common Use Cases for C++ Auto
Iterators in STL (Standard Template Library)
The auto keyword shines when working with STL iterators. It helps remove the burden of specifying lengthy iterator types, which can be quite verbose. Below is an example using a range-based for loop, which is a preferred way to iterate through containers:
for (auto& element : vec) {
// process element
}
In this example, auto allows the loop to deduce the type of `element`, enhancing both the simplicity and clarity of the code.
Leveraging Auto with Lambdas
Lambdas, introduced in C++11, benefit immensely from the auto keyword. By allowing parameter types to be inferred, developers can write concise and expressive lambda expressions. Here’s how it looks:
auto lambda = [](auto a, auto b) { return a + b; };
This example captures the essence of generic programming, where you can create a function that works with any type, thanks to type deduction.
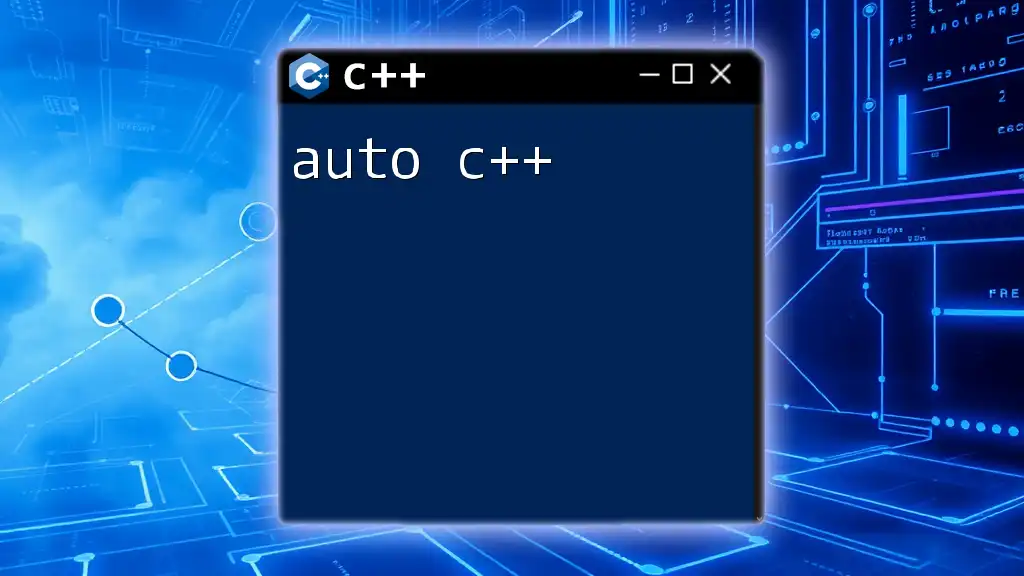
Limitations and Precautions of Using Auto
Loss of Explicit Type Information
While the auto keyword brings numerous advantages, it also poses a challenge: the potential loss of explicit type information. This can sometimes lead to unintended consequences, especially in situations involving implicit conversions. Consider the following:
auto a = 1; // deduced as int
auto b = 1.0; // deduced as double
This could lead to confusion down the line, especially if the types have different behaviors when operated on.
Performance Considerations
Generally speaking, using auto does not impact performance negatively. In fact, it can lead to cleaner and potentially more optimized code. However, caution should be exercised when using auto in cases where performance-critical sections require explicit and tailored types for optimization.
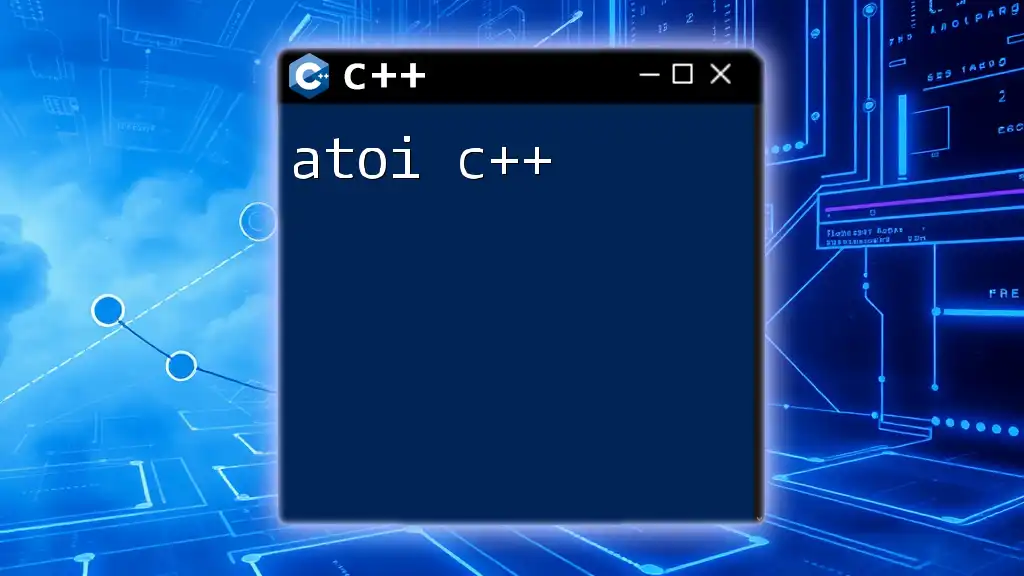
Best Practices for Using the Auto Keyword in C++
When to Use Auto
The auto keyword is particularly advantageous in specific situations:
- When dealing with complex types or iterators where explicit typing would clutter the code.
- Within range-based for loops or STL algorithms (e.g., `std::transform`).
- In template-heavy code or generic programming scenarios, where types may vary significantly.
Code Style Recommendations
To ensure maintainability and readability in your code, consider the following recommendations regarding the use of auto:
- Maintain consistency in using auto throughout your codebase. It creates a uniform style that enhances understanding.
- Comment on type deductions if they may be ambiguous or unintuitive for other developers reviewing your code.
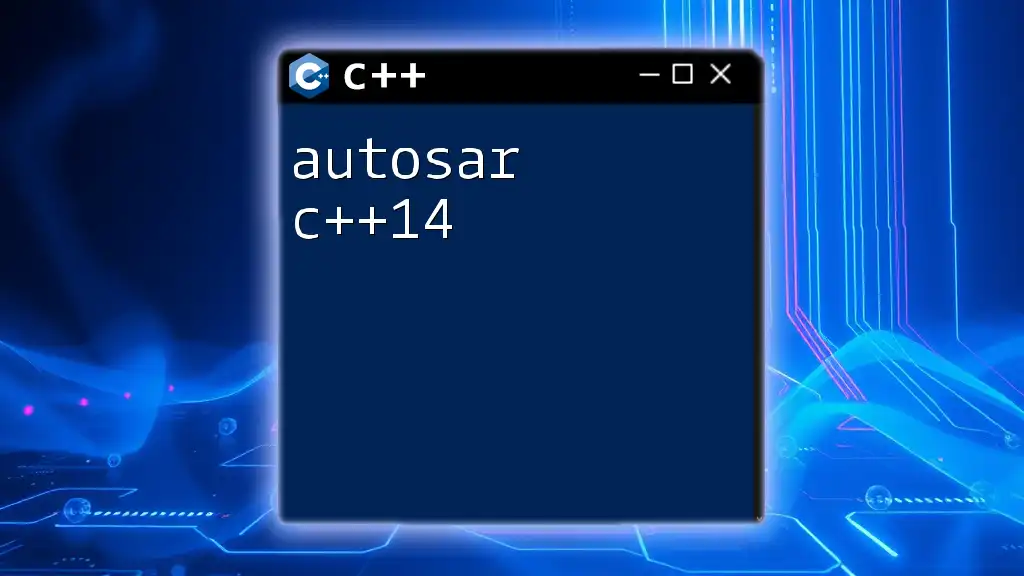
Conclusion
The auto in C++ keyword is indeed a game-changer, simplifying variable declarations, enhancing readability, and allowing for easy adaptability in changing code contexts. By understanding its strengths, limitations, and best practices, developers can leverage auto to write clean, maintainable, and efficient C++ code. Embracing modern C++ programming practices will ultimately lead to improved productivity and code quality.
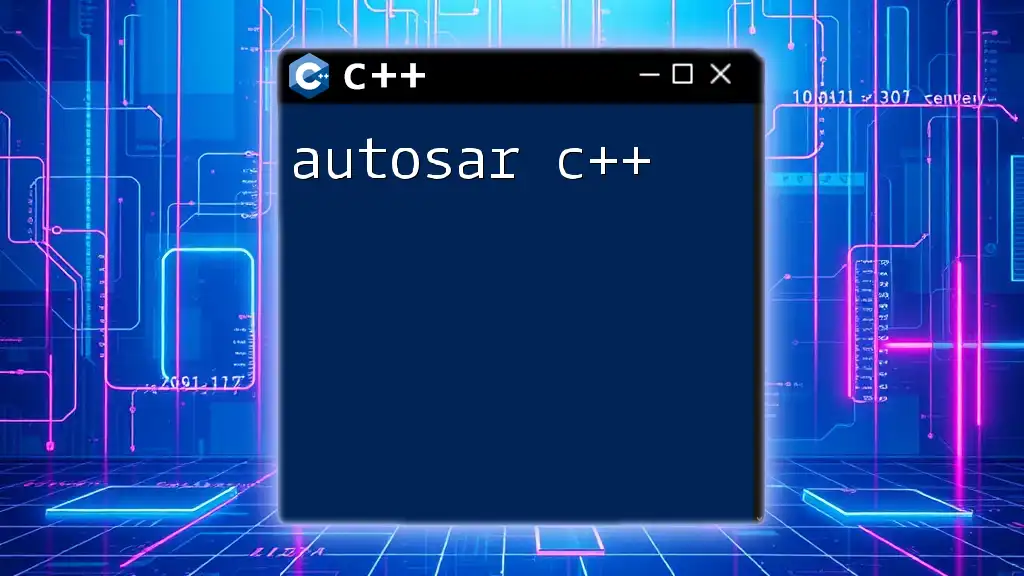
Additional Resources
For more in-depth understanding, consider exploring documentation resources such as:
- C++ Standard Documentation
- C++ Programming Tutorials from renown universities or online courses
- Books focused on modern C++ practices and advanced programming techniques