`dynamic_cast` in C++ is used to safely cast pointers and references of base class types to derived class types, ensuring that the conversion is valid at runtime.
#include <iostream>
class Base { virtual void foo() {} }; // Base class with a virtual function
class Derived : public Base {}; // Derived class
int main() {
Base* basePtr = new Derived(); // Base pointer to Derived object
Derived* derivedPtr = dynamic_cast<Derived*>(basePtr); // Safe downcast
if (derivedPtr) {
std::cout << "Cast successful!" << std::endl;
} else {
std::cout << "Cast failed!" << std::endl;
}
delete basePtr; // Clean up
return 0;
}
The Basics of C++
Before diving into `dynamic_cast in C++`, it's essential to understand some foundational concepts of casting in C++. C++ provides several methods for casting types, including `static_cast`, `reinterpret_cast`, and `const_cast`, each serving different purposes based on the type of conversion required.
When to Use `dynamic_cast`
`dynamic_cast` is particularly useful when working with polymorphic types (classes that have at least one virtual function). This feature allows you to safely downcast pointers or references of base classes to derived classes. However, it is crucial to use `dynamic_cast` in scenarios where you expect the actual object type to differ from the base type.
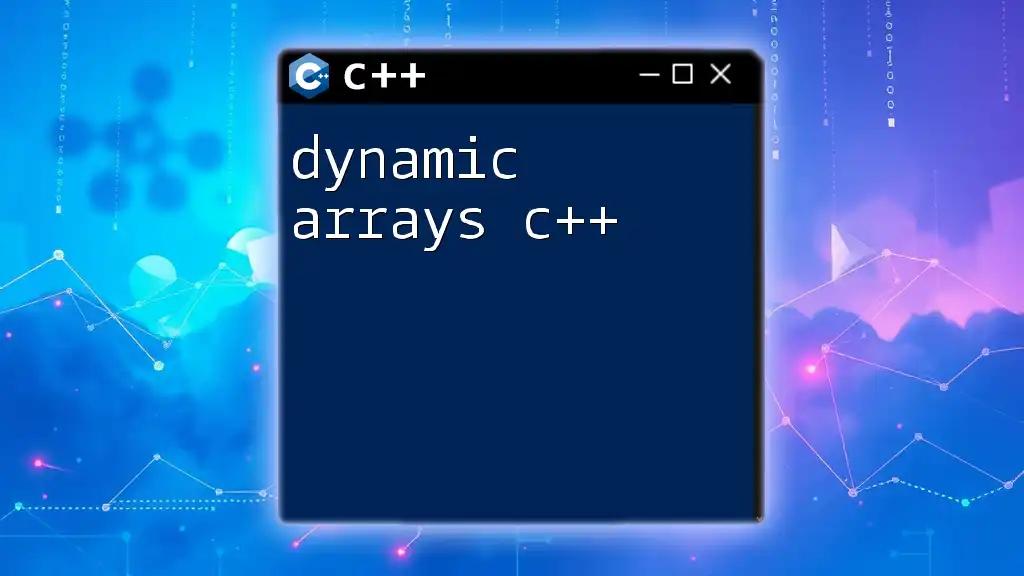
Understanding `dynamic_cast`
What is `dynamic_cast`?
In essence, `dynamic_cast` is a powerful tool that allows you to perform safe downcasting in C++, leveraging Run-Time Type Information (RTTI). When you utilize `dynamic_cast`, it checks the actual type of the object at runtime before attempting the cast, ensuring that you maintain type safety in your applications.
Syntax and Usage
The syntax for `dynamic_cast` is quite straightforward. To apply it, the type you want to cast to is included in angle brackets, followed by the expression you want to cast. Here’s the general syntax:
TargetType* targetPtr = dynamic_cast<TargetType*>(basePtr);
This `dynamic_cast` returns a nullptr if the cast fails, making it easy to check validity.
Code Snippet: Basic Syntax
Let’s consider a practical example using `dynamic_cast`:
class Base {
public:
virtual ~Base() {} // Virtual destructor
};
class Derived : public Base {
public:
void derivedFunction() {}
};
Base* basePtr = new Derived();
Derived* derivedPtr = dynamic_cast<Derived*>(basePtr);
if (derivedPtr) {
// Successful cast
derivedPtr->derivedFunction(); // Calling derived function
} else {
// Cast failed
std::cout << "Cast failed!" << std::endl;
}
In this example, we create instances of `Base` and `Derived`. The `dynamic_cast` checks if `basePtr` can be downcasted to `Derived*`. Since `basePtr` actually points to an instance of `Derived`, the cast succeeds.

How `dynamic_cast` Works
Behind the Scenes
When `dynamic_cast` is used, it relies on RTTI to make informed decisions about what type to cast. Each polymorphic class carries type information that the runtime can use to check the object's true type against the specified target type.
Checking Validity
One of the significant advantages of `dynamic_cast` is its ability to validate the cast. If a base pointer refers to an object of a type that is incompatible with the cast, `dynamic_cast` will return nullptr rather than allowing unsafe behavior.
Code Example: Successful and Unsuccessful Casting
To illustrate further, let’s demonstrate both a successful and an unsuccessful cast.
class Base {
public:
virtual void foo() {}
};
class Derived : public Base {};
class AnotherDerived : public Base {};
Base* basePtr = new AnotherDerived();
Derived* derivedPtr = dynamic_cast<Derived*>(basePtr);
if (derivedPtr) {
std::cout << "Cast successful!" << std::endl;
} else {
std::cout << "Cast failed!" << std::endl; // This will be executed
}
In this case, since `basePtr` points to an `AnotherDerived` object, the cast fails, demonstrating the safety of `dynamic_cast`.

Benefits of Using `dynamic_cast`
Type Safety
One of the most significant benefits of using `dynamic_cast` is its ability to ensure type safety, allowing developers to avoid potentially dangerous implicit type casts that can lead to runtime errors or undefined behavior.
Maintaining the Integrity of the Code
Using `dynamic_cast` allows you to work within the principles of object-oriented programming, particularly polymorphism. This ensures that your code remains extensible and easier to maintain, as you are always aware of the type of objects being manipulated.
Real-world Application Examples
You'll often find `dynamic_cast` in scenarios involving complex class hierarchies and components that require interaction based on the actual object type. For instance, in a game engine, where different types of game entities inherit from a common base class, `dynamic_cast` can be pivotal in handling different behaviors based on the actual entity type.

Limitations of `dynamic_cast`
Performance Overhead
Although `dynamic_cast` is incredibly useful, it comes with some performance overhead due to the runtime checks involved. This can be of concern in performance-critical applications, where the benefits need to be weighed against the potential slowdowns.
Dependency on RTTI
Using `dynamic_cast` requires RTTI, which in certain applications can add complexity and bloat to the binary. If RTTI is disabled (via compiler options), `dynamic_cast` will not work, prompting developers to rely on alternative casting methods.
When Not to Use `dynamic_cast`
It's crucial to avoid using `dynamic_cast` in situations where performance is prioritized over safety, or when you are certain about the types being dealt with. In such cases, `static_cast` or `reinterpret_cast` may be more appropriate.
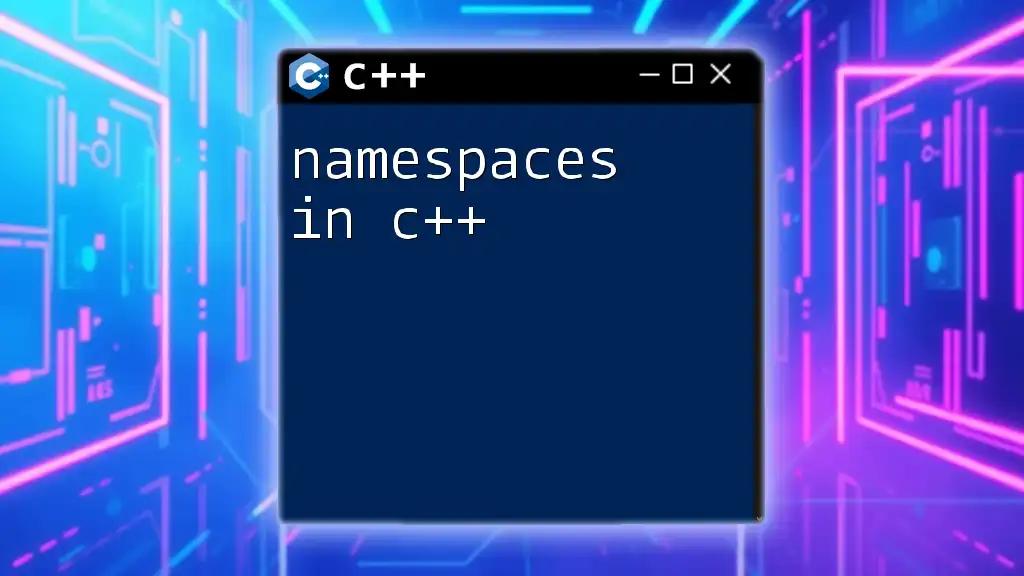
Alternatives to `dynamic_cast`
Comparing with Other Casting Methods
- `static_cast` - This is a compile-time cast that does not perform any runtime checks, making it faster but less safe.
- `reinterpret_cast` - This cast performs low-level reinterpretation of bit patterns and should be used with extreme care.
Use Cases for Each Casting Method
- Use `dynamic_cast` when dealing with polymorphic types and you need runtime safety.
- Use `static_cast` when you have full control over the type conversions and can guarantee their safety.
- Use `reinterpret_cast` when you need a low-level operation that may involve data structures or raw memory manipulation.

Best Practices
Using `dynamic_cast` Wisely
While `dynamic_cast` is a potent tool, it should be used judiciously. Ensure that your class hierarchy is designed with polymorphism in mind, and always check the result of the `dynamic_cast` before utilizing the pointer if the cast is successful.
Common Mistakes to Avoid
Avoid overusing `dynamic_cast`, especially in performance-sensitive applications. Rely on your class design and use `static_cast` whenever possible for efficiency. Also, be cautious when disabling RTTI; understand the implications it has on `dynamic_cast`.
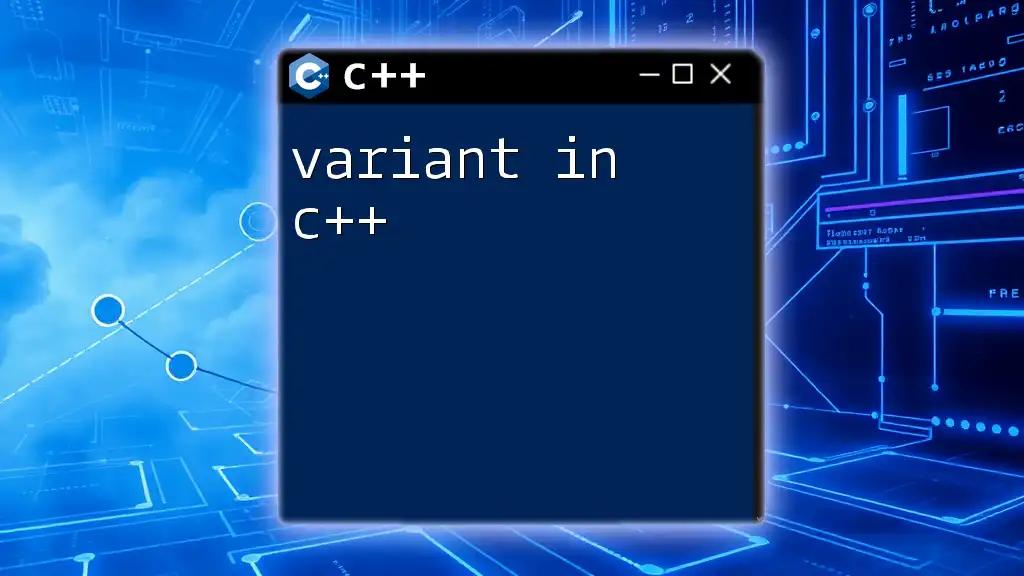
Conclusion
In summary, `dynamic_cast in C++` offers a safe, efficient way to handle type casting within polymorphic class hierarchies. By using `dynamic_cast`, you ensure type safety and maintain the integrity of your code, adhering to the principles of object-oriented programming. As you incorporate it into your projects, always weigh the benefits against potential performance impacts and choose the appropriate casting method for your specific needs.

Further Resources
For those eager to dive deeper into this topic, consider exploring advanced C++ resources, including books focused on polymorphism, class design strategies, and comprehensive guides on type casting. Engaging with community forums and documentation can also provide invaluable insights and support as you navigate the intricacies of C++.