In C++, `static const` is used to declare a constant variable that retains its value across instances of a class and cannot be modified, ensuring that the same value is shared among all objects of the class.
Here is a code snippet demonstrating its use:
class MyClass {
public:
static const int myConstant = 10; // Declaration of a static const variable
};
#include <iostream>
int main() {
std::cout << "The value of myConstant is: " << MyClass::myConstant << std::endl;
return 0;
}
What is `static const` in C++?
In C++, `static const` is a powerful combination that serves as a dual modifier for a variable. The keyword `static` denotes that a variable has static storage duration, while `const` implies that the variable's value is constant and cannot be modified after initialization. This means that once you set a `static const` variable, its value remains consistent throughout the program's execution.
The `static` Keyword
What does `static` mean?
The `static` keyword impacts the lifetime of the variable or function in a significant manner. When a variable is declared as static, it retains its value between function calls, unlike regular local variables that are destroyed when the function exits. This feature is crucial for preserving state without exposing variables to global scope.
Example:
#include <iostream>
void counterFunction() {
static int count = 0; // Static variable
count++;
std::cout << "Count is: " << count << std::endl;
}
int main() {
counterFunction(); // Output: Count is: 1
counterFunction(); // Output: Count is: 2
return 0;
}
Static Variables in Different Contexts
-
Static variables in functions: These variables are initialized only once and retain their value during subsequent calls.
-
Static variables in classes: When declared in a class, a static variable exists independently of any class instances. This means that there is only one copy of the variable, shared among all instances.
Code Snippet:
class Example {
public:
static int sharedCount;
};
int Example::sharedCount = 0; // Definition outside the class
The `const` Keyword
What does `const` mean?
The `const` keyword in C++ restricts modifications to a variable. By declaring a variable as `const`, you communicate that its value should remain unchanged throughout its scope. This is particularly useful in programming as it promotes immutability, lessening errors from inadvertently changing values.
Using `const` in Different Contexts
- const with variables: Declaring a variable as constant ensures that its value cannot be changed later in the code.
Code Snippet:
const int MAX_VALUE = 100;
- const with pointers: You can declare a pointer to a constant type, which prevents changes to the data being pointed to, or a constant pointer, which prevents the pointer itself from pointing elsewhere.
Code Snippet:
const int* ptrToConst = &someInt; // Pointer to constant integer
int* const constPtr = &someInt; // Constant pointer to integer
- const member functions: A member function declared as `const` cannot modify any member variables or call non-const member functions.
Code Snippet:
class MyClass {
public:
void doSomething() const {
// Can't modify member variables here
}
};
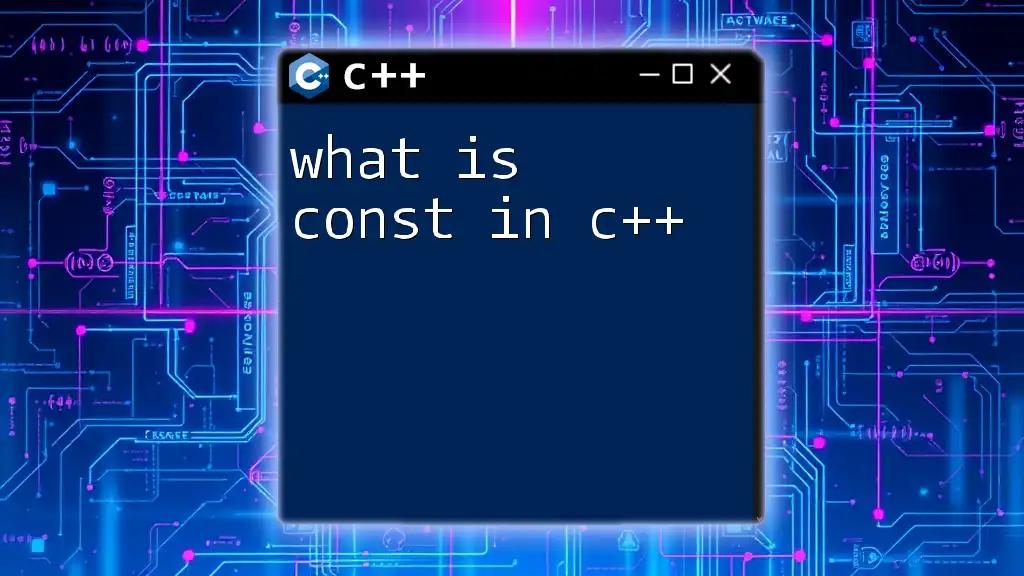
Working with `static const` in C++
Static Class Member Variables
To declare a `static const` member variable in a class, you use the `static` and `const` keywords together within the class definition. It is important to note that the `static const` variables must be defined outside the class if they require a definition (e.g., for non-integral types).
Example:
class Configuration {
public:
static const int MAX_CONNECTIONS;
};
// Definition outside the class
const int Configuration::MAX_CONNECTIONS = 10;
Initialization of `static const`
Initializing `static const` member variables can be done right in the class definition if it is of integral type (like `int`, `float`, etc.). For other types, you need to provide an out-of-class definition.
Example:
class Config {
public:
static const int SERVICE_PORT = 8080; // Direct initialization for integral type
static const std::string SERVICE_NAME; // Declaration without initialization
};
// Out-of-class definition
const std::string Config::SERVICE_NAME = "MyService";
`static const` and Enum
Another common usage of `static const` is with enums. Using `enum` with `static const` provides better type safety and easier management of constant values, making the code clearer and more maintainable.
Code Snippet:
class Shape {
public:
enum { CIRCLE, SQUARE, TRIANGLE };
static const int DEFAULT_SHAPE = CIRCLE;
};

Scope and Lifetime of `static const`
The scope of a `static const` variable is confined to the block in which it is declared, but its lifetime lasts for the duration of the program. Unlike regular `const` variables that exist within the stack, `static const` exists in the data segment, preserving its value for the entirety of the program's execution.

Common Use Cases for `static const`
Constants in Classes
`static const` is particularly useful for defining fixed settings or parameters within classes that do not change. This provides clarity in code and ensures no unintentional modifications occur.
Code Snippet:
class Circle {
public:
static const double PI; // Declaration
};
const double Circle::PI = 3.14159; // Definition
Using `static const` with Namespaces
Namespaces can also leverage `static const` to organize constants effectively. This is particularly helpful for configuration or global settings where the constants should not change.
Code Snippet:
namespace Config {
static const int RETRY_LIMIT = 5;
}

Performance Considerations
Using `static const` can lead to performance optimizations because these variables are often evaluated at compile-time. This means that when the program is running, the compiler can substitute the variable with its value directly, thereby improving performance. Additionally, it limits the footprint in memory, making it an efficient option for constants.

Conclusion
Understanding `static const` in C++ enhances your programming efficiency by providing a means for creating immutable, globally shared variables. This leads not only to cleaner code but also to fewer bugs stemming from unintended value changes.
As you develop your C++ skills, consider integrating `static const` into your coding practices to enhance clarity and maintainability.

Call to Action
Practice using `static const` in your own C++ projects, experiment with its applications, and strengthen your programming foundation. For further reading or resources, stay tuned for additional materials and updates to help with your C++ journey!

Additional Resources
Explore links to official documentation, relevant courses, or recommended books to deepen your understanding of C++. Select resources that focus on advanced C++ techniques for ongoing learning and expertise development.