In C++, `const` is a keyword used to define constant variables that cannot be modified after their initial definition, ensuring they maintain their value throughout the program.
Here’s an example of using `const` in C++:
#include <iostream>
int main() {
const int MAX_VALUE = 100; // MAX_VALUE is a constant
std::cout << "The maximum value is: " << MAX_VALUE << std::endl;
// MAX_VALUE = 200; // This line would cause a compile-time error
return 0;
}
Understanding `const`
What is `const`?
`const` is a keyword in C++ that defines a variable as constant. This means that once a variable is initialized with a value, it cannot be modified during its lifetime. The basic syntax is simple:
const type variableName;
For example:
const int maxUsers = 100;
In this case, `maxUsers` is a constant integer, and trying to change it later in the code will result in a compilation error. The primary purpose of `const` in C++ is to enforce immutability, letting the compiler know that a variable's value should not change.
The Importance of `const` in C++
Using `const` in your code offers several benefits:
Enforcing Immutability
When a variable is declared as `const`, it adds a layer of protection against accidental changes. This is particularly useful in large codebases where variables can be modified in multiple places.
Enhancing Code Readability
Declaring a variable as `const` provides immediate context to anyone reading the code. It signals that the variable's role is fixed and should not be altered, making it easier to understand the code's intent.
Potential Performance Improvements
The compiler can make optimizations when it knows certain values won't change. This can lead to more efficient code execution, especially in performance-critical applications.
Common scenarios where using `const` is beneficial include defining constants, implementing function parameters, and managing pointer behaviors.
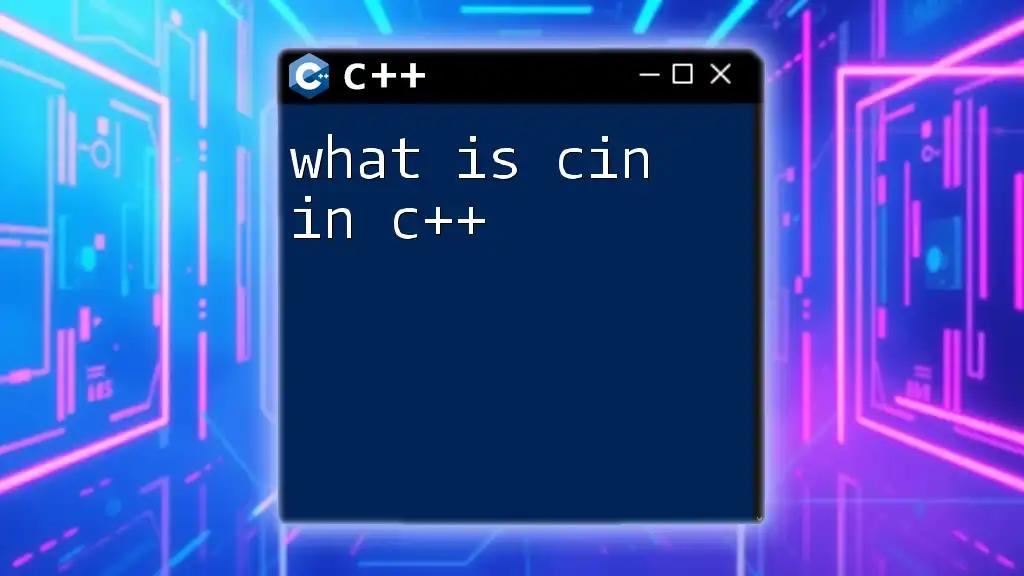
Using `const` with Variables
Declaring Const Variables
Declaring `const` variables is straightforward. For instance, if we declare:
const int maxUsers = 100;
The value of `maxUsers` is fixed at 100. Attempting to change it later, like this:
maxUsers = 200; // This will cause a compilation error
will result in a compilation error, thereby enforcing the intended immutability.
Const and Scope
The behavior of `const` variables is also affected by their scope. Inside functions, `const` variables are local, meaning they do not affect variables declared with the same name outside the function.
void function() {
const int maxUsers = 200; // This maxUsers is different from the global one
}
In classes, `const` can be used to define class-level constants, further enforcing the principle of immutability.

Using `const` with Pointers
Pointer Basics
Pointers in C++ are variables that store the memory address of another variable. When declaring pointers with `const`, it’s important to understand the distinction between pointer-to-const and const-pointer.
Pointer-to-const vs. Const Pointer
A pointer-to-const means that the value pointed to cannot be altered through that pointer. Here’s an example:
const int* ptr = &maxUsers;
In this case, `ptr` points to a constant integer, meaning you cannot change the value of `maxUsers` using `ptr`.
A const-pointer, on the other hand, means that the pointer itself cannot be changed to point to another address after initialization:
int* const pConst = &someVariable;
Here, while `pConst` can change the value of `someVariable`, it cannot point to a different variable.
When to Use Each
Choosing between pointer-to-const and const pointer typically depends on the specific use case—use pointer-to-const when you want to ensure that the pointed-to value remains unchanged, and const pointer when you want to maintain the integrity of the pointer itself.

Using `const` with Functions
Const Function Parameters
Using `const` in function parameters allows you to pass arguments without the risk of modifying the original values. For example, passing a constant reference would look like this:
void display(const int& value);
This function will not modify `value`, ensuring safety in your code.
Const Member Functions
Const member functions are those that promise not to modify any member variables of the class. They are declared by adding `const` after the function signature:
class Sample {
public:
void show() const; // This function cannot modify class members
};
When a member function is declared as `const`, it can be called on constant instances of the class, enforcing read-only access on the object.
Return Type as Const
In C++, you can also have a function return a const value. This is useful when you want to return a reference to a private member, ensuring that it cannot be changed. An example would be:
const int& getMax() const;
This function returns a constant reference to the maximum value without allowing modification.

Common Pitfalls of Using `const`
Misunderstandings About `const`
Many developers misunderstand how `const` works, especially in the context of function overloading. Function overloads can change based on whether the parameter or return type is const, leading to unexpected behaviors.
Debugging Issues Related to `const`
When working with `const`, you might encounter errors that are not immediately apparent. For instance, trying to assign a non-const reference to a const variable will lead to compilation errors. One practical tip for troubleshooting is to read error messages carefully, as they often point to where the immutability violation occurred.

Best Practices for Using `const`
To maximize the advantages of `const`, consider the following guidelines:
- Use `const` by default: If a variable doesn’t need to change, declare it as `const`. This practice improves code safety and clarity.
- Pass parameters by const reference: This avoids unnecessary copies and protects the original data.
- Utilize const member functions: Use them to document your intentions clearly and allow use on const instances.
By maintaining good practices around the use of `const`, you can significantly improve the overall quality and maintainability of your code.

Conclusion
Understanding what is const in C++ is crucial for writing robust and maintainable code. By using `const` effectively, you can enforce immutability, enhance readability, and even improve performance. Explore how you can implement `const` in your own code and see the benefits firsthand. It's time for you to practice using `const` in your daily coding efforts!