In C++, `int` is a fundamental data type used to declare variables that can store integer values, which are whole numbers without decimal points.
Here's a simple example of using `int` in C++:
#include <iostream>
int main() {
int age = 25;
std::cout << "Age: " << age << std::endl;
return 0;
}
Understanding Data Types in C++
What Are Data Types?
In programming, data types serve as classifications that define what kind of data can be stored and manipulated. They dictate how much memory is allocated for a variable and the types of operations that can be performed on that variable.
In C++, data types are crucial because they provide type safety, ensuring that operations are performed on compatible data types. This promotes stability and performance optimization in applications.
Why C++ Uses Data Types
C++ employs data types to enhance type safety and performance. When a variable's type is known, the compiler can optimize code execution, prevent errors, and manage memory efficiently. For example, when a variable is declared as `int`, the compiler knows it must allocate 4 bytes (in most systems).
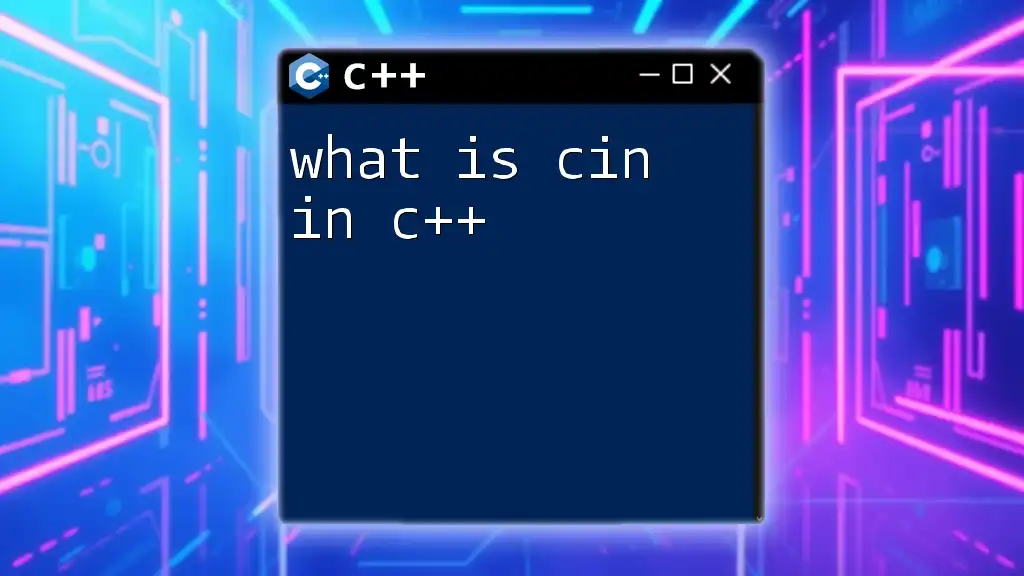
The `int` Data Type in C++
Definition of `int`
The `int` keyword in C++ is a fundamental data type used to represent integer values. It can store positive and negative whole numbers, making it versatile in applications that require counting, indexing, or calculations without decimal points.
Characteristics of `int`
- Size: The size of an `int` can vary by platform. On most modern systems, it is typically 4 bytes.
- Range: The permissible range for a standard 4-byte `int` is usually from -2,147,483,648 to 2,147,483,647. This range can vary based on different architectures.
Variants of `int`
Signed vs. Unsigned
Integers in C++ can be signed or unsigned. A signed integer can hold both positive and negative values, while an unsigned integer can only represent non-negative values.
Example Code Snippet:
int signedInt = -10; // A signed integer
unsigned int unsignedInt = 10; // An unsigned integer
Short and Long Integers
C++ also provides `short` and `long` data types. The `short` type generally uses less memory and has a smaller range, while the `long` type can represent larger values.
Example Code Snippet:
short shortInt = 32767; // Example of short
long longInt = 2147483647; // Example of long
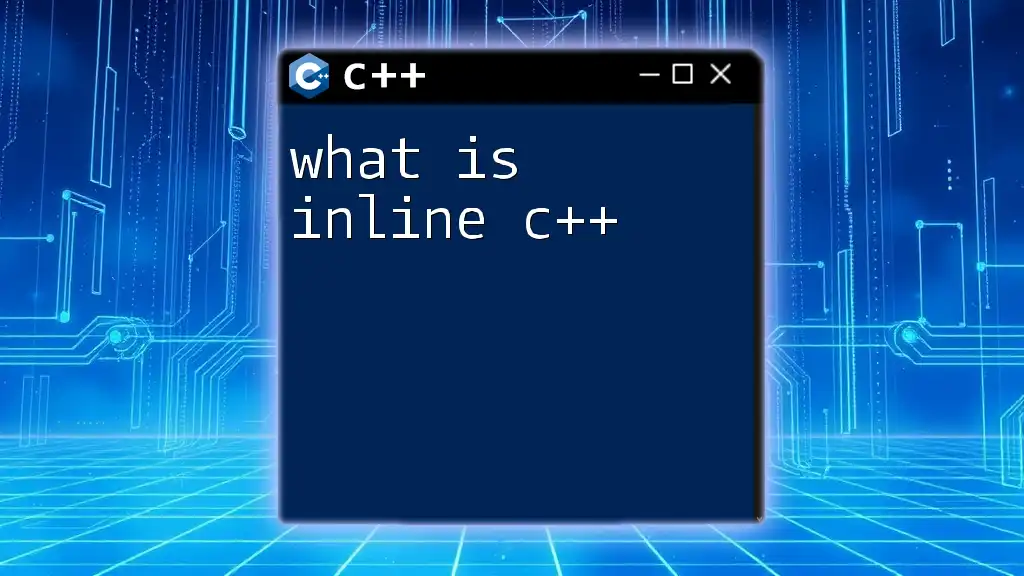
Declaring and Initializing `int`
Syntax for Declaration
Declaring an `int` in C++ is quite straightforward. You specify the data type followed by the variable name.
General syntax:
int variableName;
Initializing an `int`
To initialize an `int`, assign a value during declaration. This allocates memory for that variable and sets it to a specific number.
Example Code Snippet:
int number = 42; // Initialization of an int variable
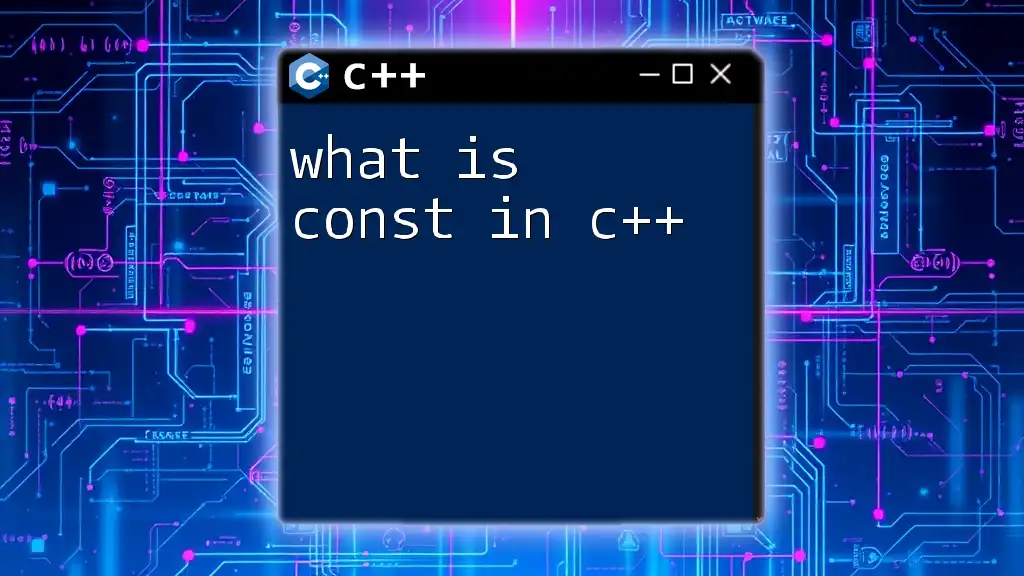
Performing Operations with `int`
Arithmetic Operations
With `int`, you can perform various arithmetic operations such as addition, subtraction, multiplication, and division. Each of these operations is essential for mathematical computations in your programs.
Example Code Snippet:
int a = 5, b = 10;
int sum = a + b; // Addition, sum will be 15
int product = a * b; // Multiplication, product will be 50
Increment and Decrement Operators
C++ supports increment (`++`) and decrement (`--`) operators, which allow you to easily modify an `int` variable's value by one.
Example Code Snippet:
int count = 0;
count++; // Increment, count will now be 1
count--; // Decrement, count will revert back to 0
Type Casting with `int`
Sometimes, you may need to convert a variable from one type to another. This process is known as type casting. It can be implicit (automatically handled by the compiler) or explicit (specific to the programmer).
Examples of implicit and explicit type casting:
double pi = 3.14;
int wholeNumber = (int)pi; // Explicit casting, wholeNumber will be 3
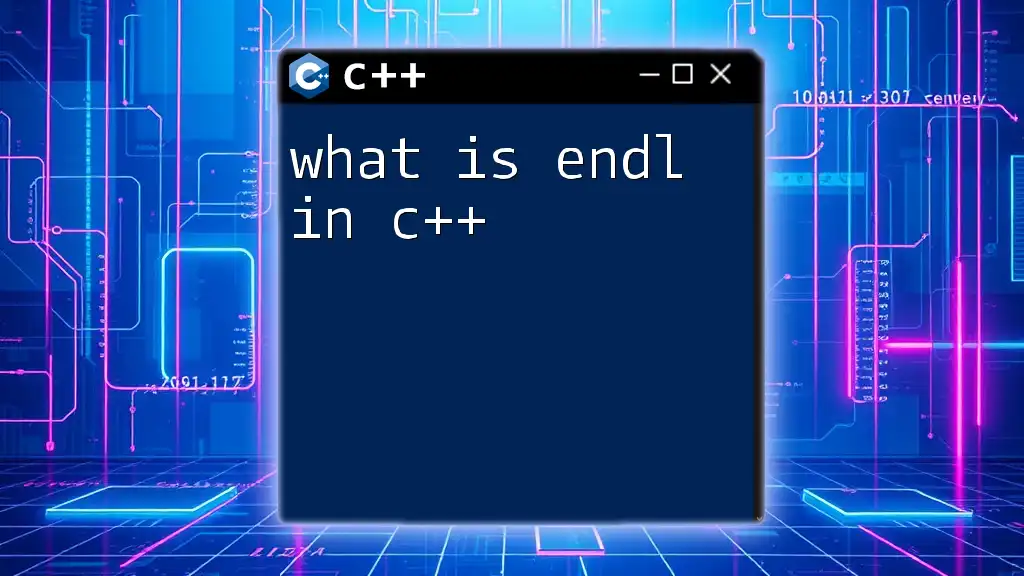
Common Errors Related to `int`
Type Mismatch
A type mismatch occurs when operations are performed between incompatible types, leading to compilation errors. This is often a common error for beginners. It's important to ensure that you are manipulating compatible types.
Overflow and Underflow
When an `int` variable exceeds its maximum or minimum limit, it leads to overflow or underflow. This can cause unexpected behavior in your programs.
Example Code Snippet:
int largeNumber = 2147483647; // Example of maximum int value
largeNumber++; // This will cause overflow
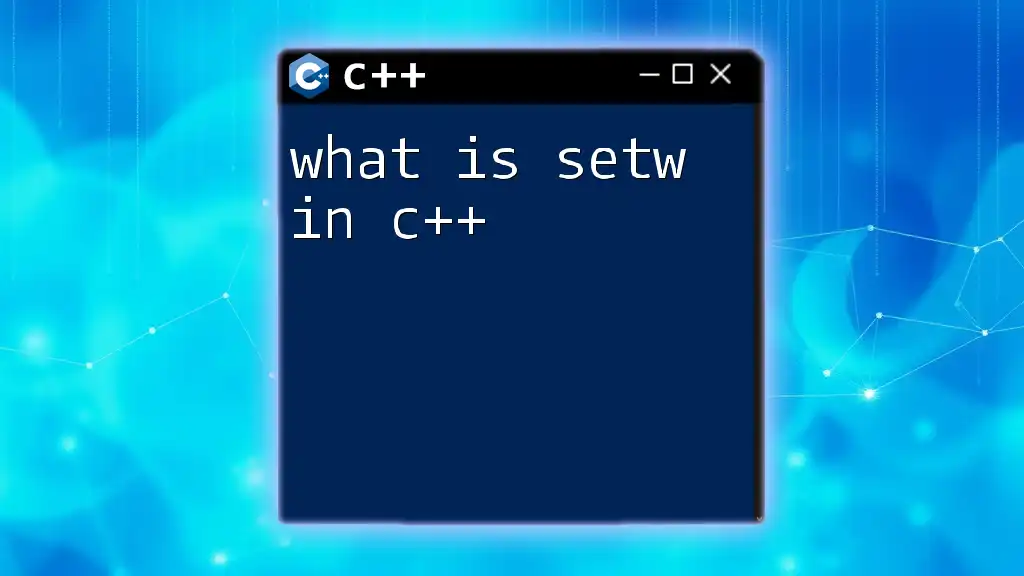
Best Practices When Using `int`
Choosing the Right Integer Type
While `int` is commonly used, always consider the range of values you expect and choose the most appropriate integer type (`int`, `short`, `long`, or `unsigned`). This can help prevent errors like overflow.
Consistency and Naming Conventions
Consistency is key in programming. Use clear and meaningful names for your integer variables, avoiding ambiguous terms or magic numbers that can confuse readers of your code.
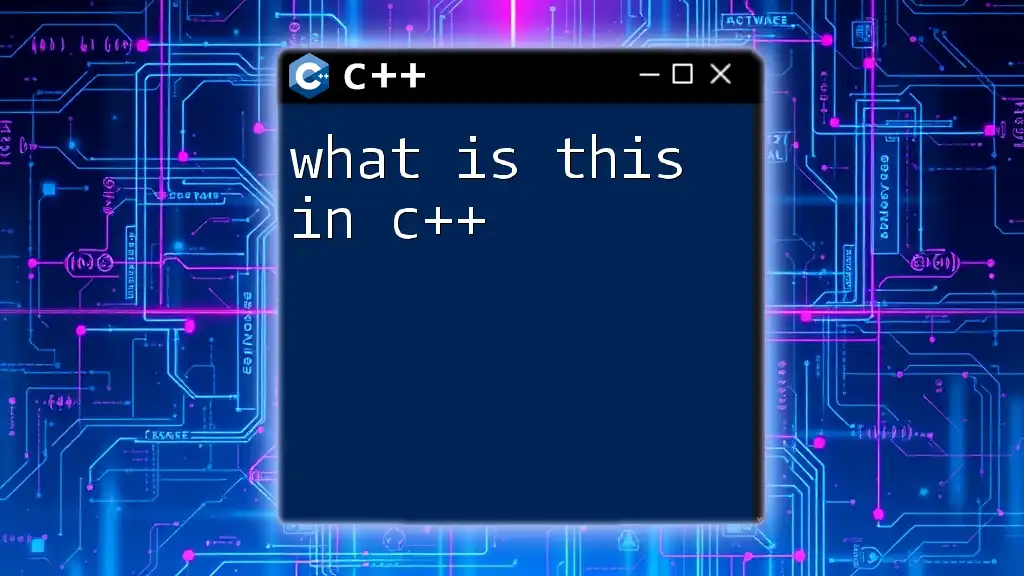
Conclusion
Understanding what is `int` in C++ is fundamental for anyone delving into the language. The `int` data type plays a vital role in various programming tasks, from simple counting to complex calculations. Embrace the nuances of integer data types, as they form the backbone of numerical operations in C++.
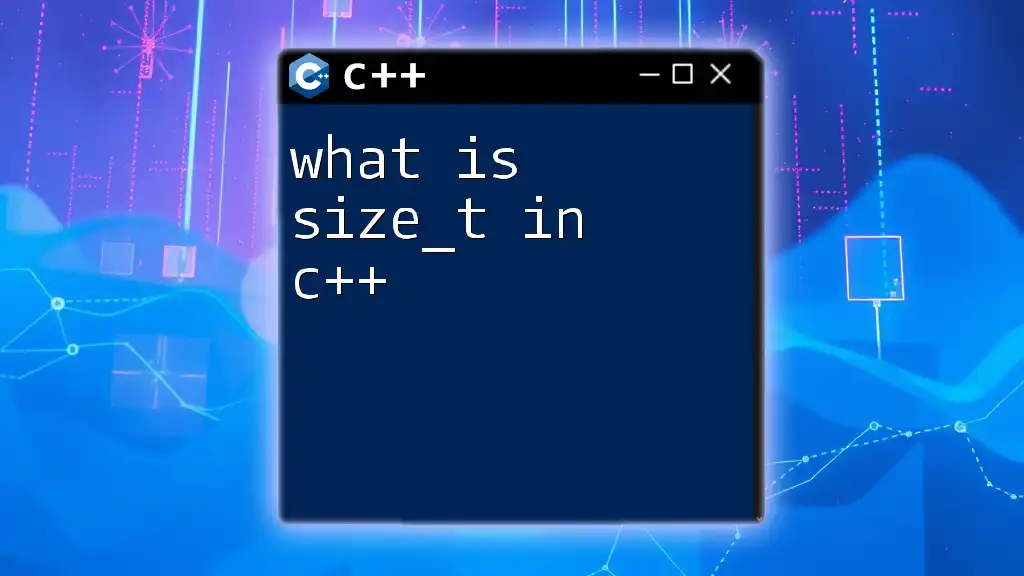
Call to Action
Stay tuned and follow our company for more easy-to-follow tips and tricks in C++. Empower your C++ journey by downloading our free beginner’s resource and take your first step towards mastering this powerful language!