Visual C++ is an integrated development environment (IDE) from Microsoft that allows developers to create applications using the C++ programming language, leveraging tools for building, debugging, and compiling code.
Here's a simple code snippet demonstrating a basic "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Visual C++?
Visual C++ is a powerful integrated development environment (IDE) provided by Microsoft for writing, debugging, and compiling C++ applications. It offers a comprehensive suite of tools that streamline the software development process and support a variety of programming paradigms.
Historically, Visual C++ dates back to the early 1990s and has evolved significantly over the years, adapting to the ever-changing landscape of programming languages and development needs. Today, it supports modern C++ standards and features, making it a preferred choice for many developers.
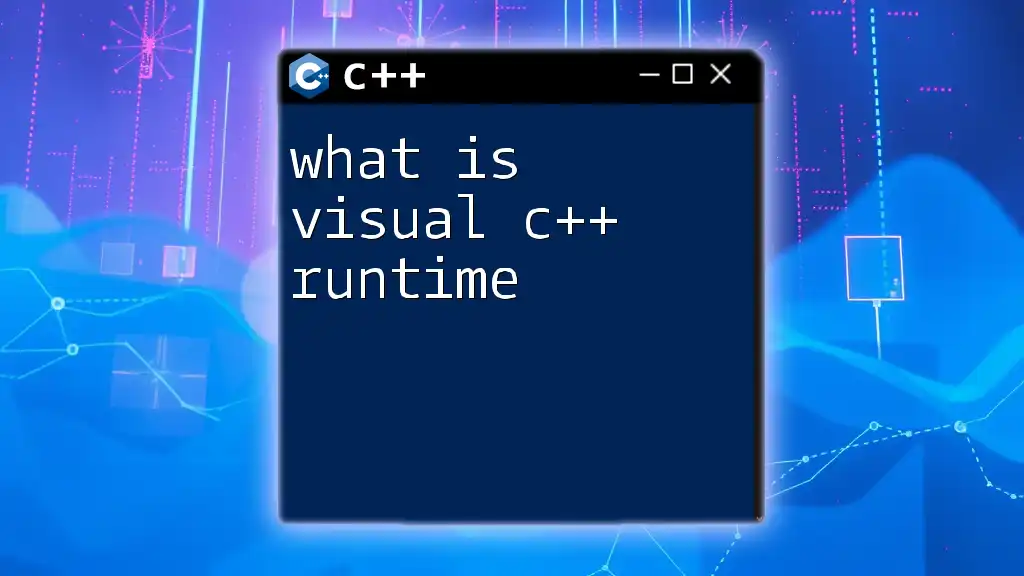
Key Features of Visual C++
Integrated Development Environment (IDE)
The IDE is one of the hallmarks of Visual C++, providing a user-friendly interface for writing code. It includes several essential tools that enhance productivity:
- Code Editor: Syntax highlighting, code completion, and code snippets make writing code faster and more efficient.
- Debugging Tools: Visual C++ offers a robust debugging environment with features such as step-through debugging, variable watches, and immediate windows, ensuring that developers can easily identify and fix issues.
Support for Standard C++
Visual C++ prides itself on its compliance with the C++ standards, allowing developers to write code that is both efficient and portable. This compliance ensures that developers can use the latest features of the language, such as lambda expressions, smart pointers, and more.
Using Standard C++ in Visual C++ not only enhances code portability but also empowers developers to utilize a vast array of libraries and frameworks that conform to these standards.
C++/CLI Support
C++/CLI is an extension of C++ that integrates seamlessly with the .NET framework. This feature allows developers to create applications that leverage both managed and unmanaged code, enabling interoperability between different programming languages.
The benefits of C++/CLI in Visual C++ include the ability to access .NET libraries, which can significantly speed up development time while maintaining high performance.
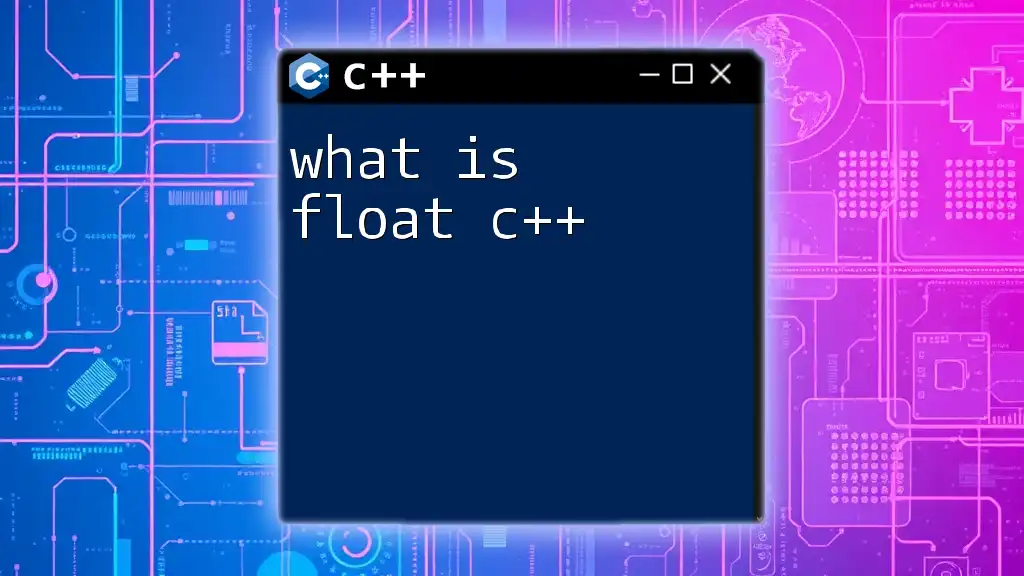
Getting Started with Visual C++
Installation Guide
To get started with Visual C++, first, you'll need to download and install Microsoft Visual Studio. The process is straightforward:
- Visit the Visual Studio website and choose the Community edition, which is free for individual and small-scale use.
- Follow the installation instructions and ensure that you select the C++ components during setup.
- After installation, confirm your system specifications meet the required standards for optimal performance.
Creating Your First Project
Once you have Visual C++ installed, it's time to create your first project.
- Setting Up a New Project: Launch Visual Studio, click on "Create a new project," and select the C++ project template that best fits your needs (e.g., Console Application).
- Sample Project - "Hello World!": Let’s walk through creating a basic C++ console application that prints "Hello, World!" to the screen. Use the code below:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
By compiling and running this code, you'll see the familiar greeting in the console, marking your entry into C++ programming.
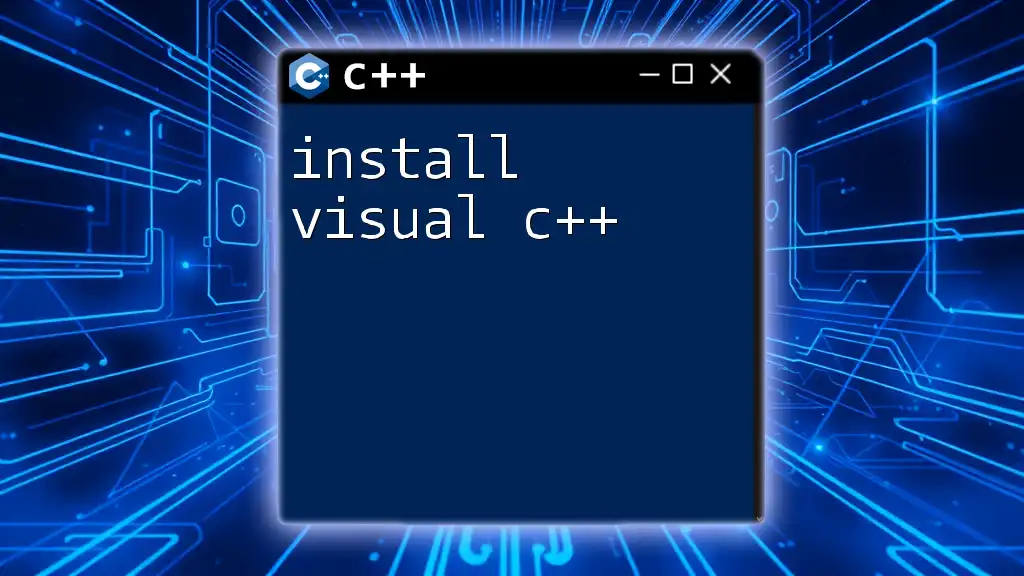
Visual C++ Libraries and Frameworks
MFC (Microsoft Foundation Classes)
MFC is a set of classes that wrap Windows API functionality, streamlining the development of Windows-based applications. With MFC, you can create complex, feature-rich applications quickly.
A typical MFC application structure includes classes for Windows, controls, and documents, allowing developers to focus on application logic rather than low-level API details.
ATL (Active Template Library)
The Active Template Library is another powerful feature of Visual C++, specifically designed for creating COM (Component Object Model) objects and ActiveX controls. ATL offers a lightweight alternative to MFC, making it an excellent choice for developers focused on performance.
Here's a simple example of defining a COM object using ATL:
#include <atlbase.h>
#include <atlcom.h>
class ATL_NO_VTABLE MyCOMObject :
public CComObjectRootEx<CComSingleThreadModel>,
public CComCoClass<MyCOMObject, &CLSID_MyCOMObject>
{
// COM implementation
};
STL (Standard Template Library)
STL is a powerful library that provides several generic classes and functions that can significantly reduce development time. It includes algorithms and data structures like vectors, lists, and maps.
Here’s a practical example using a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (const auto& num : numbers) {
std::cout << num << " ";
}
return 0;
}
This example demonstrates how easy it is to work with dynamic arrays in C++ using STL.
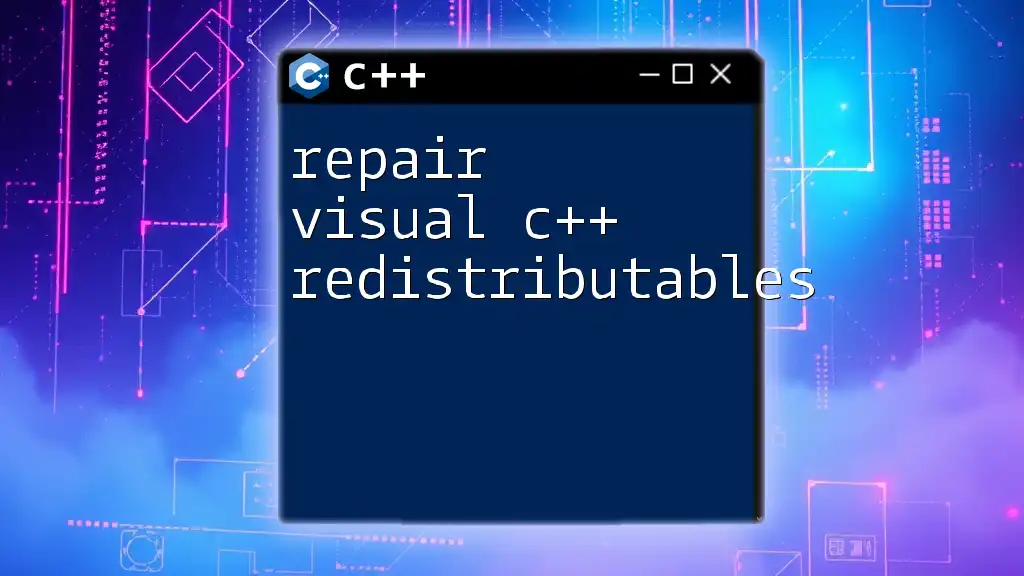
Debugging and Testing in Visual C++
Debugging Tools in Visual C++
Debugging is an essential part of the development process. Visual C++ offers numerous debugging features that streamline this process.
- You can set breakpoints in your code to pause execution and inspect variable states.
- The watch windows allow you to track variable values in real-time as you step through your code, making it easier to pinpoint issues.
Unit Testing in Visual C++
Visual C++ makes it easy to implement unit testing using frameworks like Google Test. Testing is crucial for ensuring that code functions as expected. Here's a simple example of a unit test using Google Test:
#include <gtest/gtest.h>
int Sum(int a, int b) {
return a + b;
}
TEST(SimpleTest, SumFunction) {
EXPECT_EQ(Sum(1, 1), 2);
EXPECT_EQ(Sum(2, 3), 5);
}
By integrating unit tests into your workflow, you can maintain code quality and facilitate easier debugging.
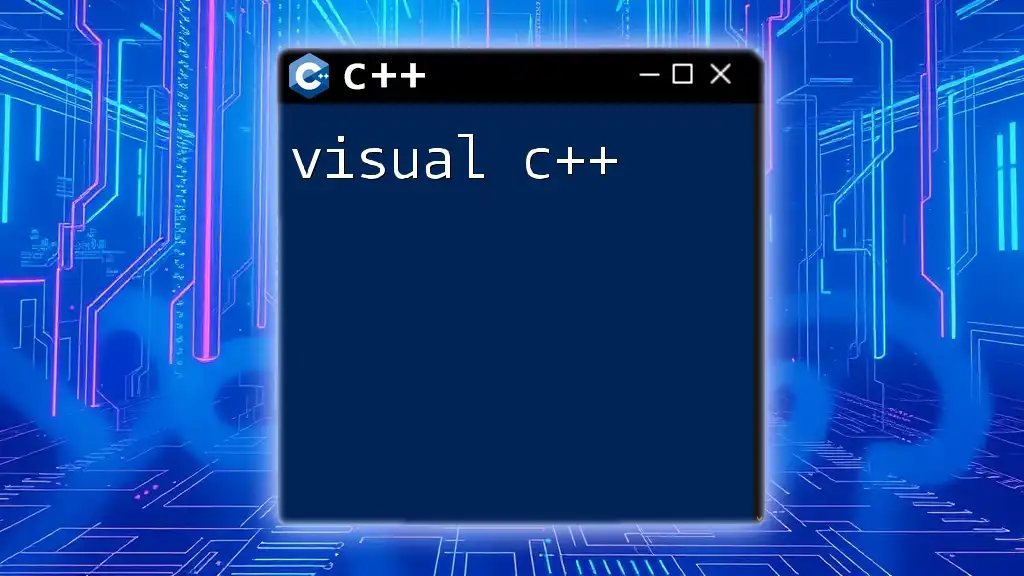
Common Use Cases for Visual C++
Game Development
Visual C++ is highly regarded in the game development industry, notably for its use in Unreal Engine. The engine’s reliance on C++ allows for high-performance gaming applications, giving developers the flexibility to craft intricate gaming experiences.
Application Development
Visual C++ is also used for creating desktop applications on Windows. Developers can leverage the powerful GUI capabilities provided by MFC and Win32 API to build robust applications tailored to specific user needs.
Embedded Systems
Another significant application for Visual C++ is in the field of embedded systems. With support for low-level programming, developers can create efficient code optimized for performance in resource-constrained environments.
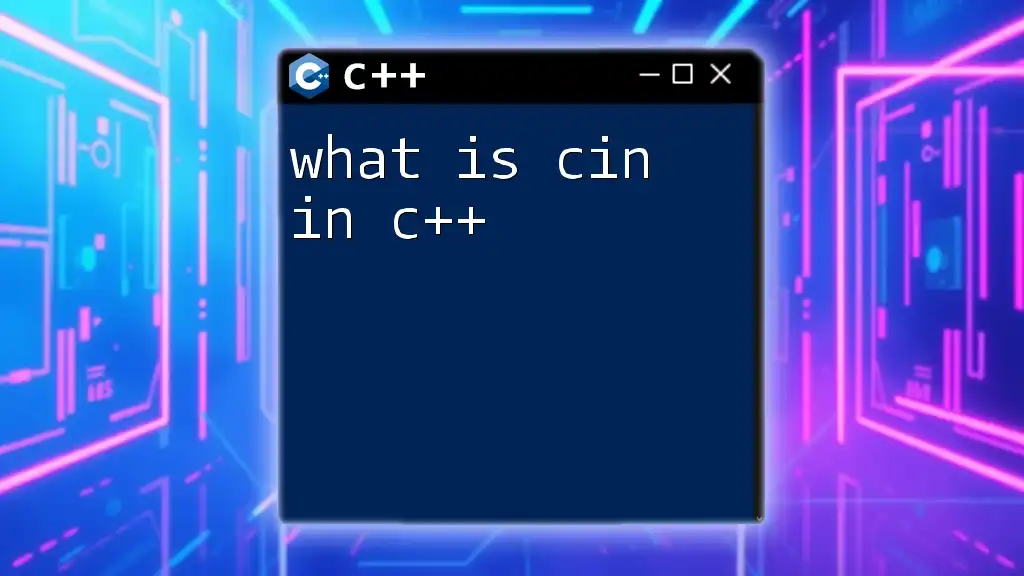
Advantages of Using Visual C++
Performance Benefits
One of the most significant advantages of Visual C++ is its performance capabilities. C++ is known for its efficient memory usage and execution speed, making it a top choice for performance-critical applications.
Extensive Library Support
Visual C++ provides access to a vast array of Windows libraries and APIs, allowing developers to harness powerful functionalities and enhance their applications with minimal effort.
Community and Resources
The extensive community surrounding Visual C++ means that developers can find an abundance of resources such as forums, online documentation, and tutorials. This support can be invaluable when encountering challenges during development.
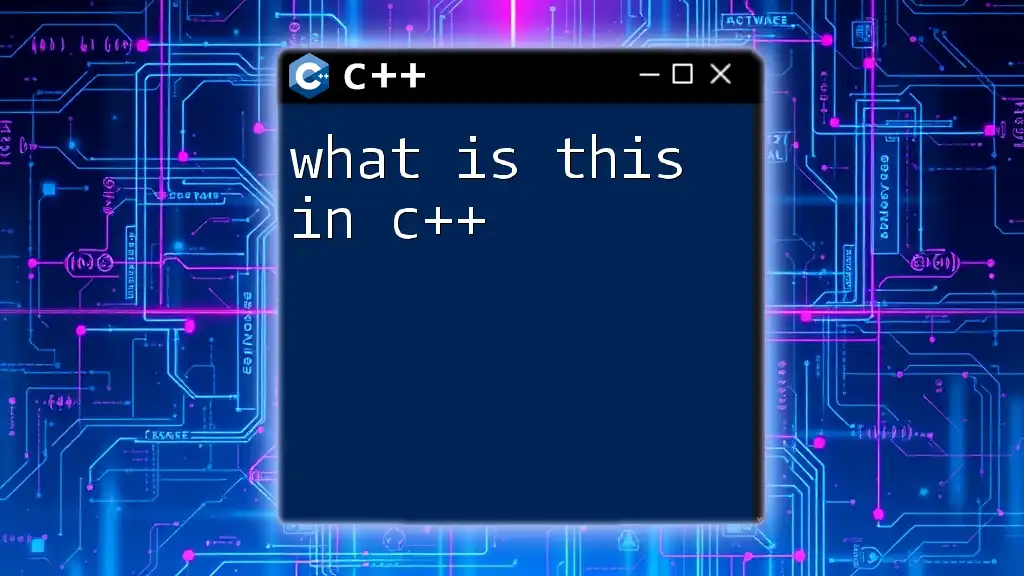
Conclusion
Visual C++ remains a vital tool in the programming landscape, revered for its robust features and performance capabilities. By exploring what Visual C++ can offer, developers can deepen their understanding and leverage the language in various applications. With the right resources at your disposal, diving into Visual C++ can open new avenues for software development.
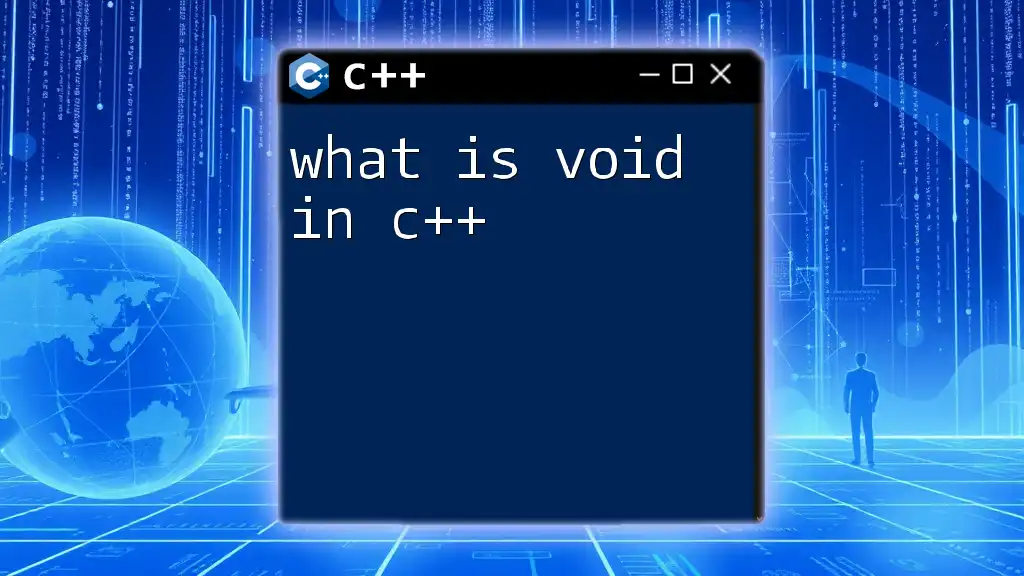
Further Resources
For further exploration, check out the official documentation on Microsoft’s website, or consider enrolling in online courses that can enhance your Visual C++ skills. Additionally, books on C++ programming and software development can offer both foundational knowledge and advanced techniques that can propel your understanding even further.