Llama CPP is a lightweight and efficient C++ framework designed for rapid application development, providing simple abstractions for common programming tasks.
Here’s a simple code snippet demonstrating the basic syntax in C++:
#include <iostream>
int main() {
std::cout << "Hello, Llama CPP!" << std::endl;
return 0;
}
Understanding Llama.cpp
What is Llama.cpp?
Llama.cpp is a modern C++ framework that streamlines and optimizes command execution within C++ applications. Designed with an emphasis on user-friendliness and performance, Llama.cpp aims to bridge the complexity of traditional C++ syntax with straightforward command implementations. The essence of Llama.cpp lies in its ability to abstract complex commands into simpler, more approachable structures, making it an invaluable tool for both novice and experienced C++ developers.
Key features of Llama.cpp include:
- Performance Optimization: Leveraging advanced compiler techniques to generate efficient executable code.
- User-Friendly Syntax: Simplifying frequently used commands, enhancing readability and ease of use.
The Genesis of Llama.cpp
Llama.cpp emerged from a need to facilitate rapid development cycles in C++ programming by addressing common pain points that developers encounter. Since its inception, it has evolved through contributions from a diverse range of open-source developers and organizations committed to enhancing C++ usability.
The evolution of Llama.cpp reflects the growing need for modern development tools that prioritize both speed and simplicity, catering to a broad spectrum of users, from hobbyists to industry veterans.
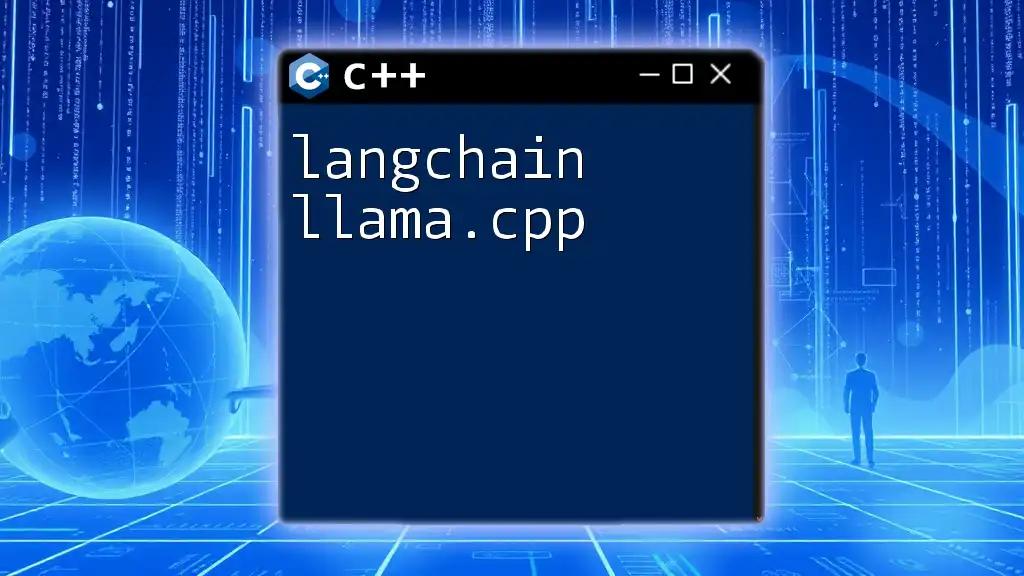
Key Features of Llama.cpp
Performance Enhancements
One of the standout attributes of Llama.cpp is its performance optimization capabilities. Benchmarks reveal that applications built with Llama.cpp often outperform those using traditional C++ methodologies. Performance improvements come from well-optimized command execution patterns and reduced overhead in function calls.
For example, consider a scenario where a simple computation task needs to be executed. A comparison between traditional C++ and a Llama.cpp implementation would showcase significant runtime advantages.
// Traditional C++ computation
#include <iostream>
int main() {
int result = 0;
for(int i = 0; i < 1000000; ++i) {
result += i;
}
std::cout << "Result: " << result << std::endl;
return 0;
}
// Using Llama.cpp
#include <llama.h>
int main() {
int result = llama::compute([](int i) { return i; }, 1000000);
llama::output("Result: ", result);
return 0;
}
In the above example, Llama.cpp encapsulates the complexity of the loop, streamlining the command to focus on the end result while maintaining efficiency.
User-Friendly Interface
Llama.cpp champions simplicity by introducing a user-friendly interface that makes C++ more accessible. By reimagining standard C++ functions, it creates an intuitive command structure that minimizes the cognitive load on developers.
For instance, standard file operations can often involve cumbersome syntax. Llama.cpp simplifies this:
// File operations with standard C++
#include <fstream>
#include <iostream>
int main() {
std::ofstream outputFile("example.txt");
outputFile << "Hello, World!" << std::endl;
outputFile.close();
return 0;
}
// File operations with Llama.cpp
#include <llama.h>
int main() {
llama::write("example.txt", "Hello, World!");
return 0;
}
This transformation reduces the amount of boilerplate code, making it easier for developers to focus on functionality rather than syntax.
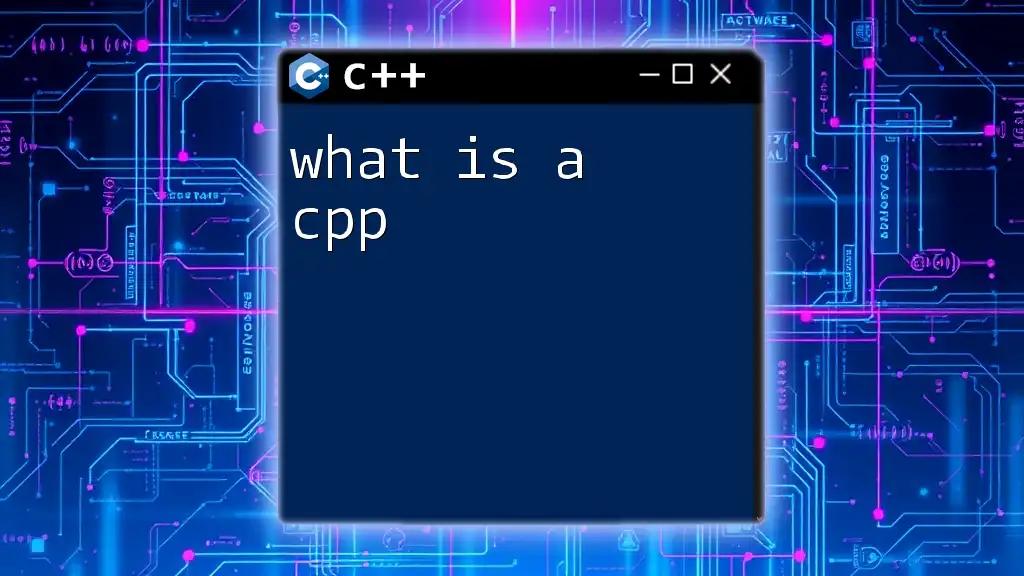
Getting Started with Llama.cpp
Setting Up Your Development Environment
To begin using Llama.cpp, you'll need to set up your development environment. The prerequisites include:
- A C++ compiler that supports C++17 or later.
- The Llama.cpp library, which can usually be installed via package managers like vcpkg or downloaded from the official repository.
Instructions for installation will vary depending on your system, but a typical setup procedure involves the following steps:
-
Install the library:
- On Windows, use a package manager like vcpkg or clone the repository directly.
- On macOS, install with Homebrew.
- On Linux, use apt-get or compile from source.
-
Link the library to your development environment following your IDE’s instructions.
-
Verify the installation by compiling a simple Llama.cpp program.
Writing Your First Llama.cpp Program
Once your environment is set up, writing your first program is a straightforward process. A basic "Hello, World!" example can effectively illustrate the fundamentals of Llama.cpp.
#include <llama.h>
int main() {
llama::output("Hello, World!");
return 0;
}
This concise program demonstrates how Llama.cpp can simplify even basic tasks, encouraging developers to embrace its capabilities from the outset.
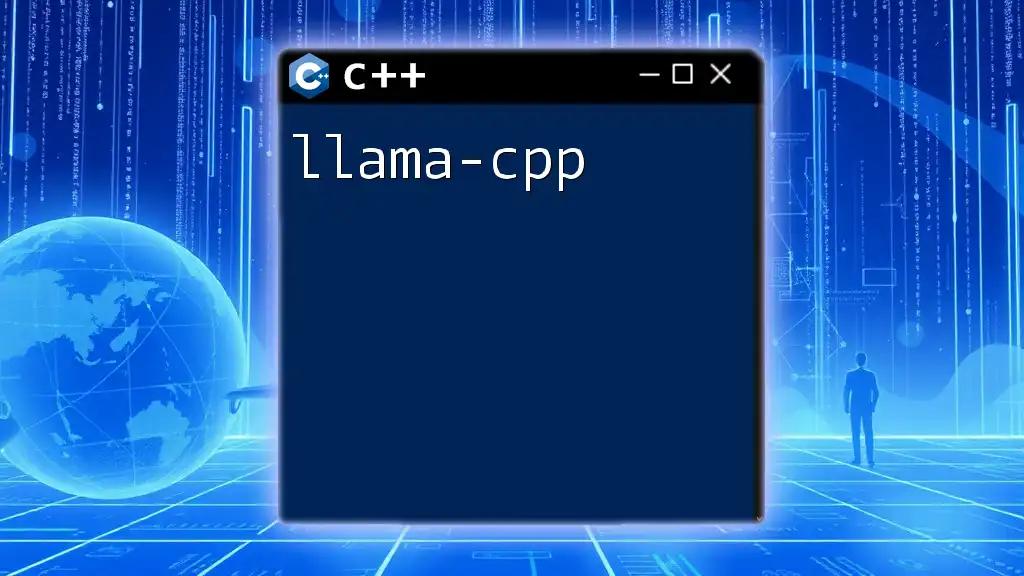
Advanced Features of Llama.cpp
Command Line Interface (CLI) Capabilities
Llama.cpp offers extensive support for Command Line Interfaces (CLI), allowing developers to create applications that interact seamlessly with user input via terminal commands. Its built-in CLI functionalities enable developers to parse input and manage outputs effortlessly.
Setting up a simple CLI application might look like this:
#include <llama.h>
int main(int argc, char* argv[]) {
if (argc > 1) {
llama::output("Welcome, " + std::string(argv[1]));
} else {
llama::output("Welcome, Guest!");
}
return 0;
}
In this example, the program checks for command-line arguments, demonstrating Llama.cpp's capabilities with minimal syntax.
Integration with Other Libraries
One notable aspect of Llama.cpp is its ability to integrate seamlessly with other popular C++ libraries such as Boost and the Standard Template Library (STL). This compatibility allows developers to leverage the strengths of multiple libraries to enhance their applications.
For instance, integrating with Boost to create a threaded application would look like this:
#include <llama.h>
#include <boost/thread.hpp>
void threadFunction(int id) {
llama::output("Thread ID: " + std::to_string(id));
}
int main() {
boost::thread t1(threadFunction, 1);
boost::thread t2(threadFunction, 2);
t1.join();
t2.join();
return 0;
}
This snippet highlights how Llama.cpp's user-friendly syntax complements the more complex threading capabilities provided by Boost.
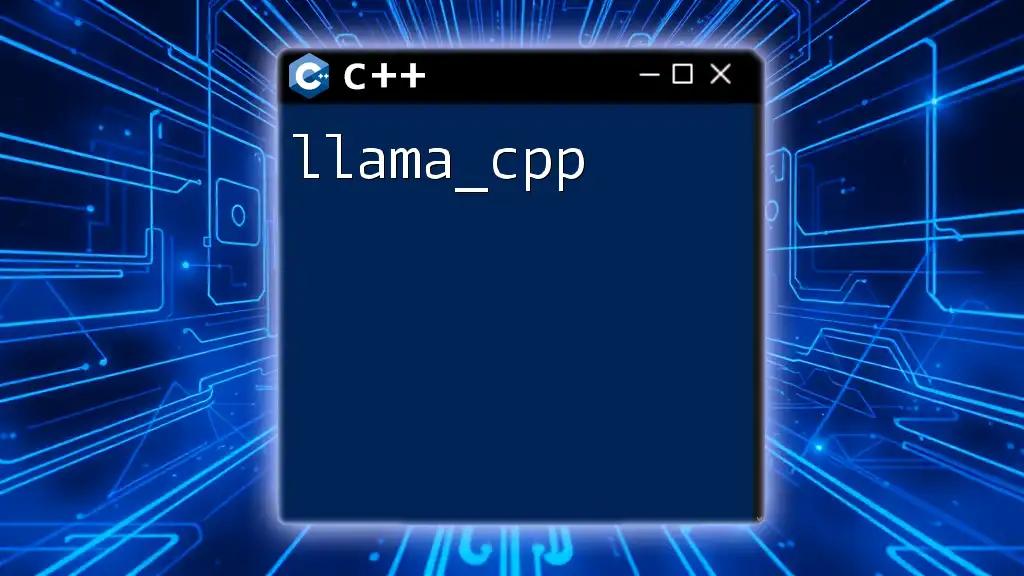
Best Practices for Using Llama.cpp
Coding Standards
When working with Llama.cpp, following recommended coding standards is vital. This includes using consistent naming conventions, structuring your code logically, and incorporating comprehensive documentation. Clear comments enhance readability and help fellow developers navigate your work with ease.
Performance Tuning Tips
To ensure optimal performance in your Llama.cpp applications, consider these strategies:
- Profile your code to identify bottlenecks. Using profiling tools will help you understand where optimizations are needed.
- Minimize function calls in performance-critical sections by inlining when appropriate.
- Utilize built-in Llama features that are optimized for speed and efficiency.
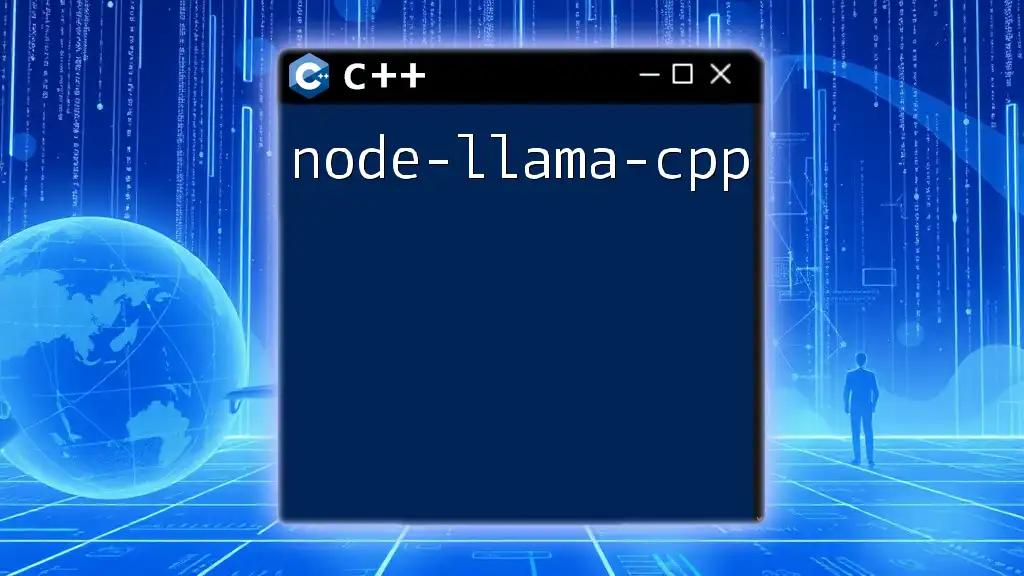
Community and Resources
Where to Find Support
As you delve into Llama.cpp, you’ll find a supportive community ready to assist you. Engage with fellow developers on dedicated forums, such as Stack Overflow or GitHub discussions. These platforms offer invaluable resources, including documentation, tutorials, and real-world project examples where Llama.cpp shines.
Future of Llama.cpp
The future of Llama.cpp looks promising, with ongoing contributions aimed at expanding its capabilities. Upcoming features and enhancements are regularly discussed in community forums, and you are encouraged to participate in contributing to further versions. Your insights and contributions can help shape the next generation of Llama.cpp.
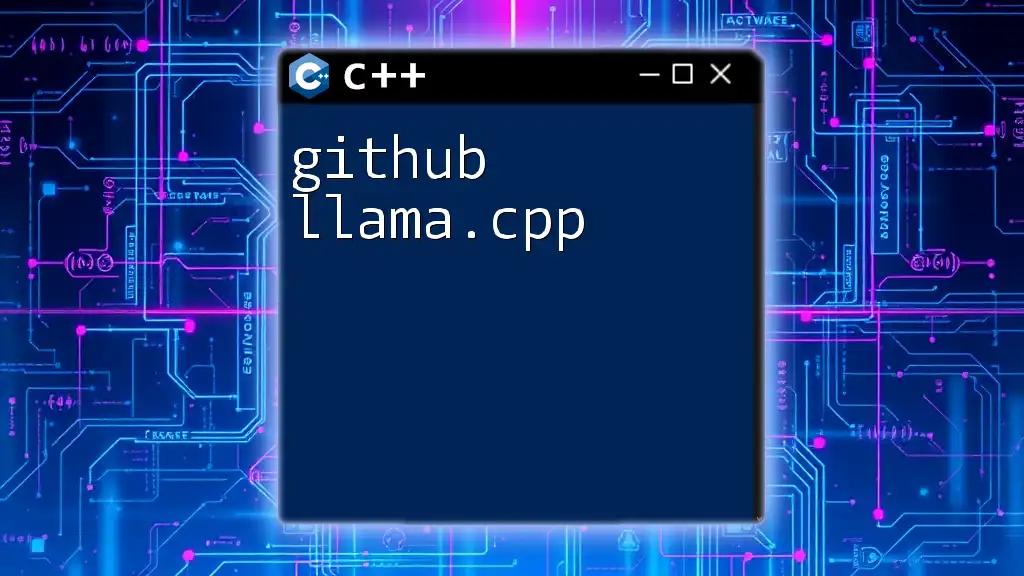
Conclusion
Llama.cpp serves as a robust solution for modern C++ programming, offering significant benefits in performance and simplicity. By encouraging developers to adopt clearer syntactical constructs, it has positioned itself as an essential tool for both new and experienced programmers. Exploring Llama.cpp can help you elevate your C++ development experience and create more efficient applications with ease.
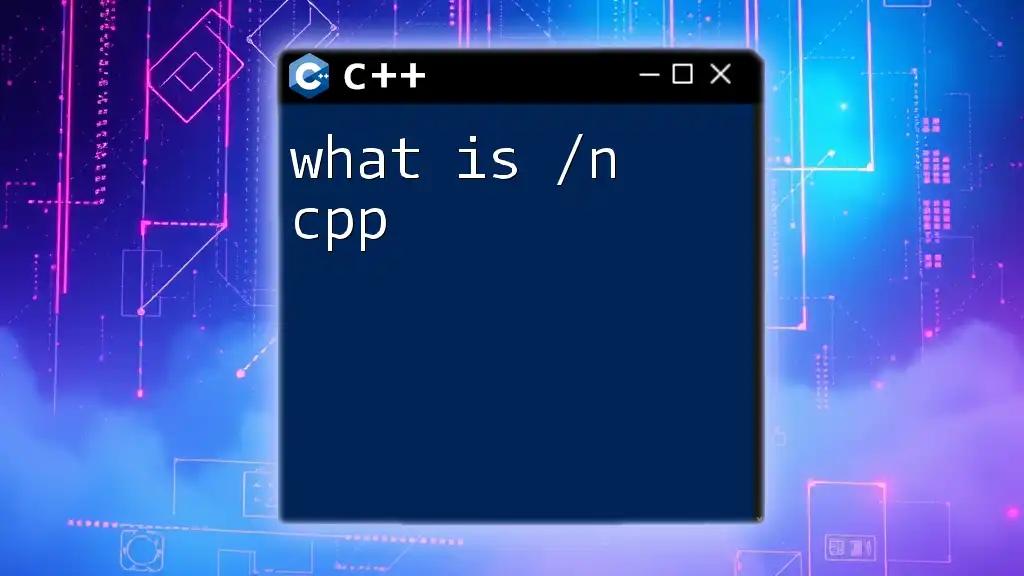
Call to Action
Ready to enhance your programming with Llama.cpp? Dive deeper into the framework, tackle some examples, and explore the community resources available. Your journey into the world of Llama.cpp awaits!