In the comparison of `vllm` and `llama.cpp`, `vllm` is optimized for efficient GPU utilization in Machine Learning tasks, while `llama.cpp` focuses on lightweight, CPU-based implementations for running large language models.
Here’s a simple code snippet demonstrating how to load a model using `llama.cpp`:
#include <llama.h>
int main() {
LlamaModel model("path/to/model");
model.load();
model.generate("Hello, world!");
return 0;
}
Understanding vllm
What is vllm?
vllm stands for "Very Lightweight Library for Memory," and it is a specialized library within the C++ ecosystem designed to optimize memory management and enhance performance. This library is particularly useful when building high-performance applications that require efficient memory usage without compromising speed.
Key Features of vllm
Performance Optimization
One of the standout features of vllm is its ability to significantly optimize performance in memory-intensive applications. This optimization is achieved through advanced memory allocation algorithms that minimize fragmentation and enhance cache coherence.
Example: Below is a simple code snippet demonstrating how vllm can optimize memory allocation:
#include <vllm/vllm.h>
int main() {
vllm::Allocator allocator;
int* myArray = allocator.allocate<int>(100); // Allocate an array of 100 integers
// Use myArray
allocator.deallocate(myArray); // Efficient deallocation
return 0;
}
In this example, the vllm allocator helps ensure that memory is allocated and freed efficiently, reducing the chances of memory leaks.
Ease of Use
vllm is known for its user-friendly interface that streamlines command usage. Developers can easily get up to speed with its simple syntax, making it accessible to beginners and experienced programmers alike.
Example: Here’s how vllm simplifies common memory management tasks:
#include <vllm/vllm.h>
void process() {
vllm::Allocator allocator;
auto myData = allocator.allocate<float[]>(512); // Allocate 512 floats
// Perform operations on myData
allocator.deallocate(myData); // Clean up
}
As seen above, vllm's straightforward function calls allow for quick memory management without diving into complex syntax.
Use Cases of vllm
Applications in Industry
vllm has found applications in various industries where performance and memory efficiency are crucial. One notable example is in the gaming industry, where resource management can significantly affect framerate and user experience.
Example: A game development team utilizing vllm for real-time memory handling reported a 20% increase in frame rates, owing to reduced computational overhead.
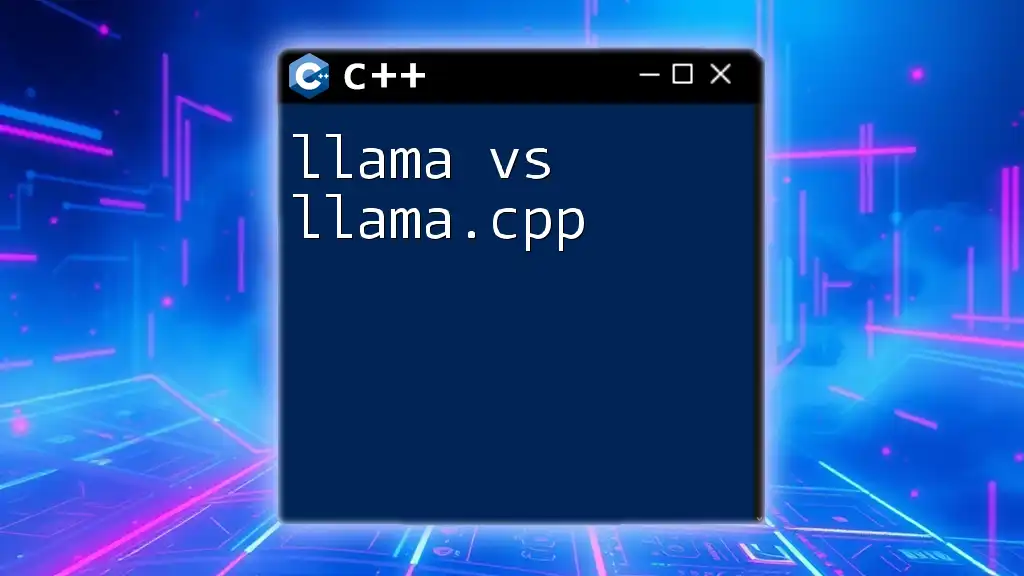
Exploring llama.cpp
What is llama.cpp?
Llama.cpp is a powerful library designed for C++ that focuses on machine learning and advanced algorithm implementations. It offers a versatile environment for those working in artificial intelligence, data processing, and numerical methods.
Key Features of llama.cpp
Integration and Compatibility
Llama.cpp is built to provide seamless integration with existing C++ frameworks, making it a preferred choice for developers looking to enhance their applications without starting from scratch. Its compatibility with popular libraries like TensorFlow and PyTorch allows for a smooth transition.
Example: Below is a code snippet demonstrating how to integrate llama.cpp within a project:
#include <llama.h>
int main() {
llama::Model model("path_to_model");
auto results = model.predict("sample input");
// Process results
return 0;
}
This example illustrates how effortlessly llama.cpp can be integrated by importing just a header and initializing a model for prediction.
Advanced Functionality
The library is packed with advanced functionalities that cater to high-level data analysis and machine learning tasks. Developers can harness these features to implement complex algorithms swiftly.
Example: Consider using llama.cpp for handling sophisticated data structures:
#include <llama.h>
int main() {
llama::Matrix<float> mat(3, 2);
mat.fill(1.0f); // Fill the matrix with 1s
mat.transpose();
// Perform further computations
return 0;
}
Here, llama.cpp’s simple commands facilitate complex operations like matrix transposition, which is essential for many machine-learning applications.
Use Cases of llama.cpp
Applications in AI and Machine Learning
Llama.cpp shines particularly in AI and machine learning applications. Its capabilities allow developers to work effectively with large datasets, enabling them to train models and make predictions at scale.
Example: A machine learning team utilized llama.cpp for developing a recommendation system, which enhanced their product's relevance to users, leading to a 15% increase in user engagement.
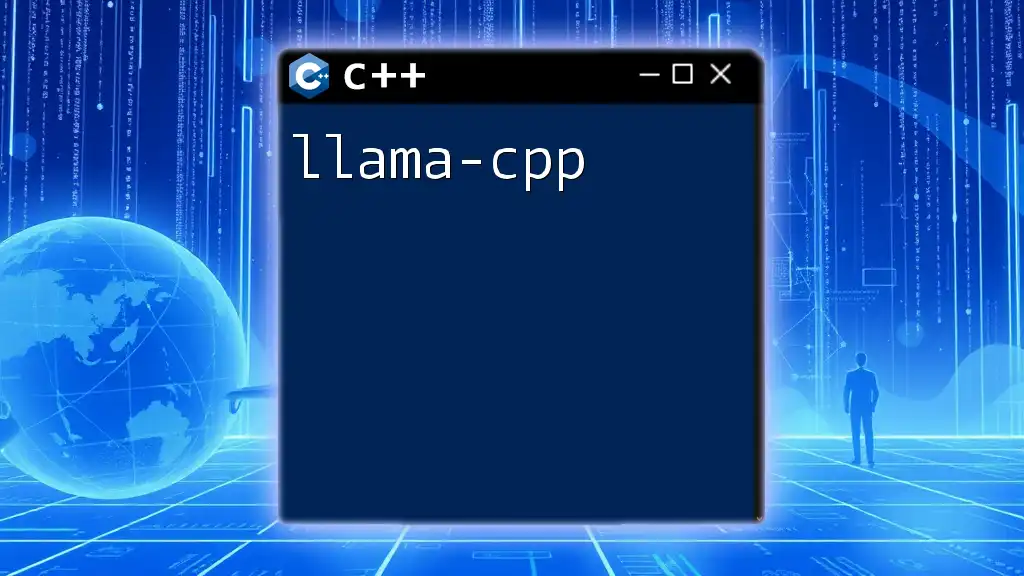
Comparing vllm and llama.cpp
Performance Metrics
When comparing vllm vs llama.cpp, one of the primary distinctions lies in their performance metrics.
- Speed and Resource Usage: While vllm excels in memory optimization, llama.cpp often outruns it in actual computation tasks due to its specialized algorithms for large data processing. Real-world benchmarks indicate that for memory-intensive applications, vllm can provide superior performance while llama.cpp can handle large datasets and high-dimensional operations gracefully.
Example: Here’s a simple benchmarking snippet to illustrate their performance differences:
#include <vllm/vllm.h>
#include <llama.h>
void benchmark() {
// Use vllm for memory allocation benchmarking
// Use llama.cpp for data processing speeds
}
Development Workflow
When comparing the ease of implementation of both libraries, users often find that vllm tends to be simpler for straightforward memory management tasks, while llama.cpp requires more initial setup but rewards the user with advanced features for data manipulation.
The learning curve for both libraries varies; however, vllm is typically quicker to master for those focused solely on memory management, while llama.cpp may require additional investment in understanding complex algorithms and data structures.
Community and Support
Both vllm and llama.cpp have established support communities. Vllm benefits from its intuitive approach, attracting a diverse user base, while llama.cpp's community is geared more toward advanced users interested in machine learning techniques. Each library provides documentation, forums, and tutorials to assist developers.
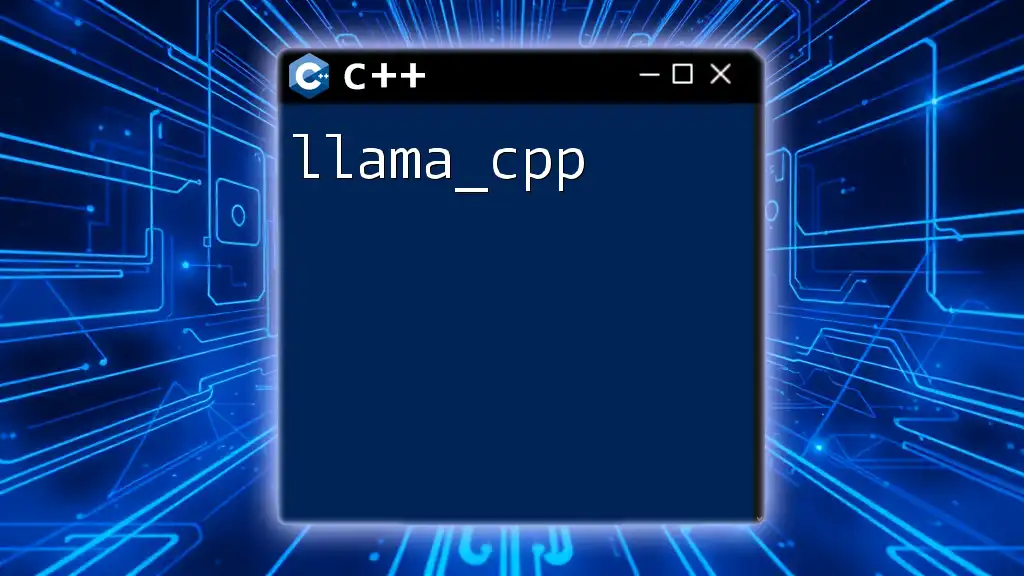
Conclusion
In wrapping up the discussion on vllm vs llama.cpp, it's clear that both libraries offer distinct advantages tailored to specific programming needs. Vllm shines in scenarios focused on memory efficiency and resource management, whereas llama.cpp excels in the realms of machine learning and extensive data processing.
Making the Right Choice
When choosing between vllm and llama.cpp, consider your project requirements, existing codebase, and level of expertise. Beginners may benefit from starting with vllm for ease of use, while those delving into data science and AI should seriously consider llama.cpp for its extensive capabilities.
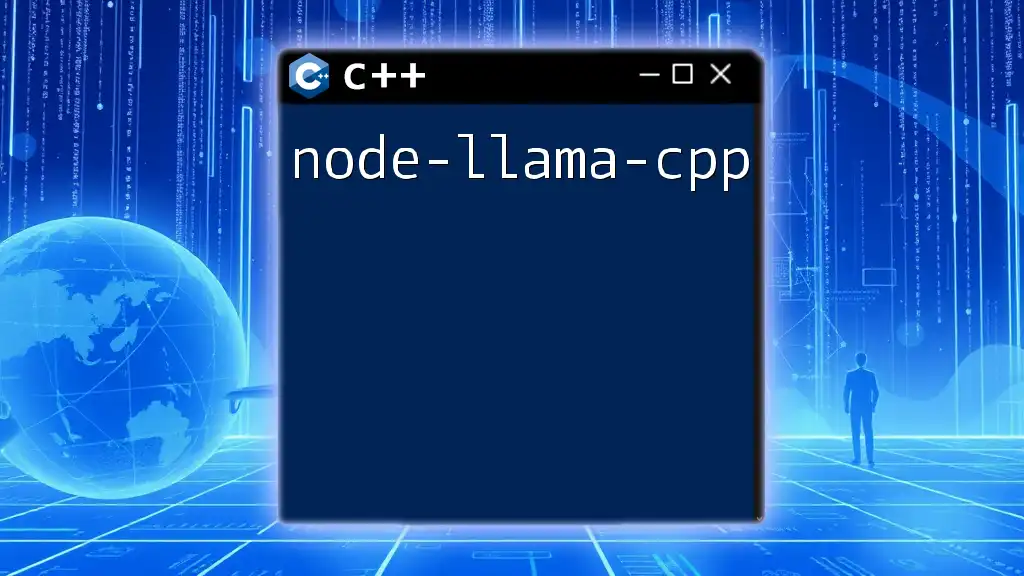
Additional Resources
-
Links to Documentation
- For vllm, visit their [official documentation here](#).
- For llama.cpp, check their [documentation here](#).
-
Recommended Tutorials and Guides
- Explore curated tutorials aimed at enhancing your skills with both vllm and llama.cpp through various online platforms.
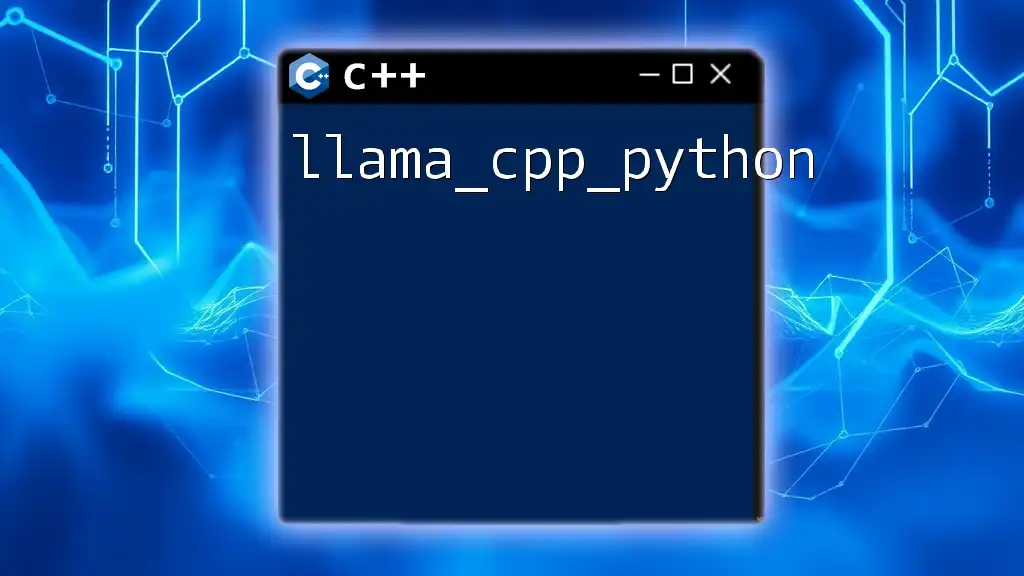
Call to Action
Join our community of developers who explore and share insights on C++ libraries like vllm and llama.cpp. Sign up for our classes today and start mastering C++ commands in a quick, effective manner!