"llama.cpp docker" refers to using Docker to easily manage and deploy applications that utilize the LLaMA model developed in C++, enabling developers to efficiently run and scale their workloads in isolated environments.
# Dockerfile for llama.cpp
FROM ubuntu:20.04
RUN apt-get update && apt-get install -y \
build-essential \
git \
cmake \
&& rm -rf /var/lib/apt/lists/*
WORKDIR /app
RUN git clone https://github.com/your-repo/llama.cpp.git
WORKDIR /app/llama.cpp
RUN mkdir build && cd build && cmake .. && make
CMD ["./build/llama"]
What is Docker?
Docker is an open-source platform designed to automate the deployment, scaling, and management of applications in lightweight, portable containers. These containers encapsulate an application and all of its dependencies, ensuring that it runs uniformly across various computing environments.
One of the major advantages of using Docker is portability. With Docker, you can run the same containerized application on any machine that has Docker installed, eliminating the classic "works on my machine" problem. Docker also provides environment consistency, allowing developers to replicate the production environment on their local machines. Furthermore, it offers isolation, which means that multiple applications can run on the same host without interfering with each other.
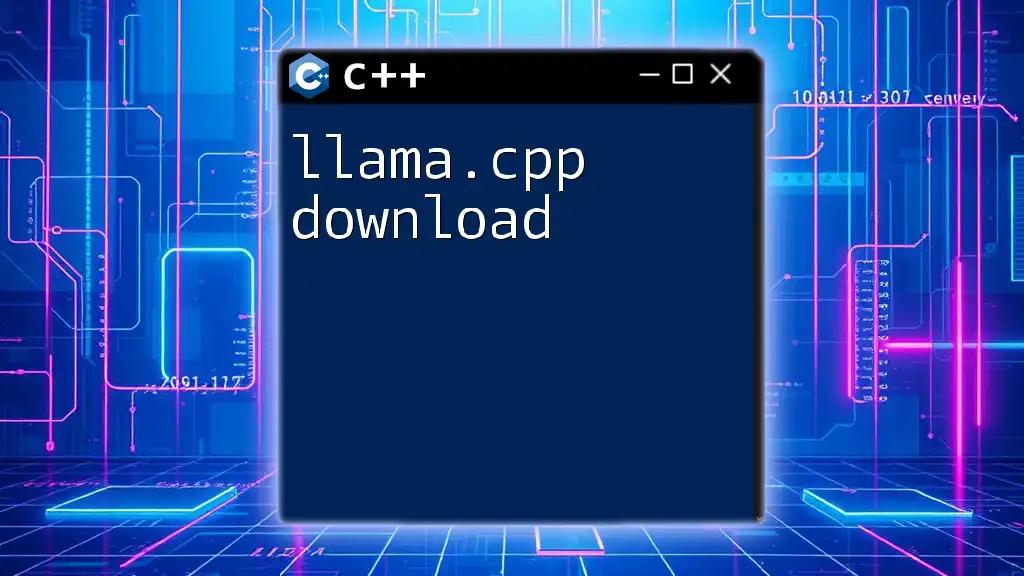
Setting Up Docker for llama.cpp
Prerequisites
Before diving into using Docker for llama.cpp, ensure you have set up Docker on your machine. Follow these steps to install Docker based on your operating system:
- Windows: Download Docker Desktop and follow the installation prompts.
- macOS: Download Docker Desktop from the official website and complete the installation.
- Linux: Use your package manager to install Docker. For example, on Ubuntu, you can use:
sudo apt-get install docker-ce
Familiarizing yourself with basic Docker commands is essential. Here are a few commands you should know:
- `docker run` — Used to run a container from a specified image.
- `docker build` — Builds a Docker image from a Dockerfile.
- `docker-compose` — A tool for defining and running multi-container Docker applications.
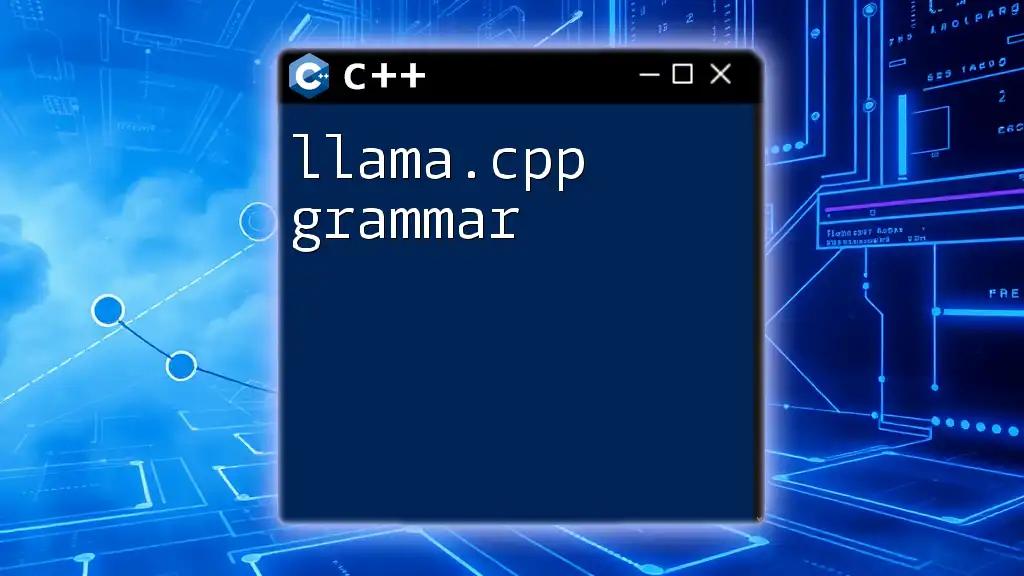
Understanding llama.cpp Structure
Overview of llama.cpp Files
To use llama.cpp effectively within a Docker container, it's important to understand its structure. Typically, a llama.cpp repository would include:
- Source files: The core files where the functionality is defined.
- CMakeLists.txt: A build configuration file for CMake, if applicable.
- README.md: Documentation on how to use the project and its functionality.
Understanding this structure will help in determining what needs to be copied into the Docker image and how to manage dependencies effectively.
Dependencies
llama.cpp may require specific libraries or tools to run. In your Docker setup, be prepared to install these dependencies as part of the Docker image creation process. This is critical for ensuring that your container runs smoothly, mirroring the requirements of the development environment.
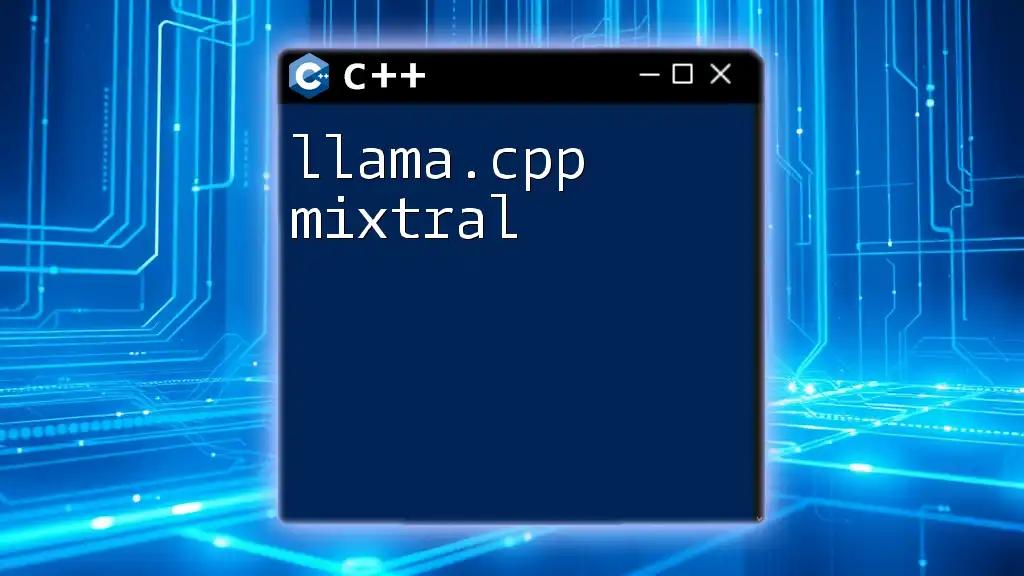
Creating a Dockerfile for llama.cpp
What is a Dockerfile?
A Dockerfile is a script composed of various commands and instructions that Docker uses to build a Docker image. It automates the process of setting up software environments.
Step-by-step Guide to Writing a Dockerfile
Now, let’s create a Dockerfile for llama.cpp. Here’s how to go about it:
-
Base Image Selection: Begin by selecting a base image that contains the necessary environment for your application. A popular choice could be `ubuntu:latest`.
-
Setting Up Environment Variables: You can define environment variables that can be accessed during the build and runtime.
-
Installing Dependencies: Utilizes the `RUN` instruction in the Dockerfile to install necessary libraries and tools.
-
Copying Files: You'll need to copy the llama.cpp files into the Docker container.
-
Building the Project: If using CMake, include a command to build the project.
Here is an example of how your Dockerfile may look:
FROM ubuntu:latest
# Set working directory
WORKDIR /app
# Install dependencies
RUN apt-get update && apt-get install -y \
build-essential \
any-other-required-packages
# Copy llama.cpp files
COPY . .
# Build the project
RUN make
# Command to run the application
CMD ["./llama"]
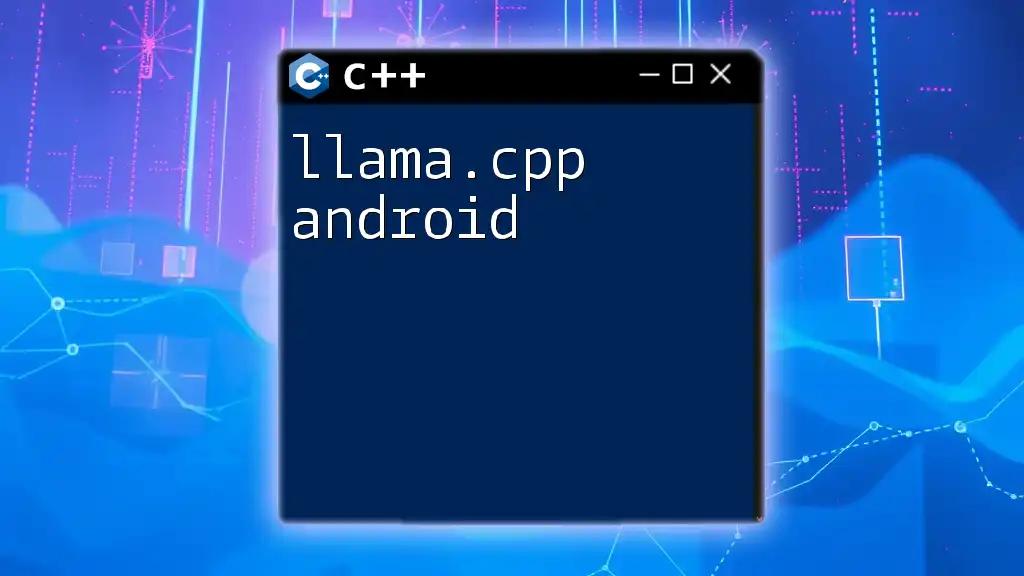
Building and Running the Docker Container
Build the Docker Image
After writing your Dockerfile, you need to build your Docker image. You can execute the following command within the directory containing your Dockerfile:
docker build -t llama-image .
This command uses the Dockerfile to create a new image tagged as `llama-image`. During this process, Docker retrieves the base image, installs dependencies, and copies your code into the new image.
Run the Docker Container
Once your image is built, you can run it using:
docker run -it llama-image
This command starts a new container from the specified image, granting you an interactive terminal to work within.

Using Docker Compose with llama.cpp
What is Docker Compose?
Docker Compose is a tool that simplifies the management of multi-container applications. It allows you to define services and their relationships in a single YAML configuration file.
Creating a docker-compose.yml File
Here's how to structure a `docker-compose.yml` file for llama.cpp:
version: '3'
services:
llama:
image: llama-image
build: .
ports:
- "8080:80"
This YAML file defines a service named `llama`, which Docker will build from the provided Dockerfile. The `ports` map the container's internal port 80 to the host's port 8080, making the application accessible.
Running Docker Compose
To start all defined services in your `docker-compose.yml`, run:
docker-compose up
This command builds the images and starts the containers, ensuring that the necessary dependencies and configurations are set up.
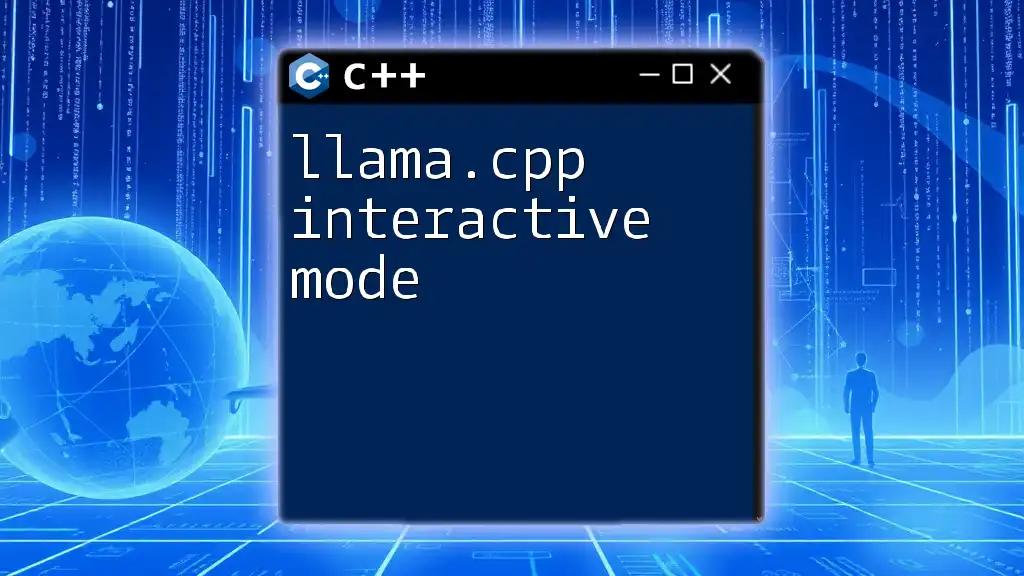
Best Practices for Using Docker with llama.cpp
Efficient Layering
When writing your Dockerfile, it’s essential to optimize layers. Group commands that modify the same files into a single `RUN` instruction. This reduces the number of layers and speeds up build times.
Managing Environment Variables
Utilize a `.env` file to manage environment variables effectively. This keeps sensitive information out of your Dockerfile and helps in making your containers more portable.
Cleaning Up
Regularly clean up unused Docker images and containers to save disk space. The following commands can help:
docker system prune -a
Use this command cautiously, as it will remove all stopped containers and dangling images.
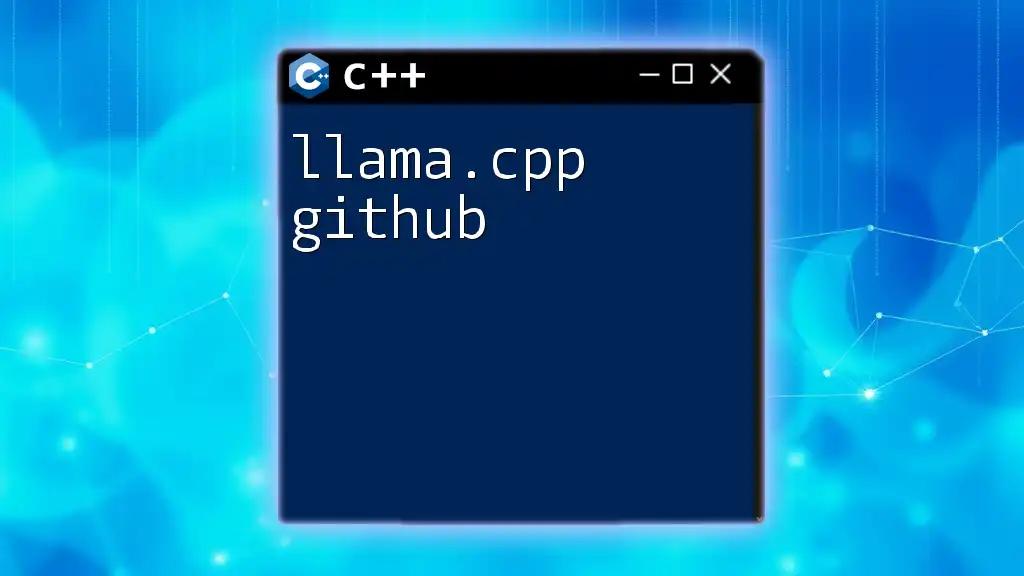
Troubleshooting Common Issues
While using Docker with llama.cpp, you may encounter common issues like dependency resolution failures or image build errors.
Common Errors and Solutions
- Build Failures: Ensure all dependencies are correctly specified in your Dockerfile. Consult the error messages for hints on missing packages.
- Container Crashes: Check runtime logs using:
docker logs <container_id>
- Environment Issues: Validate that all necessary environment variables are set up properly.
Logs and Debugging
Debugging containers can be challenging. Docker provides log outputs to help identify issues. Use the command mentioned above to view logs directly from the command line.
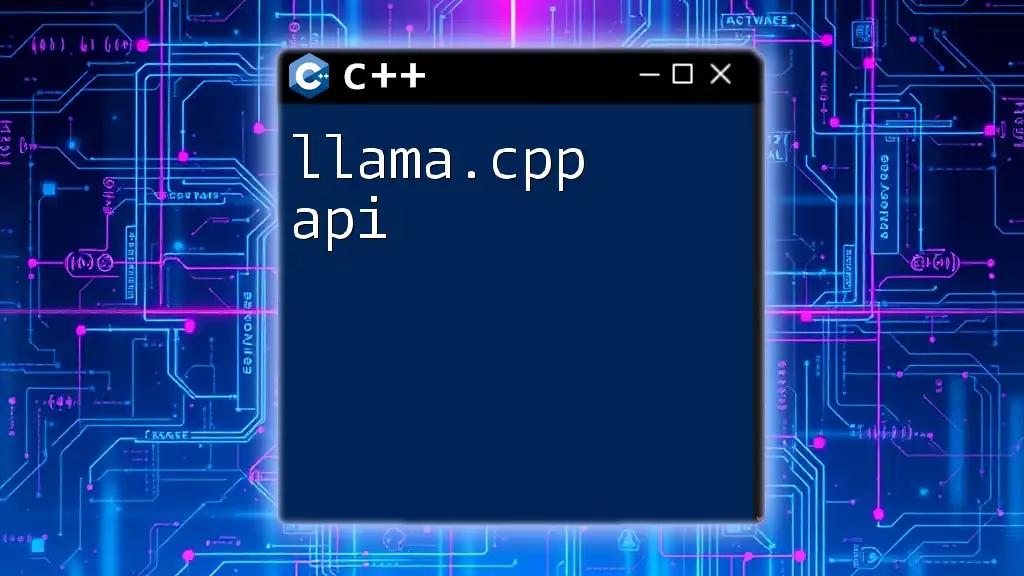
Conclusion
Using Docker with llama.cpp creates a streamlined, portable, and efficient environment for your application. It mitigates configuration issues while enabling faster deployments. By following this guide, you can leverage Docker effectively, ensuring that you get the most out of your llama.cpp implementation.
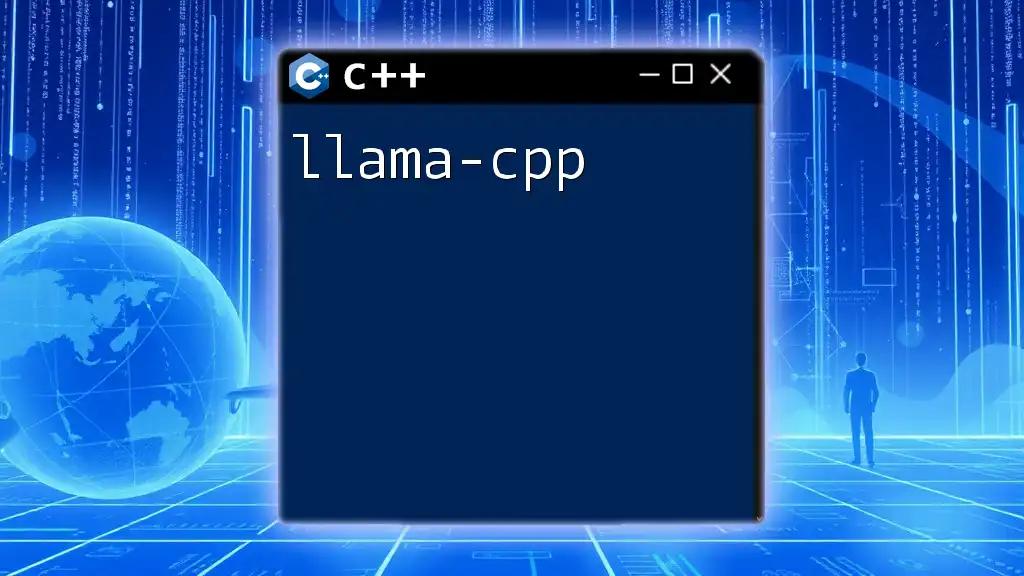
Additional Resources
To further enhance your Docker knowledge, explore additional resources like:
- Docker official documentation for updates and advanced concepts.
- Community forums where you can share experiences and learn from others.
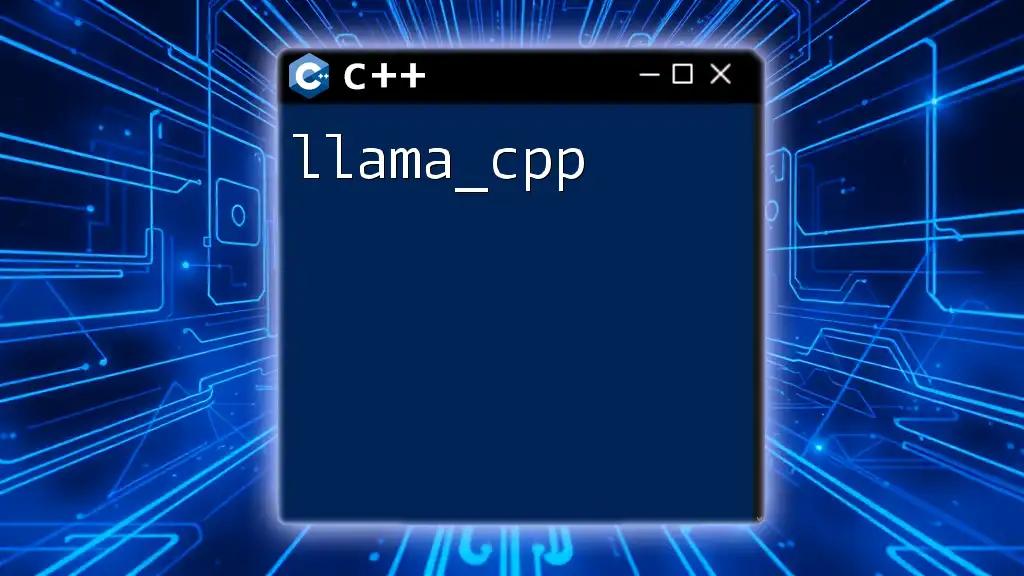
Call to Action
We invite you to share your experiences using Docker with llama.cpp! Join our learning platform for more concise tutorials and guides on C++ commands and Docker usage. Your journey towards mastering these technologies starts now!