The comparison between `llama.cpp` and `ollama` highlights their distinct approaches to leveraging C++ commands, with `llama.cpp` focusing on simplicity and speed, while `ollama` aims for extensibility and advanced features.
Here's an example of using a C++ command with `llama.cpp`:
#include <iostream>
int main() {
std::cout << "Hello, Llama!" << std::endl; // Simple output in llama.cpp style
return 0;
}
And here's a basic command snippet using `ollama`:
#include <ollama/ollama.h>
int main() {
ollama::print("Hello, Ollama!"); // Output with ollama library functionality
return 0;
}
What is Llama.cpp?
Background
Llama.cpp is an innovative C++ framework designed primarily for building robust and efficient applications. Introduced as a solution for developers seeking flexibility and control, Llama.cpp brings a myriad of features that cater to advanced programming needs. Its architecture provides fine-tuned performance, making it suitable for both small and large-scale projects.
Use Cases
Llama.cpp excels in several scenarios:
- High-Performance Applications: When speed and resource efficiency are paramount, Llama.cpp’s low-level access to hardware can lead to optimized performance.
- Game Development: With the ability to manage resources directly, Llama.cpp is a favored choice for programmers in the gaming industry who require real-time responsiveness.
- Embedded Systems: C++'s proximity to hardware makes Llama.cpp an excellent choice for developing software for embedded devices.
Getting Started with Llama.cpp
To get started with Llama.cpp, follow these installation steps:
// Sample installation command
git clone https://github.com/example/llama.cpp.git
cd llama.cpp
make
Once installed, you can create a basic program with Llama.cpp. Here’s an example:
#include <iostream>
int main() {
std::cout << "Hello from Llama.cpp!" << std::endl;
return 0;
}
This snippet demonstrates a simple C++ program utilizing the Llama.cpp framework. The `#include <iostream>` directive allows input and output operations, while the `main()` function is the entry point of the program.
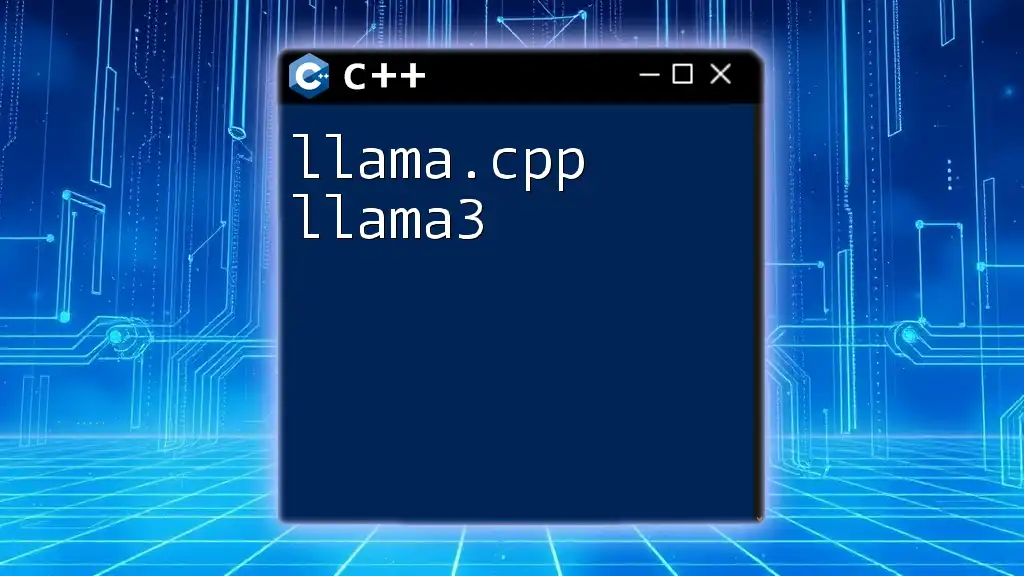
What is Ollama?
Background
Ollama is a modern JavaScript-based framework designed with the goal of simplifying web development. Particularly popular among developers who favor rapid prototyping, Ollama provides a plethora of features aimed at boosting productivity and efficiency.
Use Cases
Ollama shines in various environments:
- Web Applications: For developers who need to create dynamic and responsive web applications, Ollama enables easy integration with front-end frameworks.
- API Development: Ollama’s user-friendly syntax facilitates efficient API development, making it easier to build and maintain web services.
- Full Stack Development: Combining server-side and client-side capabilities, Ollama is ideal for full-stack developers looking for a seamless workflow.
Getting Started with Ollama
Begin using Ollama by installing it via npm:
# Sample installation command
npm install -g ollama
After installation, you can create a simple script with Ollama:
console.log("Hello from Ollama!");
This basic example illustrates how easy it is to write scripts in Ollama. The `console.log()` function outputs a message to the console, demonstrating Ollama’s straightforward syntax.
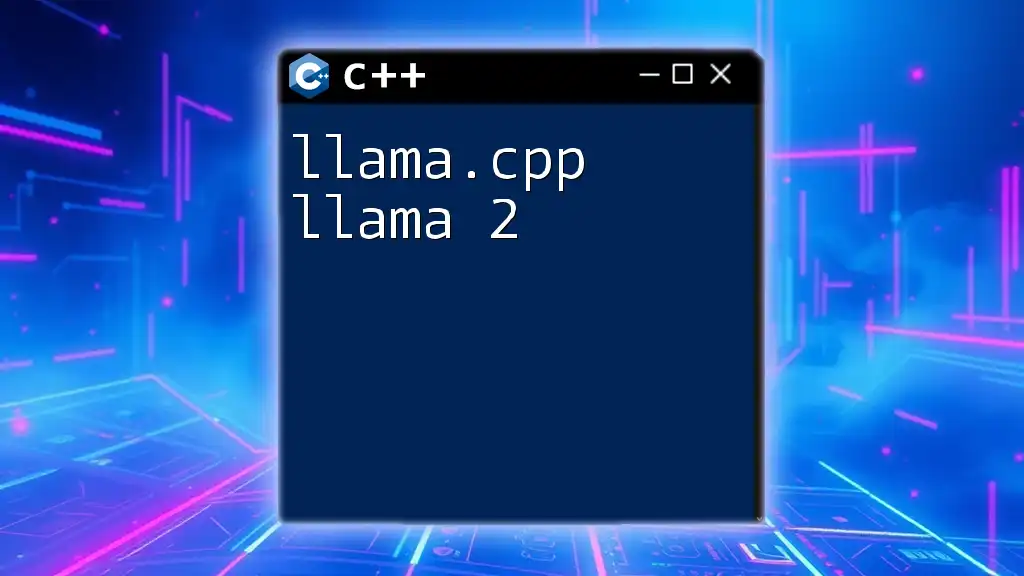
Comparative Analysis: Llama.cpp vs Ollama
Performance
When discussing performance, Llama.cpp generally outshines Ollama in raw execution speed. Since Llama.cpp is built with C++, it offers low-level programming capabilities which can lead to better optimization. Benchmarks typically show that applications utilizing Llama.cpp can handle more intensive computational tasks more swiftly compared to those developed with Ollama.
For example, consider a scenario where you have an algorithm performing matrix multiplication. Implementing this using Llama.cpp may look like this:
#include <vector>
void matrixMultiply(const std::vector<std::vector<int>>& A, const std::vector<std::vector<int>>& B, std::vector<std::vector<int>>& C) {
// Implementation of matrix multiplication
}
In contrast, while Ollama can handle such tasks efficiently, it might not match the raw speed of Llama.cpp due to the abstraction and overhead involved in JavaScript.
Language Features
C++ Advantages with Llama.cpp: C++ offers features such as memory management and extensive libraries that allow greater control over program execution. With Llama.cpp, developers can leverage these features to create highly optimized software that can run on a variety of hardware architectures.
JavaScript Capabilities with Ollama: Ollama capitalizes on JavaScript's versatility, making it adept for web development. The simplicity of Ollama’s syntax allows developers to focus on building applications quickly without getting bogged down in extensive syntax rules.
Development Environment
Llama.cpp typically benefits from C++ development environments like Visual Studio or Eclipse, providing robust debugging and profiling capabilities. Meanwhile, Ollama shines in environments like Node.js or modern web browsers, enabling a highly interactive development experience.
Community and Support
Both Llama.cpp and Ollama have active communities. For Llama.cpp, resources include:
- GitHub repositories
- Forums dedicated to C++ development
- Documentation on C++ libraries
In contrast, Ollama benefits from the extensive resources available in the JavaScript ecosystem. It boasts:
- Comprehensive online forums
- Social media groups specifically for JavaScript tools
- A plethora of tutorials and guides on web development
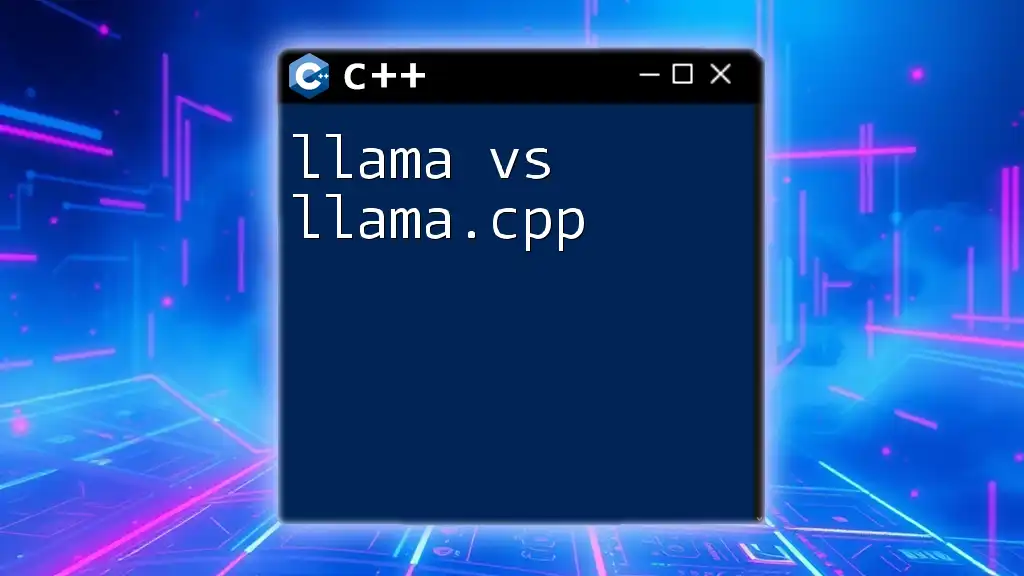
Ollama vs Llama.cpp: Choosing the Right Tool
Project Requirements
When deciding between Llama.cpp and Ollama, consider the specific requirements of your project:
-
Choose Llama.cpp if your project requires high performance, low-level hardware access, or if you are developing performance-critical applications such as games or embedded systems.
-
Choose Ollama if you are looking for rapid development cycles, particularly in web applications or full-stack development, where ease of use and integration are crucial.
Future Trends
The evolution of programming tools is ever-changing. As C++ continues to mature, Llama.cpp will likely see enhancements focused on performance and libraries, further solidifying its position in high-performance computing.
Conversely, with the rise of web applications, Ollama may adapt to incorporate emerging technologies, such as Progressive Web Applications (PWAs), ensuring it remains a relevant tool in modern web development.
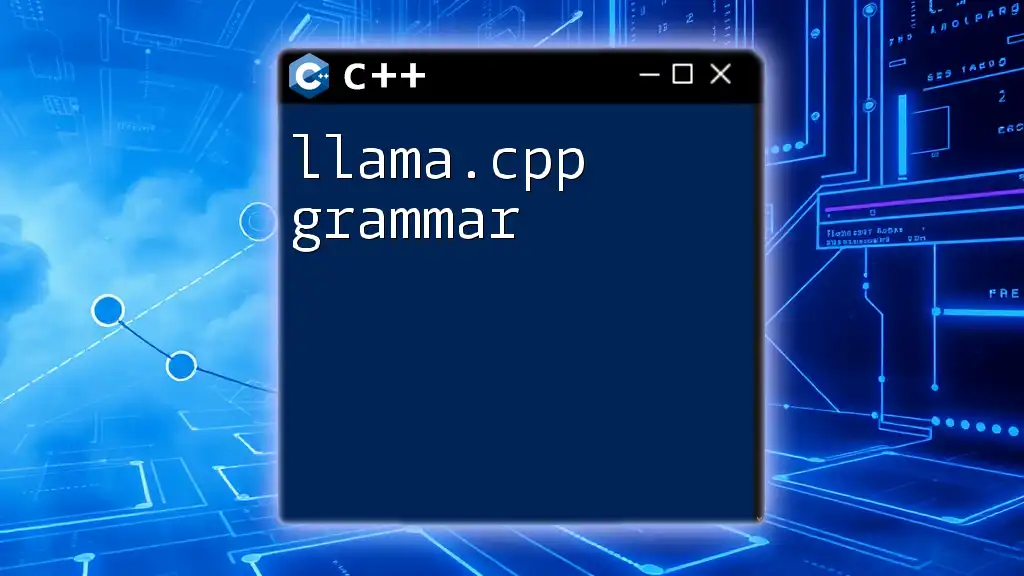
Conclusion
In the debate of llama.cpp vs ollama, both frameworks offer unique advantages that cater to different development needs. Llama.cpp stands out with its performance capabilities and control, while Ollama excels in creating accessible, user-friendly web applications. By evaluating the specific requirements of your projects, you can make an informed decision between these powerful tools.
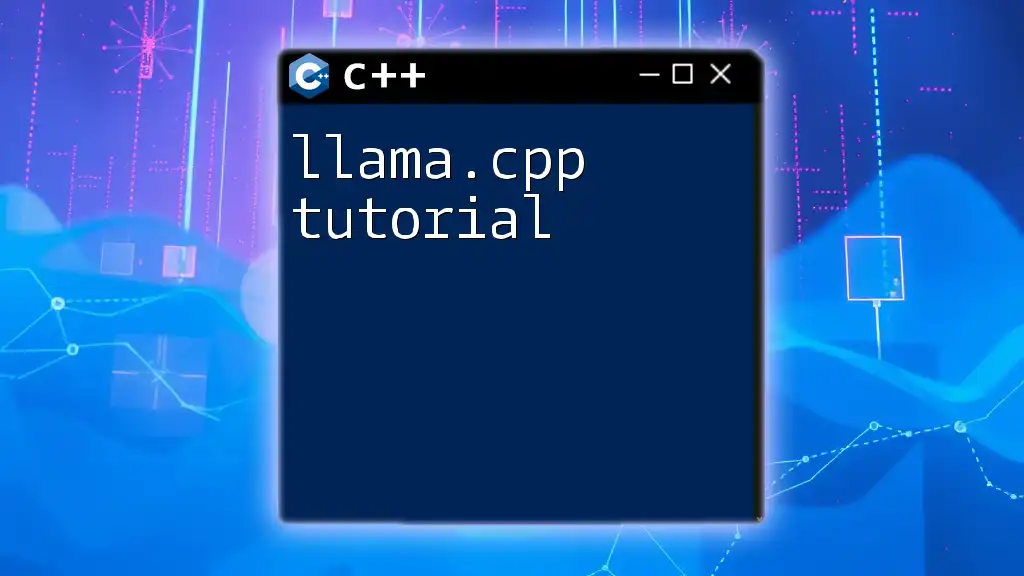
Additional Resources
For further exploration, refer to the official documentation of Llama.cpp and Ollama. Consider engaging with online tutorials and community forums to enhance your understanding and application of these tools in your development journey.