Llama.cpp embedding allows you to integrate and utilize pre-trained models in your C++ applications for tasks such as natural language processing.
Here's an example of how to embed a Llama.cpp model:
#include <llama/llama.h>
int main() {
llama::Model model("path/to/model");
std::string input = "Hello, how can I help you today?";
std::string output = model.generate(input);
std::cout << output << std::endl;
return 0;
}
What is Llama.cpp?
Overview of Llama Framework
Llama is an innovative framework designed to streamline operations in machine learning, specifically for natural language processing (NLP) tasks. It provides powerful tools for generating and handling embeddings, enabling faster training and inferencing models. The unique feature of Llama lies in its ability to manage complex data structures efficiently and deliver high-quality embeddings.
Llama in Context of C++
C++ serves as a robust environment for implementing machine learning solutions due to its speed and performance capabilities. Llama.cpp builds upon this foundation, allowing developers to leverage the benefits of C++ while harnessing the power of machine learning algorithms. By integrating Llama with C++, you gain access to optimizations that can greatly enhance your NLP projects, making computations faster and more efficient.
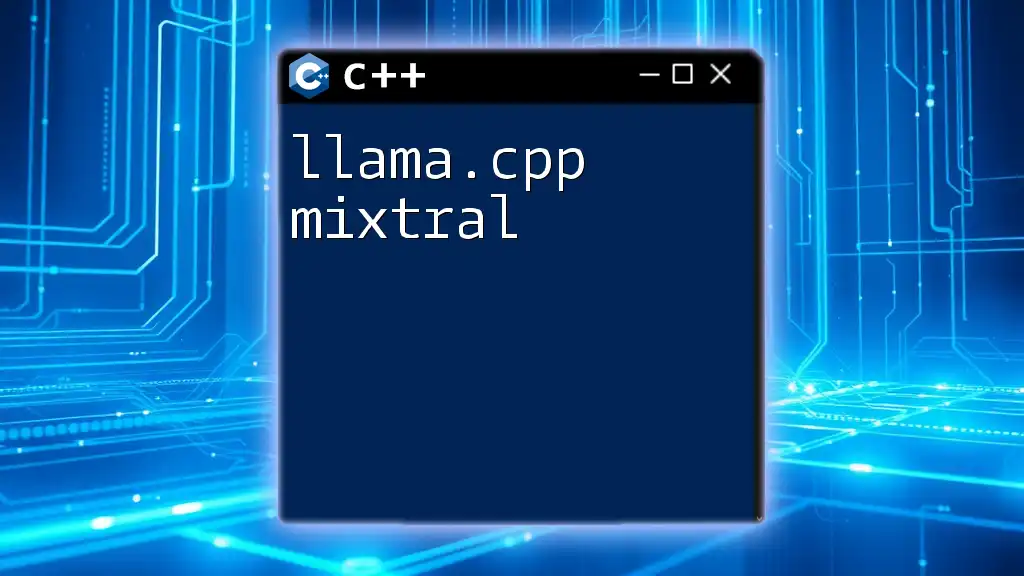
Understanding Embeddings
What are Embeddings?
In machine learning, embeddings refer to the numerical representations of objects or words in a vector space. Unlike traditional representation methods, embeddings capture semantic relationships in a way that maintains proximity between similar items. For example, words with similar meanings will be positioned closely in the embedding space, making them more interpretable for the model.
Importance of Embeddings
Embeddings are crucial in various domains of NLP, including text classification, sentiment analysis, and recommendation systems. They allow the model to understand the context and nuances of language, leading to better performance in tasks like text generation, semantic similarity, and information retrieval. The ability to represent words, phrases, or even complete sentences in a compressed format while retaining their meanings is what makes embeddings so powerful.
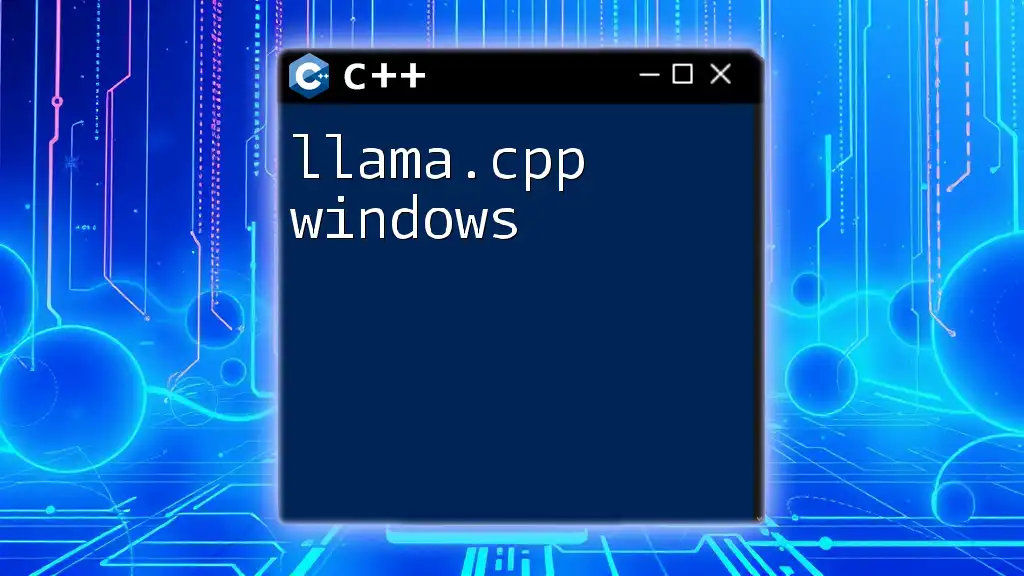
Getting Started with Llama.cpp Embedding
Prerequisites for Using Llama.cpp
Before diving into Llama.cpp embedding, ensure you have the required installations and tools. You will need a C++ compiler, libraries for numerical operations (like Eigen), and the Llama framework itself. Setting up your C++ environment correctly is critical for smooth development.
Basic Usage of Llama.cpp
Once your environment is ready, initializing Llama for embedding tasks is straightforward. Here is a minimal code snippet to help you get started:
#include <llama.h>
int main() {
LlamaEmbedder embedder;
embedder.init("path_to_model");
return 0;
}
This snippet initializes an instance of the `LlamaEmbedder` class and loads a model from a specified path.
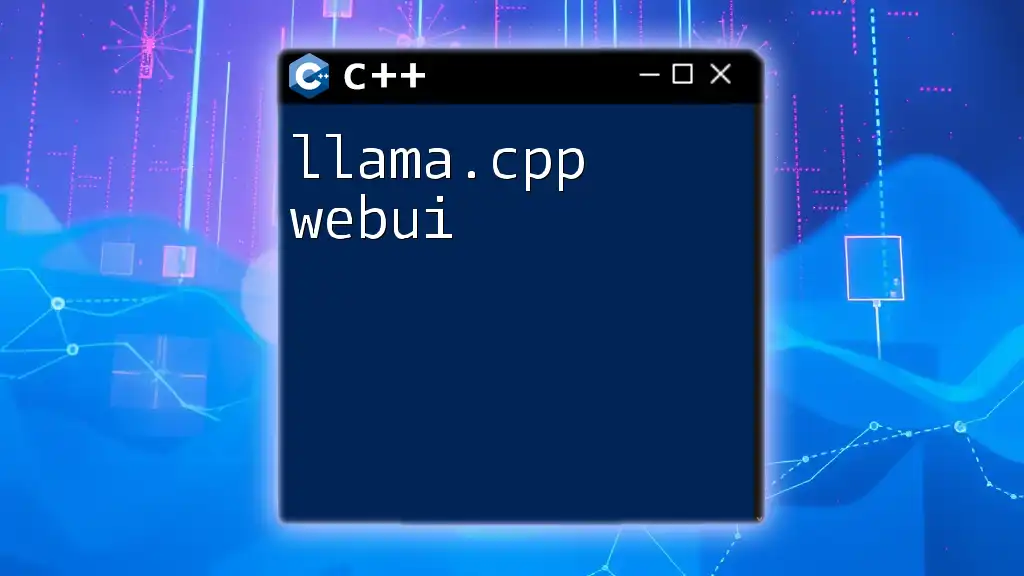
Creating and Using Embeddings with Llama.cpp
Loading Pre-trained Models
To utilize predefined knowledge, loading pre-trained models is essential. This step allows Llama to leverage existing embeddings trained on extensive datasets. Use the following code snippet to load your model:
embedder.loadModel("model_path");
Generating Embeddings
Once the model is loaded, generating embeddings from a text string is simple. Here’s an example code to generate embeddings for a sample phrase:
std::vector<float> embedding = embedder.embed("Hello, Llama!");
The output will be a vector of floats, representing the embedding for the input text—a compact representation that can be used for various downstream tasks.
Understanding the Output
The resulting vector reflects the positioning of the input text in the embedding space. The dimensionality of this vector will depend on your model’s configuration. The elements in the vector are numerical representations capturing the semantics of the text. For example, embeddings for words with similar meanings might yield vectors whose values are close in terms of Euclidean distance.
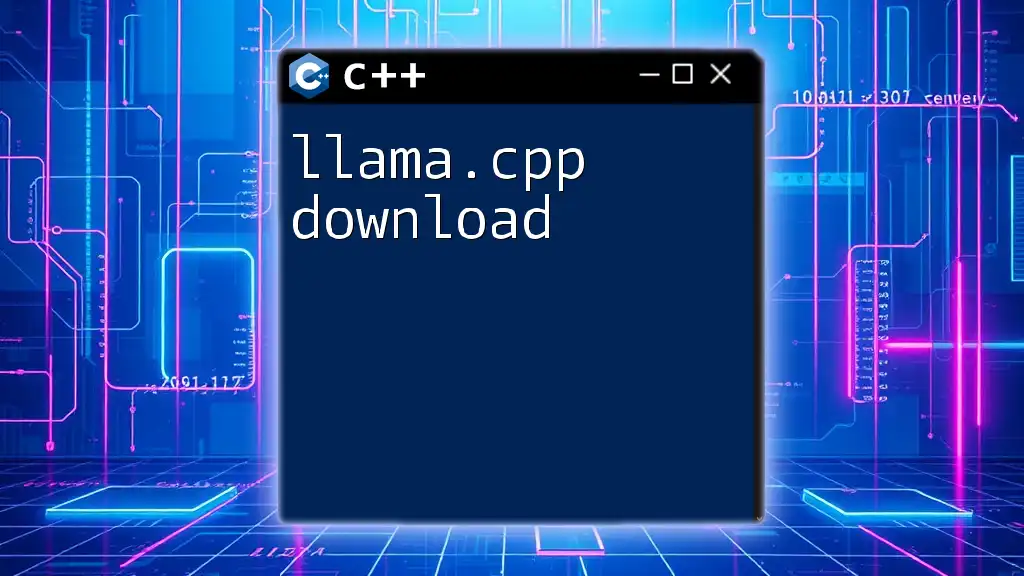
Advanced Techniques with Llama.cpp
Fine-Tuning Llama Embeddings
Fine-tuning is an essential step that allows you to adapt the embeddings to better fit your specific needs. This process entails training your Llama model on a smaller, specialized dataset. Here’s a code snippet demonstrating how to fine-tune existing embeddings:
embedder.fineTune("fine_tuning_data");
By doing so, your embeddings will gain a greater relevance to the specific context of your applications, enhancing their performance in tasks like classification or sentiment analysis.
Combining Embeddings
Combining embeddings can yield stronger representations by incorporating multiple perspectives. Whether you're aggregating embeddings from different models or merging them from different phases, this technique enhances the richness of the data. Here’s an example of how to combine embeddings in Llama.cpp:
auto combined_embedding = embedder.combine(embed1, embed2);
Utilizing combined embeddings can typically lead to more accurate predictions as the model benefits from diversified information.
Performance Optimization
To achieve optimal performance while using Llama.cpp, a few best practices should be followed. Enabling parallel processing can greatly enhance computational speed, especially when dealing with large datasets. Incorporate this feature as follows:
embedder.enableParallelProcessing();
Additionally, be mindful of memory allocation and consider strategies for managing large vectors to prevent bottlenecks during runtime.
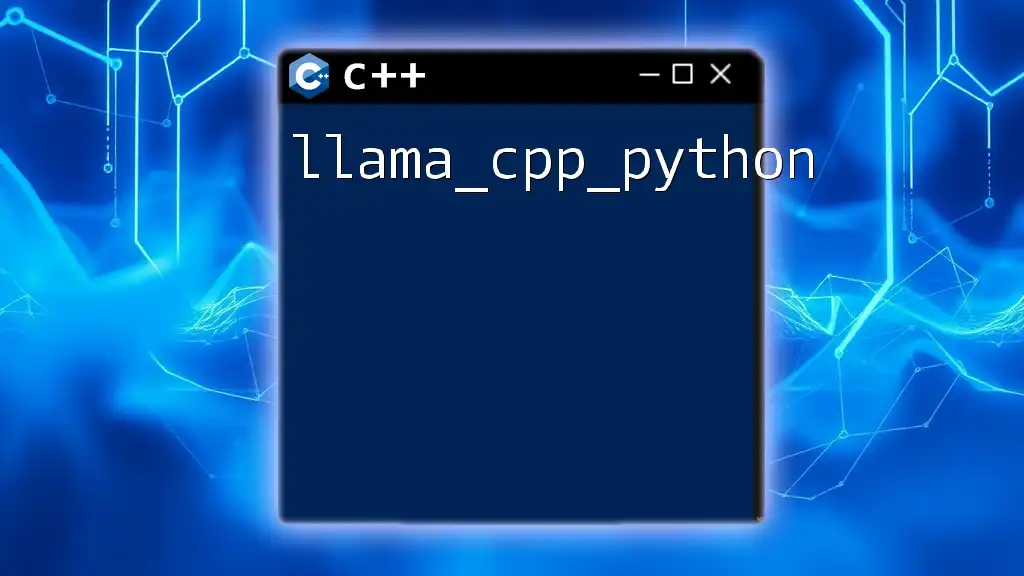
Troubleshooting Common Issues
Common Errors with Llama.cpp
Working with Llama.cpp can lead to various issues, such as model loading errors or mismatched input sizes. Familiarizing yourself with these common pitfalls will save you time. Check for correct file paths, ensure that your input data matches the expected format, and consult the Llama documentation for troubleshooting tips.
Performance Hiccups
If your implementation faces performance hiccups, consider experimenting with different model sizes or reducing your embedding dimensionality. Profiling your application can also provide insights into which areas of your codebase could benefit from optimization.
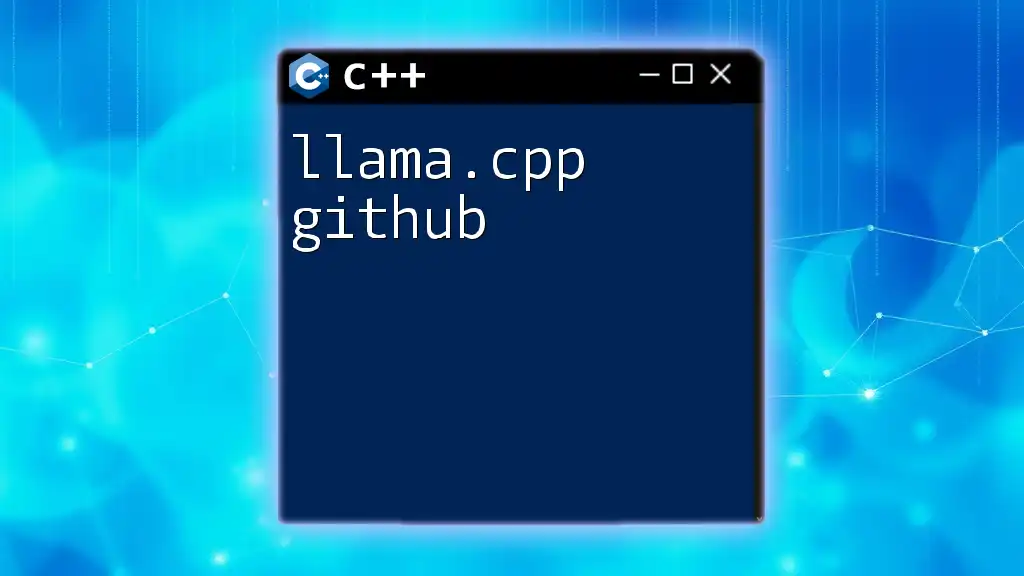
Real-World Applications of Llama.cpp Embeddings
Chatbots and Conversational Agents
Llama embeddings are especially useful in the development of chatbots. With the ability to grasp contextual nuances, embeddings help chatbots generate responses that are more human-like. They can understand user queries effectively, providing relevant and accurate replies.
Sentiment Analysis
Llama embeddings can also empower sentiment analysis applications. By mapping user reviews or social media commentary into the embedding space, the model can classify sentiments with high precision. Here’s an example of integrating Llama embeddings into a sentiment analysis model:
std::string sentiment = classifySentiment(embedding);
This capability opens a wide array of business opportunities in reputation management and customer feedback analysis.
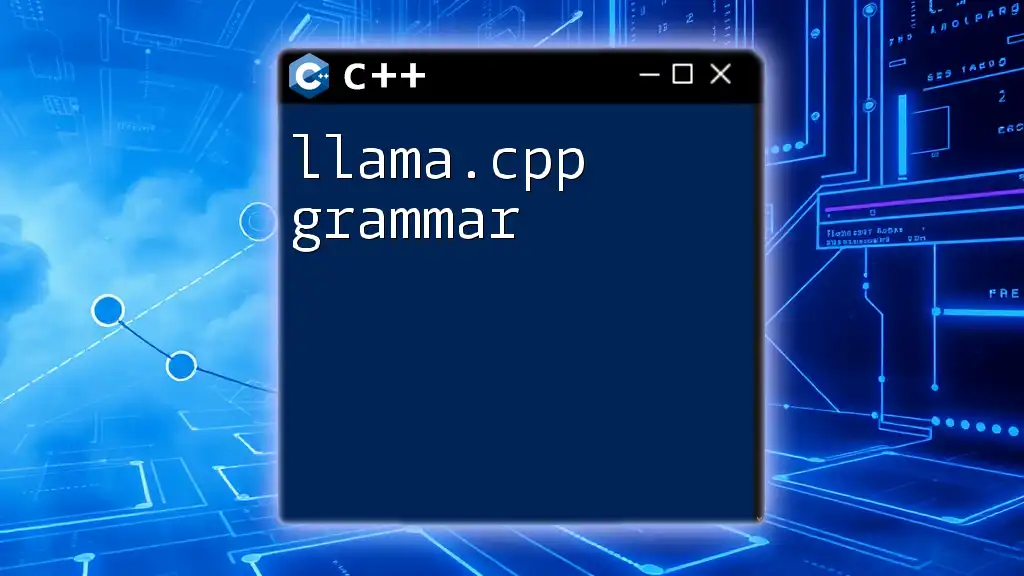
Best Practices for Using Llama.cpp
Staying Organized
When working with Llama.cpp and embedding, organization is key. Structure your projects to facilitate maintainability—clearly comment your code and segment different functionalities into separate classes or files. This practice enhances readability and aids collaborators in understanding your work.
Documentation and Community Support
Capitalize on Llama's documentation and the community around it. Engaging with forums or discussion platforms dedicated to Llama.cpp can streamline your learning process and provide solutions for challenges you may encounter.
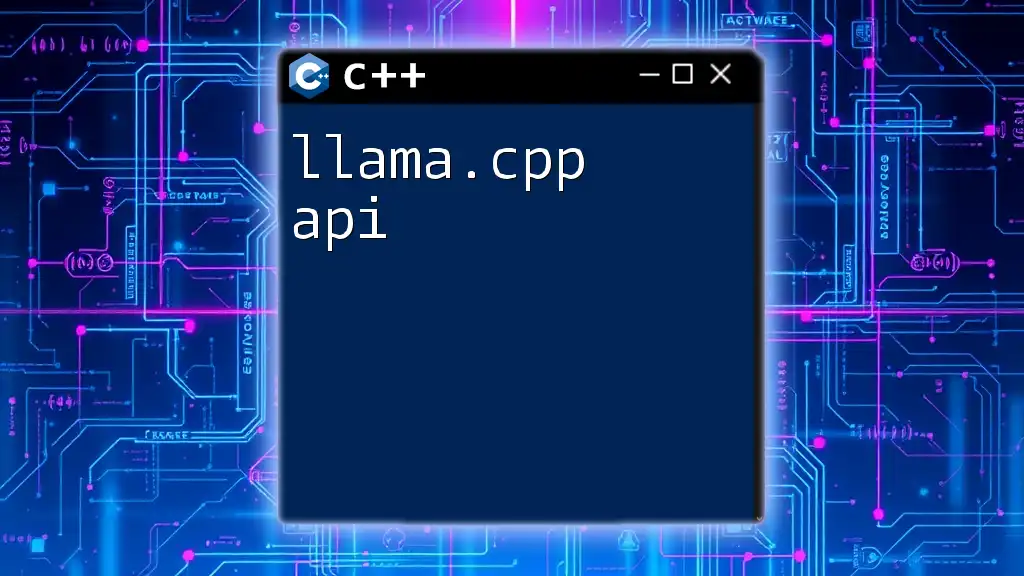
Conclusion
Utilizing llama.cpp embedding forms a cornerstone for modern NLP solutions. By understanding embeddings and their implementation within the Llama framework, you can greatly enhance the performance and capability of your machine learning applications. Embrace the exploration of Llama's features, and don't hesitate to experiment with real-world tasks to unlock the full potential of your projects.
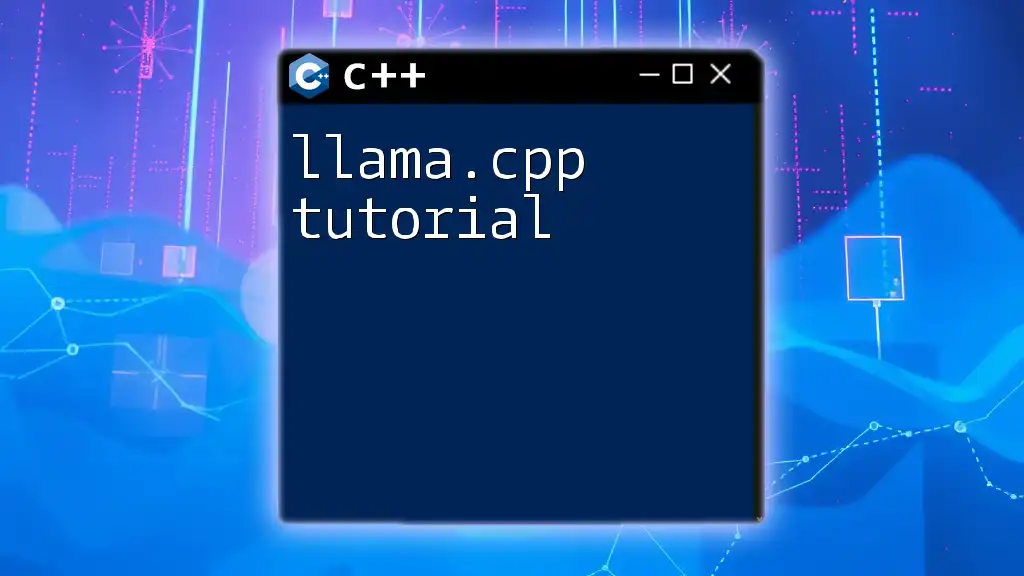
Additional Resources
Documentation Links
Refer to the official Llama framework documentation for detailed guidance on various functionalities and updates.
Community Forums
Engage in forums such as Stack Overflow or specialized machine learning communities to connect with other Llama users. Sharing experiences and solutions can significantly enhance your learning and application effectiveness.