The "llama.cpp cuda" refers to utilizing CUDA-enabled functionality in a C++ program to leverage GPU acceleration for efficient computations.
Here’s a simple example of how to use CUDA in a C++ program:
#include <iostream>
#include <cuda_runtime.h>
__global__ void add(int *a, int *b, int *c) {
int index = threadIdx.x;
c[index] = a[index] + b[index];
}
int main() {
const int size = 5;
int a[size] = {1, 2, 3, 4, 5};
int b[size] = {10, 20, 30, 40, 50};
int c[size];
int *d_a, *d_b, *d_c;
cudaMalloc(&d_a, size * sizeof(int));
cudaMalloc(&d_b, size * sizeof(int));
cudaMalloc(&d_c, size * sizeof(int));
cudaMemcpy(d_a, a, size * sizeof(int), cudaMemcpyHostToDevice);
cudaMemcpy(d_b, b, size * sizeof(int), cudaMemcpyHostToDevice);
add<<<1, size>>>(d_a, d_b, d_c);
cudaMemcpy(c, d_c, size * sizeof(int), cudaMemcpyDeviceToHost);
for(int i = 0; i < size; i++) {
std::cout << c[i] << " ";
}
cudaFree(d_a);
cudaFree(d_b);
cudaFree(d_c);
return 0;
}
Understanding Llama.cpp and CUDA
What is Llama.cpp?
Llama.cpp is a versatile C++ library designed to simplify the development of machine learning models and algorithms. It serves as an abstraction layer that allows developers to focus on implementing algorithms without worrying about the underlying complexities of performance optimizations. This library is particularly valuable for those who want to integrate advanced computational techniques into their applications rapidly.
Key features of Llama.cpp include support for a wide array of machine learning models, highly efficient memory management, and an easy-to-use API. It seamlessly integrates with CUDA, which enables developers to leverage the computational power of GPUs. This relationship provides a way to significantly speed up algorithm execution times, making it an essential tool for high-performance computing tasks.
Introduction to CUDA
CUDA (Compute Unified Device Architecture) is a parallel computing platform and application programming interface (API) created by NVIDIA. It allows developers to utilize the power of NVIDIA GPUs to enhance computation-intensive tasks. CUDA is fundamentally based on C++ and extends its capabilities by enabling developers to write code that runs directly on the GPU, thus leveraging its higher processing power to accelerate applications.
By integrating CUDA with C++, developers can achieve significant performance improvements, especially in tasks such as deep learning, scientific simulations, and complex data processing. Using CUDA can notably reduce the time taken to execute algorithms, leading to faster results and a more efficient development cycle.
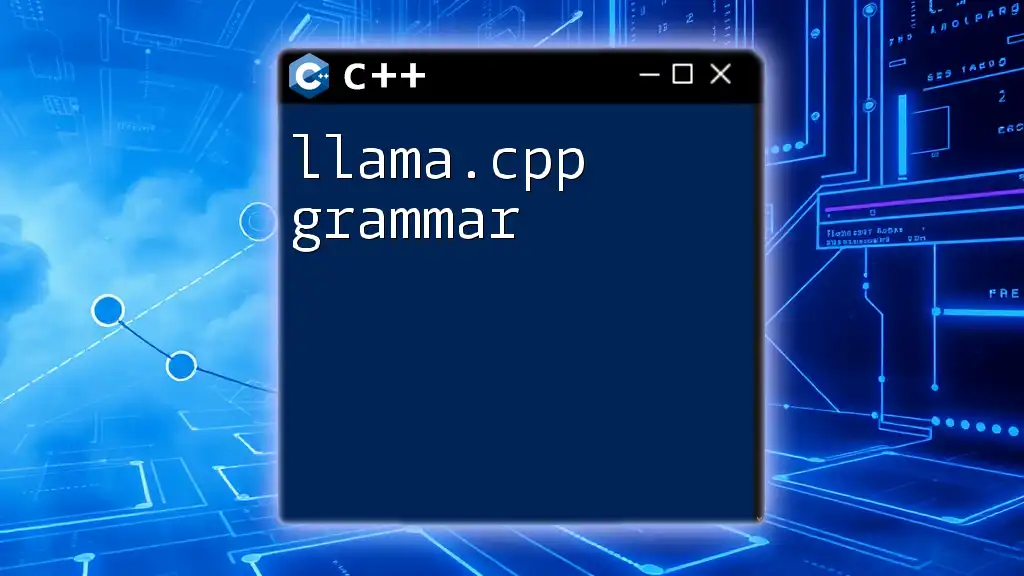
Setting Up the Environment for Llama.cpp and CUDA
System Requirements
To effectively utilize Llama.cpp and CUDA, your system needs specific hardware and software setups. Ensure you have an NVIDIA GPU compatible with the CUDA version you plan to use. Here's what you need to consider:
- Hardware: NVIDIA GPU (CUDA-capable, with sufficient memory for your applications).
- Software: A compatible operating system like Windows, macOS, or Linux, along with NVIDIA drivers and the CUDA toolkit.
Installation Steps
How to Install CUDA Toolkit
- Download the CUDA Toolkit from [NVIDIA's official website](https://developer.nvidia.com/cuda-downloads).
- Follow the installation instructions based on your operating system.
Setting Up Llama.cpp
- Clone the repository using Git:
git clone https://github.com/yourusername/llama.cpp.git
- Navigate into the repository:
cd llama.cpp
- Configure the build settings according to your system specifications as outlined in the README file provided in the repository.
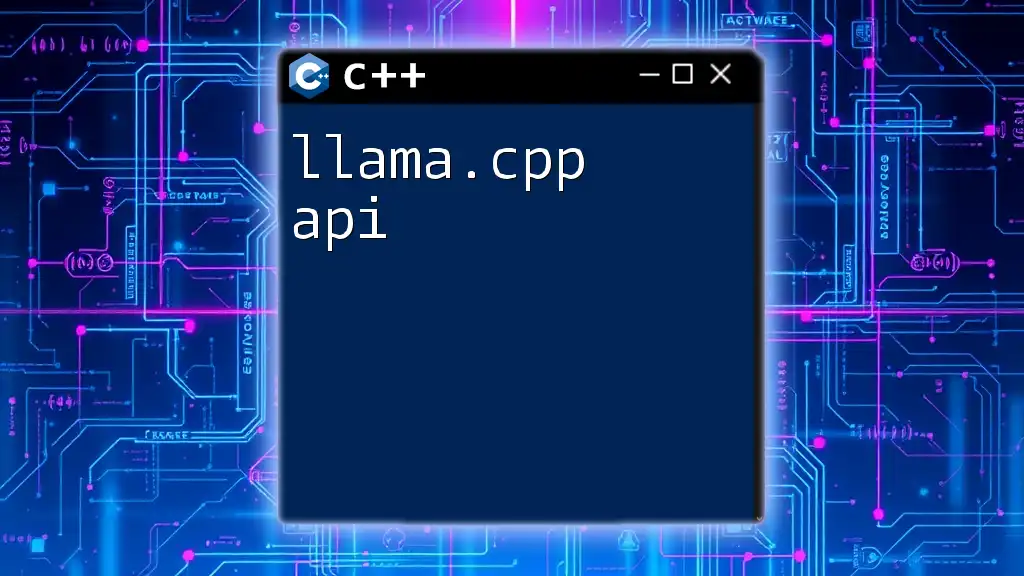
Getting Started with Llama.cpp and CUDA
Basic Usage of Llama.cpp
Getting started with Llama.cpp is straightforward. This library is designed to allow you to begin coding immediately. The key is understanding its main classes and functions. For illustration, here’s a simple "Hello, World!" program written using Llama.cpp:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This example demonstrates how to set up a basic C++ application using Llama.cpp where you can initiate your journey in machine learning and GPU programming.
Compiling with CUDA
To compile your C++ code with CUDA, you need to ensure your code adheres to the CUDA C++ guidelines. Here’s an example of how to compile a simple CUDA program:
Create a CUDA file named `my_cuda_program.cu`:
#include <iostream>
__global__ void add(int *a, int *b, int *c) {
*c = *a + *b;
}
int main() {
int a = 2, b = 7, c;
int *d_a, *d_b, *d_c;
cudaMalloc((void**)&d_a, sizeof(int));
cudaMalloc((void**)&d_b, sizeof(int));
cudaMalloc((void**)&d_c, sizeof(int));
cudaMemcpy(d_a, &a, sizeof(int), cudaMemcpyHostToDevice);
cudaMemcpy(d_b, &b, sizeof(int), cudaMemcpyHostToDevice);
add<<<1, 1>>>(d_a, d_b, d_c);
cudaMemcpy(&c, d_c, sizeof(int), cudaMemcpyDeviceToHost);
std::cout << "Result: " << c << std::endl;
cudaFree(d_a);
cudaFree(d_b);
cudaFree(d_c);
return 0;
}
To compile the program, use this command:
nvcc my_cuda_program.cu -o my_cuda_program
This sets up a basic GPU computation using Llama.cpp in conjunction with CUDA.
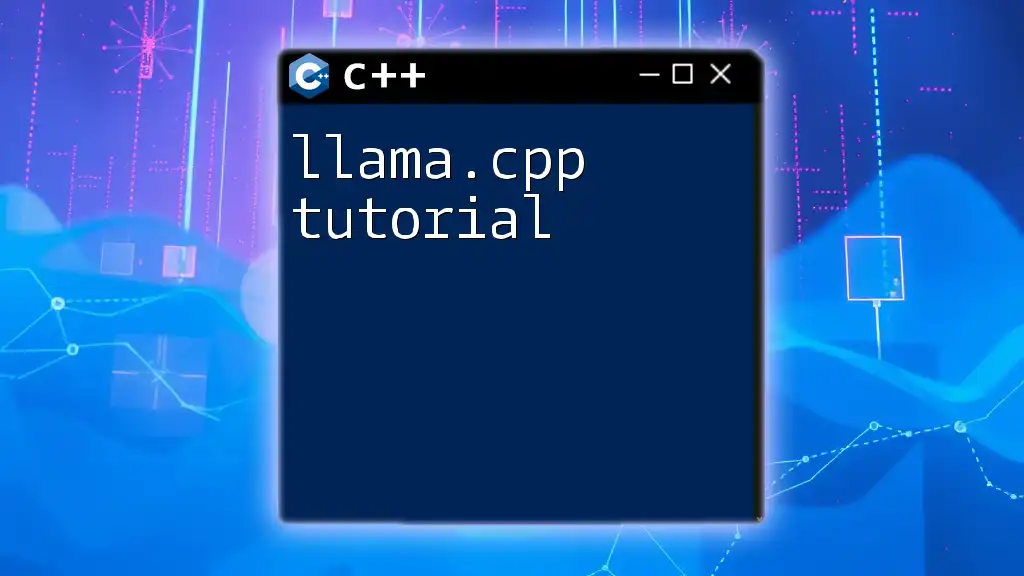
Building GPU-Accelerated Applications with Llama.cpp
GPU Architecture Basics
Understanding the basic architecture of a GPU is crucial for optimizing your applications. GPUs use thousands of small cores designed for handling multiple tasks simultaneously, which allows them to perform parallel processing efficiently. By harnessing this capability, Llama.cpp allows you to run code that scales with the number of available cores effectively.
When working on GPU memory management, ensure that you are making the best use of device memory by minimizing transfers between host and device as much as possible. This is essential for maximizing the efficiency of your applications.
Writing Efficient CUDA Kernels
A kernel is a function that runs on the GPU. Writing efficient CUDA kernels involves understanding how to structure your code to run optimally on the parallel architecture. Here’s an example of a basic kernel function to add two numbers:
__global__ void kernelFunction(int a, int b, int *c) {
*c = a + b;
}
This function, once launched from the host code, will execute on the GPU, showcasing how to perform GPU tasks using simplified syntax.
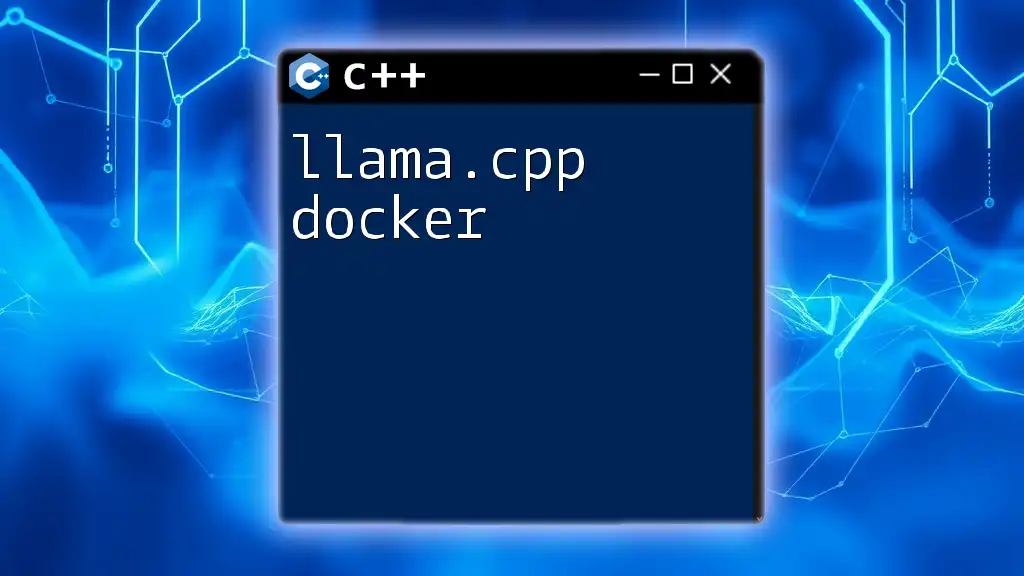
Advanced Techniques in Llama.cpp and CUDA
Memory Management
Efficient memory management is critical to achieving optimal performance in CUDA applications. CUDA provides APIs for managing memory directly on the GPU, allowing you to allocate and free memory as needed. It's important to ensure you're minimizing memory transfers to improve the speed of your application. Here’s how to allocate memory on the GPU:
float *d_array;
cudaMalloc((void**)&d_array, size * sizeof(float));
By effectively managing memory, you can reduce overhead and maximize efficiency in your applications.
Stream Processing
Stream processing enables concurrent execution of kernels and can significantly speed up your applications. Streams allow multiple operations to occur independently without waiting for previous tasks to complete. Here’s a simple implementation of stream processing in CUDA:
cudaStream_t stream;
cudaStreamCreate(&stream);
// Launch kernel in the stream
kernelFunction<<<1, 1, 0, stream>>>(a, b, d_c);
cudaStreamSynchronize(stream);
Using streams can lead to better resource utilization and improved performance in your applications.
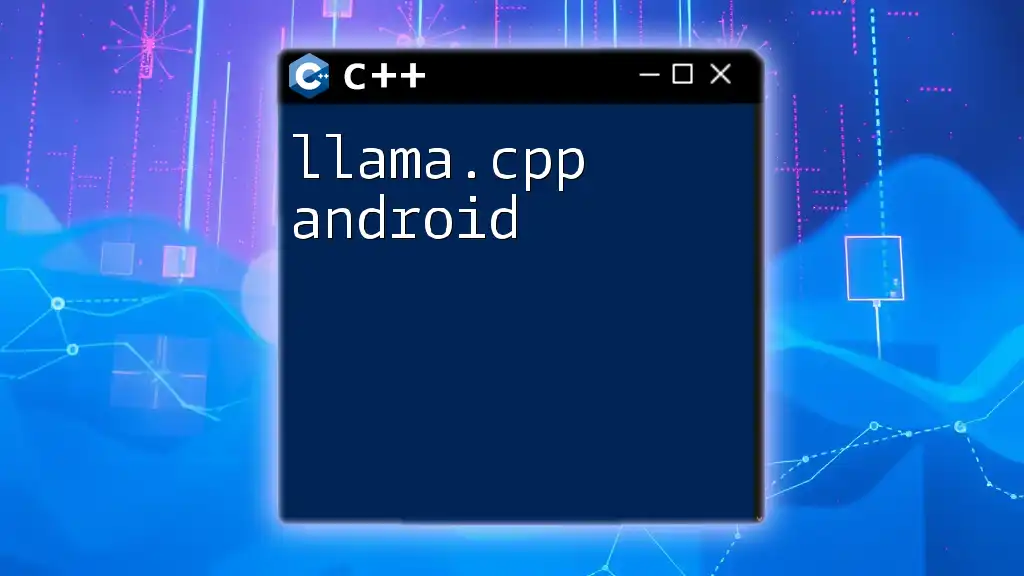
Debugging and Optimization
Common Errors in CUDA Programming
When developing CUDA applications, you may encounter typical errors such as out-of-memory conditions, kernel launch failures, or illegal memory access. To troubleshoot these errors, you can utilize tools like cuda-memcheck and NVIDIA Nsight to identify and resolve issues swiftly.
Optimization Techniques
To optimize your CUDA code, consider the following best practices:
- Minimize memory transfers between the host and the device.
- Use shared memory to enhance memory access for frequently accessed data.
- Profile your applications using tools like NVIDIA Visual Profiler to identify bottlenecks.
These techniques will help you refine your code, ensuring maximum efficiency and performance in your applications.
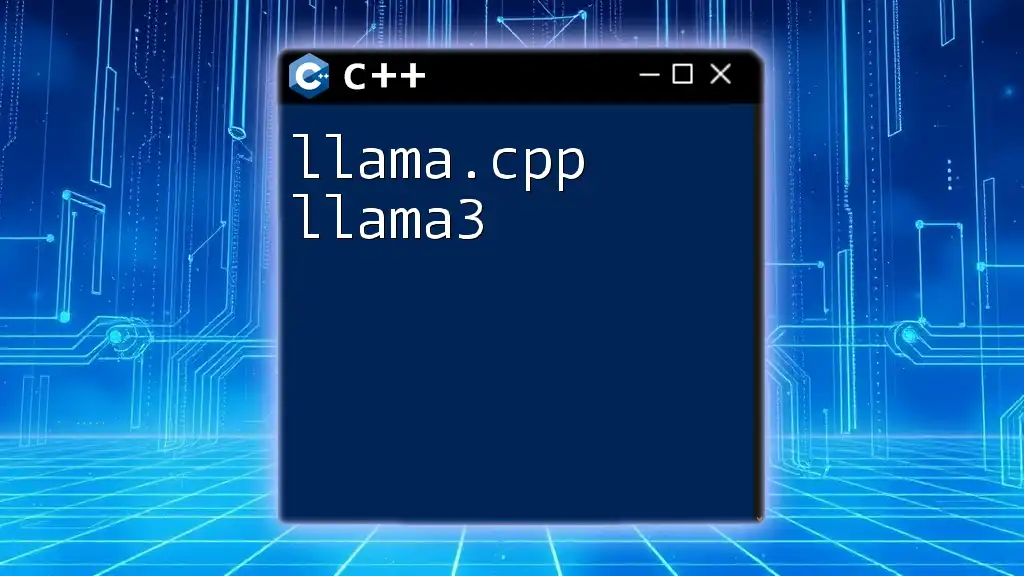
Real-World Projects with Llama.cpp and CUDA
Case Studies
Incorporating Llama.cpp and CUDA into real-world projects has shown significant performance improvements across various fields, such as image processing, machine learning, and simulations in scientific computing. For instance, many organizations report a reduction in processing time by over 50% when switching from CPU to GPU using CUDA-optimized applications built with Llama.cpp.
Contributing to the Llama.cpp Community
The Llama.cpp community is consistently growing, providing opportunities for developers to connect and share ideas. You can contribute by reporting issues, adding new features, or extending documentation. Engaging with the community enhances knowledge sharing and collaboration, leading to better development practices and expanded capabilities of the library.
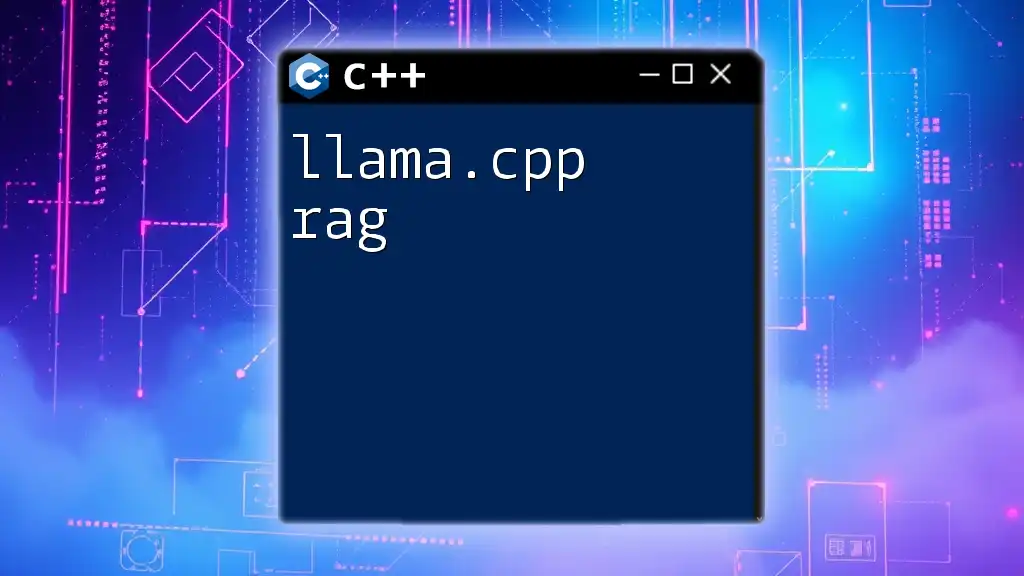
Conclusion
In summary, understanding llama.cpp cuda opens up a world of possibilities for developers interested in high-performance computing and machine learning. By leveraging the capabilities of CUDA and becoming proficient with C++ commands, programmers can create efficient and powerful applications. Exploring advanced topics in Llama.cpp will further enhance your skill set, allowing you to build innovative solutions that harness the full potential of GPU computing.
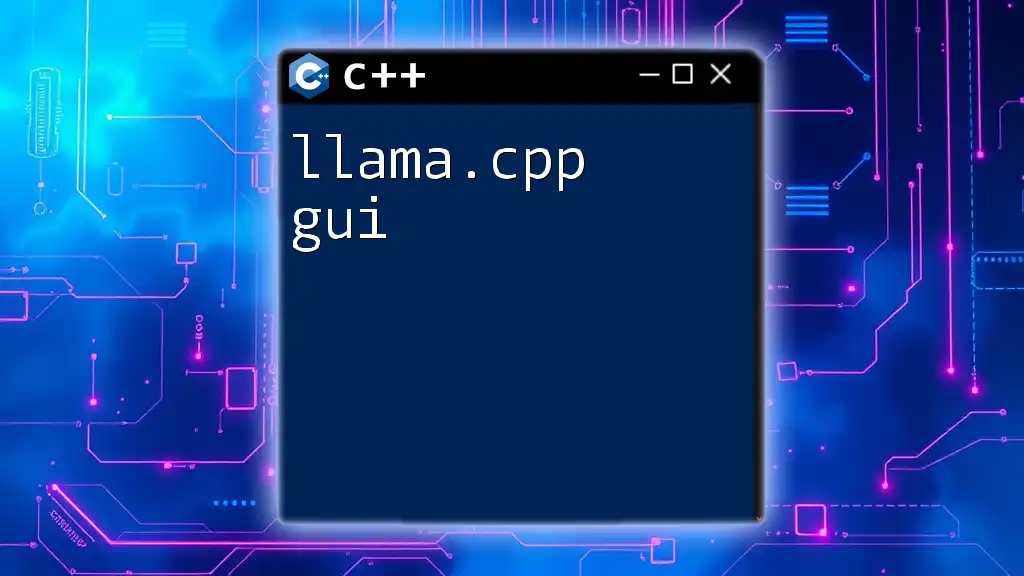
Call to Action
Explore Our Courses on C++ Commands! Dive deeper into the world of C++ and GPU programming, and join our community of C++ enthusiasts eager to learn and grow.