The "llama.cpp rag" refers to a specific component or library in the Llama C++ ecosystem that may involve utilizing command functionalities efficiently, allowing developers to integrate various capabilities into their applications.
Here’s a sample code snippet that demonstrates a basic command usage in a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, Llama!" << std::endl; // Basic output command
return 0;
}
What is `llama.cpp rag`?
`llama.cpp rag` stands for "retrieval-augmented generation" implemented in C++. It is designed to enhance text processing and facilitate interaction with Large Language Models (LLMs). This framework leverages advanced methodologies in natural language processing (NLP) and aims to improve the efficiency and accuracy of text-based applications. Understanding `llama.cpp rag` allows developers to harness the full potential of LLMs while streamlining their workflows.
Developed in response to the growing need for effective text manipulation tools, `llama.cpp rag` incorporates essential features that cater to both novice and experienced programmers. Its foundational role in modern programming practices cannot be overstated, as it acts as a bridge between raw data and meaningful insights.
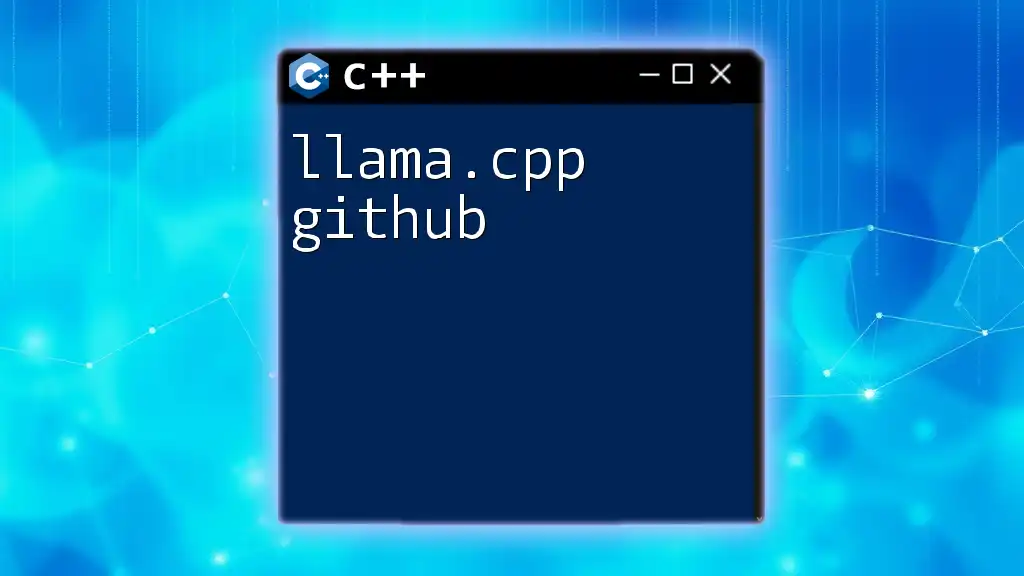
Key Features of `llama.cpp rag`
Enhanced Text Processing
One of the standout features of `llama.cpp rag` is its powerful text manipulation capabilities. By utilizing built-in functions, developers can easily transform, analyze, and generate text in no time.
Example:
// Simple text manipulation using llama.cpp rag
std::string text = "Hello, World!";
std::string modifiedText = manipulateText(text);
This example illustrates how a straightforward function can drastically change or enhance string data, making it easier to handle various text-related tasks.
Usability with Large Language Models (LLMs)
`llama.cpp rag` excels in implementing RAG strategies that augment the capabilities of LLMs. By enabling the retrieval of information from vast databases or knowledge sources, this tool improves the context of generated text. For instance, when a user poses a question, the retrieval system can identify relevant information to produce a more accurate and context-aware response. This synergistic functionality ensures that developers can build applications capable of sophisticated interactions with users or data systems.
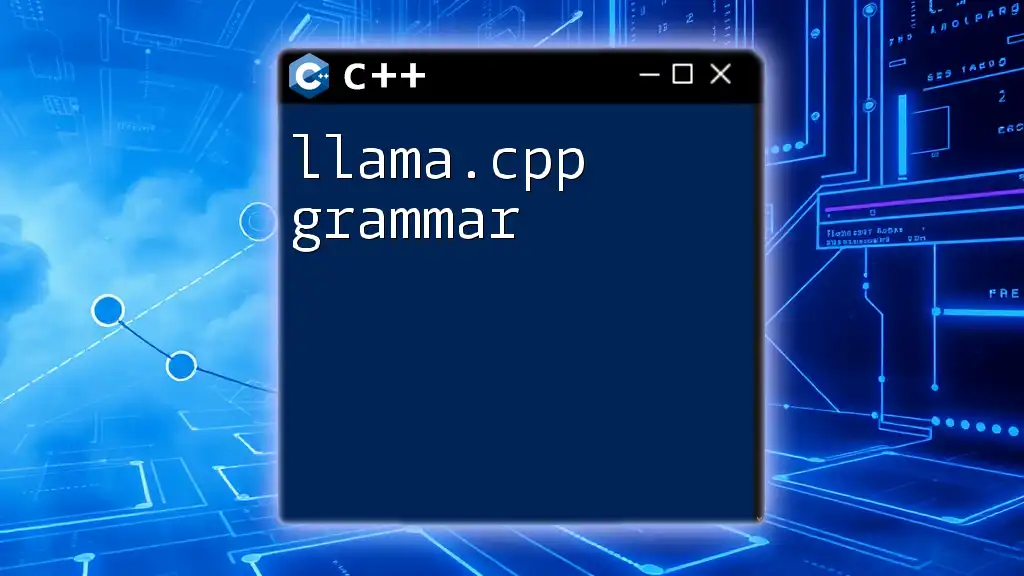
Setting Up `llama.cpp rag`
Prerequisites
Before diving into the usage of `llama.cpp rag`, it's essential to have the right environment set up. Ensure you have:
- A compatible C++ compiler (e.g., GCC or Clang).
- Libraries necessary for text processing, such as `Boost`.
- Access to the `llama.cpp rag` library files.
Installation Steps
To install `llama.cpp rag`, follow these instructions:
- Download the library files from the official repository or relevant source.
- Open your terminal or command line interface.
- Navigate to your project directory and execute the following commands:
# Clone the repository
git clone https://github.com/your-repo/llama.cpp rag.git
# Navigate to the directory
cd llama.cpp_rag
# Build the project if necessary (this might differ based on specific library requirements)
make
After installation, verify its success by checking if the commands work as expected and display no errors.
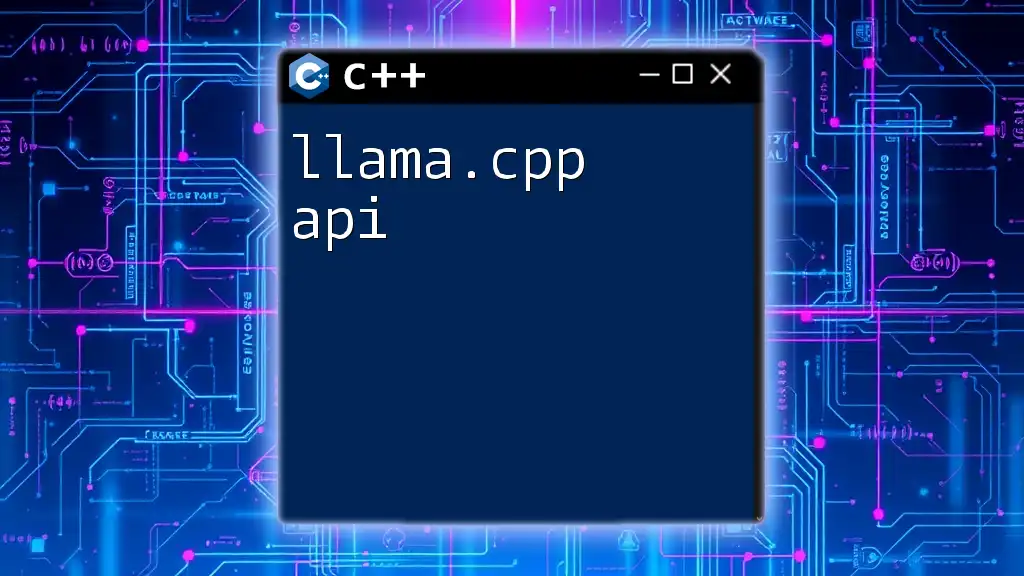
Basic Usage of `llama.cpp rag`
Importing the Library
To begin using `llama.cpp rag` in your C++ project, you first need to include the library header in your code. This is accomplished with the following line:
#include "llama_cpp_rag.h"
Including this header file is crucial as it enables the integration of all functionalities provided by the `llama.cpp rag` library.
Basic Commands and Functions
Command: Text Generation
One of the core functionalities of `llama.cpp rag` is text generation, an essential aspect for applications focused on NLP. This command allows developers to create responses dynamically based on input questions or prompts.
Example:
// Generate a sample response using llama.cpp rag
std::string response = generateResponse("What is RAG?");
This function call takes the query and generates a pertinent response by leveraging RAG methodologies, making it highly effective for interactive applications.
Command: Text Manipulation
Beyond simple text generation, `llama.cpp rag` provides advanced functions for text manipulation. You can format, tokenize, or modify strings with ease. Understanding the parameters and expected outputs is crucial for effective implementation.
// Code snippet performing text manipulation
std::string original = "Learning C++ with llama.cpp rag";
std::string modified = transformText(original); // A function defined within the library
Focusing on functions that allow for in-depth text analysis broadens the application possibilities for your C++ projects.
Error Handling
As with any programming tool, error handling is crucial for maintaining application stability. `llama.cpp rag` comes with built-in mechanisms to catch and manage common errors effectively. Below is a practical example:
try {
// Risky operation
std::string result = performRiskyOperation();
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
This code snippet showcases basic error handling techniques, helping developers gracefully manage potential runtime issues.
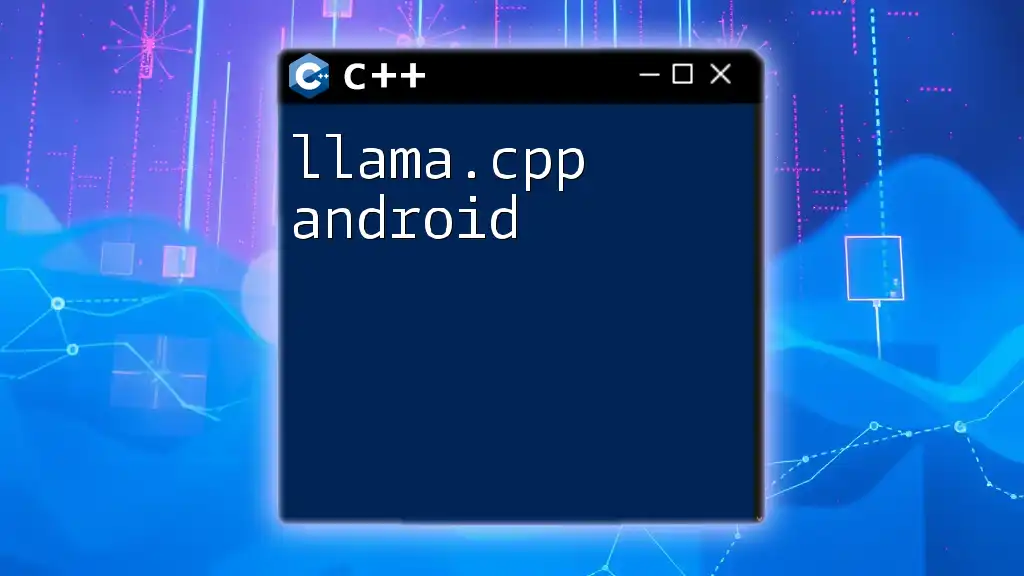
Advanced Features of `llama.cpp rag`
Customizing Functionality
For developers aiming to tailor functionalities to specific needs, `llama.cpp rag` offers extensive customization options. By extending functions or incorporating callback mechanisms, you can build applications that perform optimally under varying conditions.
Integrating with Other Libraries
To maximize usability and performance, consider integrating `llama.cpp rag` with other C++ libraries like Boost or OpenCV. These integrations can lead to enhanced functionality and a more robust application experience.
Example: Integrating with Boost allows for advanced string manipulation and memory management, which can further improve the performance of your `llama.cpp rag` implementations.
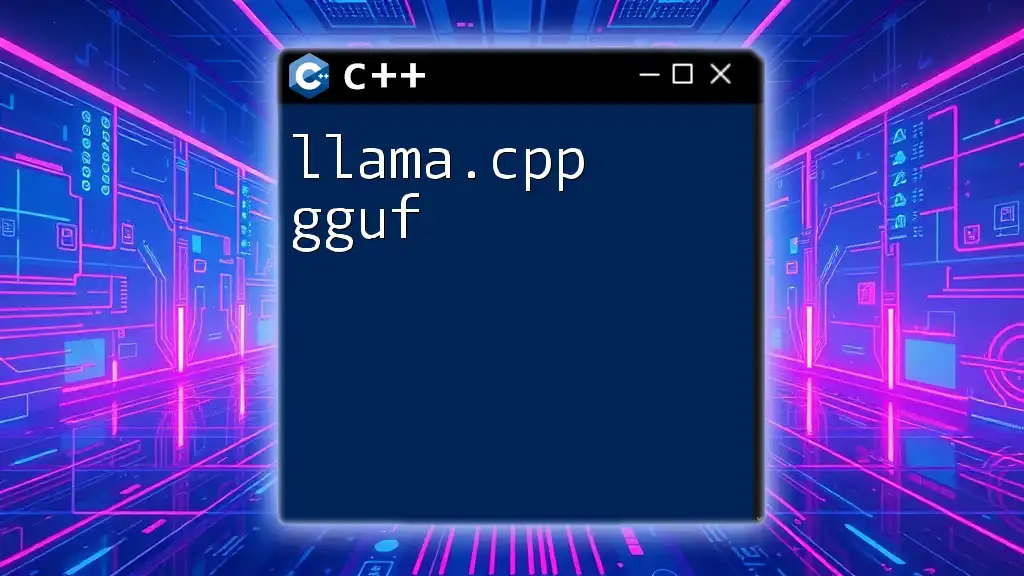
Best Practices for Using `llama.cpp rag`
Code Optimization Tips
Optimization is key to effective programming. Use these techniques when working with `llama.cpp rag`:
- Review and refactor your code frequently to eliminate redundancy.
- Analyze the performance of your processes, particularly during data handling.
Testing and Debugging
Ensure your implementations are solid by regularly testing and debugging your code. Utilize tools such as `gdb` or built-in IDE debuggers to identify issues efficiently. Create test cases that mimic real-use scenarios to ensure comprehensive coverage.
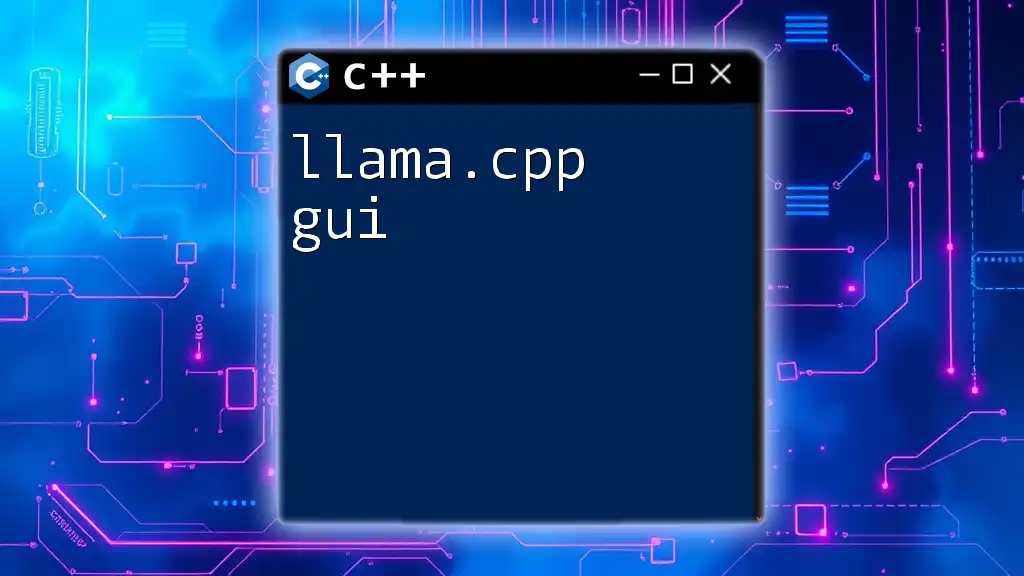
Common Use Cases
Use Case 1: Natural Language Processing
`llama.cpp rag` shines in NLP applications, allowing easy and efficient text analysis and response generation. Here’s how you can implement a simple NLP application:
#include "llama_cpp_rag.h"
int main() {
std::string userInput = "Explain RAG in detail.";
std::string generatedResponse = generateResponse(userInput);
std::cout << "Response: " << generatedResponse << std::endl;
return 0;
}
This application captures user input, queries `llama.cpp rag`, and outputs the resulting response—illustrating the power of combining user interactions with text generation.
Use Case 2: Data Analysis
In data analysis, `llama.cpp rag` can assist in processing and interpreting large datasets. For example, you could use its functionalities to derive insights and textual summaries from datasets with minimal effort.
std::vector<std::string> data = fetchDataFromSource();
std::string report = generateReport(data);
std::cout << report << std::endl;
Such capabilities facilitate efficient data evaluations and enhance decision-making processes within applications.
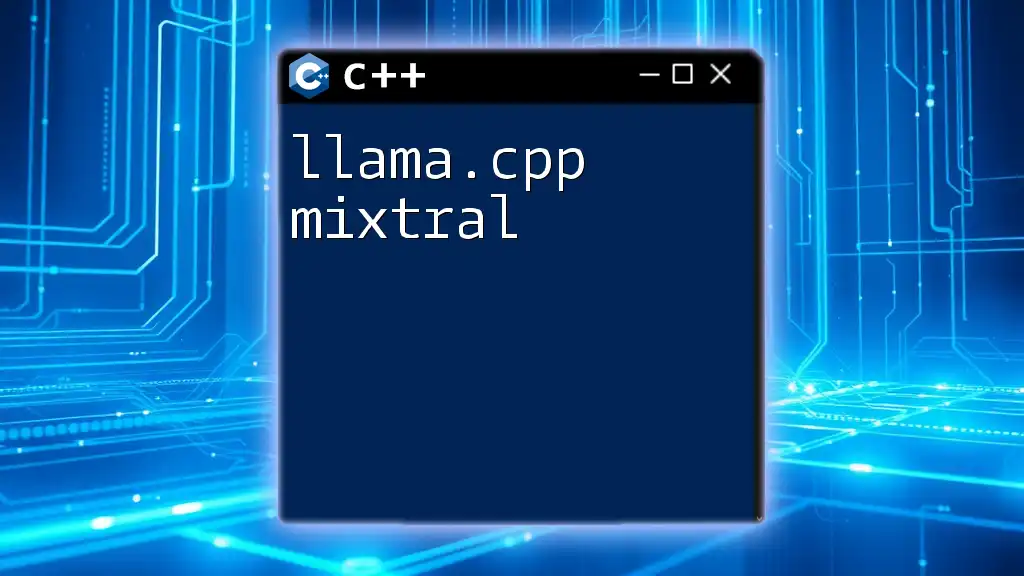
Conclusion
The in-depth exploration of `llama.cpp rag` reveals its incredible potential for enhancing C++ applications, especially those dealing with text and language-based tasks. By mastering its commands and techniques, developers can create applications that are not only efficient but also capable of sophisticated language interactions.
Take this opportunity to experiment with `llama.cpp rag`, implementing its features in your projects, and don't hesitate to explore further resources for an even deeper understanding. Your journey into advanced C++ programming awaits you, and `llama.cpp rag` is one of the invaluable tools in your toolkit.