The `llama.cpp` library facilitates the integration and usage of the LLaMA 3 model in C++ applications, enabling efficient execution of AI tasks.
Here's an example code snippet to illustrate its usage:
#include "llama.h"
int main() {
Llama::Model model("path/to/llama3/model");
model.load();
auto response = model.generate("What is the capital of France?");
std::cout << response << std::endl;
return 0;
}
What is llama.cpp?
llama.cpp is a C++ source code file that plays a crucial role in defining the foundational elements of certain algorithms and data structures you'd encounter in modern C++ programming. This file is significant because it provides modular and reusable code, which is a cornerstone of efficient programming practice.
Key features of llama.cpp
One of the standout attributes of llama.cpp is its modular design. This design philosophy promotes code reusability and organization, allowing programmers to implement specific functionalities without redundant code. Moreover, it emphasizes ease of understanding, making it accessible for developers at different skill levels.
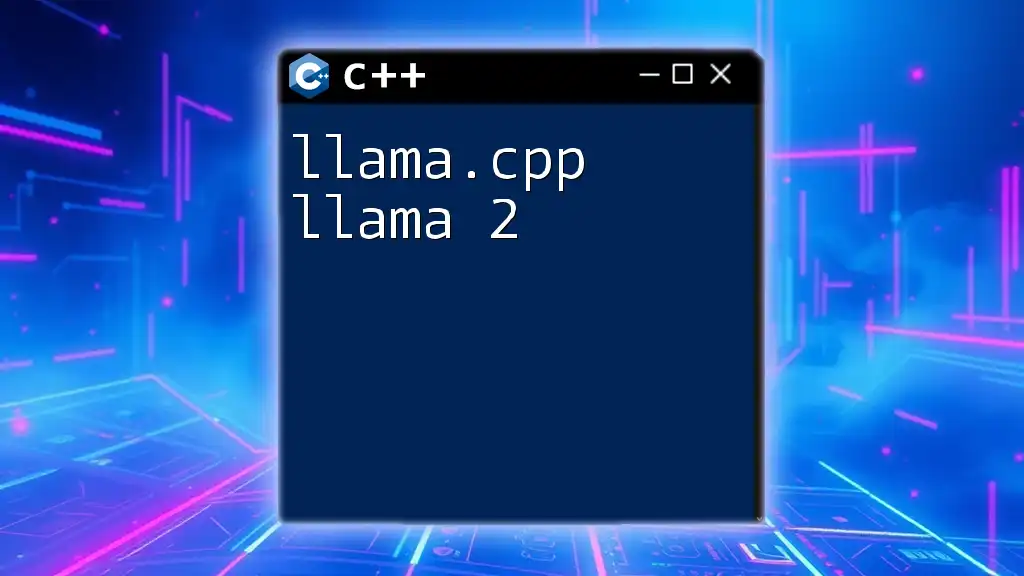
Understanding the llama3 Architecture
llama3 represents a notable evolution in the llama codebase, offering crucial enhancements over its predecessors. By focusing on performance improvements and extending functionality, llama3 sets a new standard in coding practices.
Core components of llama3
-
Data Structures: The architecture relies on well-defined data structures, which are essential for managing data efficiently. For example, lists and arrays are utilized for storing collections of items, allowing streamlined data access.
-
Functions and Methods: A significant aspect of llama3 is its collection of functions, which perform various operations on the data structures. Understanding these methods is pivotal, as they facilitate functionality and encapsulation.
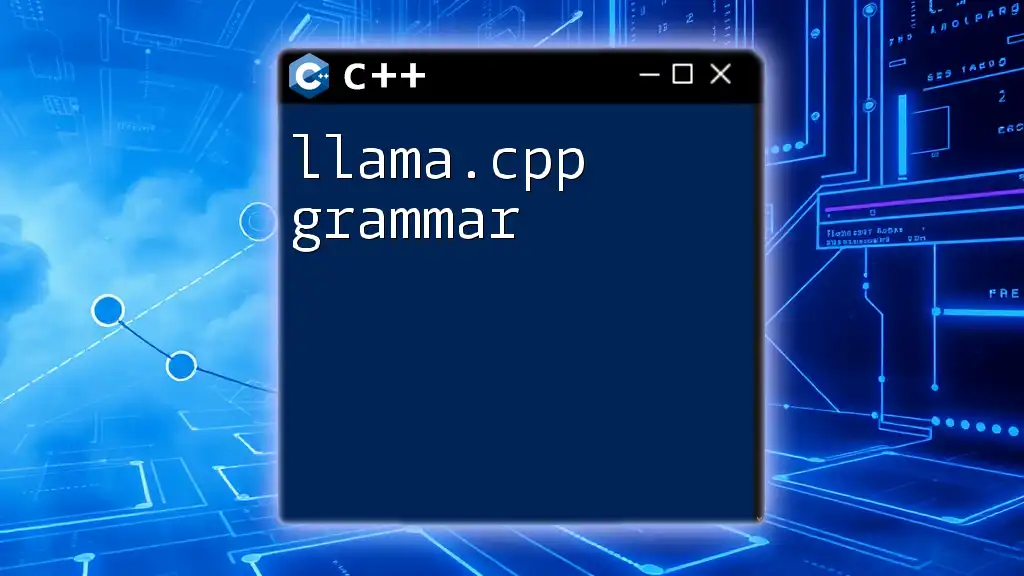
Getting Started with llama.cpp and llama3
Before diving into the code, you'll first need the right environment set up to work with llama.cpp and llama3.
Setting up your environment
To effectively utilize llama.cpp and llama3, ensure you have the following software installed:
- A modern C++ compiler (e.g., GCC or Clang)
- An IDE or text editor with C++ support (such as Visual Studio Code, CLion, or Code::Blocks)
Once your environment is ready, you can easily load llama.cpp into your C++ project by setting up the project structure appropriately.
Loading llama.cpp in your C++ project
Ensure that your project directory is organized properly:
/MyProject
├── src
│ └── llama.cpp
└── main.cpp
In `main.cpp`, integrate llama.cpp with the following code snippet:
#include "llama.cpp" // Sample integration
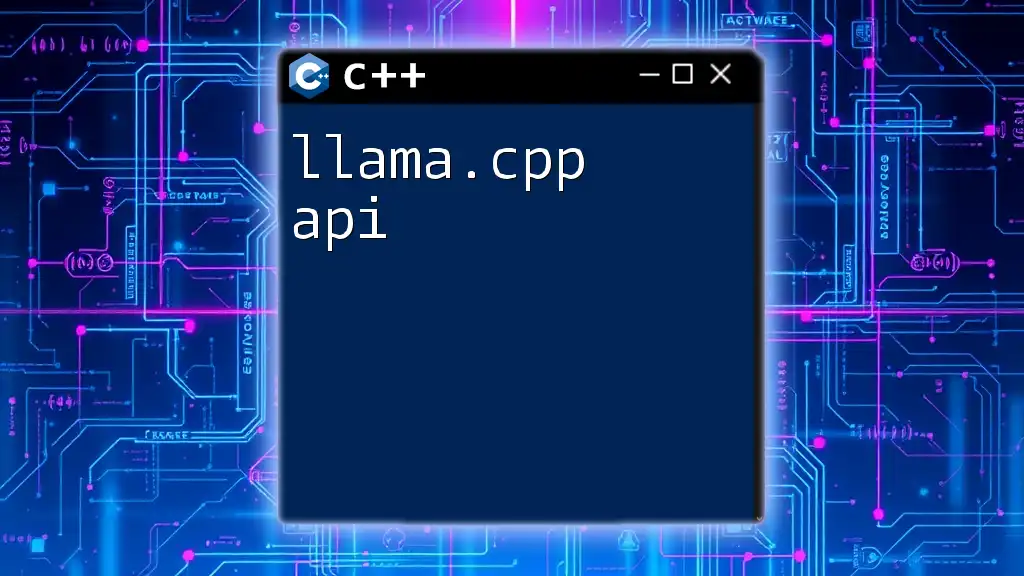
Key C++ Commands Used in llama.cpp and llama3
Basic Commands
To grasp the essence of programming with llama.cpp and llama3, you should familiarize yourself with basic C++ commands. For instance, printing to the console is as simple as:
int main() {
std::cout << "Hello, World!" << std::endl; // Basic output command
return 0;
}
This command not only provides a visual confirmation of execution but also illustrates the fundamental structure of a C++ program.
Advanced Commands
Moving to more advanced commands, you’ll encounter functions that enhance the functionality of your code. Here’s an example of a user-defined function:
void myFunction(int a, float b) {
// Sample function demonstrating C++ function syntax
}
This method shows how to encapsulate logic effectively, promoting code reuse and simplifying debugging.
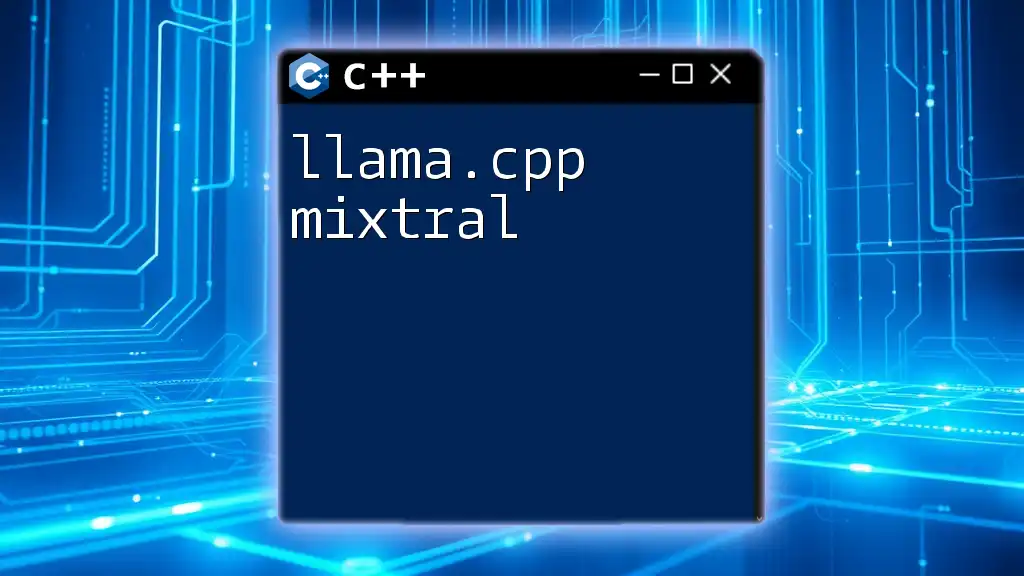
Code Examples in llama3
Simple Algorithms Implemented in llama3
Within llama3, simple algorithms such as sorting can be implemented with efficiency. For example, you can sort an array of integers with:
#include <algorithm>
#include <vector>
int main() {
std::vector<int> arr = {5, 2, 8, 1, 4};
std::sort(arr.begin(), arr.end()); // Using STL to sort the array
return 0;
}
Such examples illustrate how powerful the Standard Template Library (STL) can be when integrated with user-defined code.
Complex Algorithms
Complex algorithms also find their place in llama3. Consider a breadth-first search (BFS) algorithm for traversing a graph:
#include <queue>
#include <vector>
void bfs(int start, const std::vector<std::vector<int>>& graph) {
std::queue<int> toVisit;
std::vector<bool> visited(graph.size(), false);
toVisit.push(start);
visited[start] = true;
while (!toVisit.empty()) {
int node = toVisit.front();
toVisit.pop();
// Process node...
for (int neighbor : graph[node]) {
if (!visited[neighbor]) {
toVisit.push(neighbor);
visited[neighbor] = true;
}
}
}
}
Implementing such algorithms not only expands your coding capabilities but also enhances your problem-solving skills.
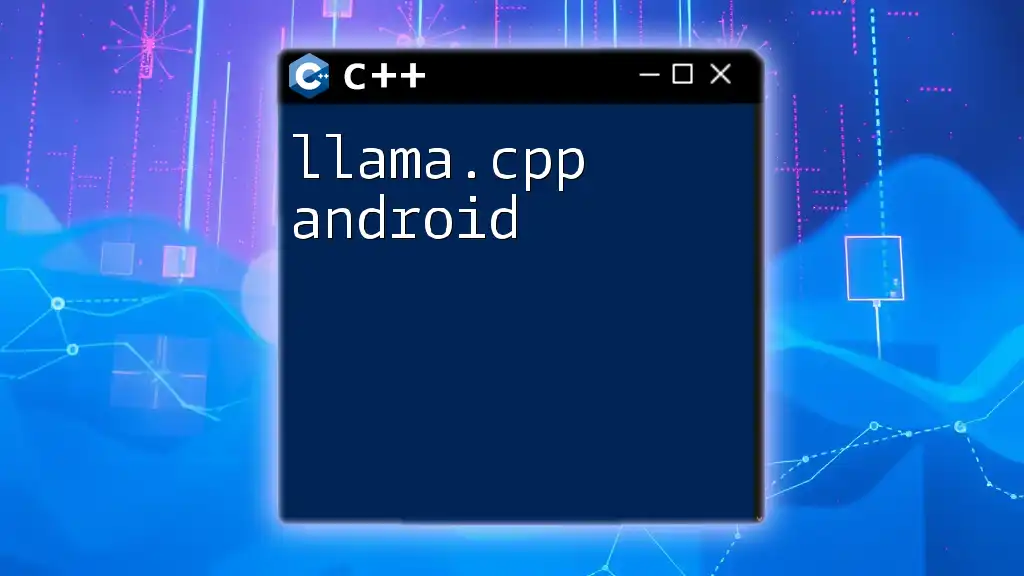
Best Practices for Using llama.cpp and llama3
When working with llama.cpp and llama3, adhering to best practices is vital for maintaining clarity and performance. A few recommendations include:
-
Code Organization: Keep related functions and data structures together, making the codebase easier to navigate.
-
Commenting and Documentation: Document your code thoroughly, ensuring future developers (or even yourself) can easily understand your design choices.
-
Performance Optimization Techniques: Profile your code to identify bottlenecks, and adopt efficient data structures and algorithms to optimize execution time.
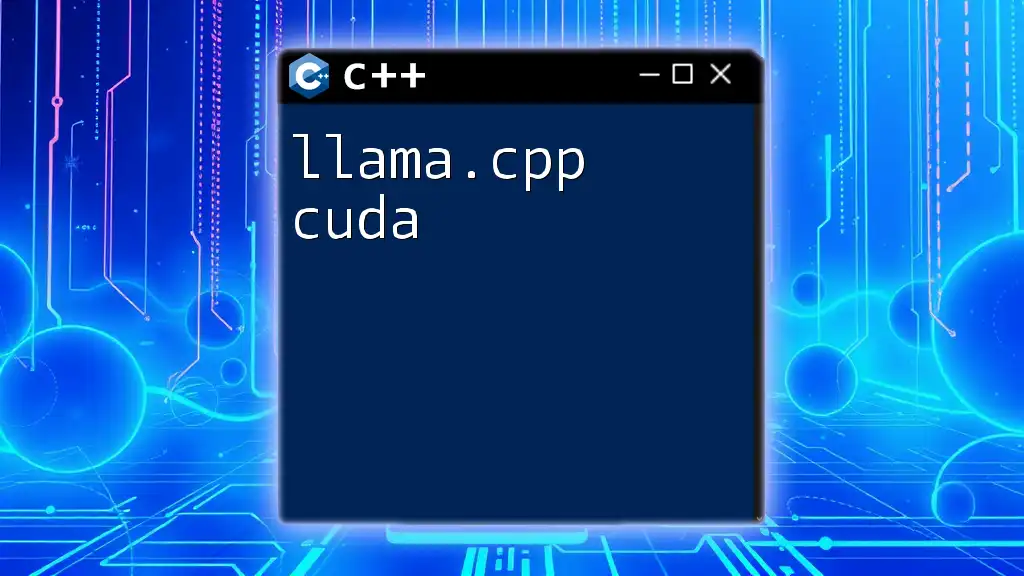
Troubleshooting Common Issues
Compilation Errors
As with any coding endeavor, you may encounter compilation errors while working with llama3. Common issues include missing files or incorrect include paths. Remember to check the console output and ensure your environment is set up correctly.
Logic Errors
Logic errors can be particularly challenging to identify. To resolve them, adopt debugging techniques, such as printing variable values at different execution stages:
if (x != expected) {
std::cerr << "Unexpected value: " << x << std::endl; // Logic error handling
}
Using debug statements will often provide clues leading you to the root of the problem.
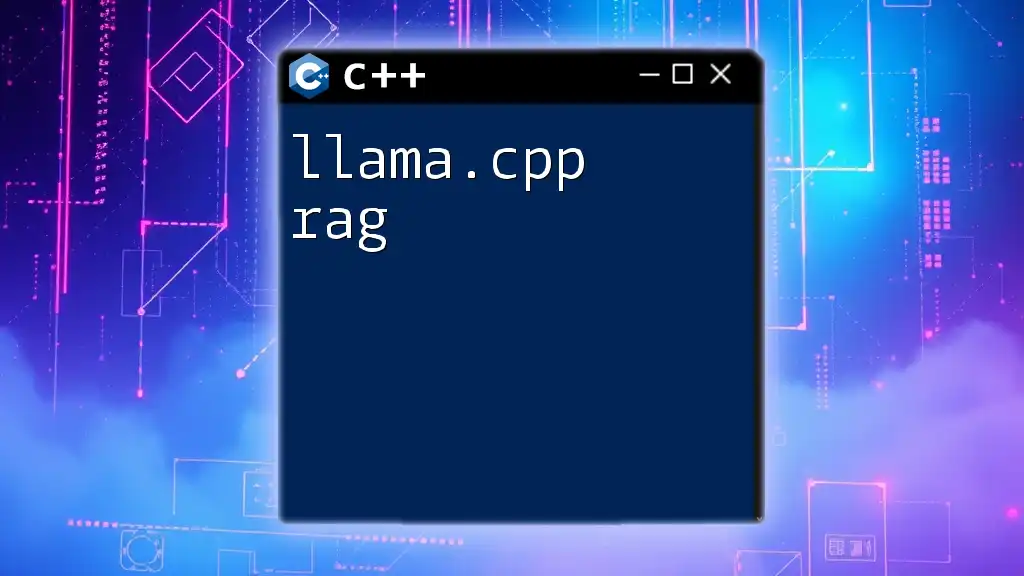
Conclusion
In summary, mastering llama.cpp and llama3 requires understanding their architecture, key commands, and best practices. By applying these lessons to real-world coding projects, you'll not only enhance your programming skills but also gain a deeper appreciation for efficiently structured code. Engage with the broader C++ community to further enrich your learning experience and to keep pace with the evolving standards of programming.
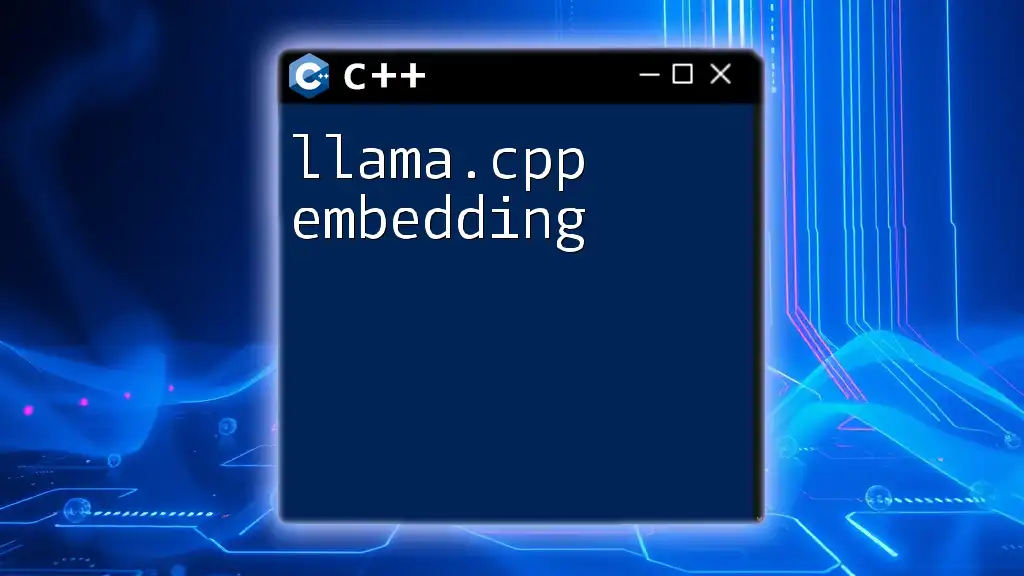
Additional Resources
To continue your journey in mastering C++, consider exploring recommended books and online courses, including documentation and tutorials that provide deeper insights into llama.cpp and llama3. Connecting with forums and support channels can also help you stay updated with the latest developments.
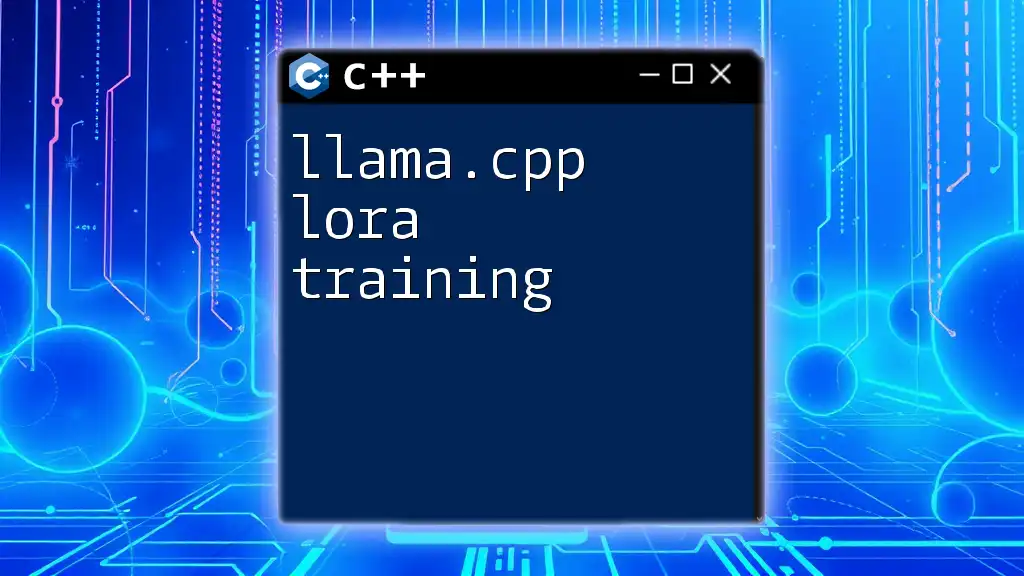
Frequently Asked Questions
What is the difference between llama.cpp and llama3?
While llama.cpp acts as a foundational code element, llama3 builds upon it by introducing performance enhancements and refined functionalities.
How can I effectively learn to use llama3?
To master llama3, practice consistently, participate in coding challenges, and utilize community resources to supplement your formal learning. Hands-on coding experience is invaluable for grasping complex concepts.