The `llama.cpp` library provides a fast and efficient way to implement the LLaMA 2 model for natural language processing tasks in C++.
Here is a simple code snippet to load the model and generate a response:
#include "llama.h"
int main() {
llama::Model model("path/to/llama2/model");
std::string text = model.generate("What is the capital of France?");
std::cout << text << std::endl;
return 0;
}
What is Llama 2?
Llama 2 is an advanced version of its predecessor designed to enhance the capabilities and functionalities of command handling within C++. It serves as a powerful tool for developers looking to streamline their programming processes, improve efficiency, and leverage advanced features for data handling and manipulation.
Understanding Llama 2 is crucial in today’s development landscape as it not only simplifies complex tasks but also allows developers to implement intricate solutions quickly. Learning how to use llama.cpp with Llama 2 can give you a competitive edge in programming.
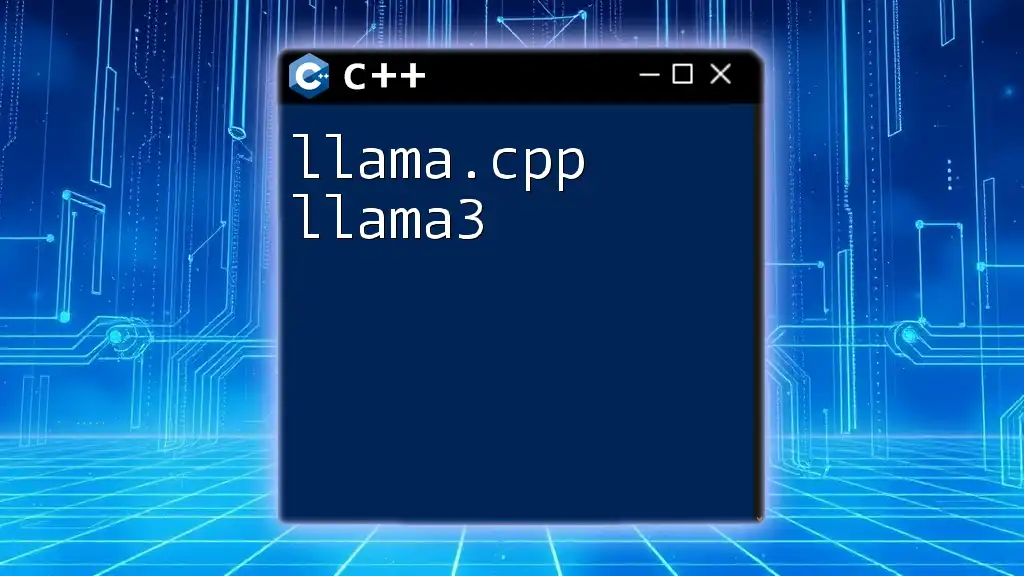
Why Use llama.cpp?
The llama.cpp interface is a lightweight framework that facilitates interaction with Llama 2 commands. It acts as a bridge, translating developer inputs into actionable commands the system can understand. Some advantages include:
- Simplicity: It provides a straightforward way to execute Llama 2 commands without delving into complex syntax.
- Efficiency: By leveraging the capabilities of Llama 2, developers can execute commands faster and with less code.
- Rich Features: It incorporates advanced functionalities that allow for sophisticated data operations.
Incorporating llama.cpp into your development process can lead to significant improvements in productivity and reduced learning time for new commands.
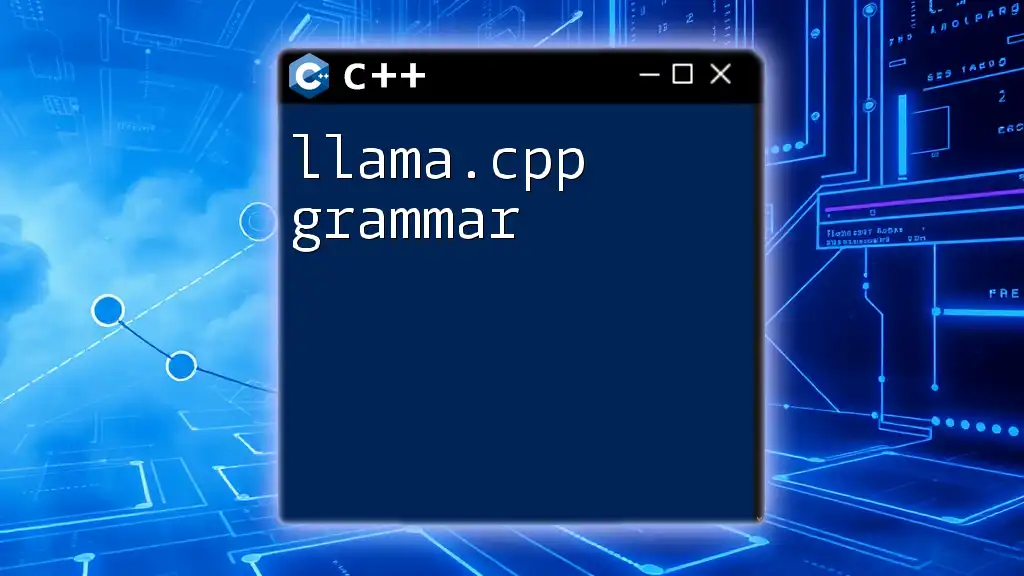
Getting Started with llama.cpp
Setting Up Your Environment
Before diving into the commands of Llama 2, you need to set up your development environment correctly. Here are the prerequisites:
- C++ Development Tools: Ensure you have a C++ compiler and development environment installed (e.g., GCC, Visual Studio).
- llama.cpp Library: Clone or download the llama.cpp repository from the official source.
To install and set up llama.cpp:
- Download the llama.cpp files and add them to your project directory.
- Include the llama.cpp header in your main C++ file:
#include "llama.h"
- Compile your project with the necessary flags to link against the llama.cpp library.
Basic Syntax and Structure
Familiarizing yourself with the syntax used in llama.cpp is essential. The basic structure typically follows this format:
llama_command("your_command_here", parameters);
For example, a simple command to print "Hello, Llama 2" would look like this:
llama_command("print", "Hello, Llama 2");
This succinct syntax allows you to quickly issue commands to Llama 2 without cumbersome setup stages.
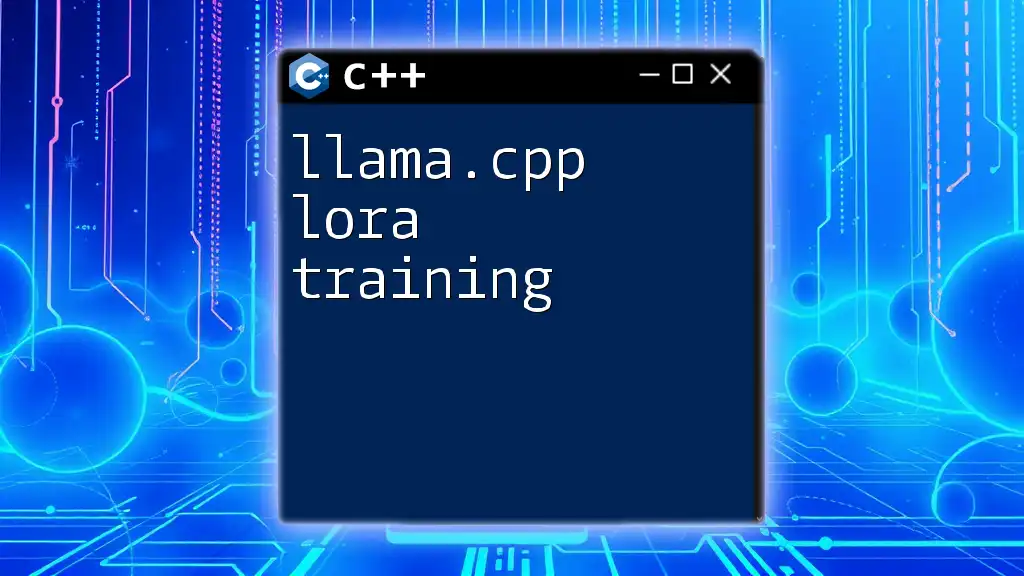
Exploring Llama 2 Commands
Core Llama 2 Commands
Understanding the core commands in Llama 2 will lay a solid foundation for more complex operations. Here are key commands you should be aware of:
- Print Command: Outputs a string to the standard output.
- Input Command: Captures user input for further processing.
- Data Manipulation Commands: Includes commands designed for sorting, filtering, and managing datasets.
Code Snippets for Each Command
Print Command Example:
llama_command("print", "Welcome to Llama 2!");
Input Command Example:
std::string userInput = llama_command("input", "Please enter your data:");
By utilizing these core commands, you can quickly build interactive applications that respond to user input.
Advanced Llama 2 Features
As you become more comfortable with basic commands, you can explore advanced features that enhance your capability to manipulate data effectively.
Working with Data Types
Llama 2 supports various data types, including integers, floats, strings, and arrays. Understanding how to manage these data types is key to leveraging Llama 2's capabilities fully.
Example for Using Arrays:
llama_command("array", {1, 2, 3, 4, 5});
Functions and Methods in Llama 2
Functions in Llama 2 are crafted to perform specific tasks. Here’s an example of defining a new function that calculates the area of a rectangle:
llama_command("defineFunction", "calculateArea", "length * width");
You can then call this function in your main program:
int area = llama_command("calculateArea", 5, 10);
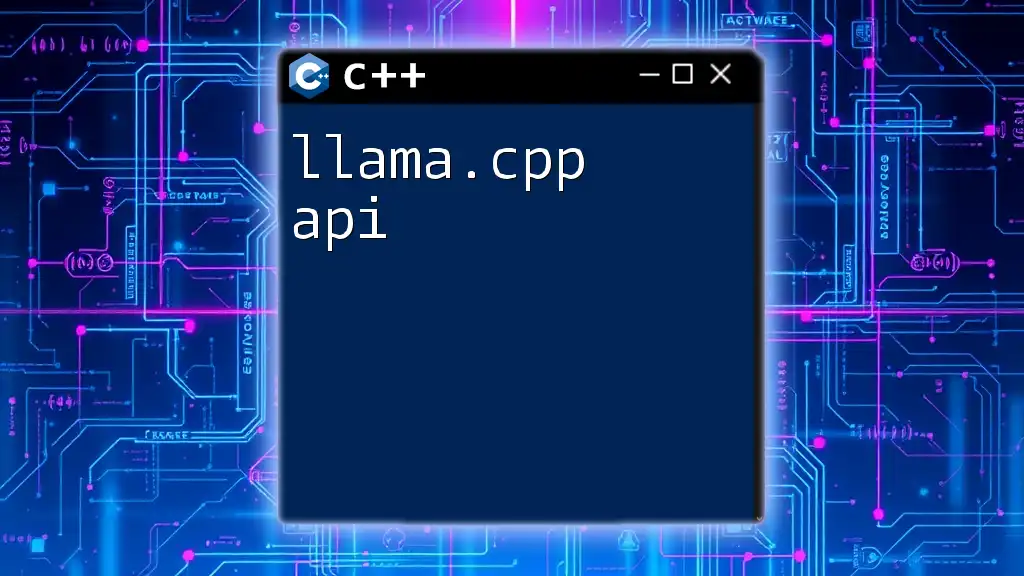
Practical Use Cases of llama.cpp and Llama 2
Real-World Applications
Llama 2 can be tremendously beneficial across various industries, such as finance for data analysis, gaming for interactive environments, and scientific research for algorithmic calculations.
Examples and Code Implementations
To illustrate some of the practical applications of llama.cpp, consider this example of processing a dataset:
std::vector<int> data = {10, 20, 30, 40, 50};
llama_command("sort", data);
This basic functionality allows you to sort datasets quickly with minimal code.
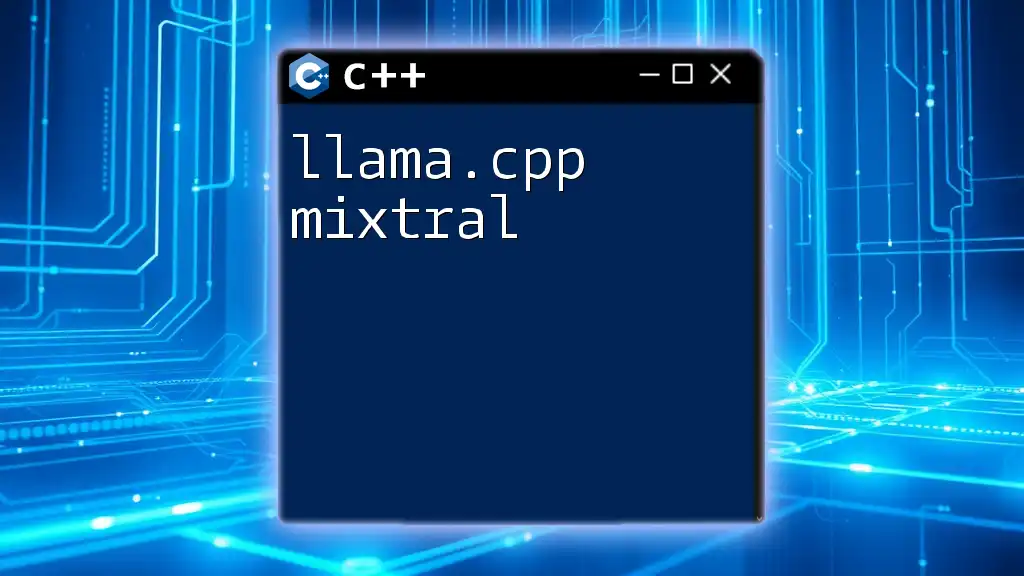
Debugging and Error Handling
Common Errors in llama.cpp
While working with llama.cpp and Llama 2, you might encounter some common issues. These can range from syntax errors to runtime exceptions caused by invalid commands.
- Syntax Errors: Ensure all commands are formatted correctly.
- Runtime Errors: Check for valid input types consistent with expected function parameters.
Solutions and Troubleshooting Tips
- Carefully read error messages and trace back to the relevant command or function.
- Utilize debugging tools in your C++ environment to set breakpoints and inspect variable states.
Best Practices
To minimize errors and enhance code clarity, adhere to these best practices:
- Keep your code modular by encapsulating functionality within functions.
- Use meaningful variable names to convey their purpose.
- Document your code for clarity and maintainability.
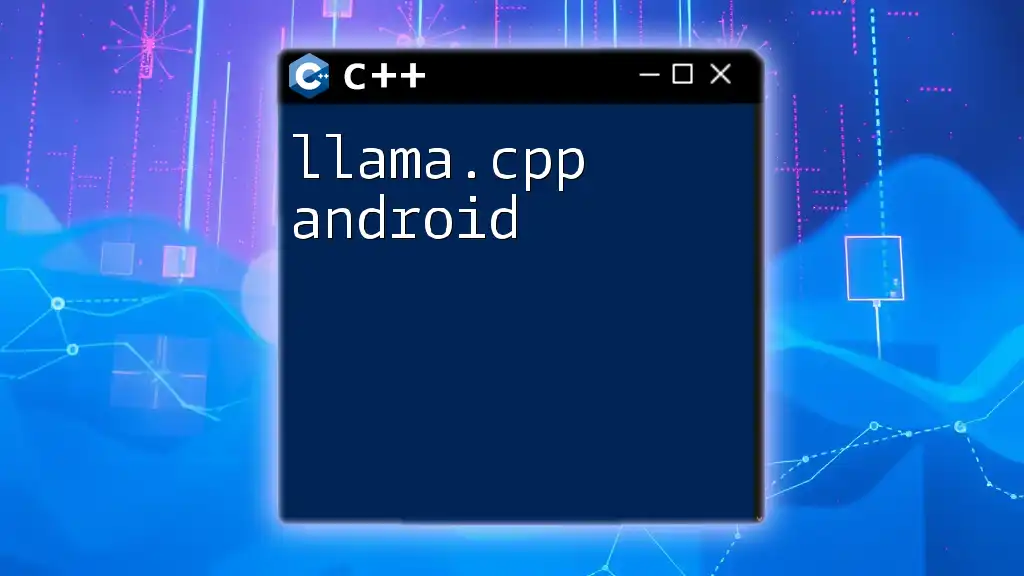
Conclusion
In summary, understanding llama.cpp llama 2 is crucial for anyone looking to enhance their programming skill set. The combination offers a powerful toolkit for executing commands efficiently. By practicing and exploring the various functionalities of Llama 2, developers can produce highly effective and interactive applications.
Next Steps for Learners
You are encouraged to dive further into the intricacies of Llama 2 and explore more advanced topics such as custom command creation and optimization techniques. Leverage available resources, tutorials, and community forums to expand your knowledge and skill set.
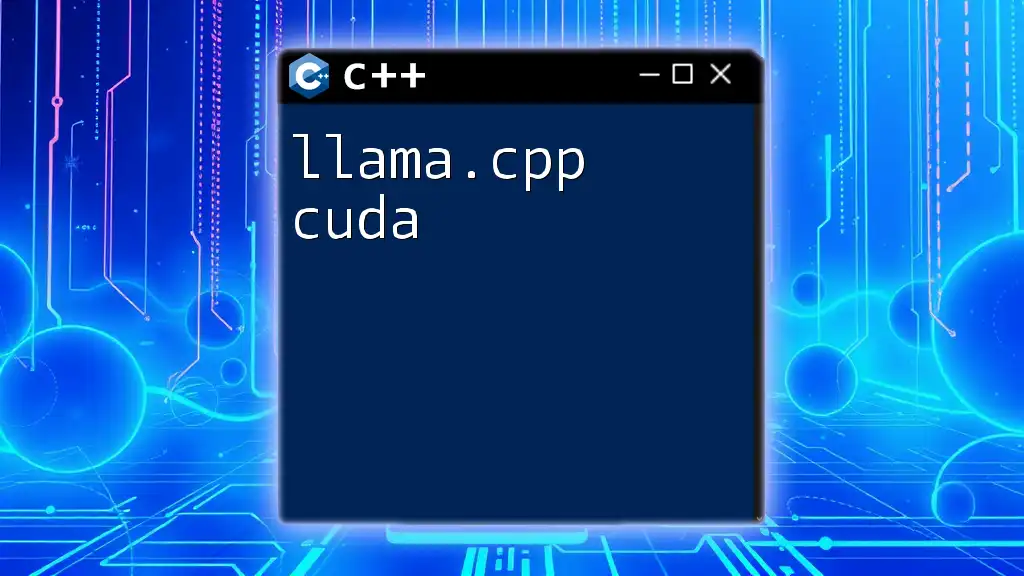
Additional Resources
Useful Links
- Official Llama 2 documentation
- C++ development tutorials and forums
- GitHub repositories with sample code and projects
Community and Support
Engage with the developer community to share knowledge, ask for help, and stay updated on evolving trends in the world of Llama 2 and C++. Collaborating with others can enhance your learning experience and lead to innovative solutions.