The `llama_cpp_python` library provides a Python interface for interacting with the LLaMA C++ model, making it easy to integrate and utilize the model's functionality within Python applications.
Here's a basic example of how to load and use the LLaMA model with this library:
# Import the llama_cpp_python module
import llama_cpp_python as llama
# Load the pre-trained LLaMA model
model = llama.load_model('path_to_model')
# Generate text using the model
output = model.generate("The future of AI is")
print(output)
What is llama_cpp_python?
The library llama_cpp_python provides a seamless integration between C++ and Python, allowing developers to leverage the efficiency and performance of C++ while maintaining the ease of scripting in Python. This bridging of two powerful programming languages is particularly advantageous in fields requiring high computational power and speed, such as data processing and machine learning.
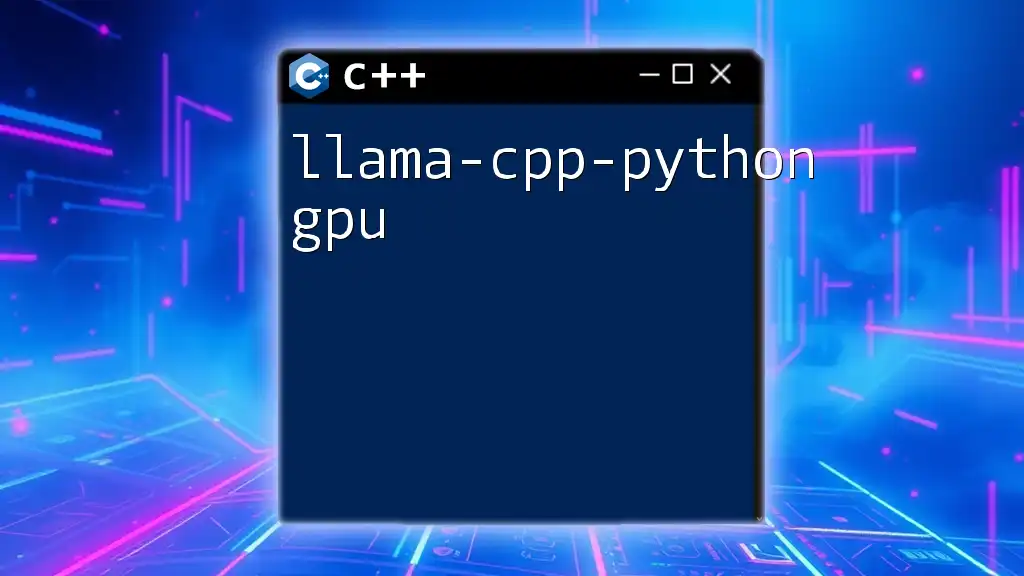
Why Use llama_cpp_python?
There are several compelling reasons to choose llama_cpp_python for your projects:
- Performance: C++ is known for its speed and efficiency, providing significant performance enhancements.
- Flexibility: You can write resource-intensive functions in C++ while keeping the user interface or high-level logic in Python.
- Ease of Use: It simplifies the process of calling C++ code from Python, enabling you to utilize existing C++ libraries without extensive refactoring.
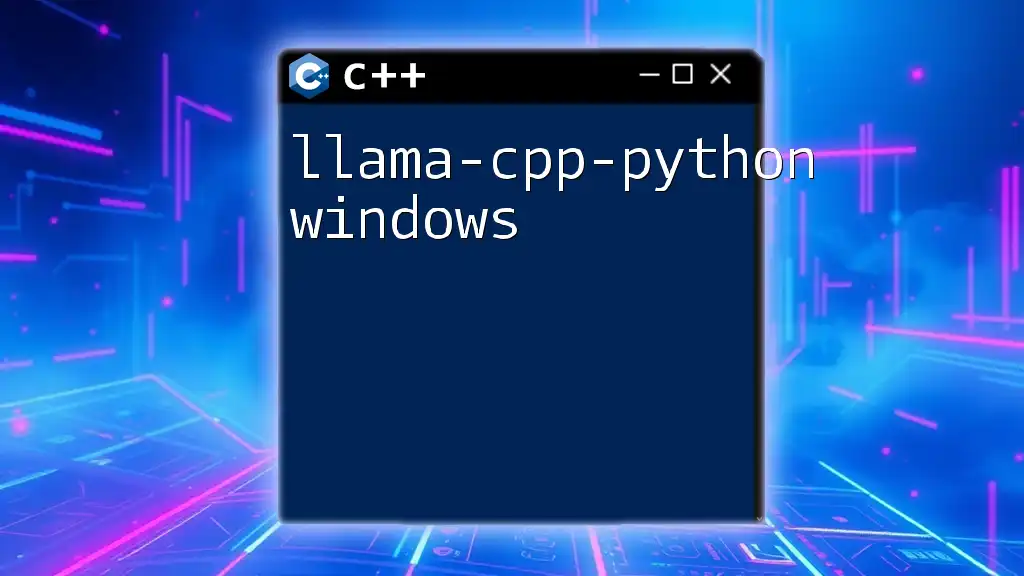
Setting Up Your Environment
Installing llama_cpp_python
To get started with llama_cpp_python, you will need to install it. Before installation, ensure you have the following prerequisites:
- Python (preferably version 3.6 or higher)
- A compatible C++ compiler (GCC for Linux, Clang for macOS, MSVC for Windows)
You can install the library via pip with the following command:
pip install llama-cpp-python
Verifying Installation
After installation is complete, it’s vital to verify that everything is working correctly. You can do this by executing a simple test script:
import llama_cpp_python
# Test if the module works
if llama_cpp_python:
print("llama_cpp_python is installed successfully!")
else:
print("Installation error.")
If you see the success message, then the installation was successful. If not, revisit the installation steps and check for common issues such as environment paths or version mismatches.
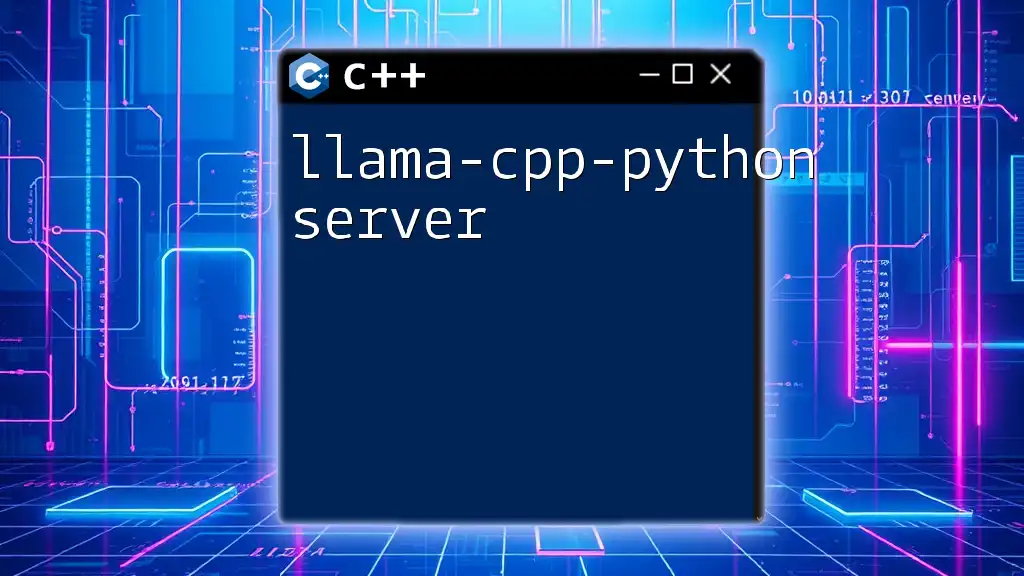
Core Concepts of llama_cpp_python
Understanding llama_cpp_python Structure
The library is structured to provide simple API access to C++ functionalities. It nests C++ code within Python functions, allowing flexible usage while maintaining performance.
- API Design: The API is designed to be intuitive, making it easier for Python developers to work with.
- Integration Capabilities: You can directly integrate C++ functions with existing Python codebases, improving productivity.
Key Features of llama_cpp_python
- Integration with Existing Python Code: You can call C++ functions seamlessly from Python, facilitating easy reuse of code.
- Performance Enhancements: Leveraging C++ core functions can lead to a reduction in computational time, making it ideal for large-scale computations.
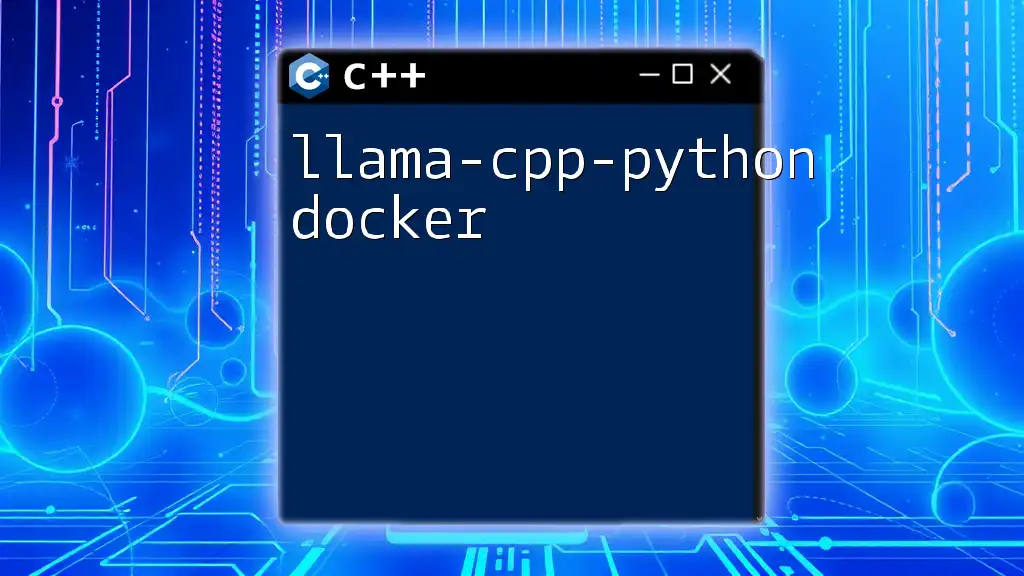
Getting Started with llama_cpp_python
Basic Usage Examples
To illustrate how to get started, let’s declare and initialize a simple variable in C++ and access it from Python:
// Example C++ code to be compiled into llama_cpp_python
extern "C" {
int add(int a, int b) {
return a + b;
}
}
You can then call this C++ function from your Python environment:
from llama_cpp_python import llama
# Load the C++ function
llama.load('path/to/compiled_cpp_code.so')
# Call the C++ function
result = llama.call('add', 5, 3)
print(f"The result is: {result}") # Output: The result is: 8
Advanced Examples
To delve deeper, let's explore working with data structures, specifically lists and dictionaries. Assume you have a C++ function that manipulates an array.
C++ Code:
#include <vector>
extern "C" {
std::vector<int> increment_all(std::vector<int> nums) {
for (auto &num : nums) {
num++;
}
return nums;
}
}
Python Code to call the above function:
array_data = [1, 2, 3, 4]
incremented_data = llama.call("increment_all", array_data)
print(f"Incremented array: {incremented_data}") # Output: Incremented array: [2, 3, 4, 5]
Parallel Processing with C++
To take advantage of C++'s parallel processing capabilities, you could implement it as follows:
C++ Code:
#include <omp.h>
#include <vector>
extern "C" {
void parallel_square(std::vector<int> &nums) {
#pragma omp parallel for
for (int i = 0; i < nums.size(); i++) {
nums[i] *= nums[i]; // Square each element
}
}
}
In Python:
numbers = [1, 2, 3, 4]
llama.call("parallel_square", numbers)
print(f"Squared numbers: {numbers}") # Output: Squared numbers: [1, 4, 9, 16]
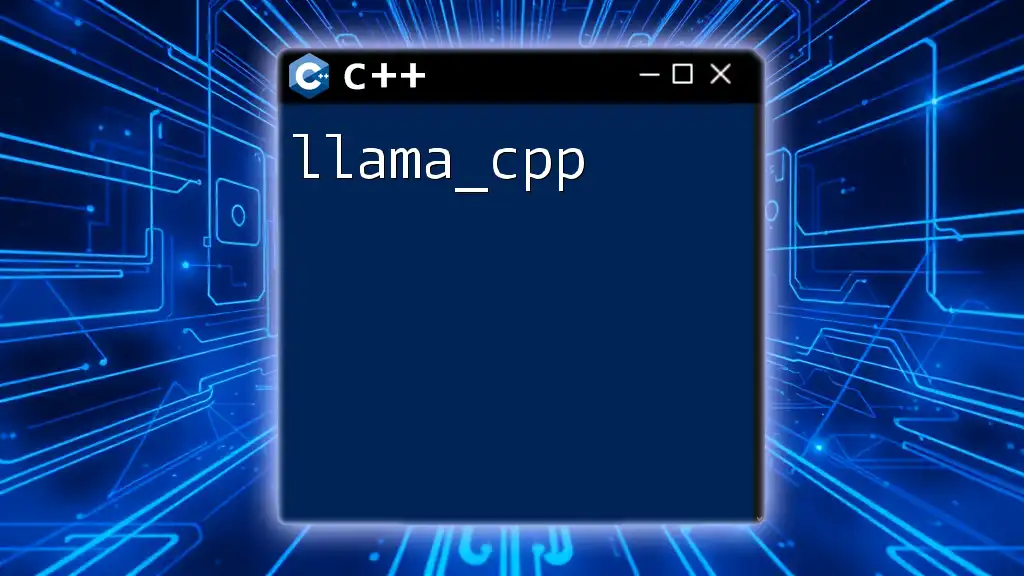
Best Practices When Using llama_cpp_python
Error Handling and Debugging
When working with llama_cpp_python, you might encounter several common errors, such as mismatched data types between Python and C++. To troubleshoot these efficiently, ensure that your C++ functions accept and return correct types. Utilize Python exception handling to catch errors gracefully.
Performance Optimization Techniques
Always be aware of bottlenecks in your code. You can use profiling tools to analyze performance. For memory management in C++, ensure that you free allocated memory when no longer in use to prevent memory leaks.
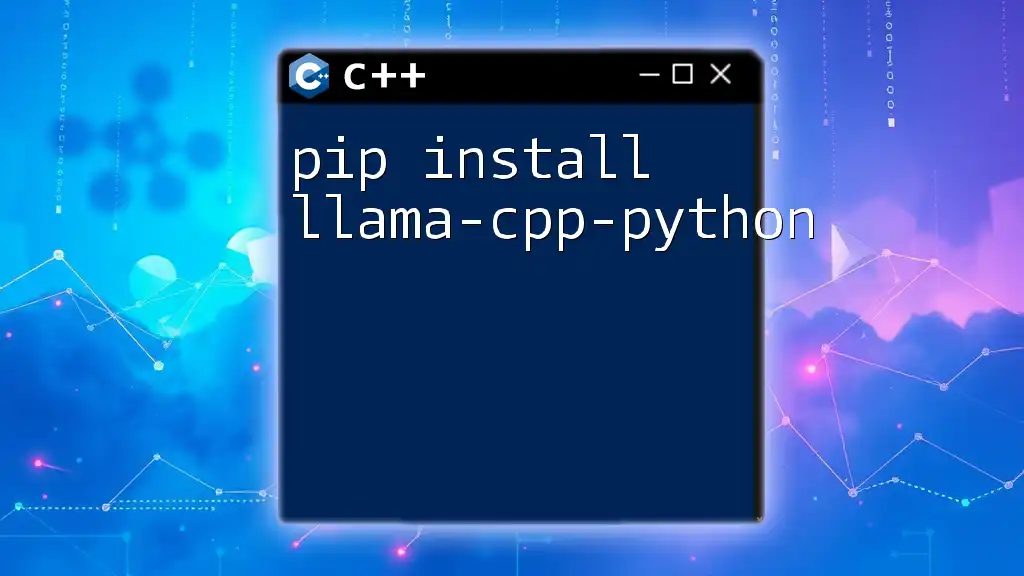
Real-World Applications of llama_cpp_python
Case Studies of Successful Implementations
llama_cpp_python has proven beneficial for numerous projects. For instance, in a data-intensive machine learning model, developers utilized this library to integrate C++-optimized algorithms, resulting in substantial speed improvements.
Potential Areas for Future Development
As technology evolves, llama_cpp_python can expand into areas such as GPU programming and deeper machine learning integrations, enabling developers to create even more powerful applications.
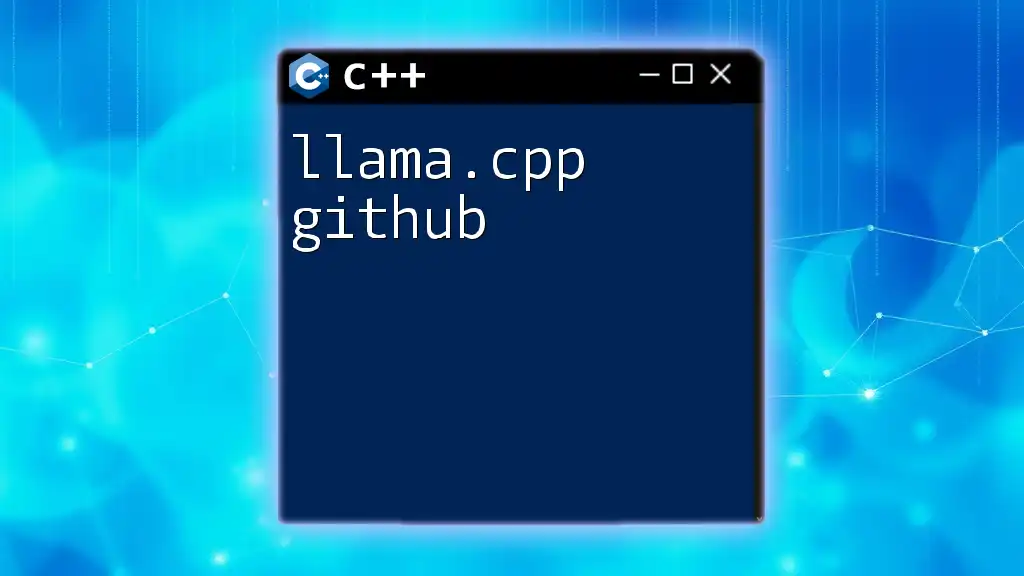
Comparison with Other Libraries
llama_cpp_python vs Other Python-C++ Libraries
When comparing llama_cpp_python with other libraries like pybind11 and Boost.Python, you will notice that llama_cpp_python offers a more user-friendly setup and faster integration time with fewer boilerplate requirements.
When to Choose llama_cpp_python?
Consider using this library when you require rapid performance improvements for specific tasks without the complexity of extensive C++ integration.
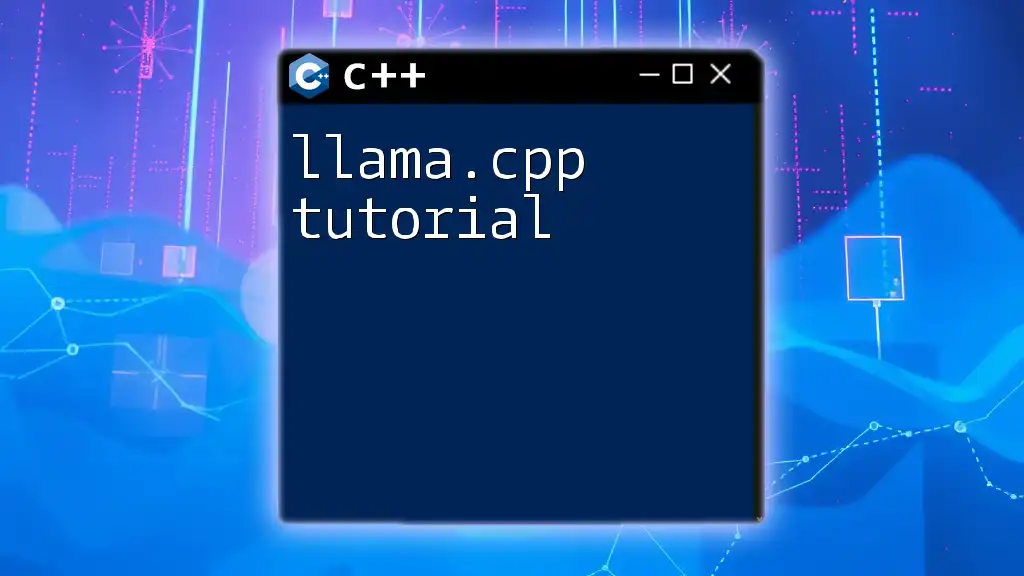
Conclusion
In summary, llama_cpp_python is a powerful tool that bridges the gap between C++ and Python, allowing developers to harness the strength of both languages efficiently. By following the guidelines and best practices discussed, you can begin leveraging this library in your projects today.
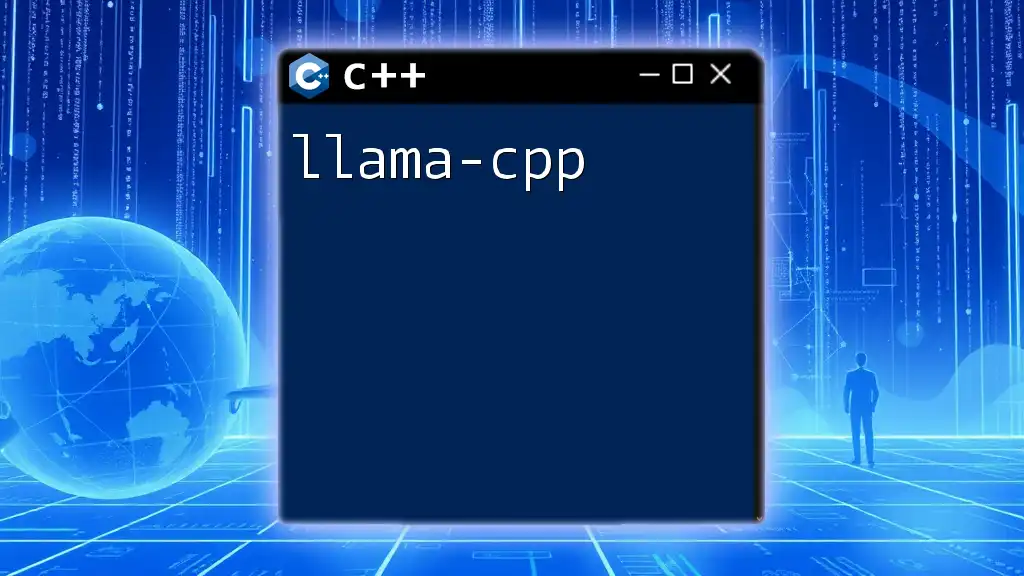
Additional Resources
Official Documentation
For further details and advanced usage, refer to the official llama_cpp_python documentation online.
Community Forums and Support
Join community forums for discussions, troubleshooting, and sharing project experiences related to llama_cpp_python.
Further Reading
Consider exploring recommended books and online courses that delve deeper into Python and C++, enriching your skill set significantly.