To install the `llama-cpp-python` package, which allows you to efficiently use the LLaMA model in Python, you can run the following command:
pip install llama-cpp-python
Understanding llama-cpp-python
What is llama-cpp-python?
Llama-cpp-python is a powerful library designed for users who want to leverage the capabilities of LLaMA (Language Model with Large Attention Mechanisms) in Python. This library provides a seamless interface to integrate LLaMA's generative capabilities into Python applications, making it easy for developers to implement advanced AI functionalities without diving deep into complex C++ code.
Comparatively, other libraries might offer generative models, but llama-cpp-python tightly integrates the efficiency of C++ with user-friendly Python environments, allowing for high performance in tasks involving natural language processing, text generation, and more.
Why Use llama-cpp-python?
The benefits of choosing llama-cpp-python are numerous:
- Performance: Built on top of efficient C++ code, it ensures quicker operations and lower latency when generating text.
- Flexibility: Easily tweak parameters to get the desired output—perfect for experiments in AI and machine learning.
- Community Support: With an engaging community around the library, users can easily find help, examples, and best practices.
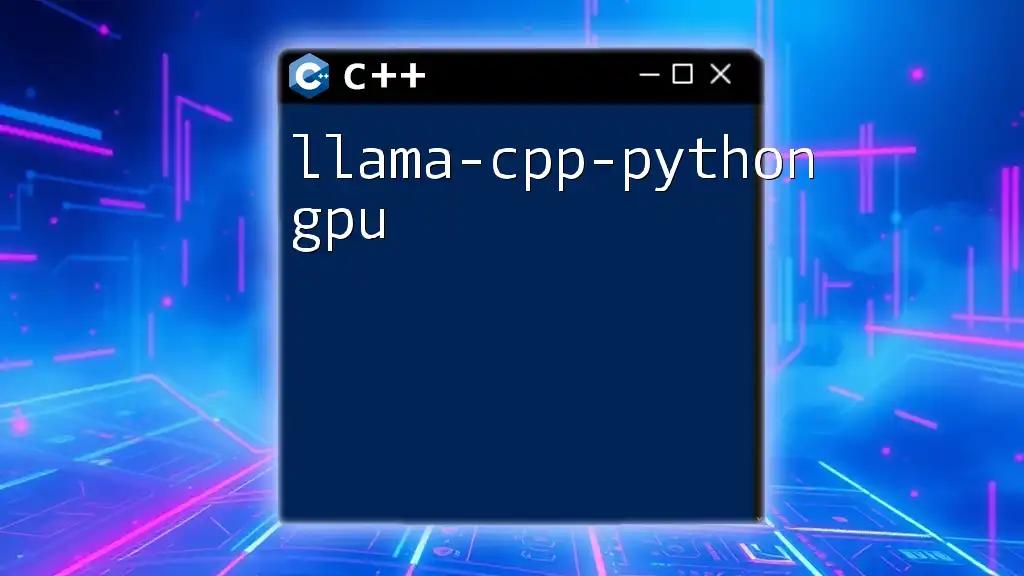
Prerequisites
Software Requirements
Before diving into the installation process, check that you have the following:
- Python Installation: Ensure Python is installed on your system. Llama-cpp-python supports Python 3.6 and above.
- Additional Libraries: Depending on your environment, other libraries might be required, such as pip or setuptools for easy installation.
Setting Up Your Environment
Creating a dedicated virtual environment for your project is a good practice. It allows you to isolate dependencies and avoid conflicts. You can set up your environment as follows:
python -m venv myenv
source myenv/bin/activate # On Windows use `myenv\Scripts\activate`
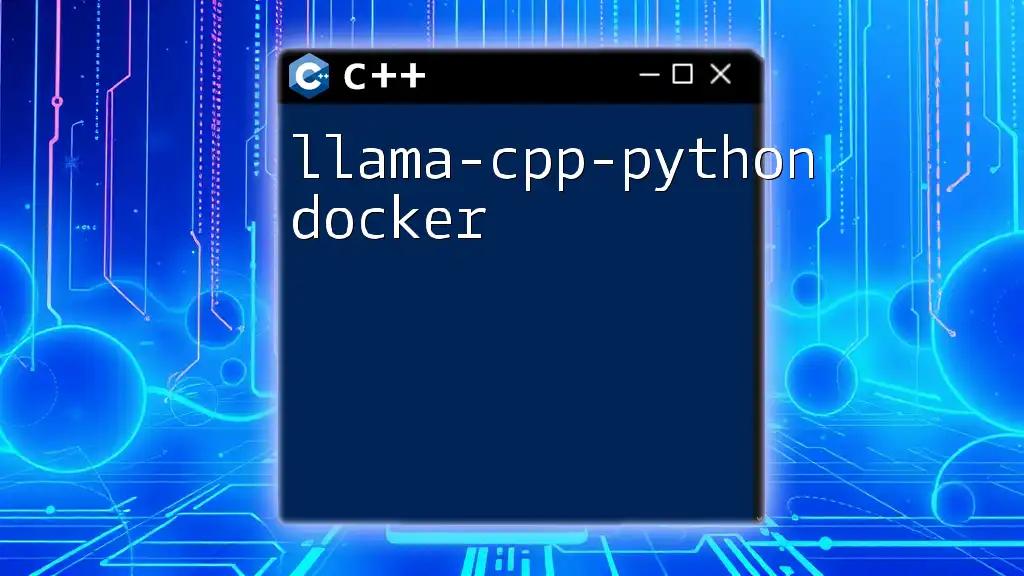
Installation Guide
How to Install llama-cpp-python
The installation of llama-cpp-python is straightforward. With pip being the go-to package manager for Python, running the following command in your terminal or command prompt will get you started:
pip install llama-cpp-python
This command does the heavy lifting by fetching the latest version of llama-cpp-python from the Python Package Index (PyPI) and installing it alongside any required dependencies.
Troubleshooting Common Installation Issues
Even though the installation process is generally smooth, users may face some common errors:
- Dependency Issues: If you encounter errors related to missing dependencies, ensure all prerequisites are met.
- Version Conflicts: Sometimes, existing installations may conflict with the required packages. You can use the command below to upgrade any outdated packages, which can often resolve these issues:
pip install --upgrade llama-cpp-python

Basic Usage of llama-cpp-python
Importing the Library
Once the installation is successful, you can start using llama-cpp-python in your Python scripts. Importing is simple:
import llama_cpp as llm
First Steps with Llama
After importing the library, creating an instance of Llama is your next step:
llama = llm.Llama()
print(llama.generate("Hello, Llama!"))
This snippet demonstrates how to invoke the library's powerful text generation capabilities with minimal code.
Exploring Functions and Features
Generating Text
Generating text using llama-cpp-python is intuitive. You can easily pass in a prompt and set parameters to customize the output. Here's a brief example:
response = llama.generate("Once upon a time", max_length=50)
print(response)
This example generates a response based on the prompt provided, allowing a maximum length of 50 tokens in return.
Fine-Tuning and Customization
Fine-tuning generated text to suit specific needs is one of the library's strengths. You can adjust parameters such as `temperature` and `top_k` to refine the output. Here's an improved version of the generation command:
response = llama.generate("Once upon a time", max_length=50, temperature=0.7)
In this snippet, the `temperature` parameter controls the randomness of the output. Lower values generate more predictable results, while higher values permit more creativity in the text generation.
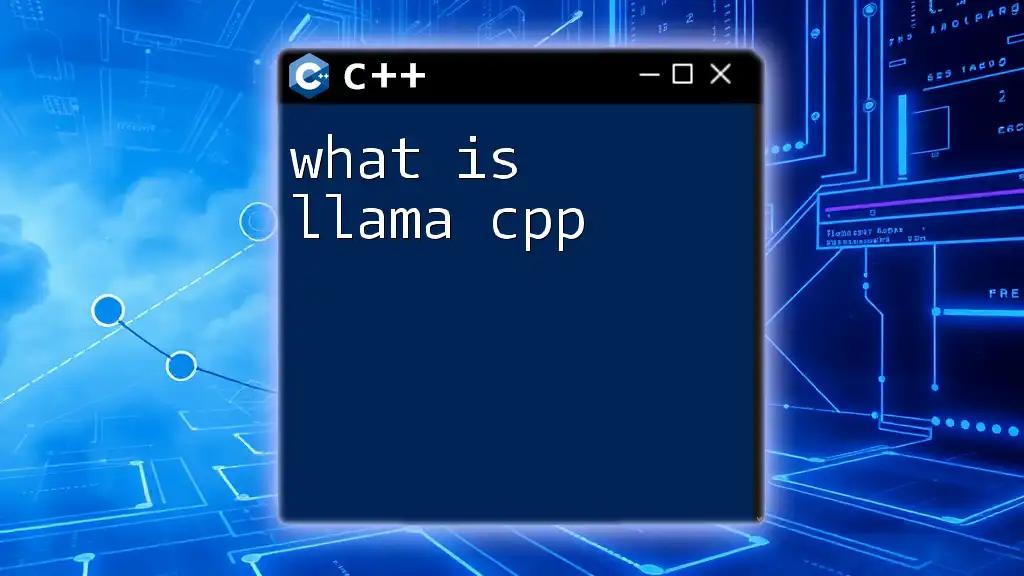
Advanced Features of llama-cpp-python
Integration with Other Libraries
Llama-cpp-python can be effectively combined with other popular Python libraries like NumPy and Pandas. This allows developers to enhance data processing before or after utilizing llama-cpp-python for text generation. For example, you might preprocess a dataset using Pandas and then pass results to Llama for natural language generation tasks.
Using llama-cpp-python in a Web Application
Llama-cpp-python can also be integrated into a web application. Below is a simple example of how you might use Flask to create an API endpoint for generating text.
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/generate', methods=['POST'])
def generate():
input_text = request.json['text']
output = llama.generate(input_text)
return jsonify({"output": output})
In this example, the `/generate` endpoint receives JSON input and returns generated text based on the input query, showcasing the ease of integrating llama-cpp-python into broader applications.
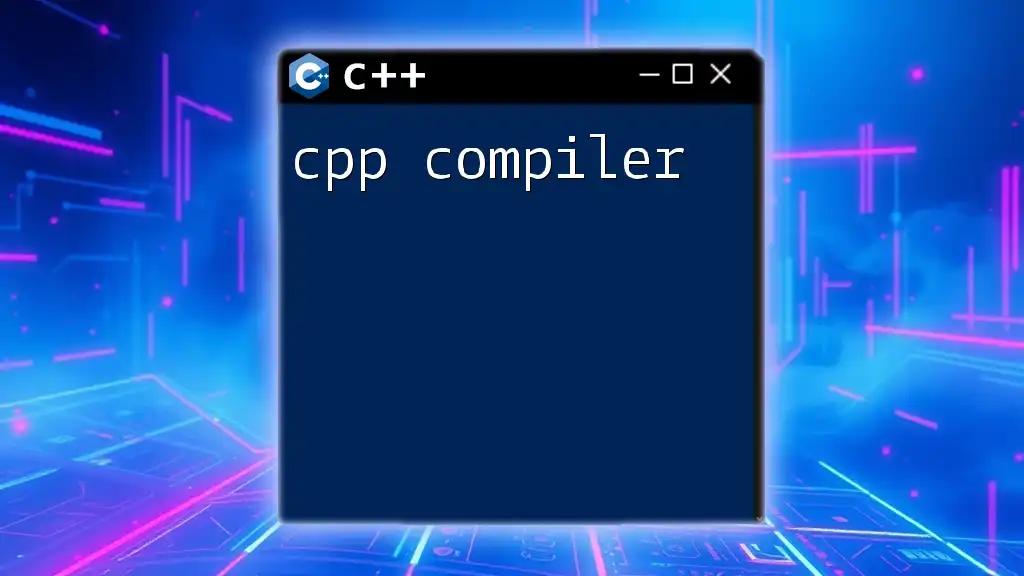
Best Practices
Optimization Tips
To maximize performance with llama-cpp-python, consider the following best practices:
- Regularly monitor resource usage when executing high-demand tasks.
- Experiment with multi-threading or async calls for concurrent requests in a web application to handle multiple users efficiently.
Keeping Up with Updates
It’s essential to keep llama-cpp-python up-to-date. Regular updates may introduce new features, improvements, or security patches. You can check for outdated packages with the following command:
pip list -o
Keeping a pulse on the library’s updates ensures that you leverage all available functionalities and improvements.
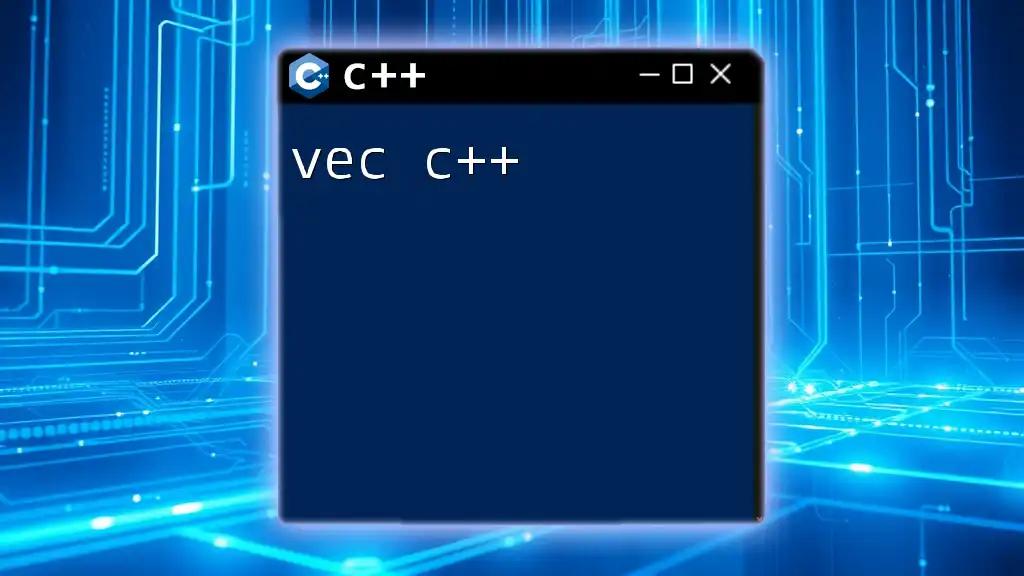
Conclusion
Llama-cpp-python exemplifies how powerful libraries can simplify the integration of advanced AI capabilities into Python applications. With its robust features and performance optimizations, this library can significantly enhance your projects. Experiment with the provided examples, and don’t hesitate to engage with the community for further insights and contributions.
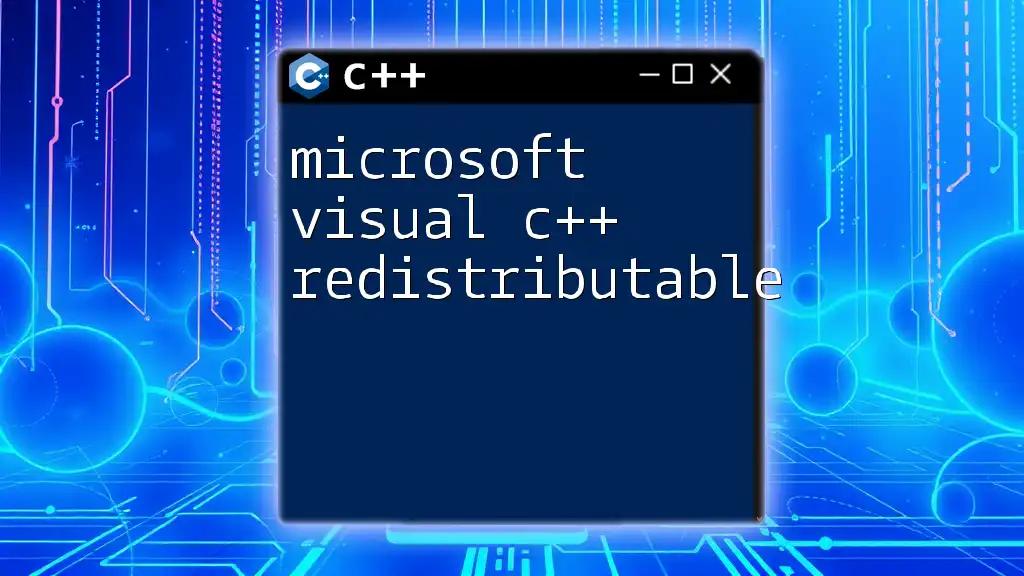
Additional Resources
For further details on using llama-cpp-python, refer to its [official documentation](insert-link-here). You can also connect with community forums, check the [GitHub repository](insert-link-here), and look for additional tutorials that complement your learning journey with llama-cpp-python.