Visual C++ is an integrated development environment (IDE) from Microsoft that allows developers to create applications in C++ with powerful tools and features tailored for Windows development.
Here's a simple example of a "Hello, World!" program in Visual C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Introduction to Visual C++
What is Visual C++?
Visual C++ is a powerful Integrated Development Environment (IDE) developed by Microsoft, specifically designed for C++ programming. It combines a series of programming tools with an easy-to-use graphical interface that simplifies the process of writing, debugging, and compiling C++ applications. With robust features tailored for Windows development, Visual C++ plays a critical role in the software development lifecycle, empowering both new and experienced programmers.
History and Evolution
Visual C++ has undergone numerous transformations since its inception in the early 1990s. With each iteration, Microsoft has introduced significant updates, enhancing functionality and performance. Starting as a compiler and IDE for C and C++, it evolved to support a rich set of libraries, allowing developers to craft everything from console applications to sophisticated GUI-based software. Notable enhancements include tighter integration with Windows SDKs, advanced debugging capabilities, and improved code optimization techniques.
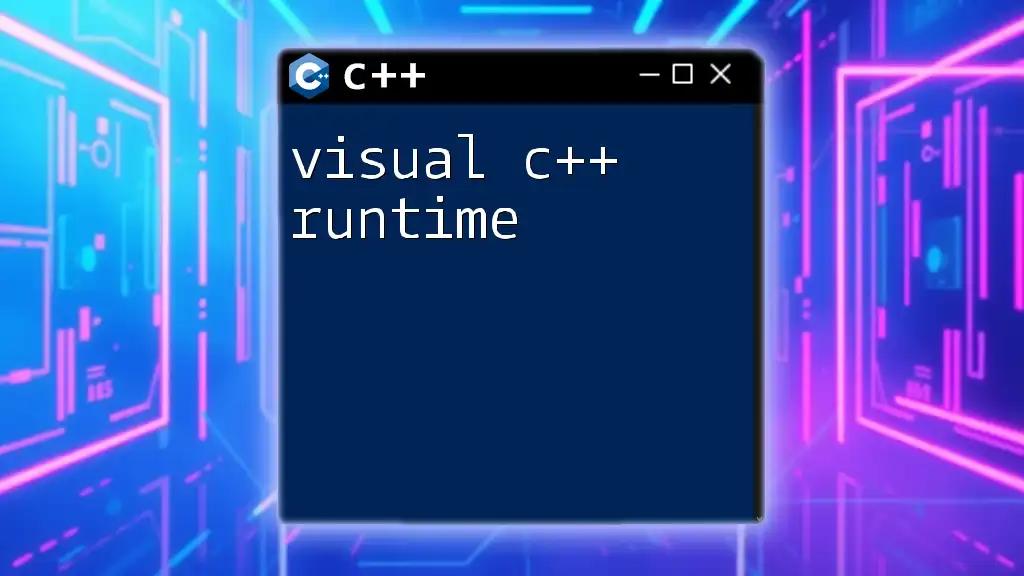
Setting Up Visual C++
System Requirements
To ensure a smooth experience with Visual C++, make sure your system meets the following prerequisites:
- Modern Windows operating system (Windows 10 or later)
- Minimum of 4 GB of RAM (8 GB or more recommended)
- At least 1 GB of available hard disk space
- Support for x64-based processor
Installation Process
- Download: Navigate to the official Microsoft Visual Studio website. Choose the version of Visual Studio that includes Visual C++ (either the Community, Professional, or Enterprise editions).
- Run the Installer: Launch the downloaded installer and choose the components you wish to install. For C++ development, ensure that the “Desktop development with C++” workload is checked.
- Configuration: Customize any additional settings according to your preferences and begin the installation.
Once installed, launch Visual C++ to explore the interface and features available.
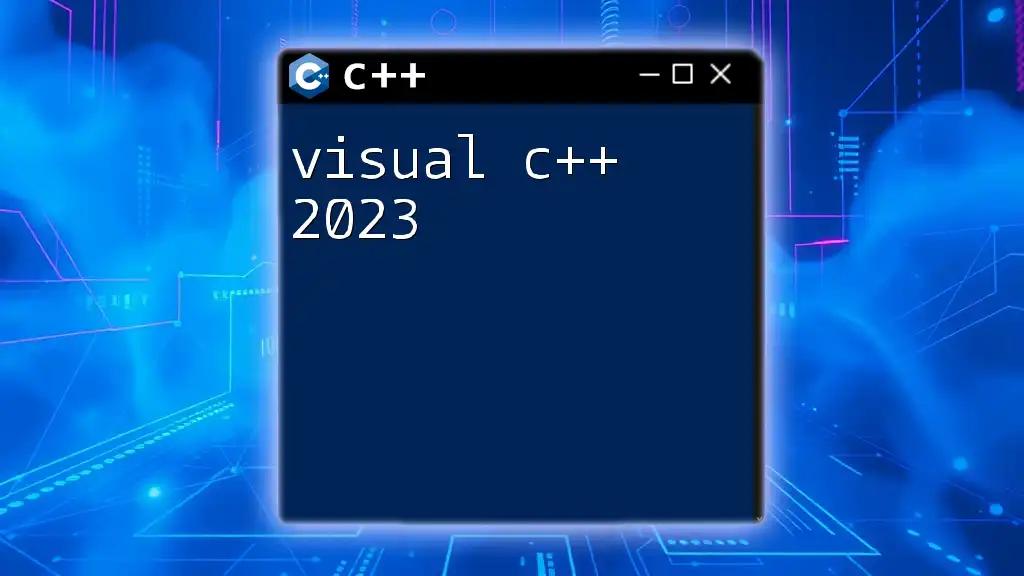
Navigating the Visual C++ Interface
Overview of the User Interface
Upon launching Visual C++, you'll encounter a user-friendly interface consisting of several key components:
- Menu Bar: Contains menus for file operations, editing, building projects, debugging, and accessing tools.
- Toolbars: Quick-access buttons for common actions, enhancing your productivity.
- Workspace: Where you manage your projects and files, displaying a hierarchical view of your project structure.
- Output Window: Shows error messages and compiler output, invaluable during debugging.
Customizing the IDE
Visual C++ provides customization options to tailor the environment to your workflow. You can:
- Adjust the layout by dragging and docking tools to your preferred positions.
- Customize themes to suit your aesthetic or to reduce eye strain during long coding sessions.
- Set user preferences that align with your coding style, such as indentation and formatting rules.
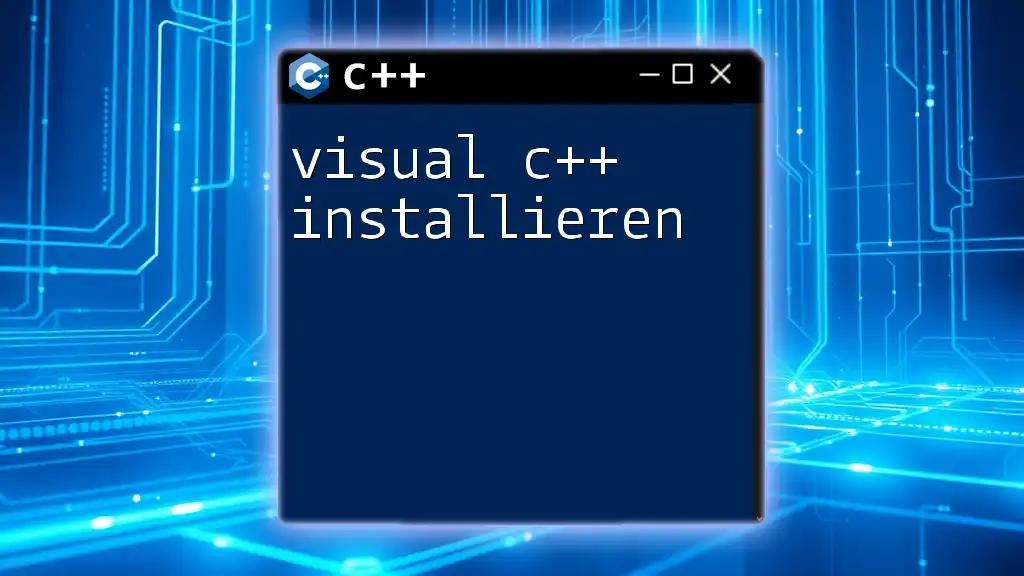
Creating Your First Visual C++ Project
Setting Up a New Project
- To initiate a new project, click on File > New > Project.
- Choose from various project types such as Console Application, Windows Application, or DLL. Each type serves different purposes, depending on the nature of your software.
Building Your First Application
Let’s create a simple “Hello, World!” program to familiarize yourself with the environment.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This basic program includes the necessary header `<iostream>`, defines the `main` function, and uses `std::cout` to print a message to the console.
Compiling and Running Your Program
To compile your application, go to the menu and select Build > Build Solution. If there are no errors, run your program by selecting Debug > Start Without Debugging (or pressing `Ctrl + F5`). If you encounter any compilation errors, review the Output Window for messages that can guide you in troubleshooting.
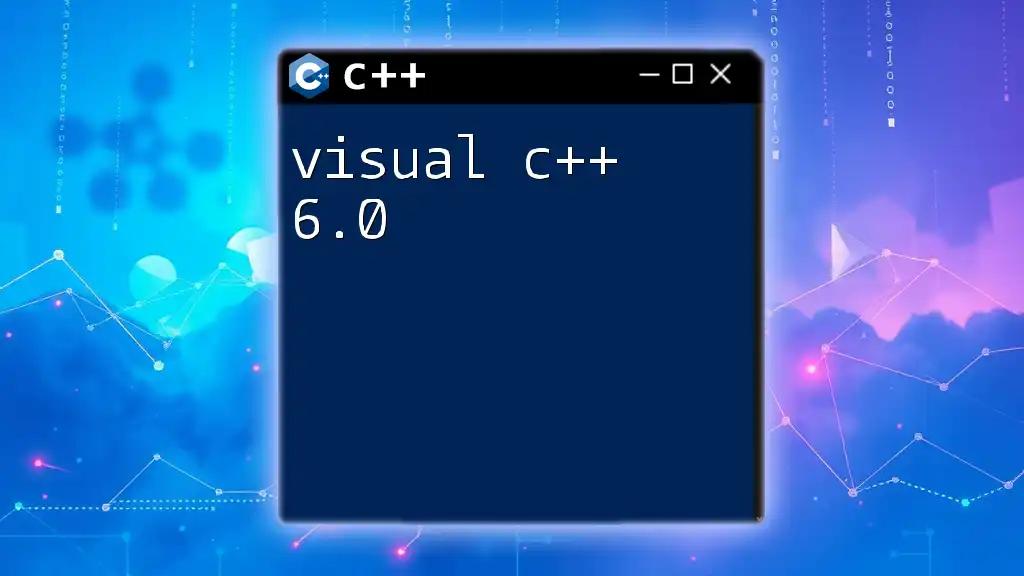
Understanding the Visual C++ Compiler
Overview of the Compiler
The Visual C++ compiler plays a crucial role in converting your C++ code into executable programs. When you hit the build button, the compiler analyzes your code for syntax errors, optimizes it for performance, and finally translates it into machine code that the computer can understand.
Optimization Options
Visual C++ offers several optimization options during compilation:
- Debug Mode: In this mode, the compiler preserves more debug information and includes checks, which is useful during development.
- Release Mode: For production builds, this mode optimizes the code for speed and size, removing unnecessary debug information.
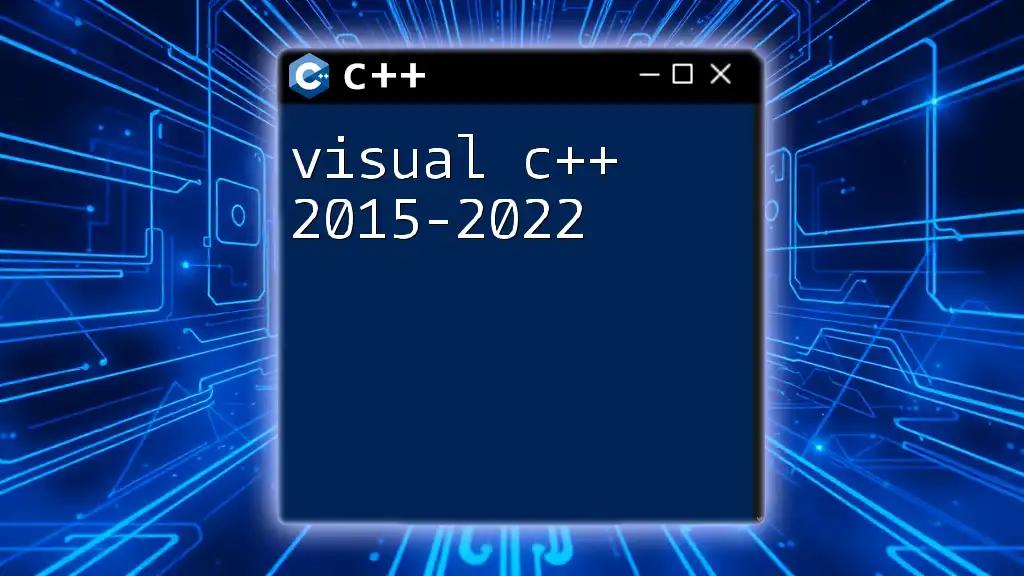
Debugging in Visual C++
Built-in Debugging Tools
Visual C++ comes equipped with powerful debugging features that simplify the problem-solving process. The debugger allows you to:
- Set breakpoints to pause execution at specified lines of code.
- Inspect variables and data structures, letting you monitor changes in real-time.
Debugging Techniques
Effective debugging is essential for writing robust applications. Here’s how to utilize debugging tools:
- Setting Breakpoints: Click on the left margin next to a line of code to insert a breakpoint. When the program runs, it will pause at this point, allowing you to analyze the state of the application.
- Step Through Code: Use the Step Over or Step Into commands to execute code line by line. This is beneficial for tracking down where things go awry.
For example, if you have a loop iterating through array elements that isn’t functioning as expected, stepping through the loop helps you see the changes in each iteration.
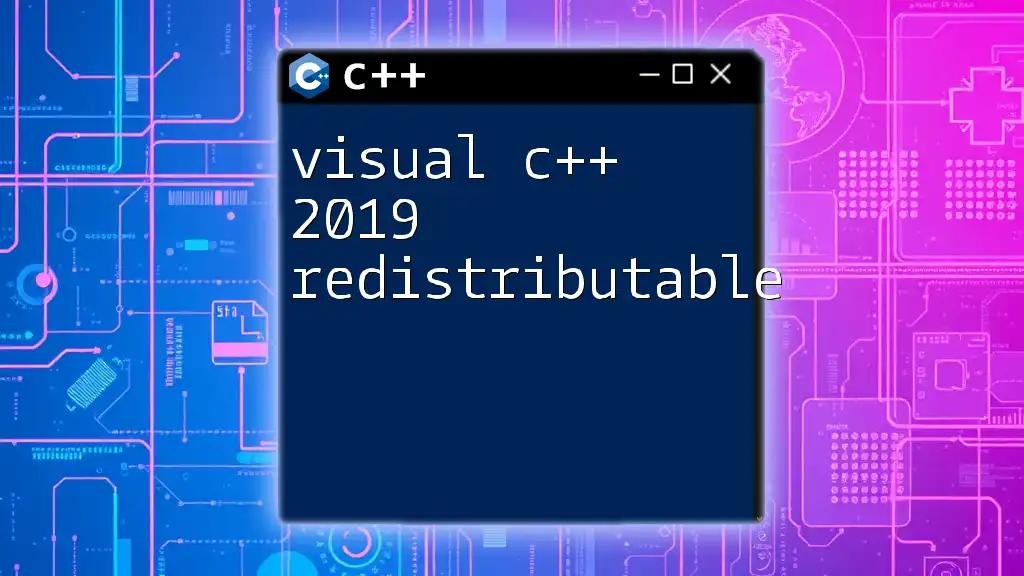
Advanced Features
Using Visual C++ Libraries
Visual C++ supports an extensive collection of libraries. Using standard libraries like `<vector>` and `<string>` enhances your programming capabilities significantly. Here’s an example of using a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
IntelliSense and Auto-Completion
One of the standout features of Visual C++ is IntelliSense, which offers real-time code suggestions as you type. This not only expedites the coding process but also helps in minimizing errors. For instance, if you start typing `std::`, IntelliSense will display a list of available functions and classes within the standard library, guiding you as you code.
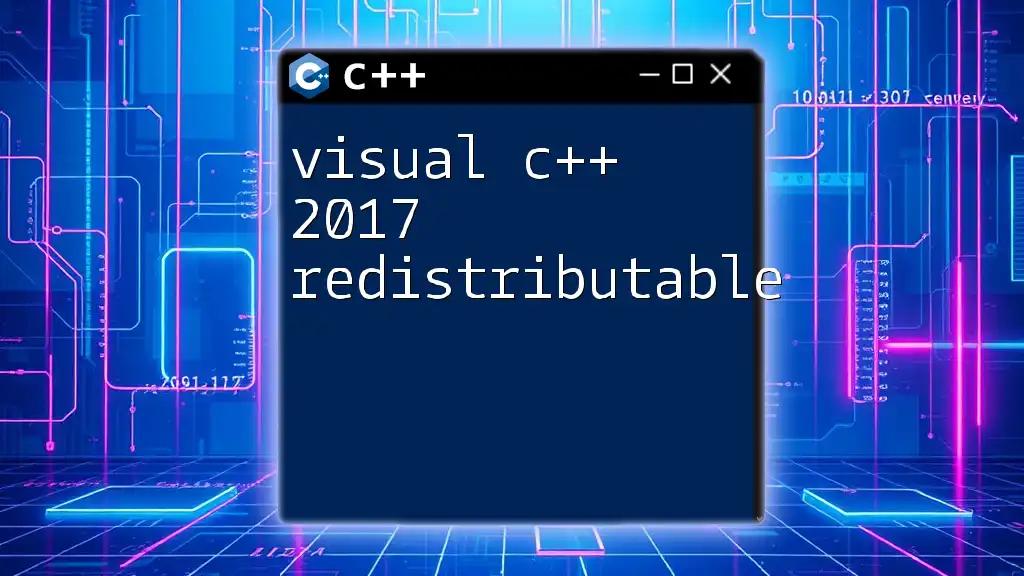
Integrating with Other Tools
Version Control Systems
Visual C++ can be integrated seamlessly with version control systems like Git. Adding version control to your development process is essential for managing changes and collaborating with others. You can:
- Clone repositories directly within Visual C++.
- Commit changes and manage branches through the built-in source control interface.
Building and Deploying Applications
When it's time to share your application, Visual C++ provides robust build configurations. This includes:
- Debug configurations for development and troubleshooting.
- Release configurations for final output designed for end-users.
Choose the appropriate configuration according to your needs, ensuring your application performs optimally.
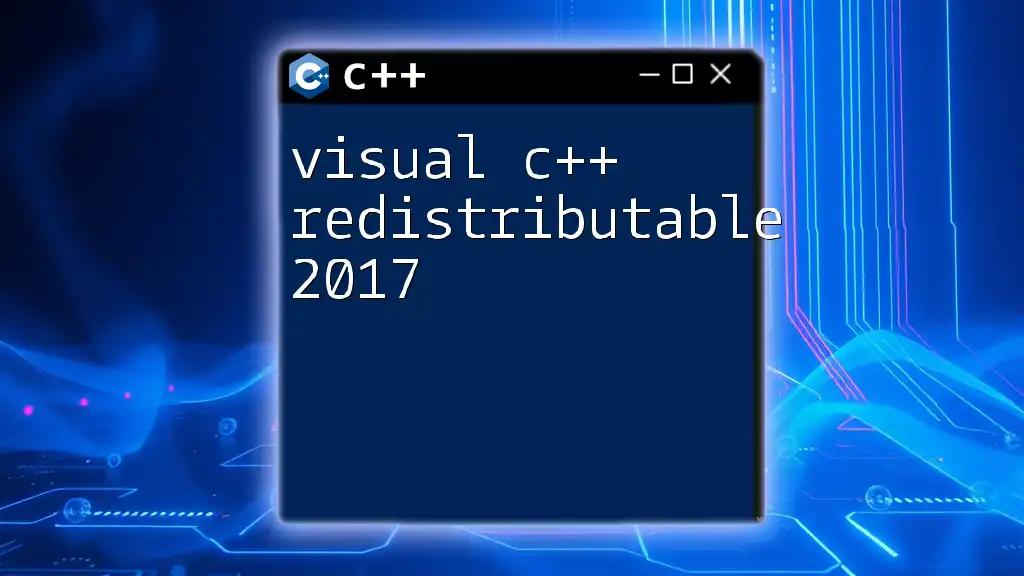
Best Practices for Visual C++
Code Organization Techniques
Keeping your project organized is crucial for maintainability. Adopt a structure where your code files and resources are organized into separate folders. Use meaningful naming conventions to make your code readable and understandable.
Writing Clean and Efficient Code
Strive to write code that is not only functional but also clean and efficient. Avoid redundancies, employ comments where necessary, and utilize functions and classes to segment functionalities. This practice can greatly reduce complexity and improve code readability.

Resources and Community Support
Learning Resources
To deepen your understanding of Visual C++, consider the following:
- Books such as "C++ Primer" and "Effective C++."
- Online platforms offering courses, including Coursera, Udemy, and official Microsoft documentation.
Community and Forums
Engage with other developers to enhance your learning experience. Platforms like Stack Overflow, Reddit’s r/cpp, and Microsoft’s own forums can provide valuable insights, tips, and support.
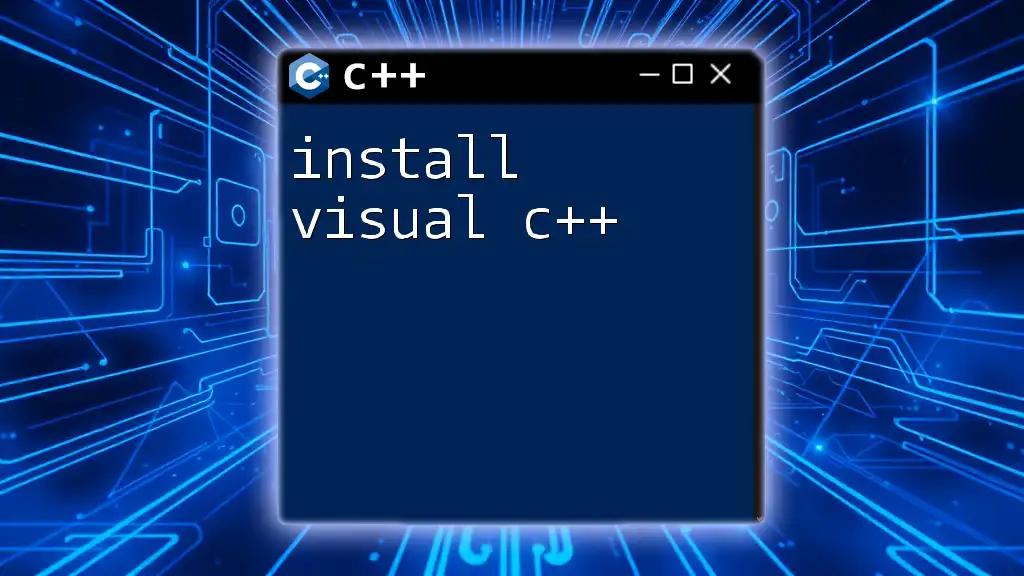
Conclusion
Visual C++ is a powerful tool for C++ development that offers extensive functionality, from writing and debugging code to optimizing it for final deployment. By familiarizing yourself with its features and best practices, you can significantly improve your programming skills and productivity. Embrace the power of Visual C++, and enjoy the journey of software development!