Visual C++ 2015-2022 is a suite of Microsoft IDEs designed for developing C++ applications, offering enhanced support for modern C++ standards, libraries, and improved performance features.
Here’s an example of a simple "Hello, World!" program in Visual C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Visual C++?
Visual C++ is an Integrated Development Environment (IDE) developed by Microsoft for C and C++ programming languages. It provides robust tools for coding, debugging, and compiling applications, enabling developers to efficiently build software across various platforms. Visual C++ 2015-2022 has evolved significantly, providing enhanced performance, better compliance with modern C++ standards, and features aimed at increasing developer productivity.
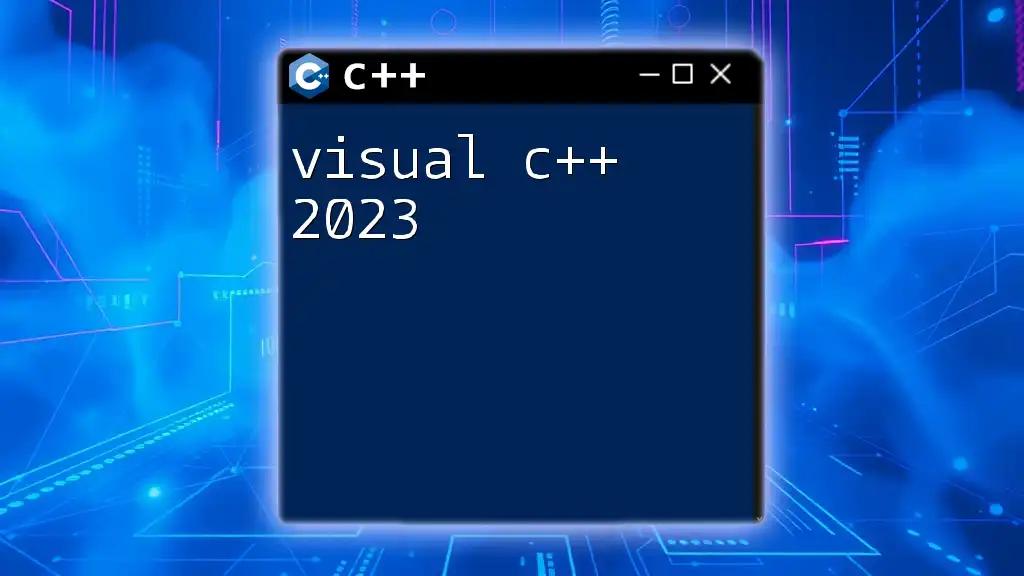
The Evolution from C++ 2015 to 2022
The landscape of C++ programming has undergone a notable transformation between 2015 and 2022. Each version brought specific improvements that cater to modern programming needs. Visual C++ 2019 and Visual C++ 2022 include support for C++17 functionalities, introducing manageable syntax and powerful new features, while maintaining backward compatibility with previous standards.
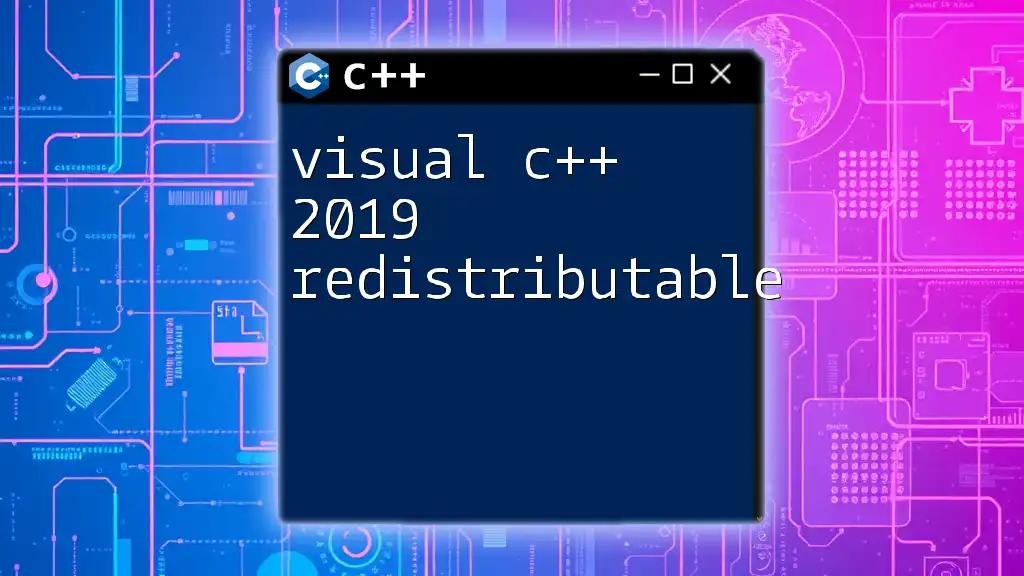
Key Features of Visual C++ 2015-2022
Compiler Enhancements
One of the standout benefits of Visual C++ 2015-2022 is the compiler improvements. The compiler streamlines optimization techniques and expands its capabilities in line with newer C++ standards.
For instance, the enhancements made in the compiler allow for more effective error detection and quicker compilation. A highlighted feature is the enhanced C++11, C++14, and parts of C++17 compliance, which make the development experience smoother and less error-prone. This means developers can leverage new syntax and language features, allowing for cleaner and more maintainable code.
Here's an example of how C++11 features can be employed:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {4, 1, 2, 3};
std::sort(vec.begin(), vec.end());
for (const auto& val : vec) {
std::cout << val << " ";
}
return 0;
}
Performance Improvements
Visual C++ 2015-2022 introduces significant runtime performance improvements that greatly enhance the efficiency of applications. Developers will notice faster execution times due to optimizations in compiled code. Benchmarks comparing older compilers will often show that Visual C++ 2022 applications run significantly quicker, which can be crucial for performance-sensitive applications, such as games and real-time processing systems.
Support for Modern C++ Standards
With Visual C++ 2015-2022, support for modern C++ standards has been a focal point of Microsoft's updates. This includes features from C++11, C++14, and C++17, such as:
- Auto Type Deduction: Simplifying variable declarations to improve code readability.
- Lambda Expressions: Enabling concise function definitions that can be passed as arguments.
Example Code Snippet for Lambda Expressions:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
std::for_each(nums.begin(), nums.end(), [](int n) {
std::cout << n * 2 << " ";
});
return 0;
}
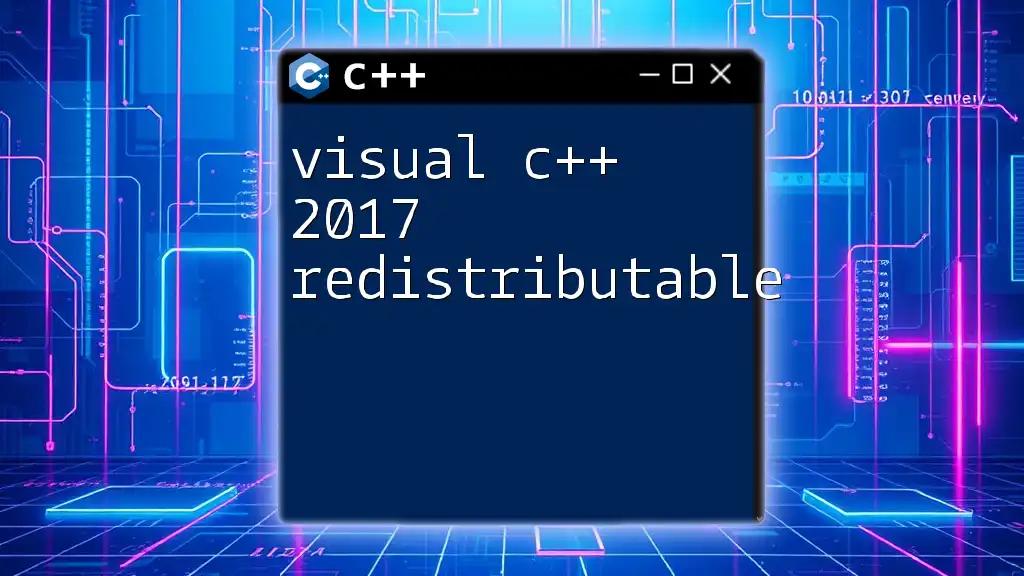
Setup and Installation
System Requirements
Before installation, it’s important to be aware of the hardware and software prerequisites:
- Windows 7 or later
- A minimum of 2 GB RAM (4 GB recommended)
- At least 6 GB of available disk space
Installation Process
Installing Visual C++ can be accomplished through the Visual Studio installer. Follow these steps to ensure a seamless installation:
- Download the Visual Studio Installer from the official Microsoft website.
- Run the installer and select C++ development workload.
- Proceed through the installation process, ensuring all necessary components are included.
- Launch Visual Studio and create or open projects as needed.
Ensure that you follow any on-screen prompts for additional setups.
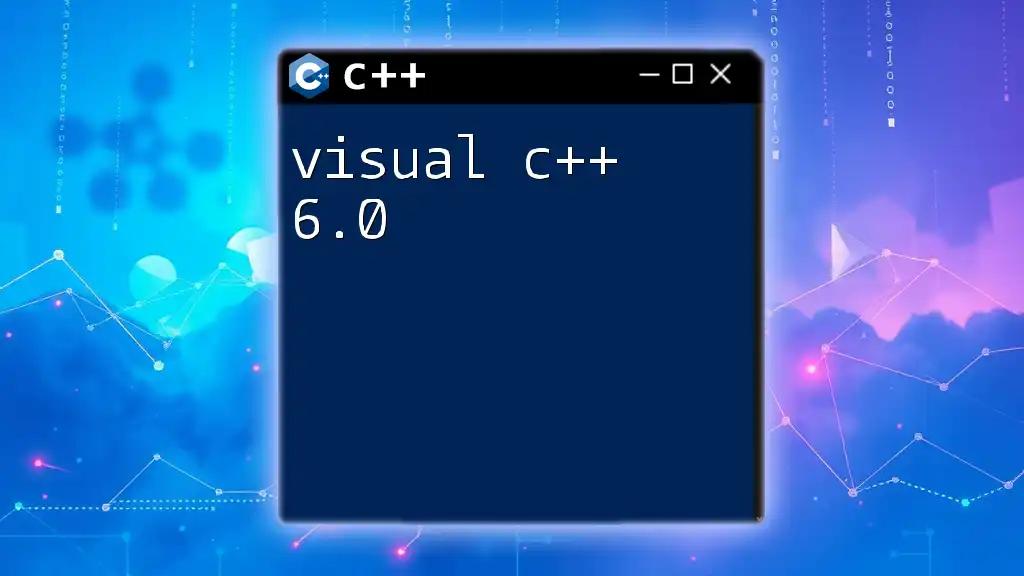
User Interface and Navigating the IDE
Overview of the Visual Studio GUI
The user interface of Visual Studio is user-friendly and organized. On launching the IDE, you are greeted by a welcome screen that allows access to existing projects or the creation of new ones. The main window contains various toolbars, a solution explorer, and a main editor area for code writing.
Creating a New Project
Starting your first Visual C++ project is simple. Here’s a step-by-step guide:
- From the main menu, select File > New > Project.
- Choose Visual C++ from the Installed Templates.
- Select a project type, such as Console Application, and click Next.
- Name your project and click Create.
Here’s a basic example of a simple "Hello, World!" application that you can create:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
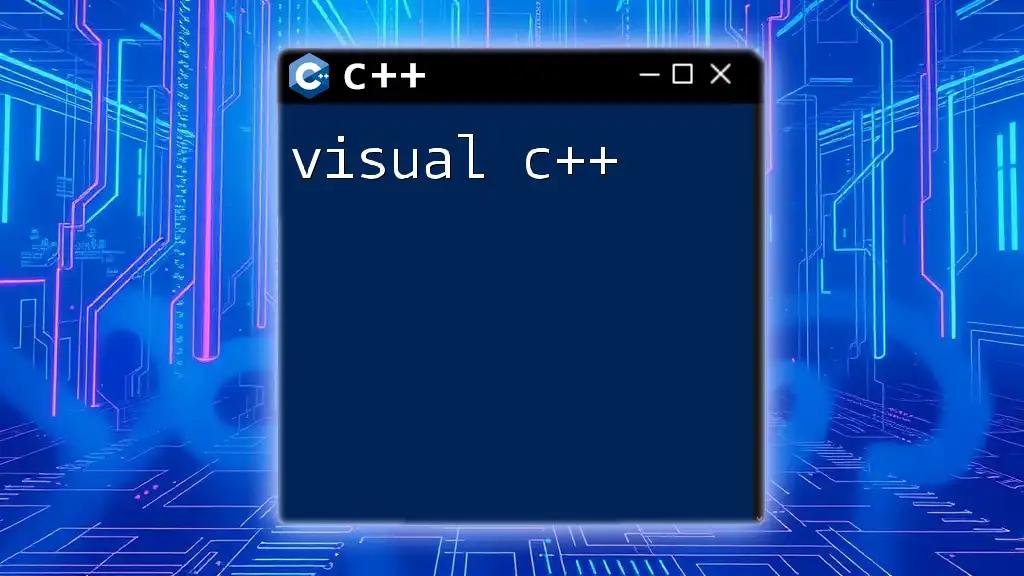
Coding Features and Best Practices
Code Editing Features
Visual C++ comes equipped with IntelliSense, offering real-time code suggestions and autocompletion that enhances programming efficiency. It helps minimize typographical errors and suggests variable types, function signatures, and available methods.
Debugging Tools
Effective debugging tools play a crucial role in software development. Visual C++ features allow you to set breakpoints, monitor variables, and step through code. This enables developers to identify issues quickly and ensure their code runs as intended.
Best Practices for Writing Clean C++ Code
Writing clean and maintainable code involves adhering to general best practices, including:
- Consistent Naming Conventions: Adopt a naming standard for variables and functions, ensuring clarity.
- Commenting Code: Utilize comments judiciously to explain complex logic but avoid redundancy.
- Organizing Code: Break down code into manageable functions and classes to promote reusability and readability.
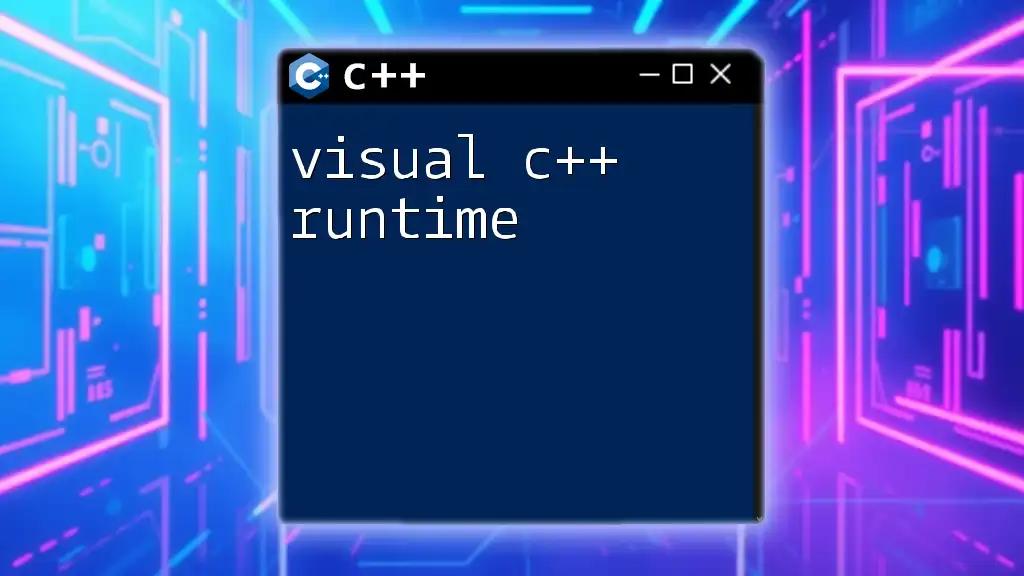
Project and Resource Management
Managing Projects and Solutions
Visual C++ allows you to manage multiple projects within a solution effectively. This helps in organizing large applications into smaller, manageable components. Make sure to familiarize yourself with the Solution Explorer, where you can add, remove, and navigators between projects swiftly.
Using Libraries and Frameworks
Integrating external libraries can expand your application’s capabilities. Visual C++ makes it easy to add libraries via:
- Right-clicking on your project in the Solution Explorer.
- Selecting Properties and navigating to C/C++ > General.
- Adding additional include directories or libraries as needed.
Example: Adding a Third-Party Library
Say you want to add the Boost library. You would include the necessary headers in your code while ensuring the library files are referenced correctly in your project settings.
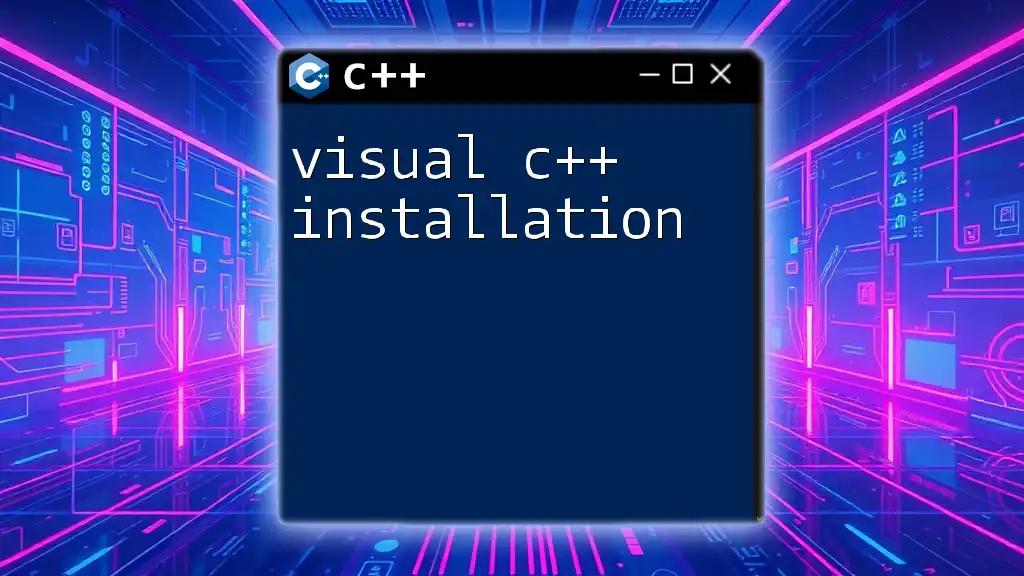
Real-World Applications of Visual C++ 2015-2022
Industry Use Cases
Visual C++ is a preferred choice in various industries due to its power and performance. For gaming, C++ provides the speed required for graphics rendering engines. In systems programming, it is invaluable due to its low-level access and efficiency.
Open Source Contributions
Visual C++ has a vibrant open-source community. Contributing to projects can be a rewarding experience. Consider checking repositories on platforms like GitHub, where numerous open-source projects welcome contributions from developers familiar with Visual C++.
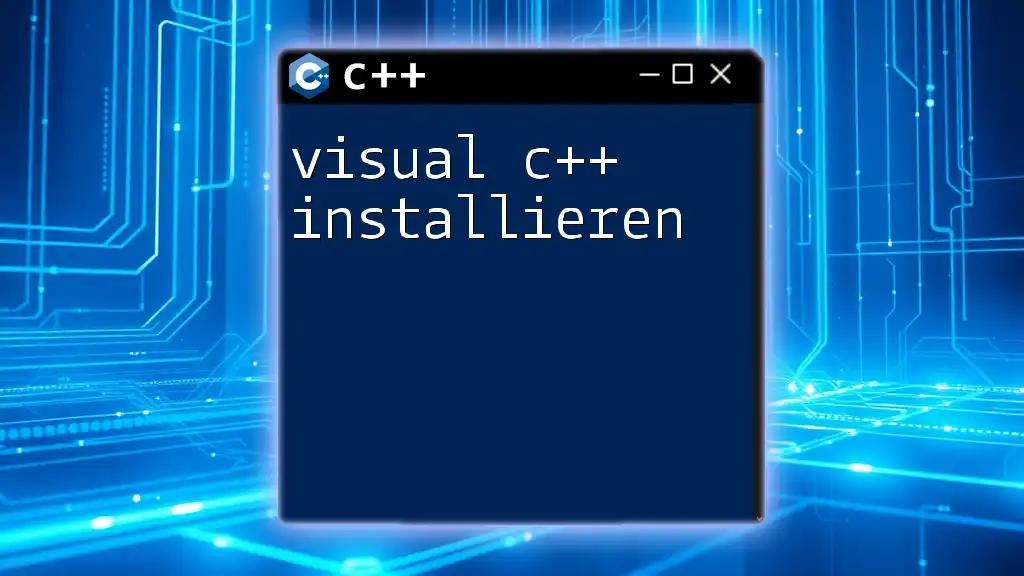
Troubleshooting Common Issues
Common Errors and Their Solutions
Developers may encounter compilation or runtime errors while using Visual C++. Common issues include misconfigured project settings or syntax errors.
Suppose you receive an error related to undefined reference. This often indicates that your project settings need to link to an external library properly. Review your configuration settings and ensure that all paths are set correctly.
Learning Resources and Community Support
There are abundant resources available for developers seeking help with Visual C++. Consider utilizing forums such as Stack Overflow, where community experts can provide assistance. Additionally, Microsoft's official documentation offers vast tutorials, guides, and examples helpful for troubleshooting.
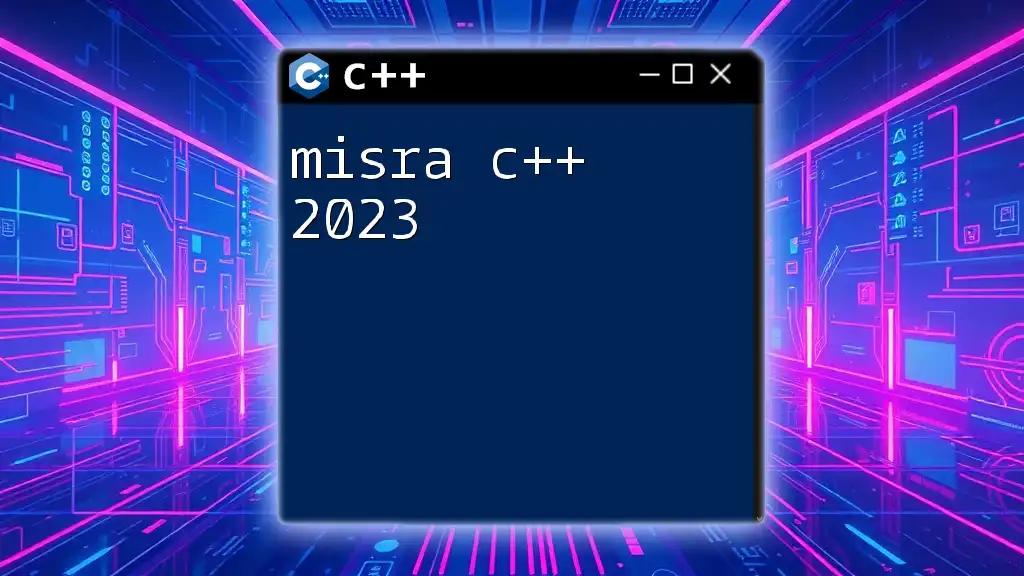
Conclusion
The advancements from Visual C++ 2015-2022 present a compelling case for developers looking to build robust applications. With enhanced performance, modern features, and tools that cater to efficiency and productivity, this IDE stands as a strong candidate for C++ development. Engaging with the community and actively practicing your skills can propel your journey into the world of C++ programming.
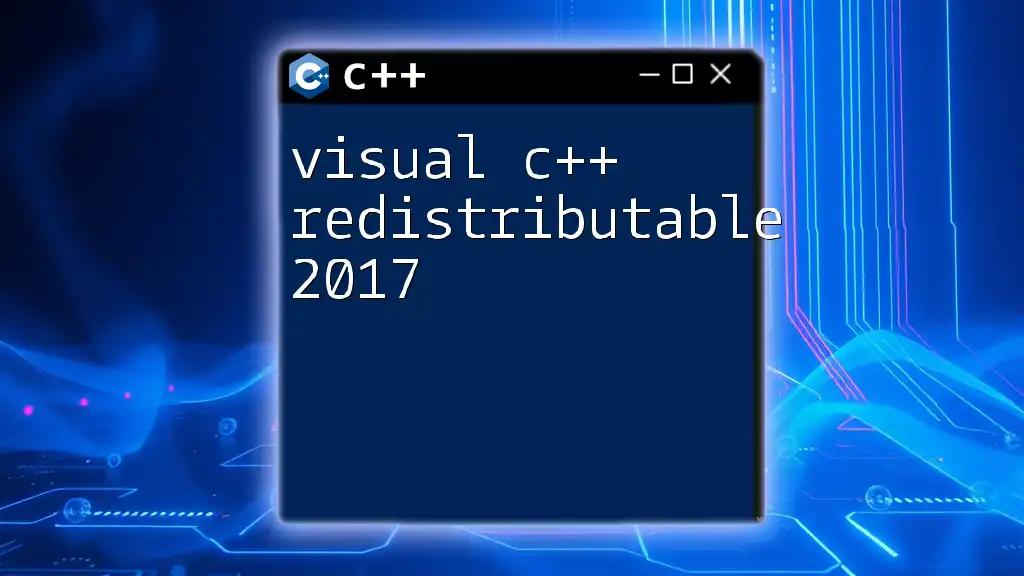
Call to Action
Join our community to stay updated on best practices, tutorials, and resources for mastering Visual C++. Explore our courses that aim to sharpen your C++ skills and equip you with the tools you need to excel in software development!