The "cpp 2024-2025" refers to the C++ programming language and its evolving standards, highlighting new features and best practices that developers should adopt for modern coding efficiency.
#include <iostream>
int main() {
std::cout << "Hello, C++ 2024-2025!" << std::endl; // Outputs a greeting to the console
return 0;
}
Key Features of C++ 2024-2025
New Language Features
One of the most exciting aspects of C++ 2024-2025 is the introduction of new language features that enhance its expressive capabilities. Two prominent improvements include enhanced type inference and additional options for structuring code.
Enhanced Type Inference
C++ now allows for even more effective type deduction, making code both cleaner and easier to maintain. The use of `auto` and `decltype` has been refined, allowing developers to infer types more seamlessly.
For example, consider the following code snippet:
auto x = 10; // Type inferred as int
decltype(x) y = 20; // y is also of type int
Here, the compiler automatically deduces that `x` is of type `int`, and `y` inherits this type through `decltype`. This minimizes the need for explicit type declarations, streamlining the coding process.
Improvements in the Standard Library
The Standard Template Library (STL) has seen substantial upgrades with new algorithms and data structures aimed at performance enhancements and usability.
Introduction of the Latest STL Features
For instance, new algorithms that support parallel execution have been introduced, allowing for more efficient data processing. The following code exemplifies how to utilize a parallel sort:
#include <algorithm>
#include <execution>
#include <vector>
std::vector<int> data = {5, 3, 8, 1, 2};
std::sort(std::execution::par, data.begin(), data.end());
This parallel execution model can significantly reduce runtime for large datasets by utilizing multiple threads under the hood.
Performance Optimizations
C++ 2024-2025 brings notable performance optimizations that focus on compilation speed and runtime enhancements.
Compilation Speed Improvements
With the introduction of modules, the time spent compiling large projects is dramatically reduced. Modules allow developers to compartmentalize code more effectively, reducing dependencies and thus speeding up compilation. This results in faster build times, which is crucial for large-scale applications where waiting can consume substantial developer productivity.
C++ Core Guidelines and Best Practices
As C++ evolves, adhering to the C++ Core Guidelines has become increasingly important for maintaining code quality. These guidelines are designed to help programmers write safe and efficient code by advocating for best practices.
Overview of C++ Core Guidelines
The guidelines include rules for resource management, type safety, and enhancing performance. Following these guidelines ensures that developers write code that is not only effective but also robust against common pitfalls.
Implementing Best Practices
A prime example of these best practices is the use of smart pointers instead of raw pointers. Using smart pointers like `unique_ptr`, `shared_ptr`, and `weak_ptr` helps manage memory automatically, reducing the risk of leaks and dangling pointers.
Consider this example:
#include <memory>
struct MyClass {
int value;
};
std::unique_ptr<MyClass> ptr1 = std::make_unique<MyClass>();
This creates a `unique_ptr` that manages the `MyClass` instance, automatically releasing the memory when it goes out of scope, ensuring efficient memory management.
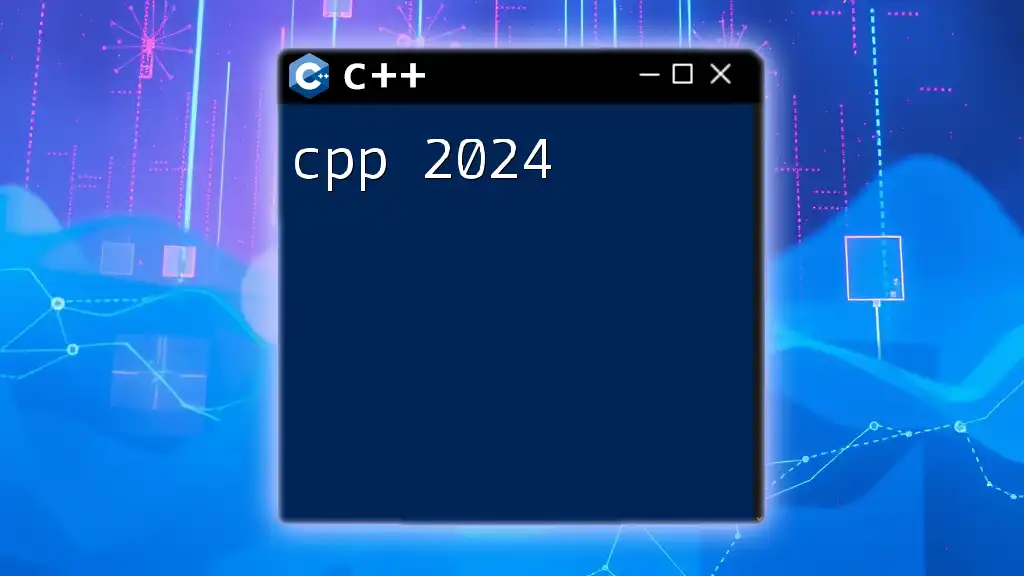
Modern C++ Development Tools
IDEs and Compilers
The choice of development tools can significantly impact productivity and code quality. Popular Integrated Development Environments (IDEs) include:
- Visual Studio: Widely used for its rich feature set and extensive debugging tools.
- CLion: Known for its intelligent code analysis and refactoring capabilities.
- Code::Blocks: A lightweight option suitable for beginners and hobbyists.
Each IDE has its merits and can cater to different developer preferences and project requirements.
Version Control and Collaboration Tools
Utilizing version control systems like Git is essential for maintaining a clean development workflow. It allows developers to track changes, revert to previous states, and collaborate effectively with others.
Adopting a simple Git workflow can enhance productivity. For instance, starting with `git init`, adding files with `git add`, and preparing commits with `git commit` keeps your project organized and version-controlled.
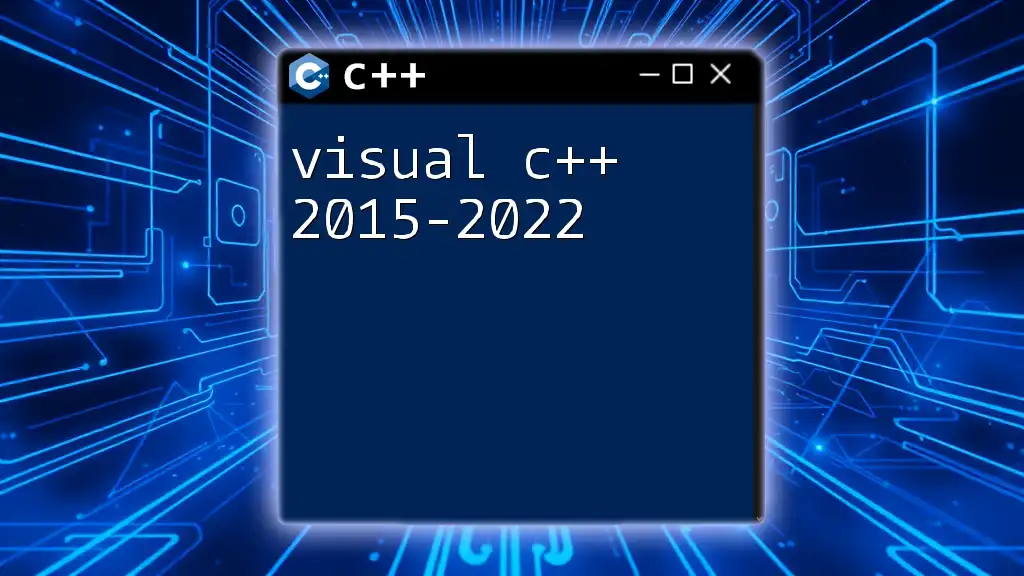
C++ Community and Resources
Learning Platforms
To stay updated with the advancements in C++, leveraging online learning platforms is key. Top options include Coursera, Udemy, and edX, offering a variety of courses covering both foundational and advanced C++ topics.
Additionally, many free resources, such as tutorials and MOOCs, are available for those who wish to learn without financial commitment.
Participating in the C++ Community
Engaging in the C++ community can provide invaluable support. Forums like Reddit’s r/cpp and Stack Overflow are excellent places to ask questions, share knowledge, and collaborate with fellow C++ enthusiasts.
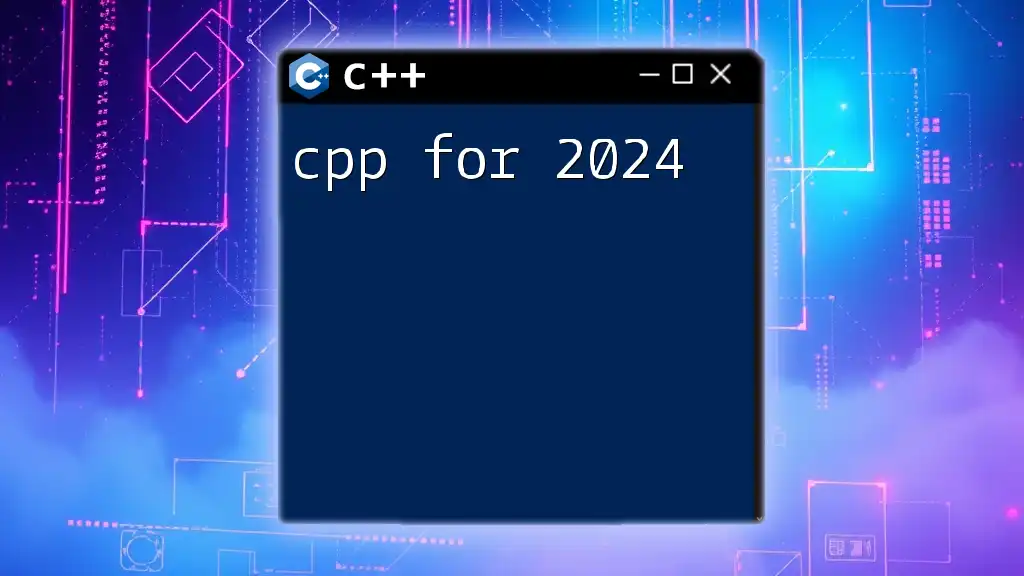
Future of C++: Trends to Look Forward To
Industry Predictions for C++
Looking ahead, C++ is poised to maintain its relevance across several sectors. Industries like gaming, financial services, and IoT continue to rely extensively on C++ for its performance capabilities and efficiency. As these sectors grow, so will the demand for skilled C++ developers.
Preparing for C++ in 2025 and Beyond
To stay competitive, developers should focus on continual learning. Emphasizing modern features, tooling, and best practices will keep you at the forefront of C++ programming. Awareness of anticipated changes in the upcoming C++ standards will further prepare you for the future landscape of software development.
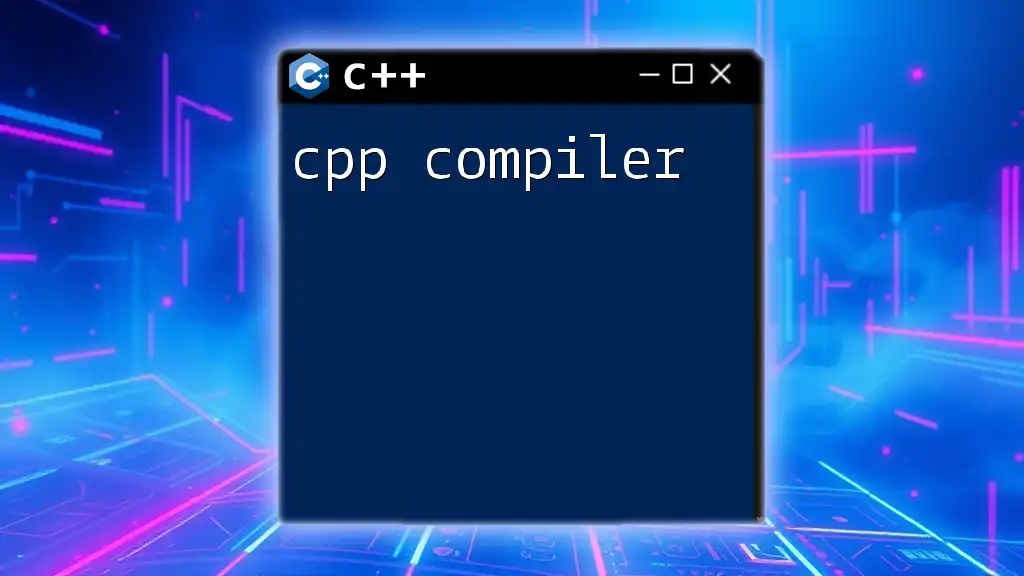
Conclusion
As we navigate through C++ 2024-2025, understanding these key advancements and evolving best practices is essential for any developer. By focusing on new features, leveraging modern tools, and engaging with the community, you position yourself for success in an ever-evolving field. Embrace these changes today and unlock the full potential of your C++ programming journey!