A C++ compiler is a specialized program that converts C++ source code into executable machine code, enabling the running of C++ applications.
Here's a simple code snippet demonstrating a basic C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Compilers
What is a C++ Compiler?
A C++ compiler is a specialized program that translates the human-readable code (source code) written in C++ into machine-readable code (object code or executable). The primary purpose of a compiler is to transform high-level programming language instructions into a format that a computer can execute.
How C++ Compilers Work
The compilation process involves several key phases, namely preprocessing, compiling, assembling, and linking. Each phase plays a crucial role in the transformation of source code into an executable program:
-
Preprocessing: The compiler processes directives (like `#include` and `#define`) before actual compilation begins. It prepares the source code by including necessary files and defining constants.
-
Compiling: In this phase, the preprocessed code is translated into assembly language, a low-level representation of the instructions.
-
Assembling: The assembler converts the assembly language into machine code, generating an object file—this is not yet linked to any libraries or functions.
-
Linking: Finally, the linker combines one or more object files into a single executable program, resolving any references to external libraries and functions.
To illustrate this process, consider the following simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
During the compilation, this will go through all phases before finally creating an executable ready for execution.
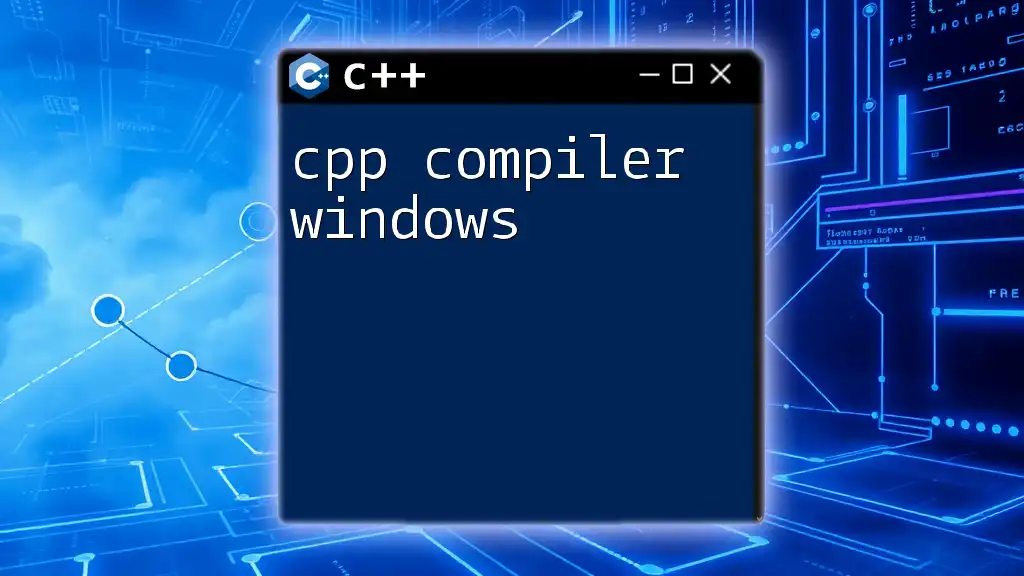
Types of C++ Compilers
Standard C++ Compilers
There are several widely-used standard C++ compilers, each with its own features and strengths. Some of the most popular include:
-
GCC (GNU Compiler Collection): A robust and versatile compiler that supports various programming languages including C and C++. It is widely used on Unix-like systems.
-
Clang: Known for its fast compilation times and supportive error reporting, Clang is a popular choice among developers using macOS and Linux.
-
MSVC (Microsoft Visual C++): This is the primary compiler for Windows development and is integrated into Microsoft's Visual Studio IDE, providing advanced debugging and performance profiling tools.
You can see differences in feature support and performance when compiling the same code with different compilers, as demonstrated by the following simple code example that prints "Hello, World!".
Cross-Platforms Compilers
Cross-platform compilers allow developers to build applications on one operating system for another. This is especially important for projects that will run on multiple platforms. Examples include:
- MinGW (Minimalist GNU for Windows): A port of the GCC for Windows that enables developers to compile native Windows applications.
- Cygwin: Provides a large collection of GNU and Open Source tools which provide functionality similar to a Linux distribution on Windows.
- Cross-compilation tools: Enable code to be compiled on one machine for another platform, making development more flexible.
Online Compilers
Online compilers offer a quick and convenient way to write and run C++ code without any installation. They are especially useful for beginners or when testing small code snippets. However, they may have limitations in terms of support for specific libraries or system-level features.
Examples of popular online C++ compilers include:
- Repl.it: Supports various programming languages and provides collaborative coding features.
- Ideone: Useful for running small snippets of code and sharing them for troubleshooting.
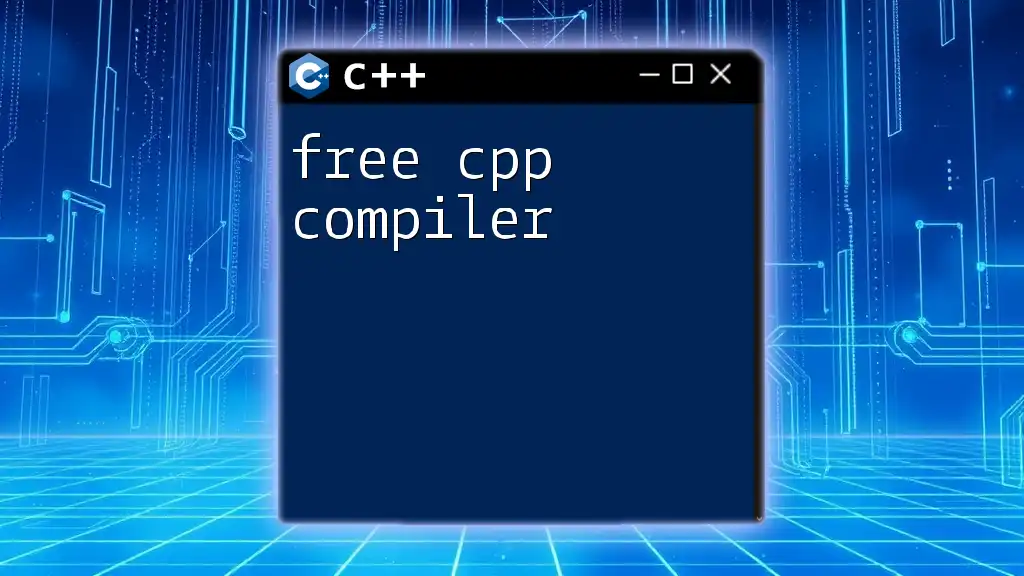
Installing a C++ Compiler
Installing GCC
To get started with GCC, follow these installation steps based on your operating system:
-
Windows: Use MinGW and download the installer. Once installed, add the MinGW bin directory to your system PATH to access the compiler via the command line.
-
Mac: GCC can be installed via Homebrew. Open Terminal and run:
brew install gcc
- Linux: Most distributions come with GCC pre-installed, but you can always update or install via your package manager. For Ubuntu, you would run:
sudo apt-get install build-essential
Setting Up Clang
Installing Clang can vary based on the operating system:
-
Windows: Usually bundled with Visual Studio, select the Clang tools during installation.
-
Mac: Clang is included in Xcode; you can install it via the Mac App Store or run:
xcode-select --install
- Linux: Similar to GCC, it can be installed using your package manager. A command for Ubuntu would be:
sudo apt-get install clang
Using MSVC
For Windows users, MSVC is typically part of Visual Studio. Here’s how to set it up:
- Download Visual Studio from Microsoft’s official site.
- During installation, choose the Desktop Development with C++ workload.
- Once installed, you can create a new project and start coding immediately.
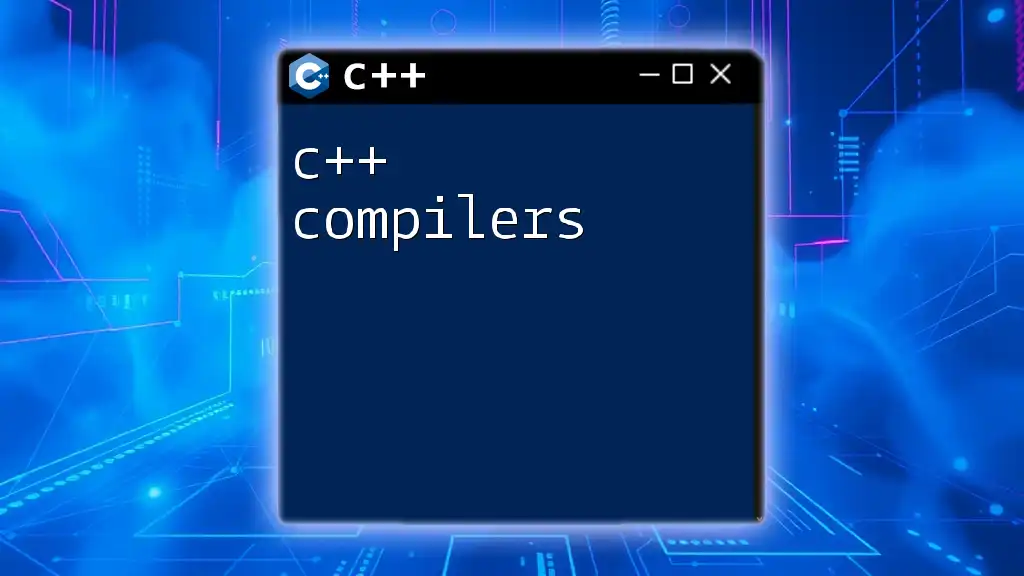
Writing Your First C++ Program
Creating a Simple C++ Program
Let’s write a classic "Hello, World!" program that serves as a foundational example.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code:
- `#include <iostream>` brings in the input-output stream library useful for console output.
- `int main()` defines the starting point of the program.
- The `std::cout` statement outputs "Hello, World!" to the console.
- Finally, `return 0;` indicates successful execution of the program.
Compiling Your First C++ Program
To compile the program using various compilers, the following commands can be used:
- GCC:
g++ -o hello hello.cpp
- Clang:
clang++ -o hello hello.cpp
- MSVC (using Developer Command Prompt):
cl hello.cpp
Executing the compiled program will display "Hello, World!" in the console.
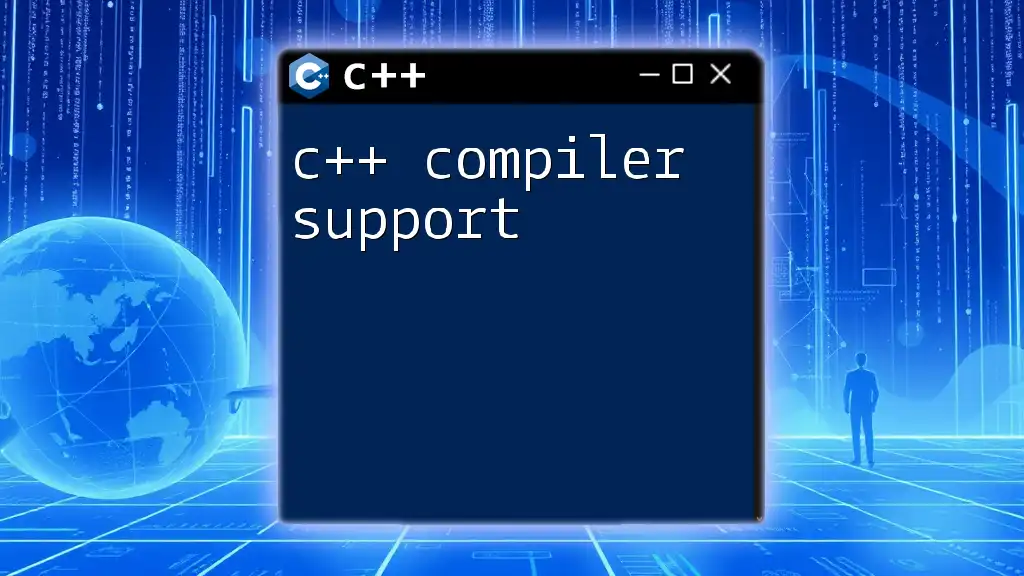
Common Compilation Errors
Understanding Compilation Errors
Compilation errors arise when the code cannot be successfully converted into an executable. The types of errors may include syntax errors, linking errors, and runtime errors.
-
Syntax Errors: Often due to missing semicolons, mismatched parentheses, etc. The compiler highlights these errors with line numbers and descriptions.
-
Linking Errors: Occur when functions or variables are declared but not defined, or related libraries are missing.
-
Runtime Errors: Happen when the program compiles successfully but fails during execution, often due to logic errors or improper resource access.
Troubleshooting Common Errors
It’s essential to learn how to read and interpret compiler error messages effectively. For instance, if there is a syntax error in the following code:
#include <iostream>
int main() {
std::cout << "Testing error" << std::endl // Missing semicolon
return 0;
}
The compiler will highlight the line and show where the mistake occurred, prompting the developer to fix it with a proper semicolon.
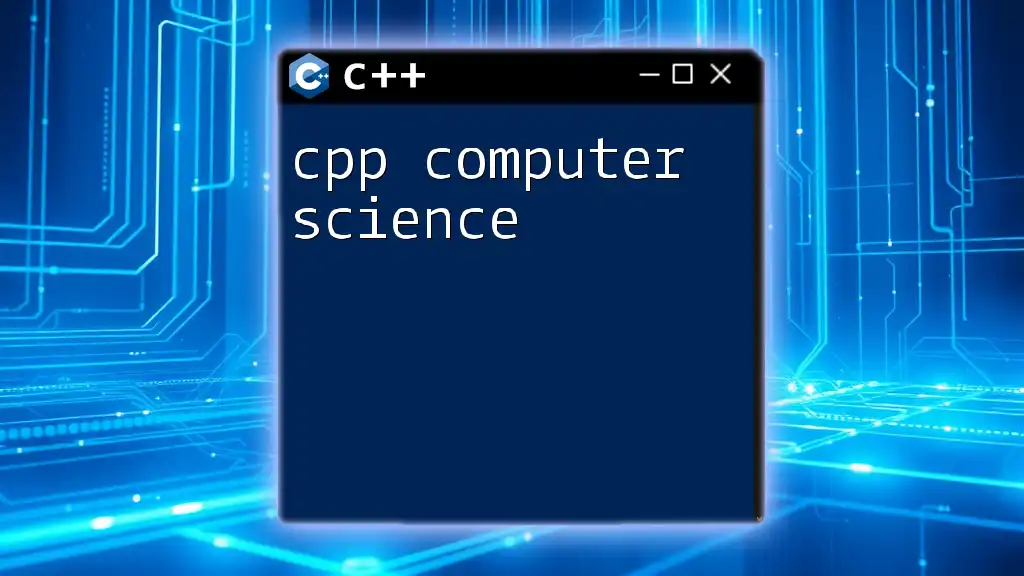
Optimizing Your C++ Code with Compiler Flags
What Are Compiler Flags?
Compiler flags are specific parameters or options that modify the behavior of the compiler during the compilation process. They can impact optimization levels, debugging information, and warnings.
Examples of Compilation with Flags
Using flags can significantly enhance performance or help identify potential issues in your code. Here are a few commonly used flags:
- `-O2`: This flag asks the compiler to optimize the code for better speed.
- `-Wall`: This enables all compiler’s warning messages, which helps in identifying potential issues early on.
- `-g`: This flag is used to include debugging information, which is essential during the debugging process.
To compile code using flags, you can use commands like:
- GCC:
g++ -O2 -Wall -g -o program program.cpp
- Clang:
clang++ -O2 -Wall -g -o program program.cpp
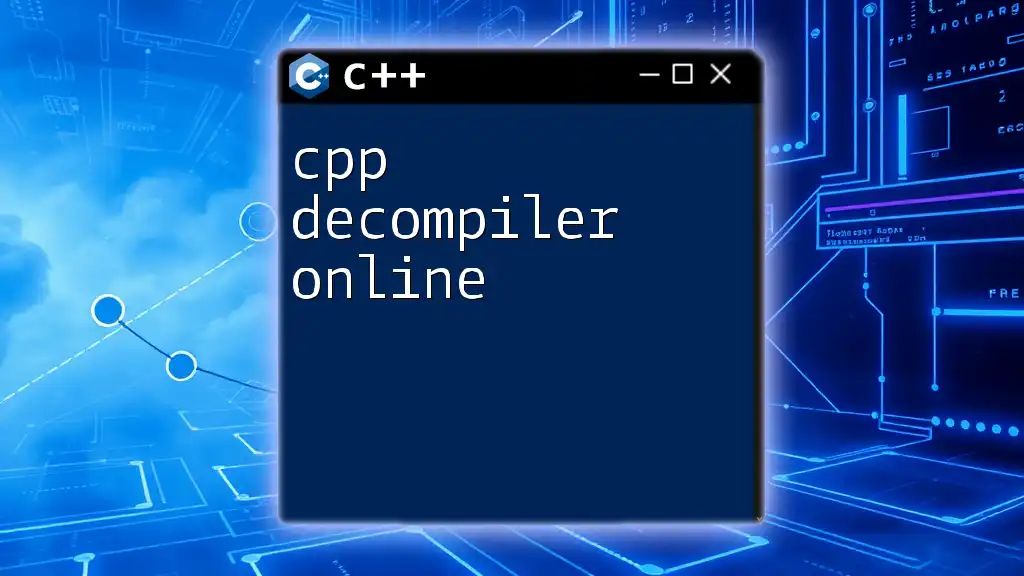
Working with Different C++ Standards
Understanding C++ Standards
C++ has evolved significantly over the years, introducing new features and improvements with each version. Important standards include:
- C++11: Introduced features such as auto keyword, nullptr, range-based for loops, etc.
- C++14: Brought enhancements to templates and lambdas, improving code efficiency.
- C++17: Added features like std::optional and std::variant, enhancing flexibility in coding.
Setting the C++ Standard in Compilers
Depending on the desired C++ standard, you can specify it in your compiler commands. For instance:
- GCC:
g++ -std=c++11 -o program program.cpp
- Clang:
clang++ -std=c++17 -o program program.cpp
- MSVC: In Visual Studio, you can set the C++ standard version in project properties under "C/C++" -> "Language".
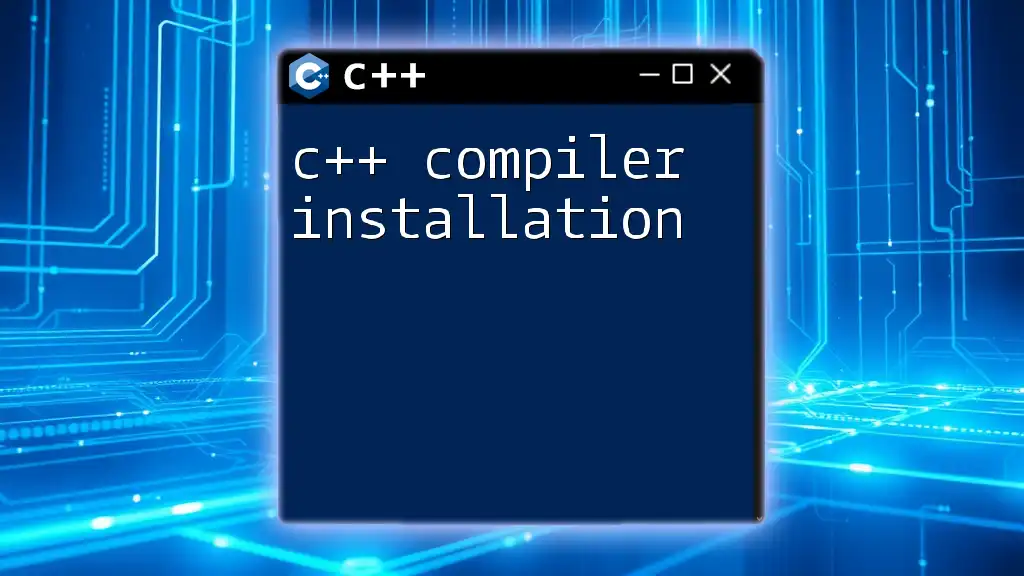
Conclusion
Recap of Key Points
In this guide, we’ve explored what a C++ compiler is, how it functions, the various types available, and how to install and use them effectively. We’ve also covered writing basic C++ programs, compiling them, common errors encountered, and tips for optimizing code.
Next Steps
To continue your journey in C++ programming, consider exploring advanced topics such as template programming, multi-threading, or design patterns.
Call to Action
To become proficient in C++, sign up for further tutorials and courses where we delve deeper into mastering C++ commands and usage, guiding you toward becoming a confident C++ developer.