C++ (or cpp) is a powerful, high-performance programming language widely used for system/software development, enabling programmers to write efficient and concise code.
Here’s a simple code snippet that demonstrates a basic C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Basics
What is C++?
C++ is a powerful general-purpose programming language that was developed by Bjarne Stroustrup in 1979 as an enhancement to the C programming language. The language is well-known for its flexibility and performance, making it a popular choice among developers in various fields, particularly computer science. C++ incorporates both high-level and low-level programming features, effectively allowing developers to directly manage memory while also enabling high-level abstractions like classes and objects.
Its key features include:
- Object-Oriented Programming (OOP): C++ supports encapsulation, inheritance, and polymorphism, characteristics that allow for the creation of complex, modular applications.
- Performance: It is compiled to efficient machine code, making it an excellent choice for systems programming and performance-critical applications.
- Rich Standard Library: C++ comes with the Standard Template Library (STL), providing numerous tools and data structures that enhance productivity and efficiency.
Setting Up Your C++ Environment
Before diving into the cpp computer science realm, you’ll need the right tools and software. Here are some essentials to get you started:
-
C++ Compilers:
- GCC (GNU Compiler Collection)
- MSVC (Microsoft Visual C++)
- Clang
-
Integrated Development Environments (IDEs):
- Visual Studio: Popular choice with robust support for C++.
- Code::Blocks: A free, open-source IDE for C++.
- Eclipse: Excellent for C/C++ development.
Installation Guide
For Windows users, Visual Studio is highly recommended. Just download the installer from the official website, select C++ development tools during installation, and you’re ready to code!
For macOS, the simplest way is to install the Xcode Command Line Tools using the terminal command:
xcode-select --install
On Linux, you can install GCC easily with:
sudo apt install g++
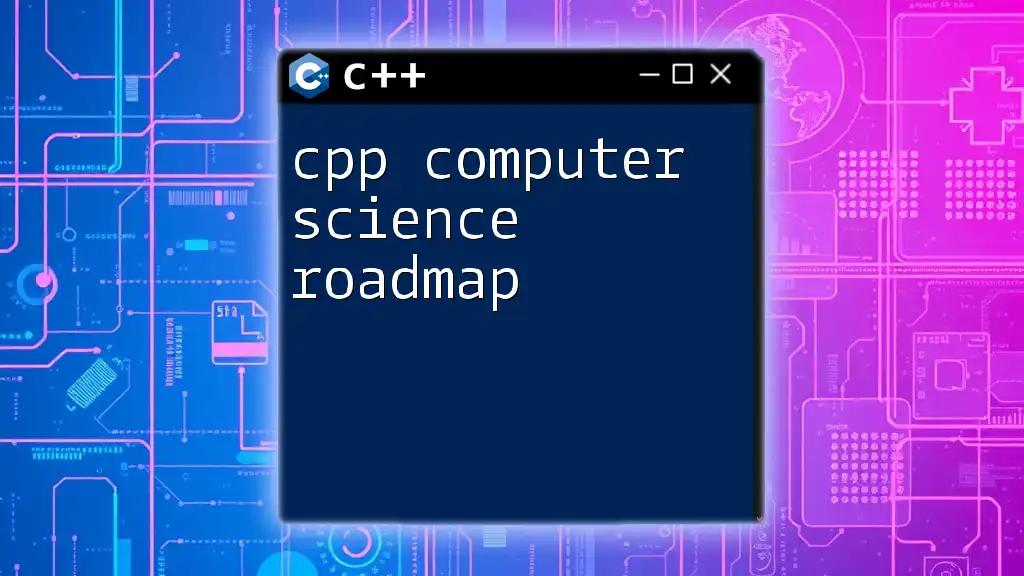
Core Concepts of C++
Syntax and Structure
Understanding the basic syntax and structure of a C++ program is crucial. A typical C++ program includes:
- Include directives
- The `main` function
- Statements and expressions
Here’s an example of a simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This code outputs "Hello, World!" to the console. Remember: The `#include <iostream>` directive is necessary to use the input-output library.
Variables and Data Types
C++ offers a variety of data types, which can be broadly categorized into:
- Basic Data Types: int, float, double, char
- Complex Data Types: string, arrays, structures
- User-Defined Data Types: classes, enums, etc.
Variable declaration and initialization is simple, as shown below:
int age = 25;
float height = 5.9;
char initial = 'A';
Control Structures
Conditional Statements
C++ allows for decision-making through conditional statements, such as `if`, `else if`, and `switch`. These structures enable execution of different code blocks based on condition evaluations.
Here’s an example of an if-else statement:
if (age >= 18) {
std::cout << "Adult";
} else {
std::cout << "Minor";
}
Loops
Loops are fundamental to repeat actions. C++ provides several loop constructs:
- For loop: Ideal for a known number of iterations.
- While loop: Useful when the number of iterations is unknown.
- Do-while loop: Executes at least once before checking the condition.
Here’s an example of a for loop:
for (int i = 0; i < 5; i++) {
std::cout << "Iteration: " << i << std::endl;
}
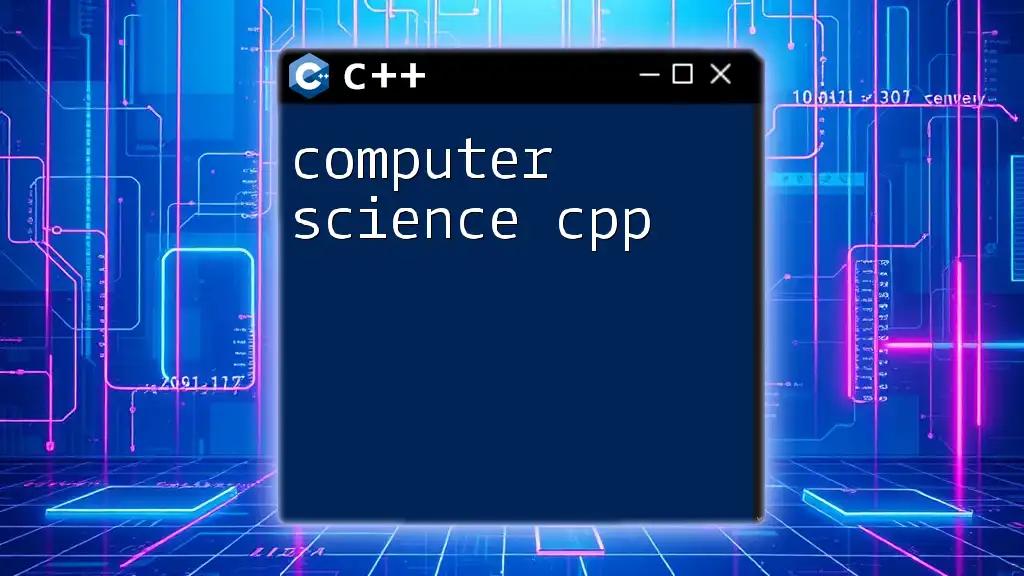
Object-Oriented Programming in C++
Classes and Objects
At the heart of C++ is its capability for object-oriented programming (OOP), which revolves around classes and objects. A class serves as a blueprint for creating objects (instances of classes) by encapsulating data and behaviors together.
Here's how to define a simple class in C++:
class Car {
public:
std::string brand;
void honk() {
std::cout << "Beep!" << std::endl;
}
};
To create an object and access its properties:
Car myCar;
myCar.brand = "Toyota";
myCar.honk(); // Outputs: Beep!
Inheritance
C++ supports inheritance, allowing new classes to derive properties and methods from existing ones. This promotes code reusability and helps in creating hierarchical models.
For instance:
class Vehicle {
public:
void start() {
std::cout << "Vehicle starting." << std::endl;
}
};
class Car : public Vehicle {
public:
void honk() {
std::cout << "Beep!" << std::endl;
}
};
Polymorphism
Polymorphism allows methods to do different things based on the object that it is acting upon, providing flexibility to your code. In C++, it can be achieved through function overloading (same function name, different parameters) and operator overloading (defining the behavior of operators for user-defined types).
Example of function overloading:
void display(int i) {
std::cout << "Integer: " << i << std::endl;
}
void display(double d) {
std::cout << "Double: " << d << std::endl;
}
Encapsulation and Abstraction
Encapsulation refers to bundling the data and the methods that operate on that data within a single unit, typically a class. By restricting access to some of an object’s components, you can protect the integrity of the data. Access specifiers (public, protected, private) control this access.
Abstraction, on the other hand, provides a way to create a simplified model of complex real-world entities. This is achieved using abstract classes and interfaces, which focus on what an object does rather than how it does it.
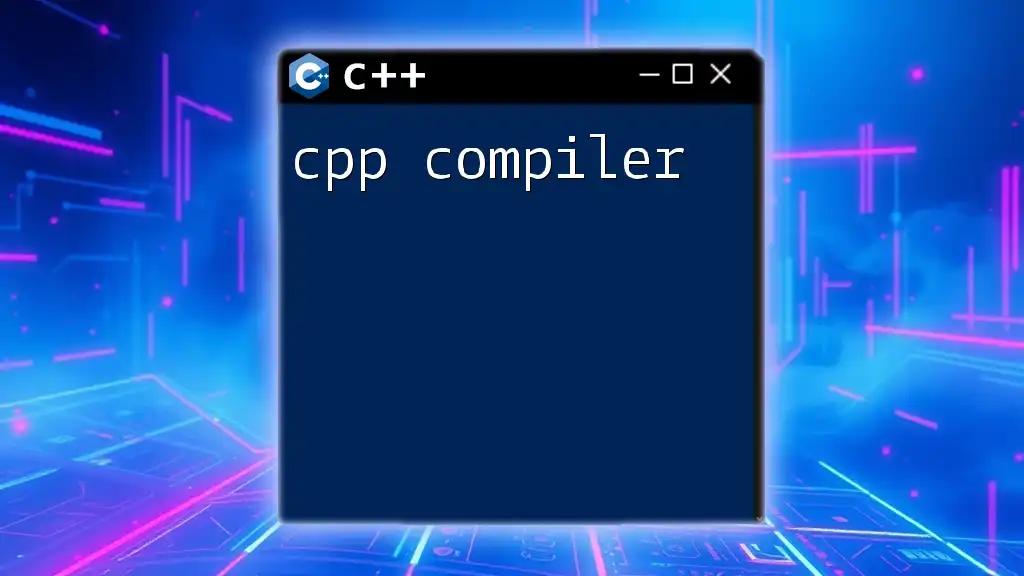
Advanced C++ Concepts
Templates
Templates are a powerful feature in C++, enabling generic programming. They allow you to create functions and classes that operate with any data type. This promotes reusability and flexibility, which is essential in cpp computer science.
Here’s an example of a function template:
template <class T>
T add(T a, T b) {
return a + b;
}
Standard Template Library (STL)
The Standard Template Library (STL) is an integral part of C++. It offers a set of common classes and functions that implement data structures and algorithms. The main components of STL include:
- Containers: Vectors, lists, sets, maps
- Algorithms: Functions to manipulate data (sorting, searching)
- Iterators: Objects that enable traversal of containers
Here’s how to use a vector from the STL:
#include <vector>
std::vector<int> scores = {100, 90, 80};
scores.push_back(70); // Adds 70 to the end of the vector
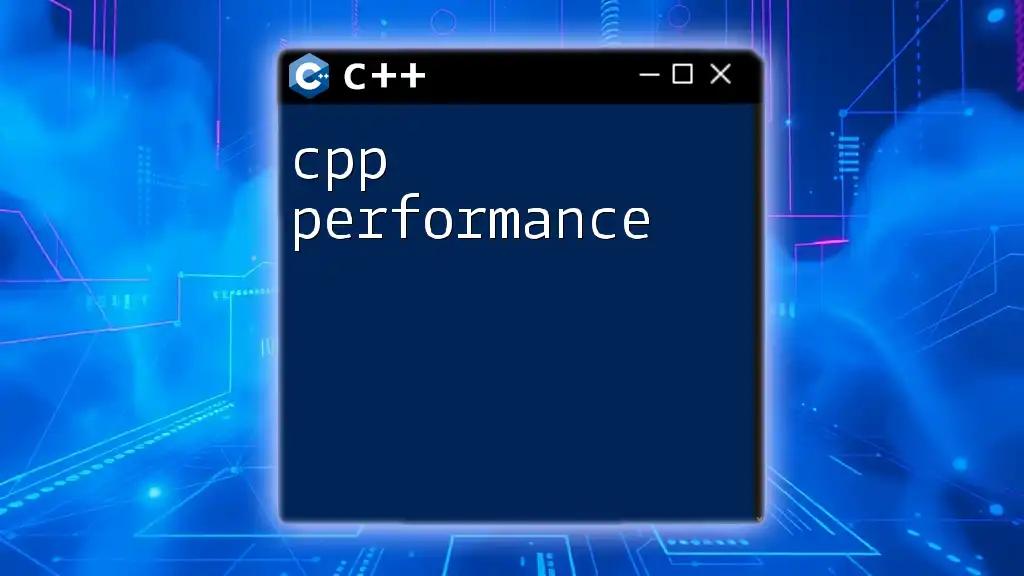
Error Handling in C++
Exception Handling
Handling errors gracefully is crucial in programming. C++ uses exceptions to manage unexpected events at runtime. You can use `try`, `catch`, and `throw` statements to achieve error handling.
Here’s an example:
try {
throw std::runtime_error("An error occurred");
} catch (std::runtime_error &e) {
std::cout << e.what() << std::endl;
}
By using exceptions, you can separate error-handling code from regular code, leading to cleaner and more maintainable code.
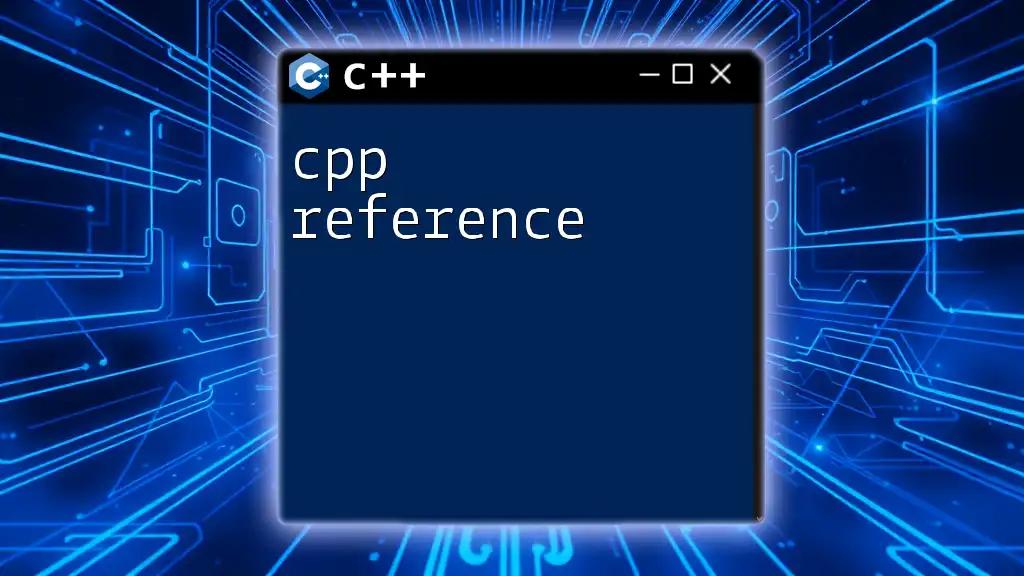
Best Practices in C++
Writing Clean and Maintainable Code
Maintaining a clean codebase is essential for long-term success in software development. To achieve this, consider the following best practices:
- Code Organization: Use modules to separate functionalities.
- Documentation: Comment your code to clarify complex logic.
- Naming Conventions: Use descriptive names for functions and variables.
By following these practices, you enhance readability and maintainability, critical components in cpp computer science.
Performance Optimization
Understanding performance implications is key for applications that demand efficiency, such as games and real-time systems. Focus on optimizing:
- Algorithm Complexity: Choose algorithms with lower time complexity.
- Memory Management: Be mindful of dynamic memory allocation and deallocation.
- Avoiding Data Copies: Use references and pointers to minimize unnecessary copies.
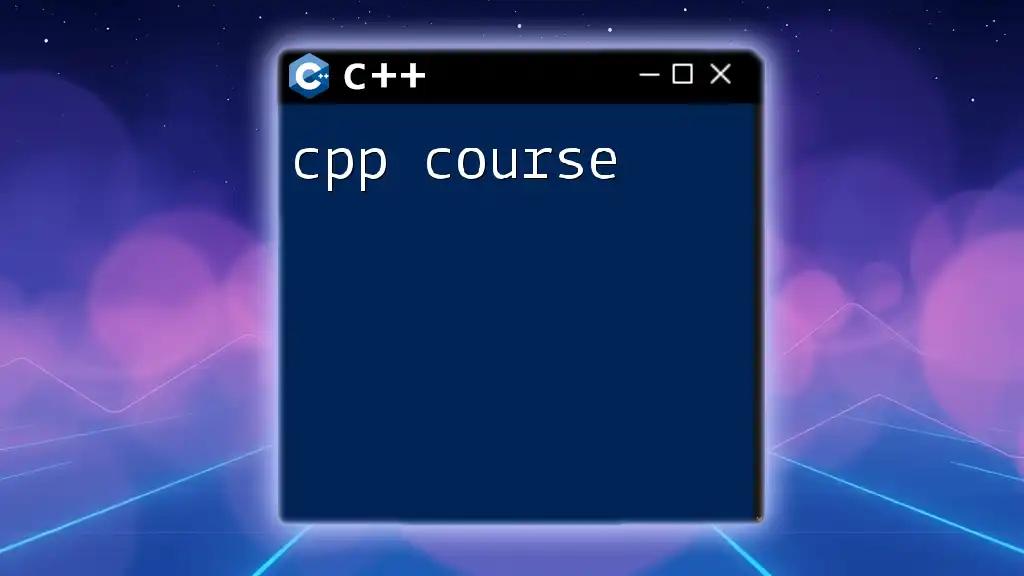
Real-World Applications of C++
C++ in Game Development
C++ is a prevalent language in game development, largely due to its performance capabilities. It forms the backbone of many popular game engines like Unreal Engine, offering full control over system resources.
Developers can leverage C++ to create complex game mechanics and physics simulations, bringing immersive gaming experiences to life.
C++ in Systems Programming
Another crucial application of C++ is in systems programming, where it’s used to develop operating systems, embedded systems, and device drivers. Its ability to interact closely with hardware combined with high performance makes it an ideal choice for such low-level programming tasks.
C++ in Data Science and Machine Learning
While Python is often favored for data science due to its simplicity, many libraries that support machine learning, like TensorFlow and OpenCV, leverage C++ for their underlying performance. Learning C++ opens pathways to understanding these libraries better, enabling you to optimize routines and algorithms.
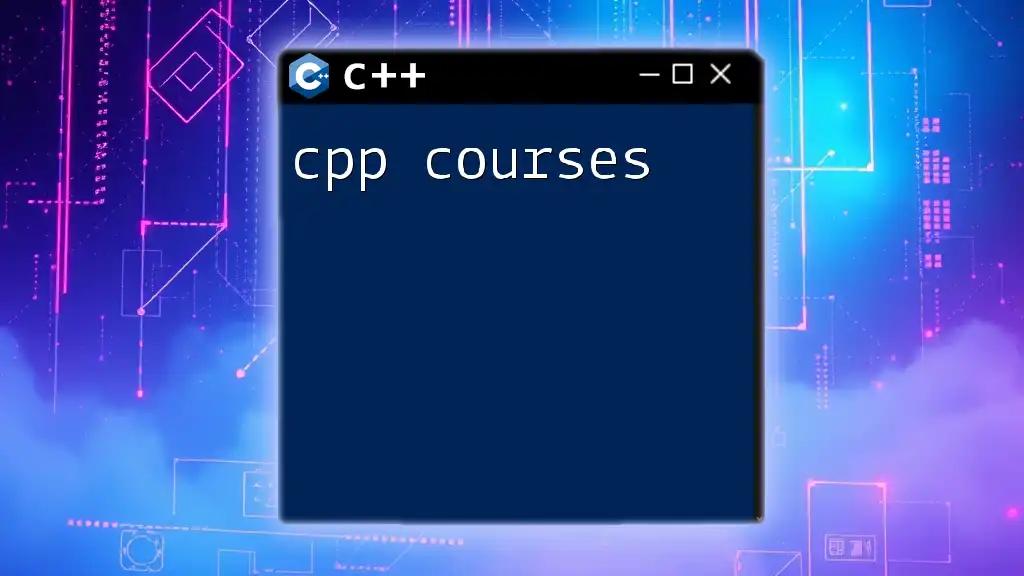
Conclusion
Mastering C++ is invaluable for anyone pursuing a career in cpp computer science. Its versatility will empower you to develop everything from simple applications to complex systems and tools. Continued practice and exploration of C++ are essential, and a plethora of resources are available to guide you along the way.
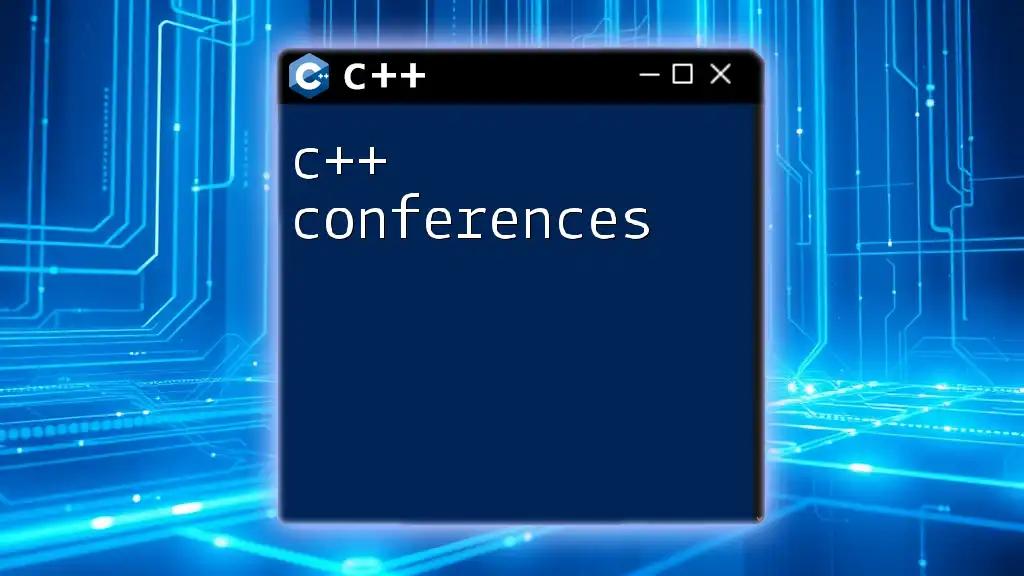
Call to Action
If you are eager to deepen your understanding of C++ or require structured learning, join our C++ course! Transform your passion into proficiency by engaging with a community of learners and experienced programmers. Share this article and connect with others on their journey to mastering C++.