"Computer Science C++" refers to the foundational concepts and programming principles utilized in C++, a powerful programming language commonly used for system/software development and game programming.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a high-level programming language that is widely used in various domains such as system software, game development, real-time simulations, and more. Developed in the early 1980s by Bjarne Stroustrup at Bell Labs, C++ extends the capabilities of the C programming language by introducing object-oriented programming (OOP) features. This evolution allowed developers to build more complex and scalable applications. Its versatility and performance make it a staple in the software industry.
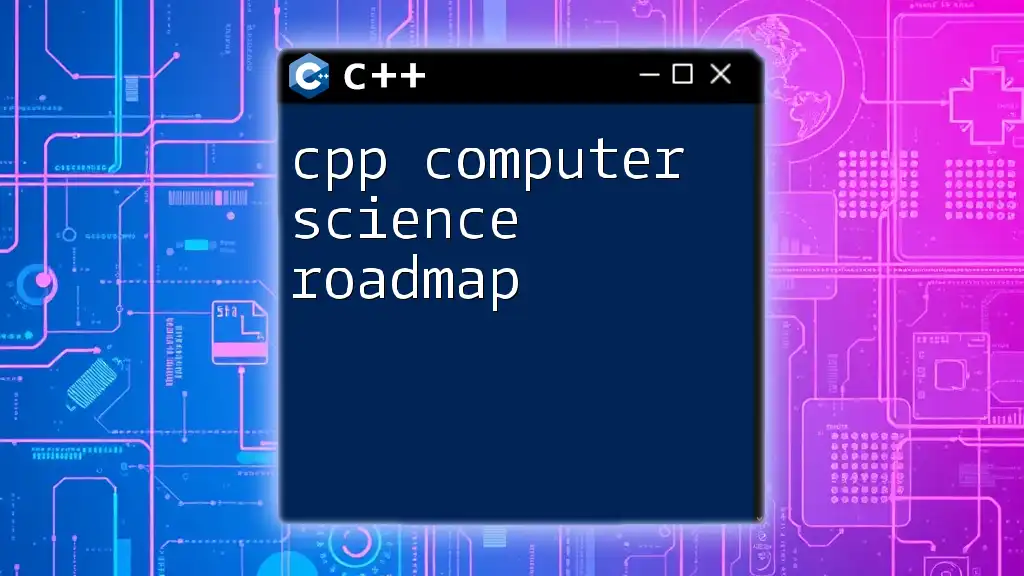
Importance of Learning C++
Studying C++ holds significant value in modern computer science due to its:
- Industry Relevance: Many large-scale applications, databases, and operating systems are developed using C++. Familiarity with the language equips you to work on critical projects.
- Performance Efficiency: C++ allows for fine control over system resources, making it favorable for performance-intensive applications.
- Foundation for Other Languages: Learning C++ provides a solid grounding in programming fundamentals that is transferable to languages like C#, Java, and even Python.
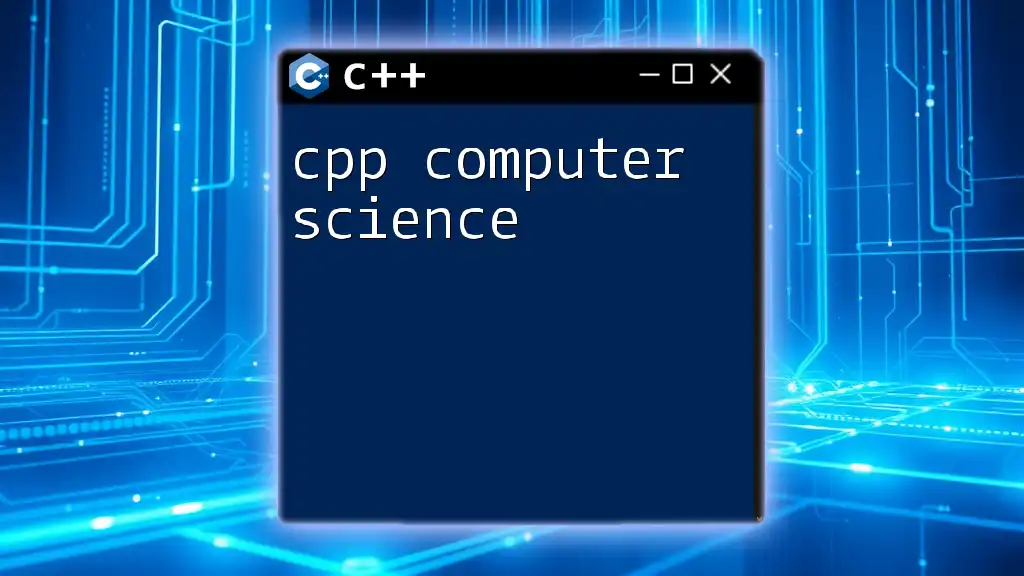
Basics of C++ Syntax
Understanding C++ Structure
A C++ program consists of functions, variables, and statements that define its behavior. The typical structure follows a straightforward pattern. Below is a simple example illustrating a basic C++ program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this code:
- `#include <iostream>` includes the input-output stream library.
- `using namespace std;` allows us to use standard functions without prefixing them.
Data Types and Variables
C++ supports various data types that allow developers to efficiently store and manipulate data. These include:
- int for integers (e.g., `int age = 30;`)
- float for floating-point numbers (e.g., `float salary = 3000.50;`)
- char for characters (e.g., `char initial = 'A';`)
- bool for boolean values (`true` or `false`).
Welcoming the flexibility of C++, you can declare variables as follows:
int age = 30;
float salary = 3000.50;
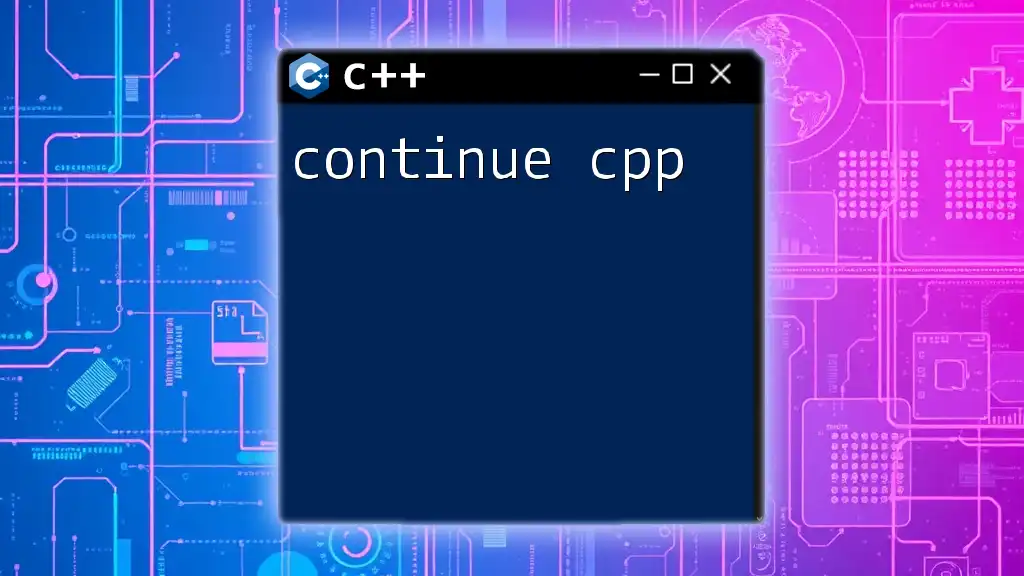
Control Structures in C++
Conditional Statements
Control flows in C++ rely heavily on conditional statements. The `if`, `else if`, and `else` constructs help in making decisions:
if (age > 18) {
cout << "Adult" << endl;
} else {
cout << "Minor" << endl;
}
Loops: The Heart of Programming
Loops allow you to execute a block of code multiple times. C++ offers several looping constructs:
- For loop: Ideal for scenarios where the number of iterations is known.
- While loop: Best when the number of iterations isn't defined beforehand.
- Do-While loop: Ensures that the block of code runs at least once.
Example of a for loop:
for (int i = 0; i < 5; i++) {
cout << "Iteration: " << i << endl;
}
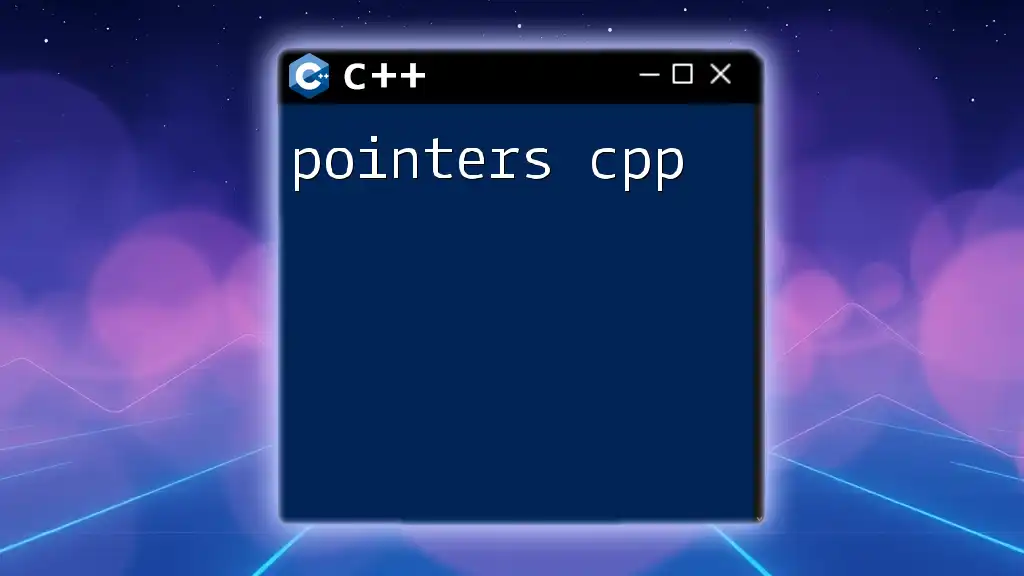
Functions in C++
Defining Functions
Functions encapsulate reusable code blocks, enhancing maintainability. Here’s how to define a basic function in C++:
int add(int a, int b) {
return a + b;
}
This piece of code depicts a function named `add`, which takes two integer arguments and returns their sum.
Function Overloading
C++ allows multiple functions to share the same name as long as their parameter types differ. This is known as function overloading:
int add(int a, int b);
float add(float a, float b);
This helps in creating a seamless experience when performing similar operations with different data types.
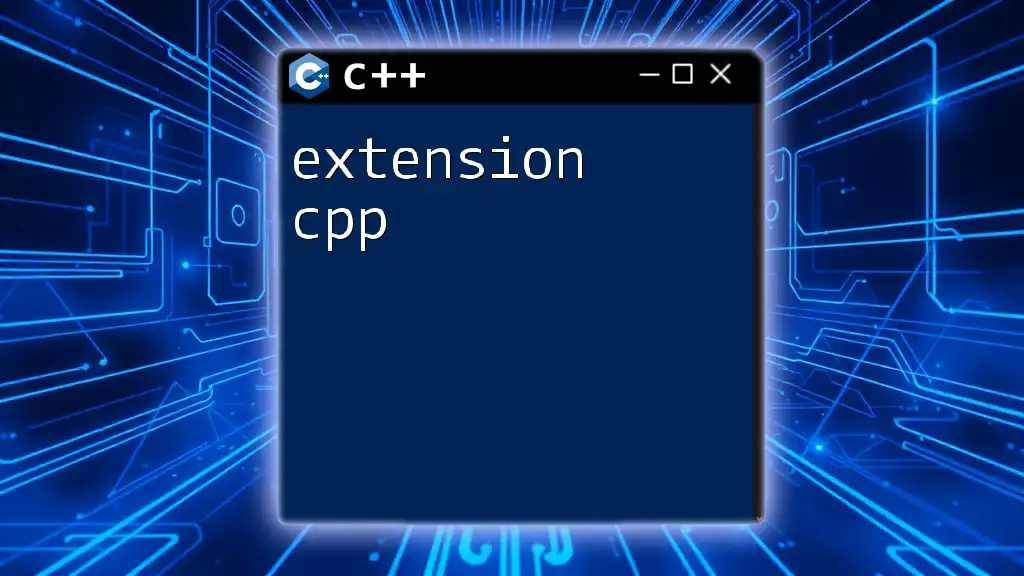
Object-Oriented Programming in C++
Introduction to OOP Concepts
Object-Oriented Programming (OOP) offers a framework based on four main principles: encapsulation, inheritance, and polymorphism.
- Encapsulation: Bundling data and methods to restrict access.
- Inheritance: Deriving new classes from existing ones.
- Polymorphism: Using a single interface to represent different data types.
Creating Classes and Objects
In C++, classes act as blueprints for objects. Here’s a simple class definition illustrating encapsulation:
class Dog {
public:
string name;
void bark() {
cout << "Woof!" << endl;
}
};
Here, `Dog` is a class with public access to its `name` attribute and `bark` method.
Inheritance and Polymorphism
Creating new classes from existing ones promotes reusability. Here’s an example of how to inherit classes:
class Animal {
public:
void speak() { cout << "Animal speaks" << endl; }
};
class Dog : public Animal {
public:
void speak() { cout << "Bark" << endl; }
};
In this snippet, `Dog` inherits from `Animal` and overrides the `speak` method, showcasing polymorphism.
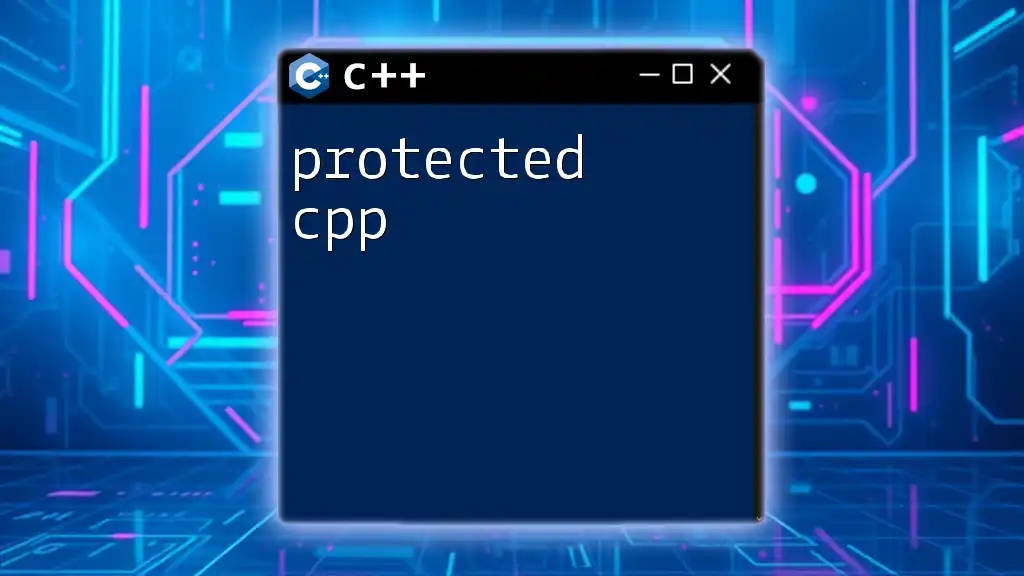
Memory Management in C++
Pointers and References
Pointers offer direct memory address access, allowing for dynamic memory manipulation, a crucial feature in C++. For example:
int a = 10;
int* ptr = &a;
cout << "Value of a: " << *ptr << endl; // Dereferencing
In this code, `ptr` stores the address of `a`, and dereferencing it provides access to the actual value.
Dynamic Memory Allocation
C++ empowers developers with dynamic memory allocation through `new` and `delete`. Here’s how:
int* arr = new int[5]; // allocate array of 5 integers
delete[] arr; // deallocate memory
Using `new` enables the creation of dynamic arrays, while `delete` is essential to prevent memory leaks.
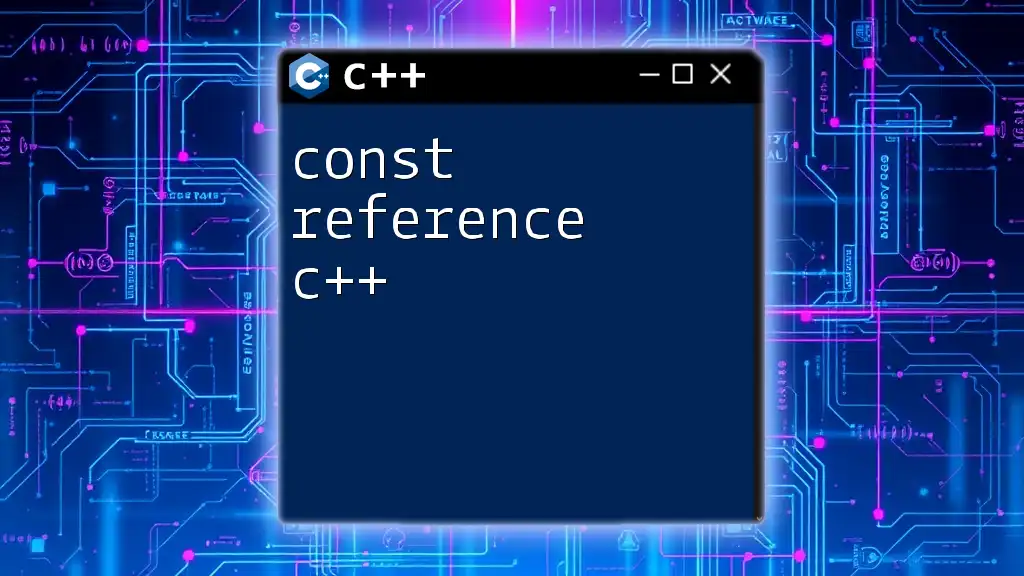
The C++ Standard Library
Overview of the STL
The C++ Standard Library (STL) is a powerful collection of classes and functions that enables data structure manipulations, algorithm implementations, and more. Key components include:
- Containers: Such as vectors, lists, and maps.
- Iterators: To navigate through container elements.
- Algorithms: House functions like sorting and searching.
Working with Vectors
Vectors are dynamic arrays that can grow in size, making them versatile for many applications. Here’s an example of how to use vectors in C++:
#include <vector>
vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
cout << num << " ";
}
In this instance, we create a vector of integers and use a range-based for loop to iterate over and print each element.
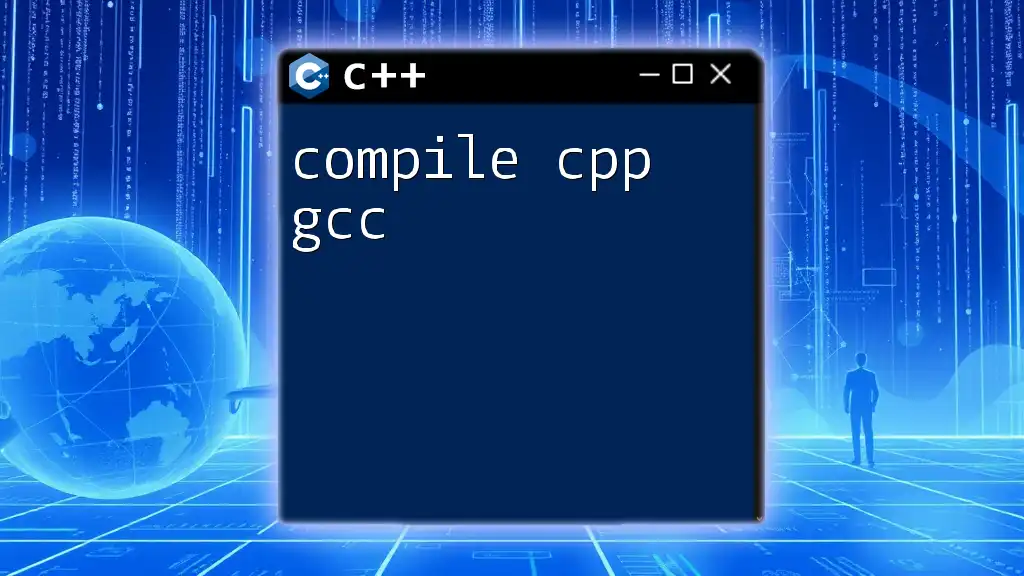
Advanced C++ Concepts
Templates
Templates allow the creation of functions and classes that work with any data type, promoting code reusability and flexibility. Here’s an example of a template function:
template <typename T>
T add(T a, T b) {
return a + b;
}
This template function `add` can work with integers, floats, and more, based on the data type supplied during function invocation.
Exception Handling
Robust applications require handling unexpected errors. C++ provides a standardized way to manage errors through exceptions. Here’s a basic example:
try {
throw runtime_error("An error occurred");
} catch (const exception& e) {
cout << e.what() << endl;
}
In this snippet, we throw a runtime error and catch it using a try-catch block, showcasing how to ensure program stability even in the face of errors.
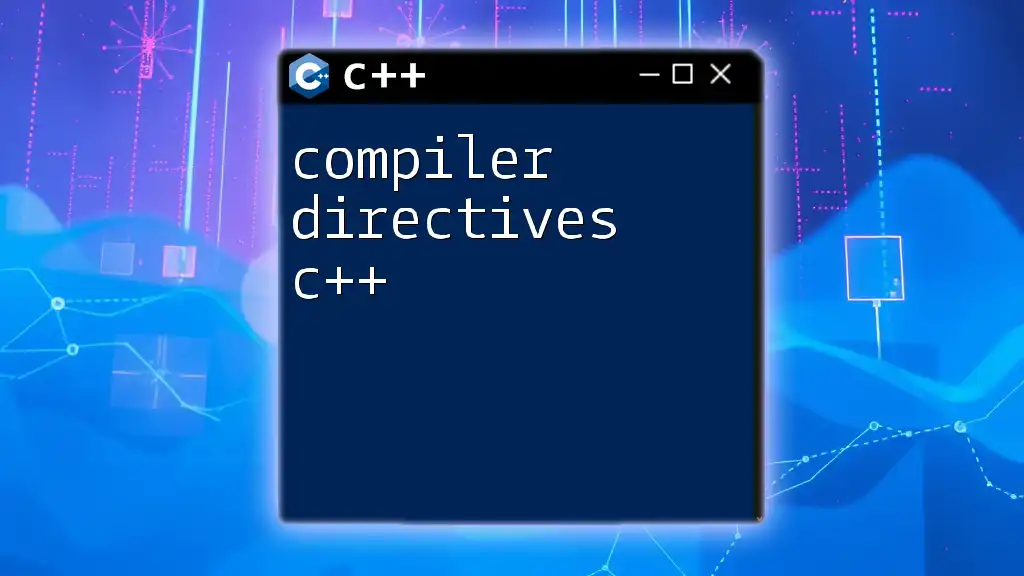
Common Practices and Challenges in C++
Writing Clean Code
Writing clean, maintainable code is crucial for long-term project success. Key practices include:
- Commenting: Provide context and explanations for complex logic.
- Naming Conventions: Choose meaningful names for variables, functions, and classes to convey their purpose clearly.
Every little detail contributes to code quality, making collaboration and future modifications easier.
Debugging and Testing
Debugging is an integral part of the software development lifecycle. Popular debugging tools like GDB, Valgrind, and integrated IDE debuggers help identify and fix issues in C++ applications. Engaging in unit testing is also invaluable, reinforcing code reliability through automated tests.
Frequently Encountered Problems and Solutions
Developers often face challenges in C++, such as memory management issues and syntax pitfalls. Understanding the importance of pointers, smart memory management practices, and strict adherence to syntax rules can help mitigate these problems.
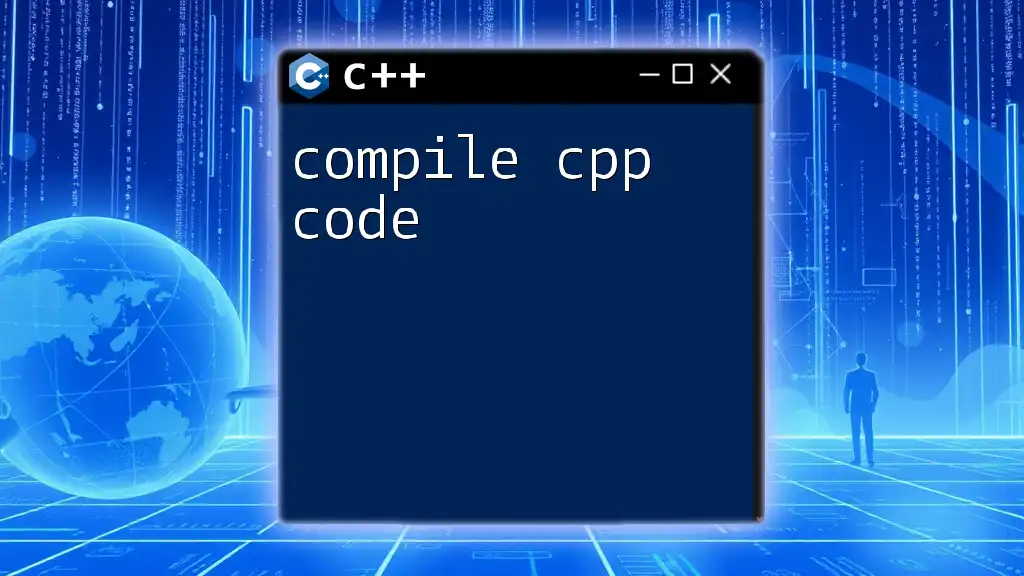
Conclusion
In summary, C++ remains a cornerstone of computer science, enabling the development of efficient and high-performance applications. Its blend of procedural and object-oriented programming makes it versatile across multiple domains. By mastering the key principles, syntactical structures, and advanced features of C++, you position yourself competitively in the ever-evolving tech landscape.
To further your journey, consider exploring additional resources and courses focusing on C++ to continue refining your programming expertise. Engage with community forums, participate in coding challenges, and practice regularly to enhance your skills. As you progress, you’ll find that knowledge of computer science CPP opens doors to countless opportunities in the field of technology.