Escape sequences in C++ are special character combinations that represent non-printable characters or allow the insertion of characters that would otherwise be difficult to include in strings, such as newline or tab characters.
Here's a code snippet demonstrating some common escape sequences in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!\n"; // Newline
std::cout << "Tabbed\tText\n"; // Tab
std::cout << "Backslash \\ and Quote \"\n"; // Backslash and double quote
return 0;
}
What is an Escape Sequence in C++?
An escape sequence in C++ is a series of characters that serve a special function in a string literal. Typically, these sequences are used to represent certain characters that may be difficult or impossible to include directly in the text. Examples include newline characters, tabs, quotes, and backslashes. Understanding escape sequences is essential for writing clear and effective C++ programs, as they enhance code clarity and facilitate smooth output formatting.

Common C++ Escape Characters
The Basics of Escape Characters
C++ provides several escape characters that allow developers to manipulate strings creatively and effectively. Here’s a brief overview of the most common escape sequences:
- `\n`: Inserts a new line in the output.
- `\t`: Adds a tab space.
- `\\`: Displays a backslash character.
- `\"`: Represents a double quote within a string.
- `\'`: Represents a single quote within a string.
Code Snippets and Examples
Utilizing escape characters can enhance the output of your program. Here's an illustrated example that demonstrates how to use various escape sequences:
#include <iostream>
using namespace std;
int main() {
cout << "Hello World!\n"; // New line
cout << "Tab\tSpace\n"; // Tab space
cout << "Backslash: \\\n"; // Displaying a backslash
cout << "Double quote: \""; // Using double quotes
cout << "Single quote: \'\n"; // Using single quotes
return 0;
}
In this example, you can see how different escape sequences directly influence the formatting of output in the console, making it more readable and visually organized.

Advanced Escape Sequences in C++
Octal and Hexadecimal Escape Sequences
Apart from common escape characters, C++ also supports octal and hexadecimal escape sequences. Octal sequences begin with a backslash followed by up to three octal digits (0-7), while hexadecimal sequences start with the prefix `\x` followed by hexadecimal digits (0-9, A-F).
Such sequences are useful when you need to represent characters whose ASCII values are cumbersome to type out. Here's how you could use them:
#include <iostream>
using namespace std;
int main() {
cout << "Octal 116: \116\n"; // Outputs the character 'N'
cout << "Hex 41: \x41\n"; // Outputs the character 'A'
return 0;
}
This example illustrates how these escape sequences can succinctly denote specific characters, showcasing a different level of control over textual representation.
Unicode Escape Sequences
For developers needing to incorporate special characters from various languages or symbols, Unicode escape sequences come in handy. These sequences start with `\u` for characters in the Basic Multilingual Plane (BMP) or `\U` for characters outside the BMP.
Here's a simple example of how Unicode escape sequences can be utilized in a C++ program:
#include <iostream>
using namespace std;
int main() {
cout << "Unicode Character: \u03A9\n"; // Outputs the Omega symbol: Ω
return 0;
}
Utilizing Unicode escape sequences allows for broader communication and representation of diverse character sets, enhancing the flexibility of your C++ programs.
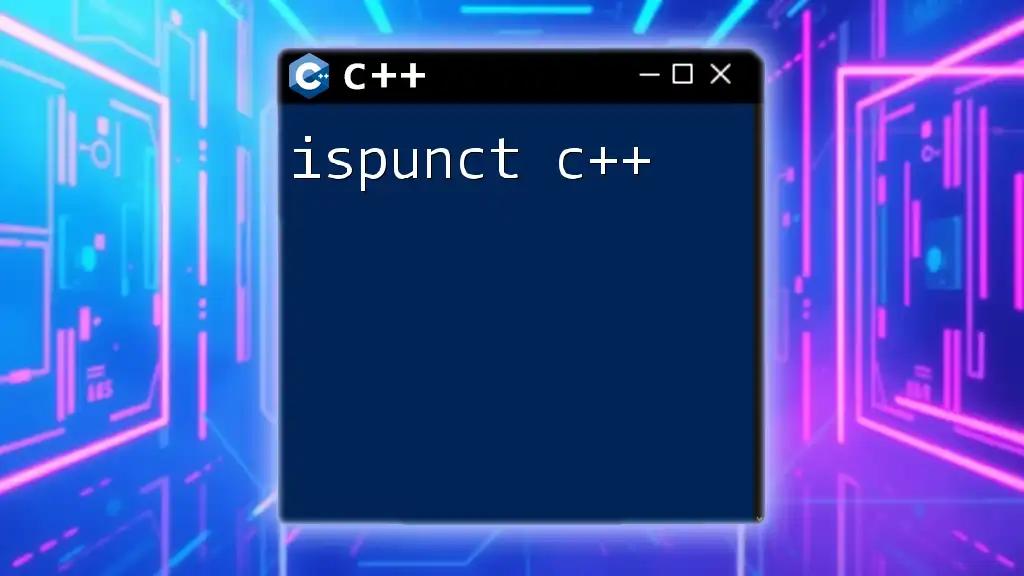
Practical Applications of Escape Sequences
Formatting Output
Formatting output is one of the primary advantages of using escape sequences. These sequences enable developers to structure console outputs, making the information more accessible to users. For instance, tabs can create orderly alignments, while new lines can separate sections of output for clarity.
Consider the following example demonstrating formatted output utilizing escape sequences:
#include <iostream>
using namespace std;
int main() {
cout << "Name:\tJohn Doe\n";
cout << "Age:\t30\n";
return 0;
}
In this instance, you'll notice how tabs and new lines make the output easier to read. This creates a structured layout for users to digest the information without unnecessary clutter.
File Paths and Escape Sequences
Another crucial application of escape sequences is in specifying file paths, particularly in Windows environments, where the backslash (`\`) is used as a directory separator. To avoid confusion with escape sequences, you must use double backslashes.
Here's how to correctly represent file paths using escape sequences:
#include <iostream>
using namespace std;
int main() {
cout << "File path: C:\\Users\\John\\Documents\n";
return 0;
}
Here, the use of `\\` allows the program to print a valid file path without misinterpreting the backslashes as escape characters, ensuring the output remains intelligible.
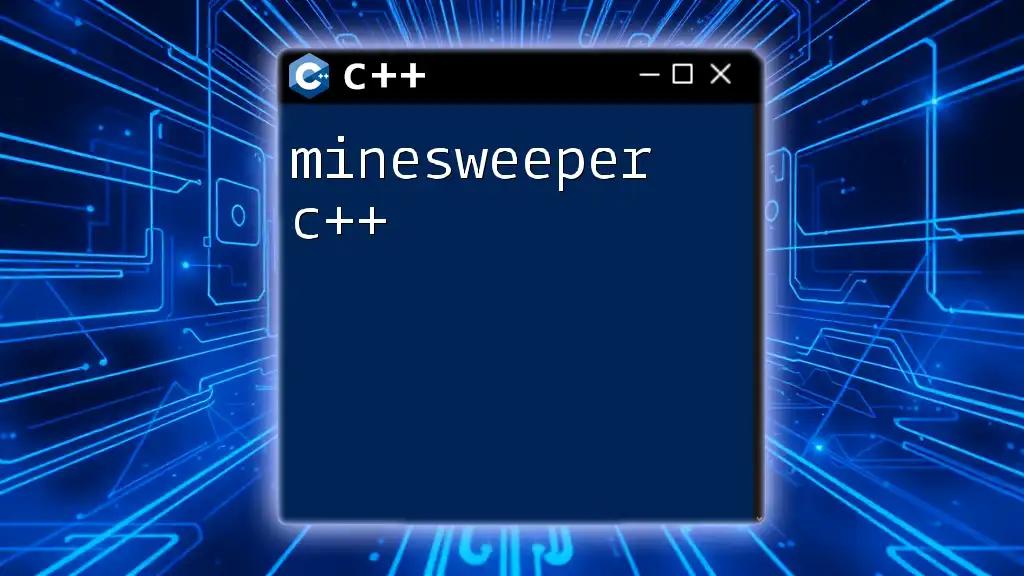
Tips and Best Practices
When working with escape sequences in C++, maintaining readability is critical. Here are some best practices:
- Be consistent: Use escape sequences uniformly throughout your code.
- Review common sequences: Familiarize yourself with escape sequences to avoid confusion.
- Comment your code: If your usage of escape sequences is not straightforward, consider adding comments for clarity.
At the same time, be wary of common pitfalls. For instance, always remember that a single backslash at the end of a string may lead to unexpected behavior, potentially causing errors.
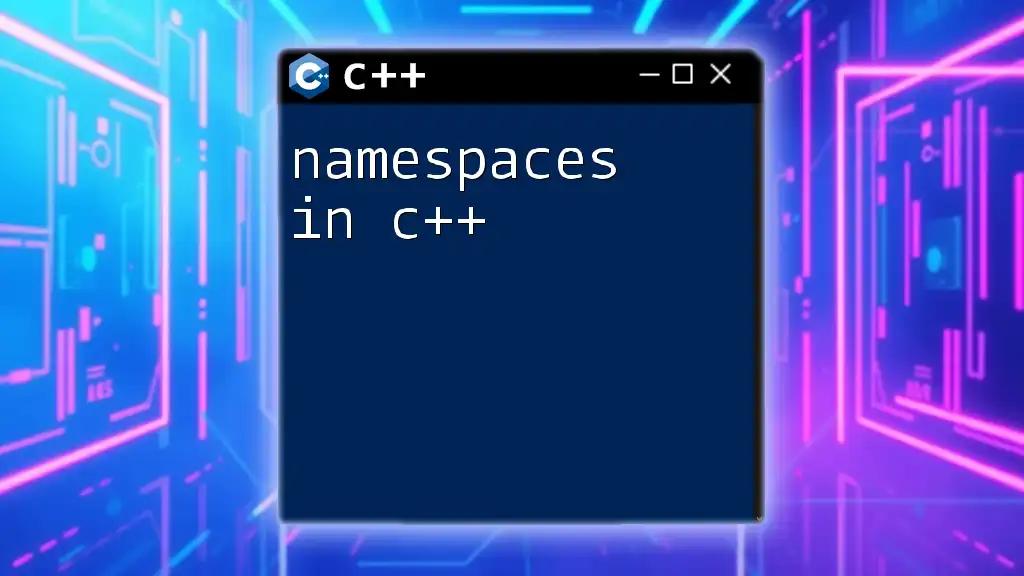
Conclusion
In conclusion, understanding escape sequences in C++ is essential for any programmer aiming to write clear and efficient code. These sequences enhance the readability of strings and the clarity of console output while preventing confusion with special characters. Embrace the practice of using escape sequences in your C++ projects to improve both your coding efficiency and the user experience. As you continue your programming journey, remember that the mastery of these sequences will benefit your overall skill set in developing robust C++ applications.