A cyclic dependency in C++ occurs when two or more classes depend on each other, creating a loop that can cause compilation errors.
Here’s a simple example to illustrate this concept:
// Example of cyclic dependency in C++
class B; // Forward declaration
class A {
public:
void funcB(B* b);
};
class B {
public:
void funcA(A* a);
};
void A::funcB(B* b) {
// Implementation
}
void B::funcA(A* a) {
// Implementation
}
In this example, class `A` and class `B` reference each other, leading to a cyclic dependency.
What is a Circular Dependency in C++?
Circular dependency refers to a situation where two or more modules, classes, or components rely on each other either directly or indirectly, forming a closed loop. In C++, this often manifests when class A relies on class B, and class B, in turn, relies on class A, creating a cycle.
Real-World Examples
Consider two classes: `Customer` and `Order`. If `Customer` needs access to `Order` (e.g., to fetch order details) and `Order` also needs to reference `Customer` (e.g., to identify who placed the order), you have a cyclic dependency.
// In Customer.h
class Order; // Forward declaration
class Customer {
public:
Order* getOrder(); // Method that returns an Order object
};
// In Order.h
class Customer; // Forward declaration
class Order {
public:
Customer* getCustomer(); // Method that returns a Customer object
};
Here, both classes depend on each other, which can lead to complications as the project grows.
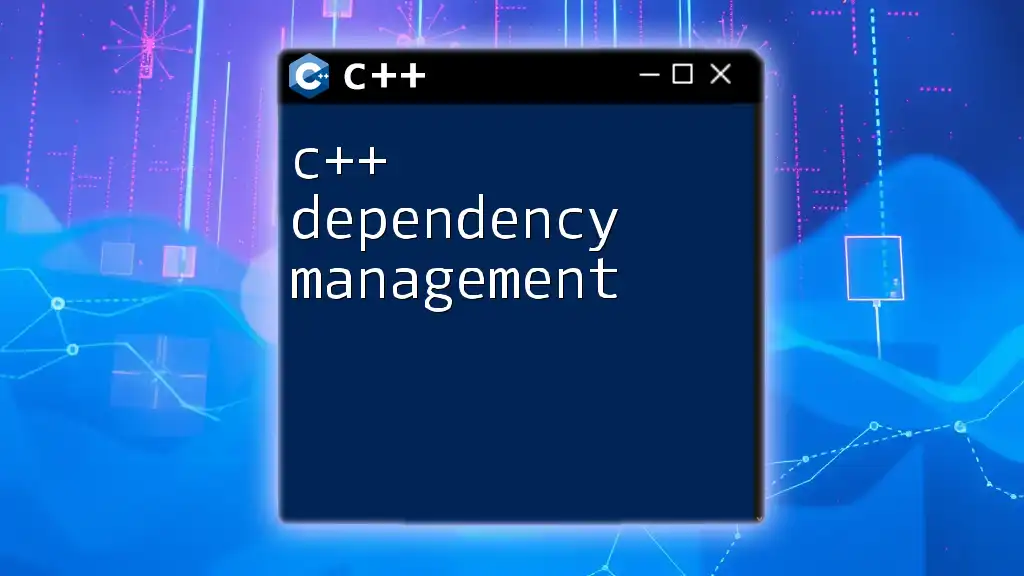
How Cyclic Dependencies Occur
Circular dependencies often arise due to inappropriate class design and poor management of header files.
Class Definitions and Header Files
C++ uses header files (`.h` files) to declare classes. If two classes are defined in each other's headers without careful structuring, it can lead to a situation where the compiler encounters an incomplete type. This is because the definitions it needs to compile the classes are not available at the time of compilation.
Inheritance and Interfaces
Inheritance can also create circular dependencies. If a base class and derived class reference each other, developers may find themselves in a cyclic trap.
For example, suppose `BaseClass` has a method that returns a type of `DerivedClass`, and `DerivedClass` inherits from `BaseClass`. This relationship can quickly become complex and difficult to manage.
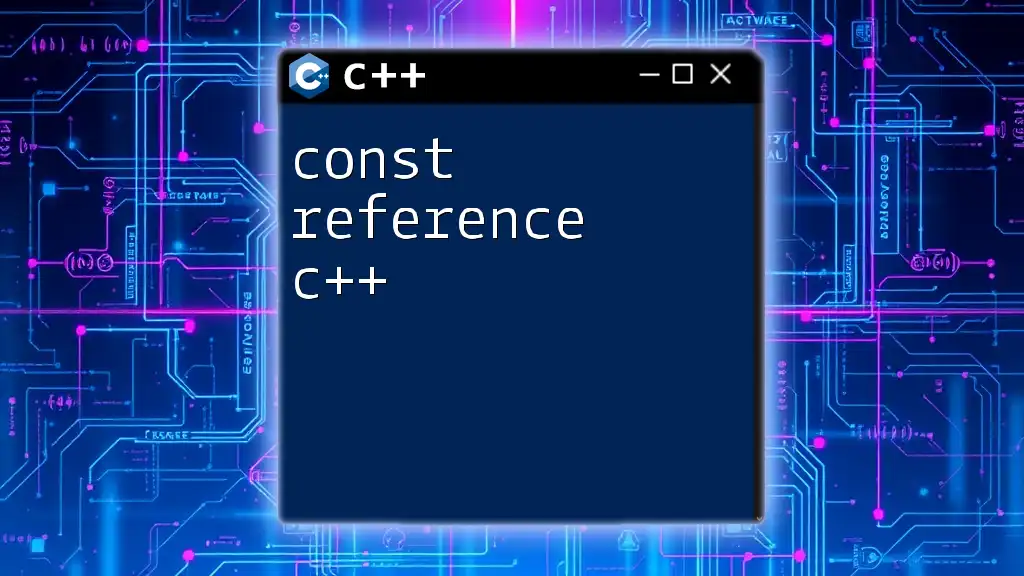
Negative Impacts of Cyclic Dependencies
Compilation Issues
Cyclic dependencies can lead to numerous compilation errors. When the compiler encounters a circular reference, it may not be able to resolve what it needs to instantiate objects or generate the necessary machine code. Common errors include:
- Incomplete type errors: This arises when the compiler sees a class type that hasn't been fully defined yet.
- Multiple definition errors: These occur when the same class or function is defined in multiple files due to improper linking.
Runtime Issues
Even if code compiles successfully, cyclic dependencies can introduce subtle runtime issues. For instance, they can lead to infinite loops if methods unintentionally call each other back and forth. Additionally, objects may fail to initialize correctly due to dependencies that cannot be resolved at runtime.
Maintenance Challenges
Code that contains circular dependencies can become incredibly difficult to maintain. Developers may struggle to understand the relationships between classes, leading to confusion and potential bugs. As a project evolves, these complications tend to exacerbate, slowing down development.
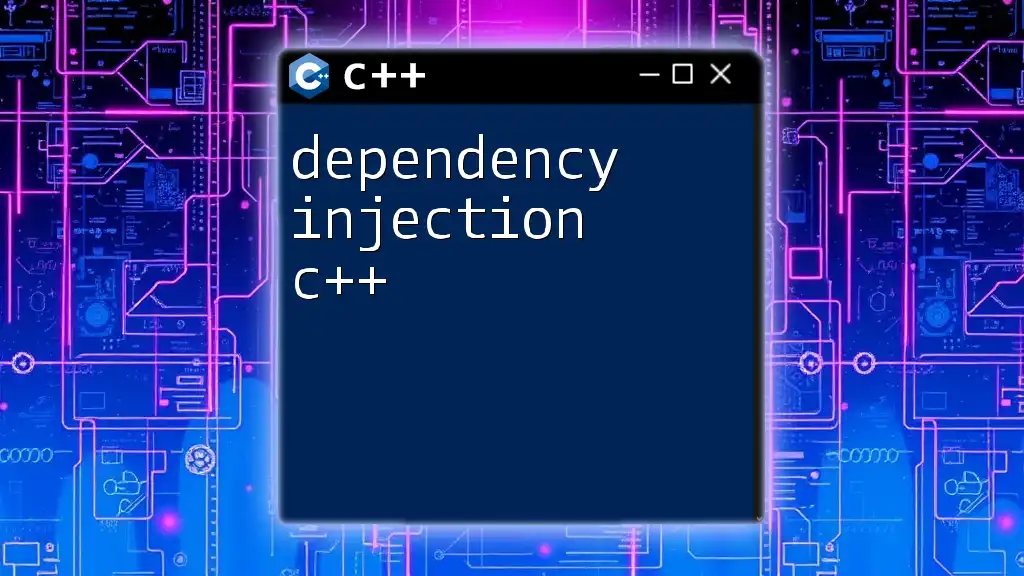
Identifying Circular Dependencies
Static Analysis Tools
To identify cyclic dependencies, developers can leverage static analysis tools. Tools like CMake, Clang Static Analyzer, and cppcheck can analyze code structure and flag possible circular dependencies.
Compiler Warnings and Errors
Common warnings and errors from the compiler can also indicate the presence of cyclic dependencies. These might include messages related to incomplete types, so keeping an eye on what the compiler reports can provide early warnings of potential issues.
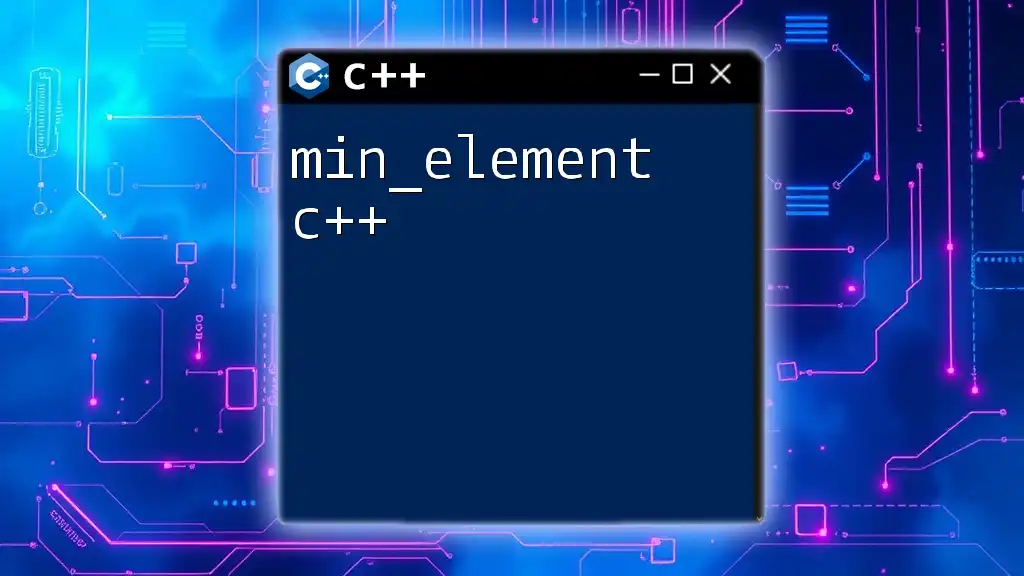
Techniques to Resolve Cyclic Dependencies
Redesigning Class Relationships
One powerful method for resolving cyclic dependencies is refactoring the class design. Streamlining class responsibility can often reduce the interdependencies between them. For example, instead of having `Customer` maintain a direct reference to `Order`, consider using a separate `OrderManager` class that handles order retrieval.
Using Composition Over Inheritance
Favoring composition over inheritance can often be a more flexible way of creating relationships between classes. This involves creating classes that manage the behavior of other classes rather than extending them.
Example:
class OrderManager {
public:
Customer getCustomer(Order order);
};
// Instead of:
class Order : public Customer {
public:
Customer* getCustomer();
};
Dependency Injection
Dependency injection is another technique to help mitigate cyclic dependencies. By injecting dependencies at runtime rather than having a class create them, you can break the cycle.
For example:
class CustomerService {
public:
void setOrderService(OrderService* orderService) {
this->orderService = orderService;
}
private:
OrderService* orderService;
};
Forward Declarations
Utilizing forward declarations can help address cyclic dependencies in header files. By declaring classes before referencing them, you can avoid incomplete type errors.
Example:
// In Customer.h
class Order; // Forward declaration
class Customer {
Order* order;
};
Split Header Files
Another effective strategy is to split header files to isolate dependencies. Creating separate headers for interface and implementation allows different parts of your code to compile independently, eliminating circular references.
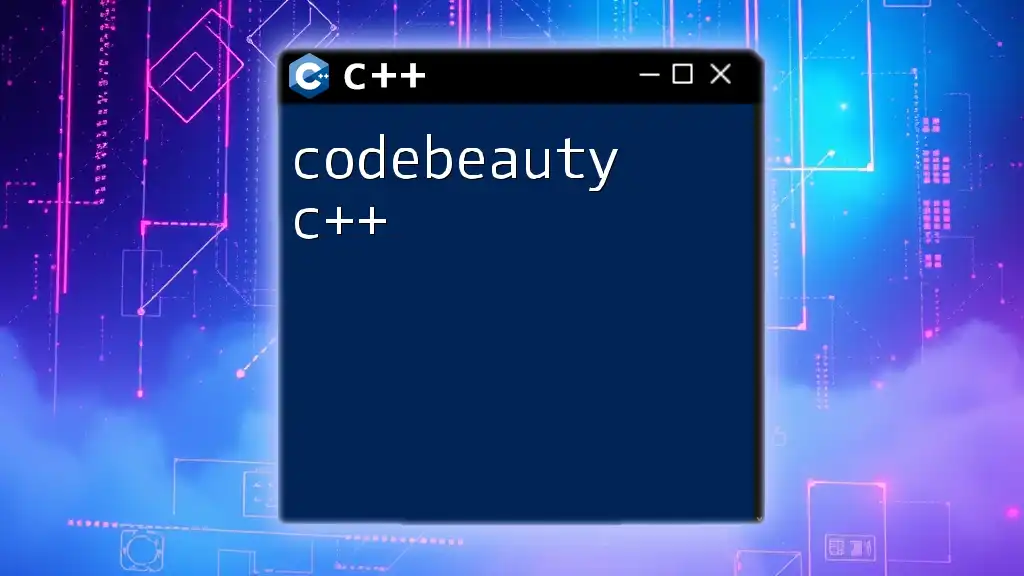
Best Practices to Avoid Cyclic Dependencies
Keep Your Classes Simple
Keeping classes simple with single responsibility principles makes them easier to manage and less likely to create circular dependencies. Aim for well-defined roles within each class.
Use Interfaces and Abstract Classes
Using interfaces and abstract classes allows for more flexible designs that can help avoid direct dependencies. They enable multiple class implementations without tightly coupling your code.
Regular Code Reviews
Incorporating regular code reviews into your workflow helps catch potential cyclic dependencies early. Peers can spot questionable relationships that may lead to circular dependencies.
Utilize Design Patterns
Employing design patterns can also be beneficial. Patterns such as the Observer Pattern or Mediator Pattern can facilitate communication between classes without direct references that can lead to cyclic dependencies.
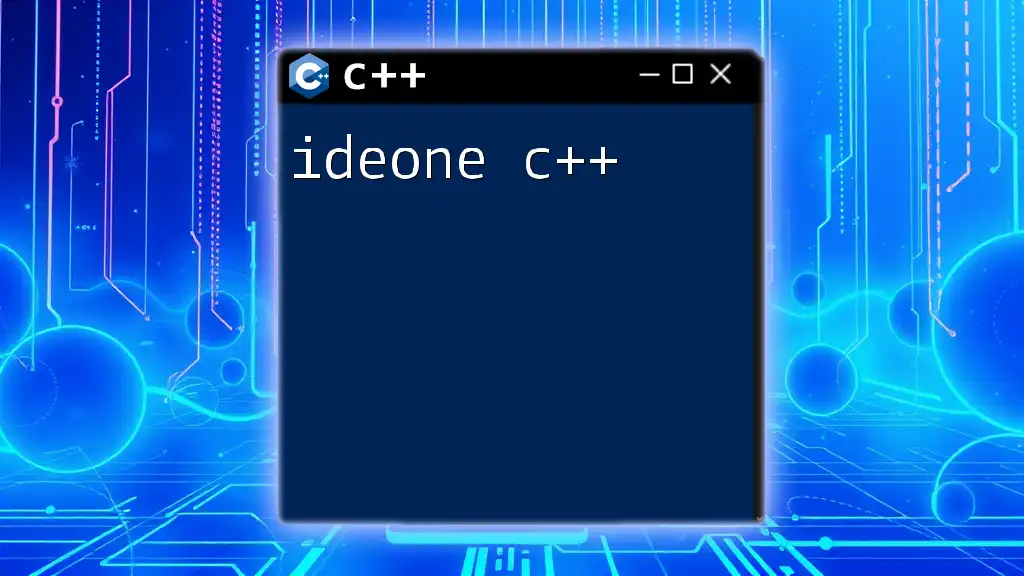
Conclusion
Understanding and managing cyclic dependencies is crucial for maintaining healthy C++ code. By implementing strategies like class redesign, dependency injection, and best practices, you can prevent the complications that arise from circular dependencies. Embracing these techniques allows your development process to remain clean, efficient, and maintainable.
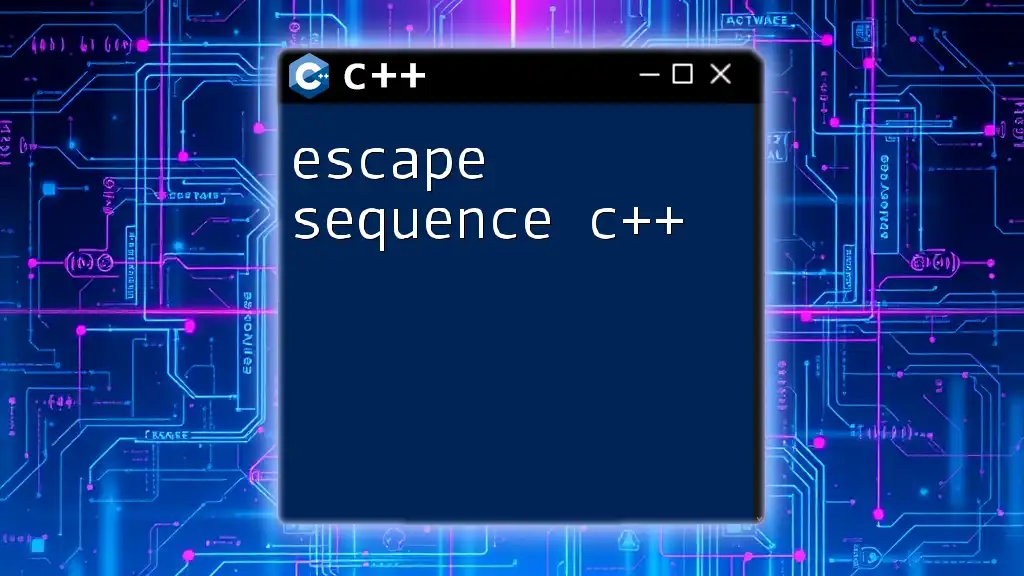
Call to Action
If you have encountered cyclic dependencies in your projects, we encourage you to share your experiences or ask questions. Join our community for more insights on how to master the complexities of C++ programming!