System design in C++ involves structuring and organizing your code to efficiently manage resources and improve maintainability, often using classes and design patterns to create scalable systems. Here's a simple example showcasing a class design for a Student management system:
class Student {
public:
Student(std::string name, int age) : name(name), age(age) {}
void displayInfo() {
std::cout << "Name: " << name << ", Age: " << age << std::endl;
}
private:
std::string name;
int age;
};
What is System Design?
System design refers to the comprehensive process of defining the architecture, components, modules, interfaces, and data for a system to satisfy specified requirements. It is crucial in software development as it helps in creating scalable, efficient, and maintainable systems. In the context of C++, which supports both low-level memory management and high-level abstractions, system design becomes particularly compelling and nuanced.
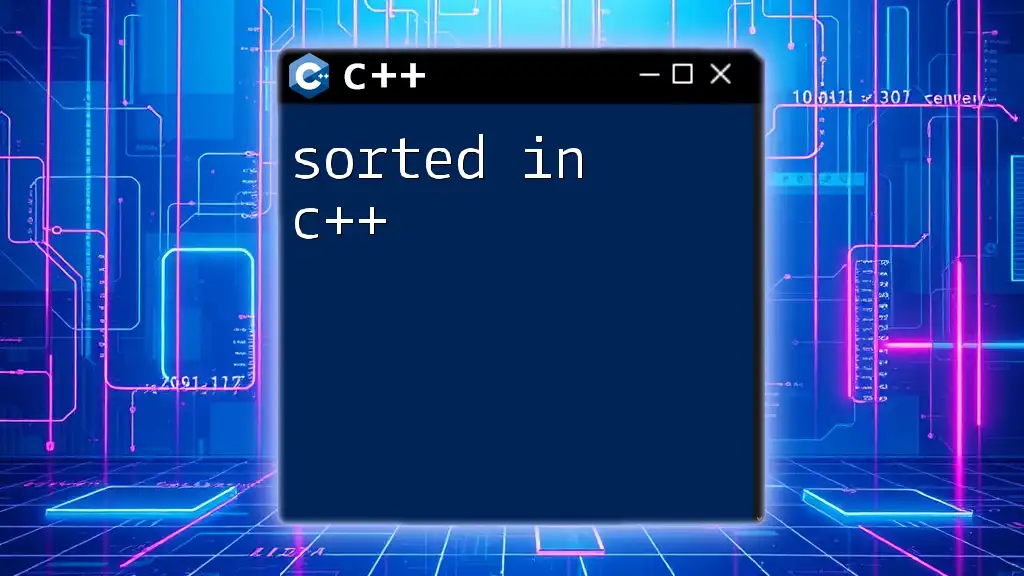
Why Use C++ for System Design?
C++ is a versatile language that combines the performance of low-level programming with powerful abstractions. It stands out due to:
- Performance Advantages: C++ compiles to machine code, which leads to faster execution times—a critical factor in system design where performance matters.
- Low-Level Memory Management: The ability to manipulate memory addresses allows for optimized resource usage, giving designers control over system performance.
- Object-Oriented Features: C++ supports OOP principles such as encapsulation, inheritance, and polymorphism, which enable better organization and reusability of code components.
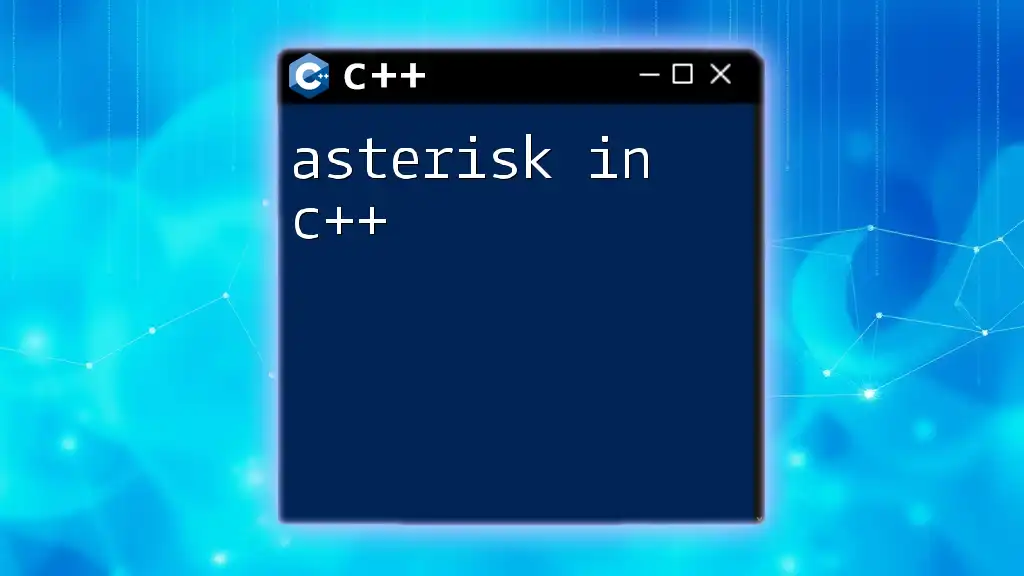
Core Principles of System Design
Understanding Requirements
Before diving into system design, it’s essential to fully understand your functional and non-functional requirements.
Gathering Functional Requirements
Functional requirements specify what the system should do. For example, in a stock trading application, functional requirements might include the ability to buy and sell stocks, track portfolio performance, and generate reports.
Gathering Non-Functional Requirements
These requirements define how the system should behave and include attributes like scalability and reliability. For instance, a trading application must be able to handle a surge in users during market opening periods while maintaining a response time of less than two seconds.
Designing for Scalability
Scalability is the capability of a system to handle growth, either by increasing resources (vertical scaling) or by adding more machines (horizontal scaling).
Implementing Scalability in C++
Design patterns play a crucial role in making systems scalable. For instance, the Singleton Pattern ensures that a class has only one instance and provides a global point of access to it. Consider the following code:
class Singleton {
public:
static Singleton* getInstance() {
if (!instance)
instance = new Singleton();
return instance;
}
private:
Singleton() {}
static Singleton* instance;
};
This pattern can be particularly useful for managing a shared resource like a database connection, ensuring that the application runs efficiently without unnecessary instantiations.
Designing for Reliability
Reliability refers to the ability of a system to function correctly even in the face of failures.
Error Handling in C++
Effective error handling is vital in building reliable systems. C++ supports structured exception handling, allowing developers to manage run-time errors gracefully. For example:
try {
// Some code that might throw
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << '\n';
}
By implementing robust error handling, you can ensure that your application does not crash unexpectedly, thus improving its reliability.
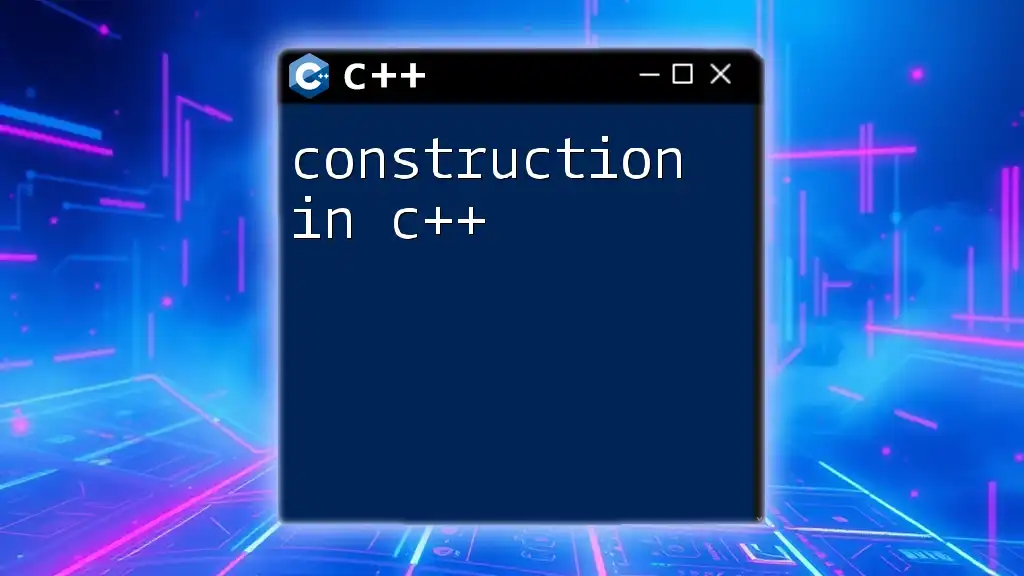
Key Components of System Design
Architecture Patterns
The architecture defines how the components of your system interact. Understanding various architecture patterns can influence your design choices.
Client-Server Architecture
In a client-server architecture, a server provides resources or services, and clients request services from it. This architecture is prevalent in web services, as it enables clear separation of responsibilities.
Microservices Architecture
Microservices divide an application into smaller, loosely coupled services that communicate over a network. This design enhances flexibility and scaling capabilities. C++ can be implemented in microservices using RESTful APIs or message brokers.
Data Management
Choosing the right data storage approach is essential for system functionality.
Choosing the Right Database
You can opt for either relational databases (like PostgreSQL) or non-relational databases (like MongoDB). Each has its strengths based on your application's requirements.
Code Example: Using SQLite in a C++ Application
SQLite can be an excellent option for lightweight data storage. Here’s how to set up a simple SQLite database in C++:
sqlite3* DB;
int exit = 0;
exit = sqlite3_open("test.db", &DB);
This code opens (or creates) a SQLite database named `test.db`, which can be utilized for various data management tasks.
User Interface Design
The user interface can be a command-line application or a graphical user interface (GUI). Each approach has its benefits, depending on the nature of the application.
GUI vs. Console Applications
While console applications are simpler and quicker to implement, GUIs improve user experience and accessibility. C++ libraries like Qt and wxWidgets are widely used for creating powerful GUIs.
Code Snippet: Simple GUI Creation Using Qt
Here’s an example of creating a basic window using Qt:
QWidget window;
window.setWindowTitle("Hello World");
window.show();
This snippet demonstrates how to set up a simple window, marking a step towards a user-friendly interface.
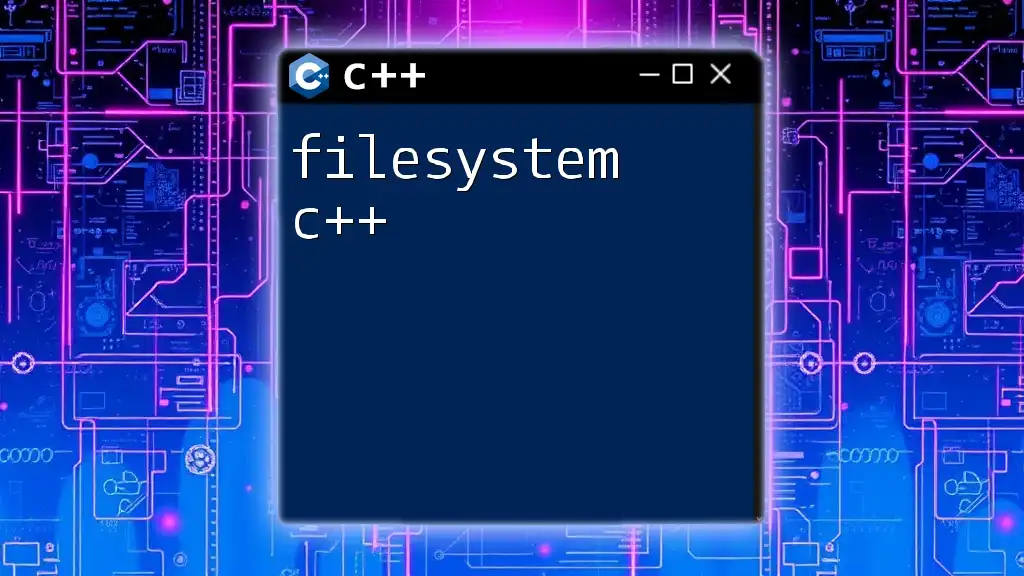
Case Studies
Case Study 1: Building a Simple Chat Application
In designing a chat application, it’s pivotal to gather requirements first, such as real-time messaging, user authentication, and message history. The architecture may follow a client-server model, where clients communicate through a dedicated server. The interaction can be implemented using C++ sockets for communication.
Case Study 2: Designing a Dynamic Library Management System
In a library management system, effective data handling is critical. Using C++, you could leverage STL containers such as `std::vector` or `std::map` for managing collections of books and user data. This case emphasizes the need for both functional (e.g., tracking book loans) and non-functional (e.g., system responsiveness) requirements.
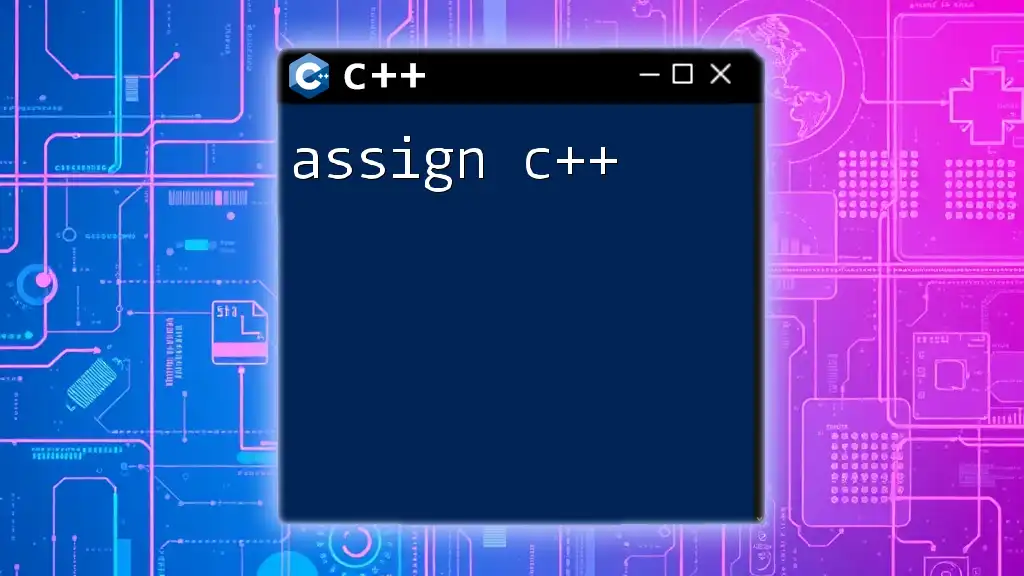
Best Practices in C++ System Design
Effective Use of Templates and Generics
C++ templates allow for the creation of functions and classes that can operate with any data type, facilitating type safety and code reusability. Here's a simple example:
template <typename T>
class Box {
public:
void set(T value) { this->value = value; }
private:
T value;
};
Using templates makes your code adaptable to different types without sacrificing performance.
Resource Management and Smart Pointers
Memory management is a critical aspect of C++. Utilizing smart pointers can prevent memory leaks and ensure that resources are handled appropriately. An example of a unique pointer is:
std::unique_ptr<MyClass> ptr = std::make_unique<MyClass>();
This automatically frees resources when the pointer goes out of scope, thus reducing the burden of manual memory management.
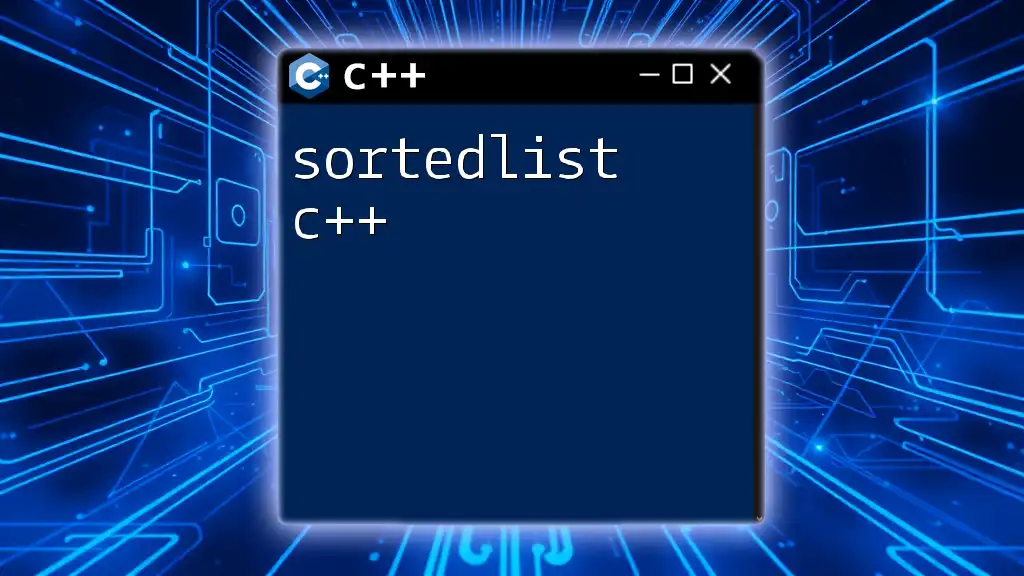
Conclusion
In summary, system design in C++ is a multifaceted process that requires a deep understanding of both the functional and non-functional aspects of the application. By leveraging C++’s strengths—its performance, control over memory, and powerful abstractions—you can create efficient, scalable, and reliable systems.
For aspiring system architects, continually learning through books, courses, and community forums is crucial to staying updated. Start building simple systems, experimenting with different architectures and patterns, and let C++ guide your design choices!