`iostream` in C++ is a standard library that enables input and output operations through streams, allowing developers to read from the console and write to the console easily.
Here's a simple example demonstrating its use:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding iostream in C++
What is iostream?
`iostream` is a part of the C++ Standard Library that facilitates input and output operations. It is essential for reading data from user input and displaying output on the screen. The name `iostream` comes from the combination of "input/output" and "stream," reflecting its purpose of managing data flow between the program and the external environment.
Components of iostream
-
Stream Classes: The `iostream` library consists primarily of three types of streams:
- `istream`: For input operations (reading data).
- `ostream`: For output operations (writing data).
- `iostream`: Combines both input and output functionalities.
-
Header Files: To use `iostream`, you must include the header file in your program with the following directive:
#include <iostream>
This inclusion provides access to the input and output stream classes and the related functions.
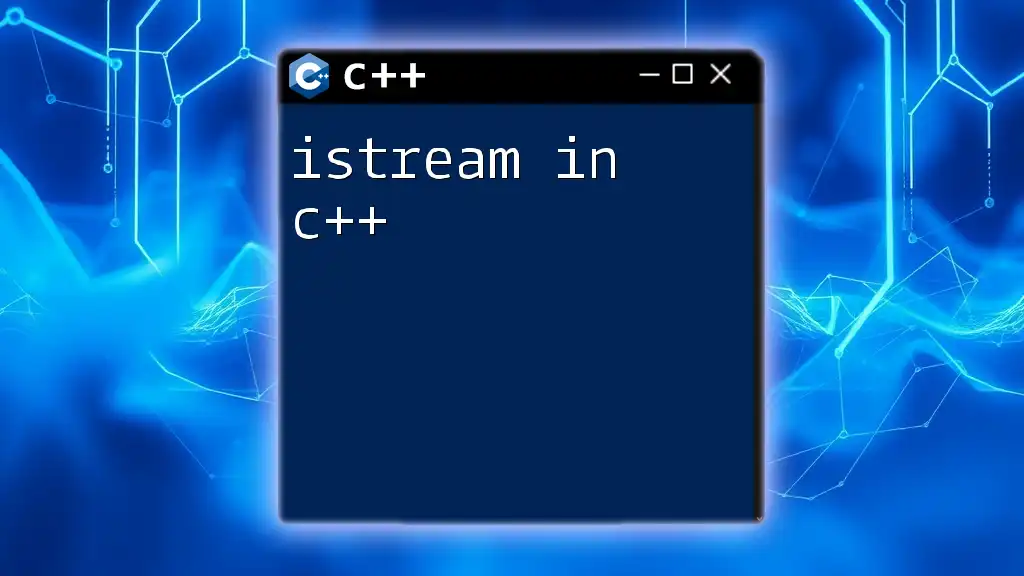
Using iostream in C++
Basic Input and Output Operations
Output with cout
In C++, `cout` (short for "character output") is used to send output to the console. Here’s a basic example:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl; // Outputs "Hello, World!" followed by a new line
return 0;
}
In this example, `endl` not only adds a new line but also flushes the output buffer. Flushing the buffer ensures that all output is displayed, which can be particularly useful when working with long-running processes.
Input with cin
Conversely, `cin` (short for "character input") allows you to read data from user input. Here's an example demonstrating how to take an integer input:
#include <iostream>
using namespace std;
int main() {
int number; // Declaring a variable to hold user input
cout << "Enter a number: ";
cin >> number; // Using cin to take input from the user
cout << "You entered: " << number << endl; // Displaying the entered number
return 0;
}
When using `cin`, it's important to ensure the input matches the expected data type. In the above example, we are expecting an integer input.
Stream Manipulators
Introduction to Manipulators
Manipulators are special functions that modify the behavior of input and output operations. They play a crucial role in formatting output for better readability.
Common Manipulators
- `std::endl`: As mentioned, `std::endl` inserts a newline character and flushes the output buffer. It is useful but can be slower than simply using `\n` when frequent flushing isn't necessary.
- `std::fixed` and `std::setprecision`: These manipulators are utilized to control the formatting of floating-point output. Here’s an example of how to use them:
#include <iostream>
#include <iomanip> // For std::fixed and std::setprecision
using namespace std;
int main() {
double number = 10.12345; // A sample floating-point number
cout << fixed << setprecision(2) << number << endl; // Outputs 10.12
return 0;
}
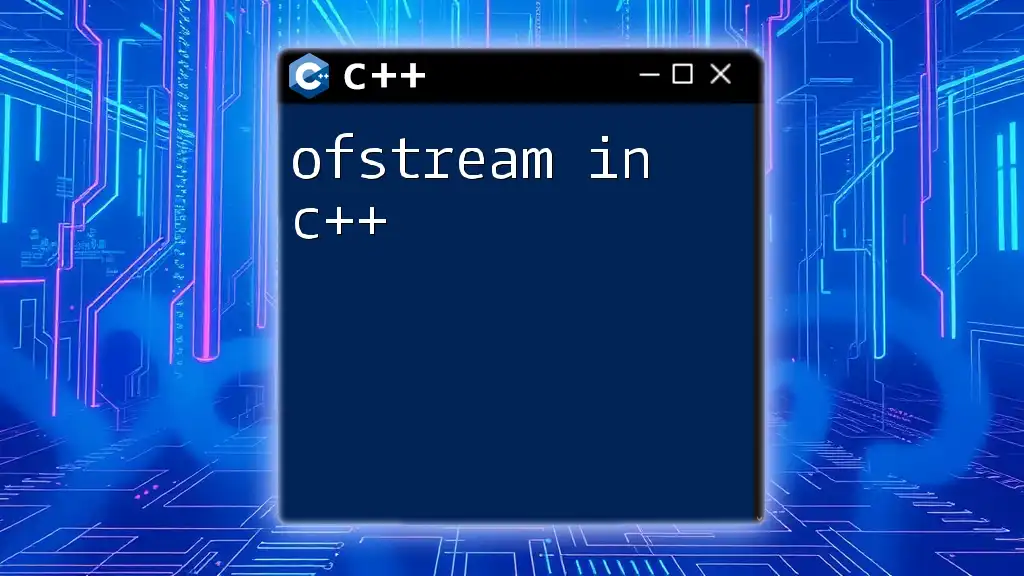
Advanced iostream Features
File I/O with iostream
File input/output is essential for reading from and writing to files, which allows for data persistence beyond program execution. To perform file operations, you can use the `fstream` library.
Reading from Files
To read data from a file, you can use `ifstream`, which stands for "input file stream." Here’s how to do it:
#include <iostream>
#include <fstream> // For file handling
using namespace std;
int main() {
ifstream inputFile("data.txt"); // Opening a file for reading
string line; // A variable to hold each line of the file
while (getline(inputFile, line)) { // Reading file line by line
cout << line << endl; // Outputting each line to the console
}
inputFile.close(); // Closing the file
return 0;
}
This code opens a file named `data.txt` and reads its contents line by line, displaying each line to the console.
Writing to Files
Similarly, you can write data to files using `ofstream`, which stands for "output file stream." Here’s an example:
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ofstream outputFile("data.txt"); // Opening a file for writing
outputFile << "Hello, File!" << endl; // Writing a line to the file
outputFile.close(); // Closing the file
return 0;
}
This example creates (or overwrites) a file named `data.txt` and writes a line of text into it.
Error Handling in iostream
Robust code requires handling possible errors. When using `cin`, it’s essential to check if the input operation was successful. Here's how you can do this:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
if (!(cin >> number)) { // Check for input errors
cout << "Invalid input!" << endl; // Inform the user of invalid input
cin.clear(); // Clear the error state of cin
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Ignore the invalid input
}
return 0;
}
In this example, if the user enters invalid data (such as text when an integer is expected), the program will handle the error gracefully instead of crashing.
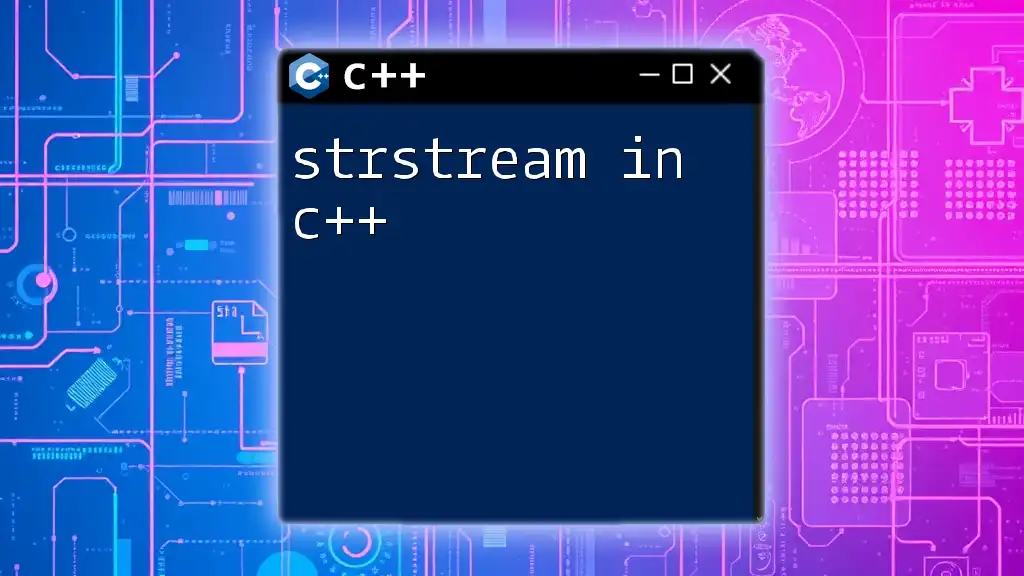
Best Practices for Using iostream
- Always Validate Input: Implement error checking to ensure that user input is in the expected format.
- Use `std::endl` Judiciously: While `std::endl` is useful, consider using `\n` for better performance when you do not need to flush the output buffer.
- Closing Files: Always close files after completing operations to free up system resources.
- Comment Your Code: Provide clear explanations of your input/output operations for better maintainability.
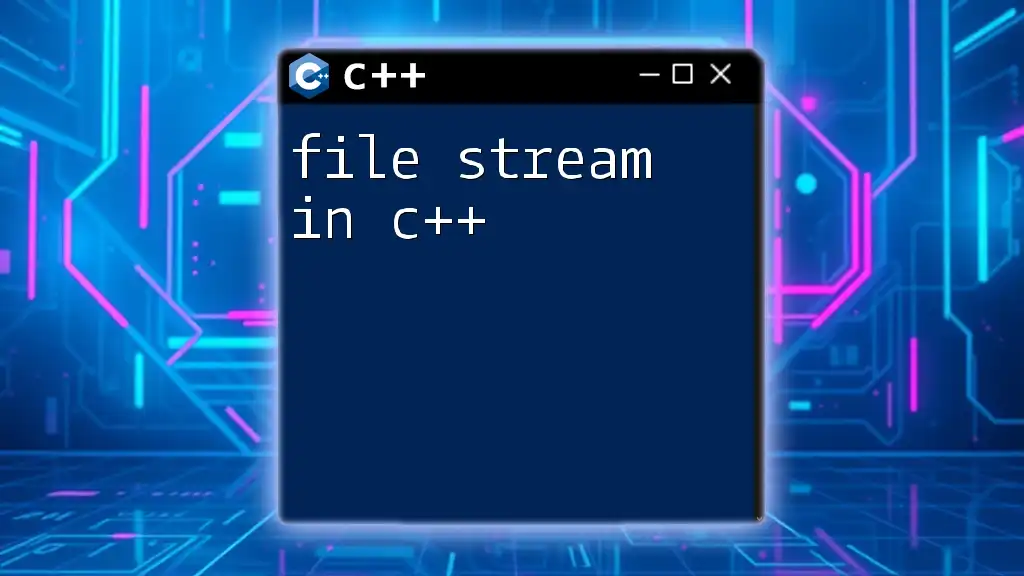
Conclusion
In conclusion, mastering iostream in C++ is essential for effective programming. From basic input/output operations to advanced file handling and error management, the `iostream` library provides the necessary tools to interact with users and files efficiently. With the knowledge of stream classes, manipulators, and error handling techniques, you can enhance your coding skills and create more robust applications. Start experimenting with the examples provided, and you’ll soon find yourself proficient in using iostream for all your C++ programming needs.
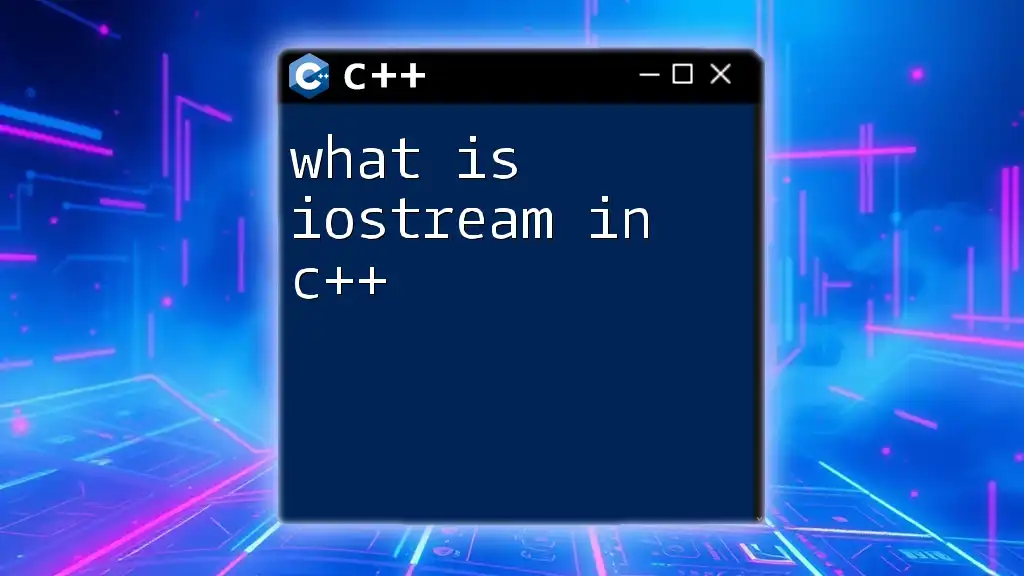
Additional Resources
For further learning and enhancement of your C++ skills, consider exploring recommended books, online tutorials, and the official C++ documentation on iostream. Dive into practical projects to apply what you've learned and solidify your understanding of input and output in C++.