`strstream` in C++ is a class that allows the use of string streams for manipulating strings as if they were I/O streams, making it easier to read from and write to strings.
Here's a simple example of using `strstream`:
#include <strstream>
#include <iostream>
int main() {
char str[20];
strstream s(str, sizeof(str));
s << "Hello, World!" << s.rdbuf();
std::cout << str << std::endl;
return 0;
}
Understanding `strstream`
Definition of `strstream`
`strstream` is a C++ library class that provides input and output for string buffers. Acting as a bridge between C-style strings and C++ I/O streams, it allows programmers to treat strings as streams, facilitating easier input and output operations.
Historical Context
Originally part of the C++ Standard Library, `strstream` has been around since the early days of the language. It was a common choice for manipulating strings until the introduction of `stringstream`. Since then, it is often recommended to use `stringstream` due to its enhanced safety and compatibility with C++ best practices.
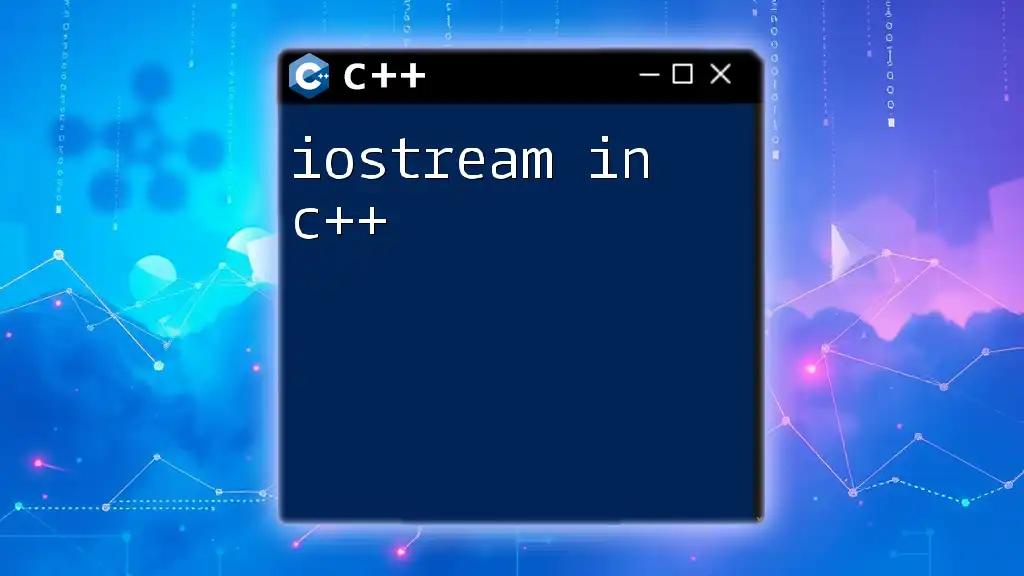
Core Features of `strstream`
Basic Constructs
A `strstream` is constructed similarly to other streams. It offers functionalities like str(), write(), and read(). Each of these functions allows you to interact with the internal buffer of the `strstream` object efficiently.
For example:
#include <strstream>
strstream ss;
ss << "Example text";
char buffer[50];
ss >> buffer; // Reads into the buffer
Managing Memory
Unlike modern alternatives, `strstream` internally allocates memory dynamically. While this brings flexibility, it comes with potential drawbacks, such as memory leaks if not carefully managed. The performance implications can vary based on use, so understanding how memory is allocated and deallocated is crucial.

Using `strstream` in C++
Creating a `strstream` Object
To create a `strstream` object, you simply include the necessary header and declare an instance:
#include <strstream>
strstream ss;
Once you've initialized the `strstream`, you can begin to manipulate data.
Writing to `strstream`
You can write to a `strstream` using the insertion operator (<<). For example:
ss << "Hello, World!";
When writing strings or other data types, make sure to terminate with `ends` to signal the end of the data:
ss << "Hello, World!" << ends; // Ensures null termination
A complete example demonstrates writing to a `strstream` and displaying the contents:
#include <iostream>
#include <strstream>
int main() {
strstream ss;
ss << "Hello, " << "World!" << ends;
std::cout << ss.str(); // Outputs: Hello, World!
return 0;
}
Reading from `strstream`
Reading from `strstream` is straightforward. You can use the extraction operator (>>). Here is an example:
int number;
ss >> number;
Below is a complete example that demonstrates reading data from `strstream`:
#include <iostream>
#include <strstream>
int main() {
strstream ss;
ss << "42";
int num;
ss >> num;
std::cout << "Number: " << num; // Outputs: Number: 42
return 0;
}
Manipulating Data Types
`strstream` supports multiple data types, including integers, floating-point numbers, and strings. Be cautious with type conversions; ensure that the types match during extraction to avoid unexpected behavior.
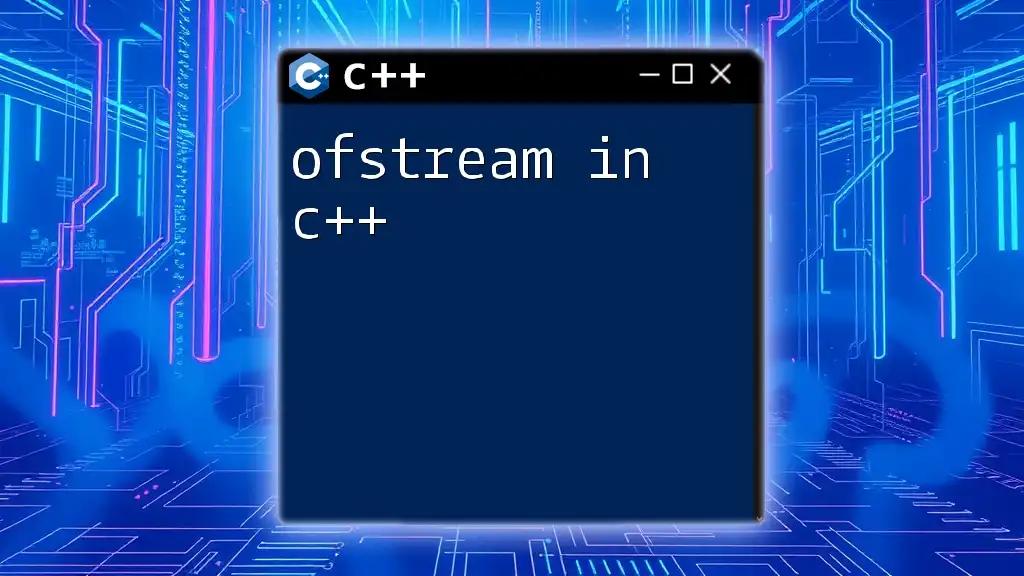
Considerations When Using `strstream`
Performance Implications
Performance can become a concern when using `strstream`, especially if you're frequently manipulating large amounts of data or performing complex operations. In many cases, switching to `std::stringstream`—which is safer and better integrated with C++'s type safety—might be a worthwhile consideration.
Deprecated Status
Despite its historical significance, `strstream` is considered deprecated in modern C++. The primary reasons include issues related to type safety and better alternatives being available. Migrating to `std::stringstream` is highly recommended; it offers similar capabilities with enhanced safety features.

Practical Examples and Scenarios
Case Studies
One common use case for `strstream` is formatting output data. For instance, when preparing a log message, manipulating strings using a `strstream` allows for dynamic content.
Another instance is temporary string manipulation, such as when creating custom messages for GUI elements without creating permanent string objects. This enables efficient transitions and reduces memory overhead.
Interactive Coding Examples
Here’s a small task: create a program that reads a user's input for name and age and then formats that data for display using `strstream`.

Common Errors and Debugging Tips
Error Handling
Be aware of common mistakes, such as underestimating character buffer sizes or improperly managing memory that can lead to segmentation faults. Always ensure your buffers are adequately sized and consider using `std::fixed` with proper formatting when dealing with floating-point output.
Debugging Techniques
When debugging `strstream`, check for issues like incorrect use of `ends`, improper data extraction, and type mismatches. Utilizing debugging tools or simply printing intermediary results can help pinpoint areas of concern.
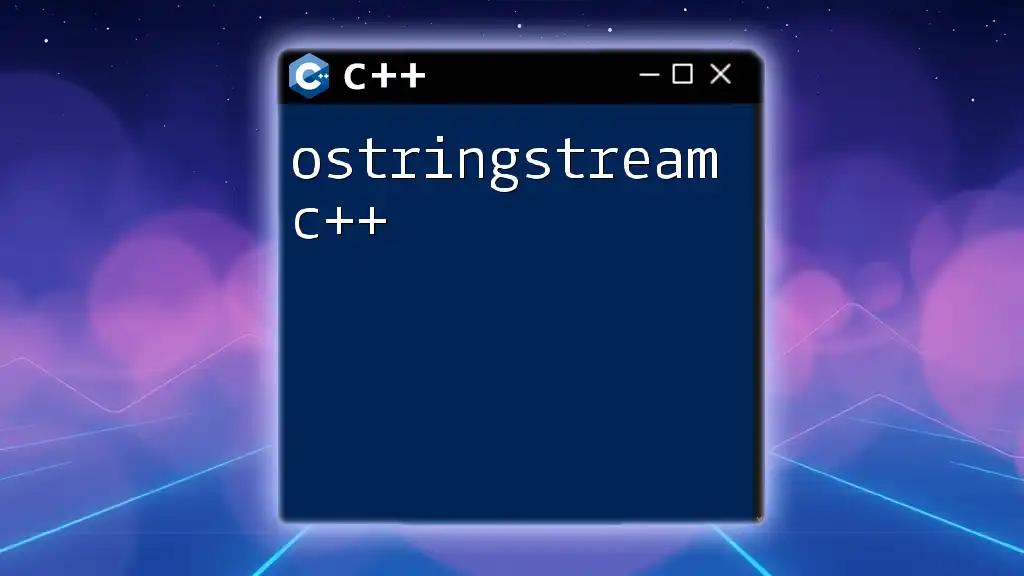
Conclusion
In summary, understanding how to work with strstream in C++ can enhance your string manipulation capabilities, though it is crucial to be aware of its deprecated status and potential pitfalls. Transitioning to `std::stringstream` will provide a more robust solution for modern C++ programming needs.
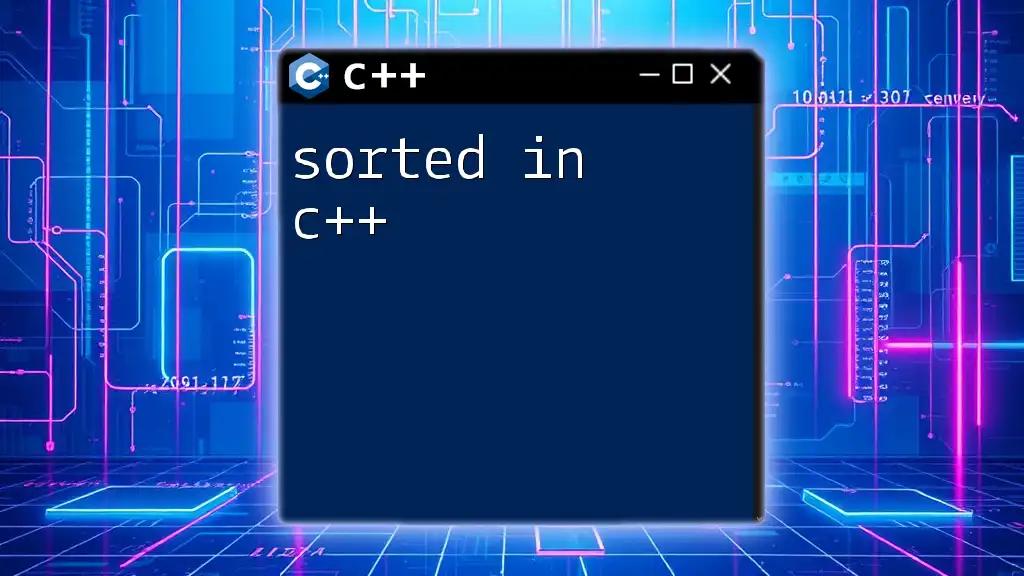
Further Reading and Resources
For those looking to deepen their understanding of C++ stream handling, consider exploring relevant textbooks, online courses, and forums where you can engage with a community of programmers. These resources often provide tutorials that cover both traditional and modern uses of string handling techniques in C++.

Call to Action
Now that you've learned about `strstream`, try experimenting with it in your own projects! If you have questions or thoughts on this topic, feel free to reach out or leave comments to share your experiences.