In C++, construction refers to the process of creating and initializing an object of a class using a constructor, which is a special member function that is automatically called when an object is instantiated.
class MyClass {
public:
MyClass() { // Constructor
// Initialization code
}
};
int main() {
MyClass obj; // Object construction
return 0;
}
What is Construction in C++?
Definition of Constructor
In C++, a constructor is a special member function that gets called automatically when an object of a class is created. Its primary role is to initialize the object. Constructors allow you to set initial values for data members, allocate resources, or perform any startup tasks required for the object. For instance, consider the following simple constructor:
class Example {
public:
Example() {
// Constructor code
}
};
Types of Constructors
There are three main types of constructors in C++: default, parameterized, and copy constructors.
- Default Constructor: This type of constructor does not take any parameters and can initialize object attributes to default values or perform basic setup. Here's a simple example:
class Dog {
public:
Dog() {
// Initialization
breed = "Unknown";
}
private:
std::string breed;
};
A default constructor is utilized when the user does not provide any specific initialization parameters.
- Parameterized Constructor: This constructor accepts arguments that allow setting initial values during object instantiation. For example:
class Dog {
public:
Dog(std::string dogBreed) {
breed = dogBreed;
}
private:
std::string breed;
};
By providing a breed when creating an object like `Dog myDog("Labrador");`, the constructor initializes the object with that breed.
- Copy Constructor: This type constructs a new object as a copy of an existing object, requiring the same class type as its argument. Here's an example:
class Dog {
public:
Dog(const Dog& other) {
breed = other.breed; // Copying breed
}
private:
std::string breed;
};
Copy constructors are particularly important for managing resources, ensuring proper deep copies, and preventing issues like double deletion.

The Construction Process
Step-by-step Object Creation
When an object is created in C++, it goes through a well-defined lifecycle. The construction process includes crucial steps such as memory allocation and the initialization phase.
-
Memory Allocation: When a constructor is invoked, memory for the object is allocated from the heap if the object is dynamically allocated, or from the stack if it’s statically allocated.
-
Initialization Phase: Following memory allocation, the constructor initializes member variables. This phase is critical for setting up an object in a valid state for its intended use.
Constructor Overloading
Constructor overloading is a feature that allows multiple constructors to coexist within a class, differing by their parameter list. This provides flexibility in how objects are initialized. Consider the following example:
class Person {
public:
Person() {
// No-arg constructor
}
Person(std::string name) {
this->name = name; // Initialization using a parameter
}
private:
std::string name;
};
With this setup, you can create a person object without passing any data, as well as initialize it with a name.
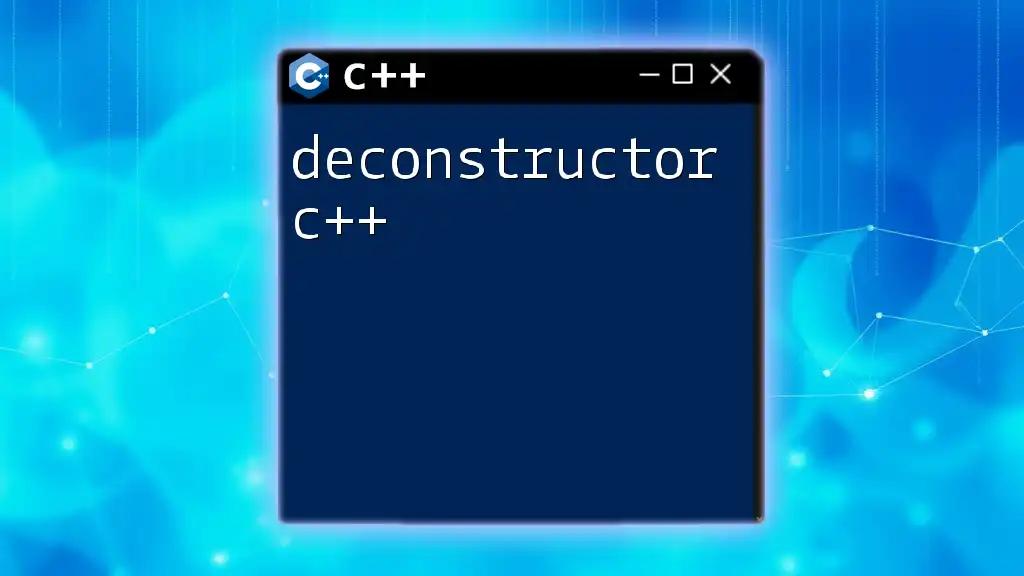
Destructor: The Flip Side of Construction
What is a Destructor?
A destructor is the reverse of a constructor, invoked when an object goes out of scope. It is responsible for cleaning up resources, deallocating memory, and ensuring that any necessary final actions are carried out. Here’s a simple destructor implementation:
class Dog {
public:
~Dog() {
// Cleanup code before the memory is released
}
};
Destructor as Part of Resource Management
Destructors play a vital role in managing resources. They help prevent memory leaks by releasing memory allocated for class attributes and other resources. Here’s an example showing both a constructor and destructor managing a dynamic resource:
class Array {
public:
Array(int size) {
this->size = size;
arr = new int[size]; // Dynamic memory allocation
}
~Array() {
delete[] arr; // Deallocation
}
private:
int* arr;
int size;
};
In this example, the destructor frees the dynamically allocated memory, preventing resource leaks.

Best Practices in Using Constructors
Initialization Lists
Using initialization lists in constructors improves performance, especially when initializing complex objects or constants. Initialization in the list avoids the extra step of assigning to variable after they are created. Here's an example:
class Dog {
public:
Dog(std::string breed) : breed(breed) {
// Constructor body can remain empty
}
private:
std::string breed;
};
This code is efficient and concise, setting the `breed` member directly through the initialization list.
Rule of Three
The Rule of Three states that if a class requires a custom destructor, copy constructor, or copy assignment operator, it likely requires all three. This ensures proper resource management. Here is an example illustrating this:
class Dog {
public:
Dog(const Dog& other) : breed(other.breed) {
// Copy Constructor
}
Dog& operator=(const Dog& other) {
if (this != &other) {
breed = other.breed; // Assignment operator
}
return *this;
}
~Dog() {
// Destructor
}
private:
std::string breed;
};
Implementing those provides a complete mechanism for copying and destructing objects safely.

Common Pitfalls in Constructor Usage
Default Initialization of Members
A common pitfall occurs when member variables are left uninitialized due to using default constructors. This can lead to undefined behavior. For instance, if you have:
class Dog {
public:
Dog() {
// No initialization
}
private:
std::string breed; // Uninitialized
};
This situation can cause issues when you try to use `breed` without assigning it a value.
Complex Initialization Scenarios
When initializing complex data members, such as other objects or dynamic resources, it’s essential to manage initialization carefully. Here's an example:
class Owner {
public:
Owner() : pet("Unknown") {
// Complex data member initialization
}
private:
Dog pet; // Dog initialized within Owner's constructor
};
Proper attention to these details can prevent many runtime errors and logical bugs.

Practical Examples
Real-world Application
Consider a simple class that simulates a bank account. Here's how you might construct such a class:
class BankAccount {
public:
BankAccount(std::string name, double balance) : owner(name), balance(balance) {
// Constructor with parameters
}
private:
std::string owner;
double balance;
};
Here, the bank account is initialized with an `owner` name and `balance` at the time of creation, demonstrating effective use of constructors.
Constructor Use Cases
Constructors are integral in creating well-structured, readable code. They help encapsulate logic behind object initialization, enabling clear class design. Utilizing constructors efficiently can lead to more maintainable and understandable code.
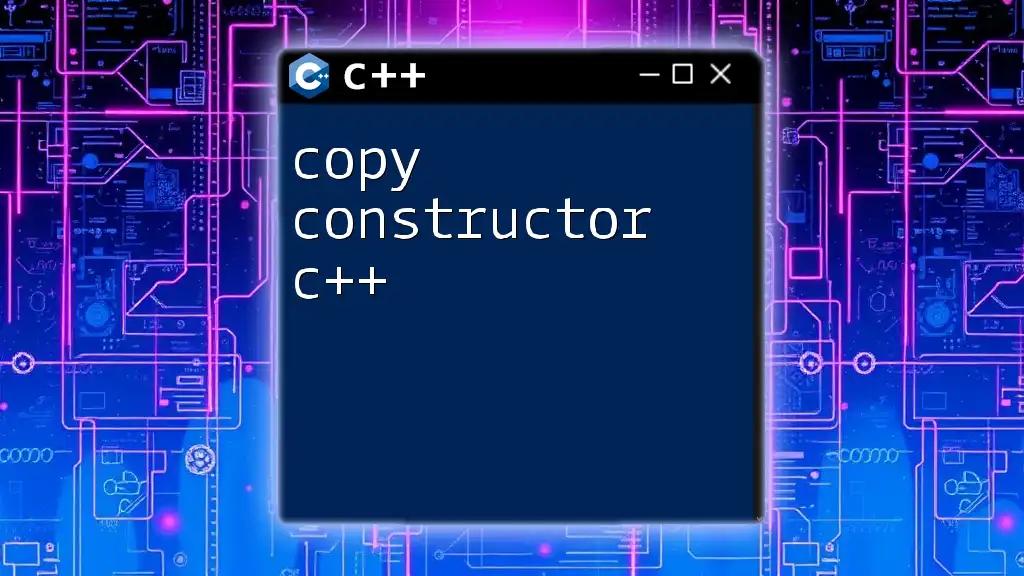
Conclusion
Recap of Key Takeaways
The importance of construction in C++ cannot be understated. Understanding the roles and types of constructors, as well as the proper use of destructors and best practices, lays a solid foundation for effective C++ programming. Employing initialization lists, the Rule of Three, and avoiding common pitfalls can significantly enhance your programming skill set.
Call to Action
Start applying what you’ve learned by practicing the creation of your own classes with various constructors. Experiment with overloaded constructors, initialization lists, and resource management to truly understand their impact on your C++ projects.
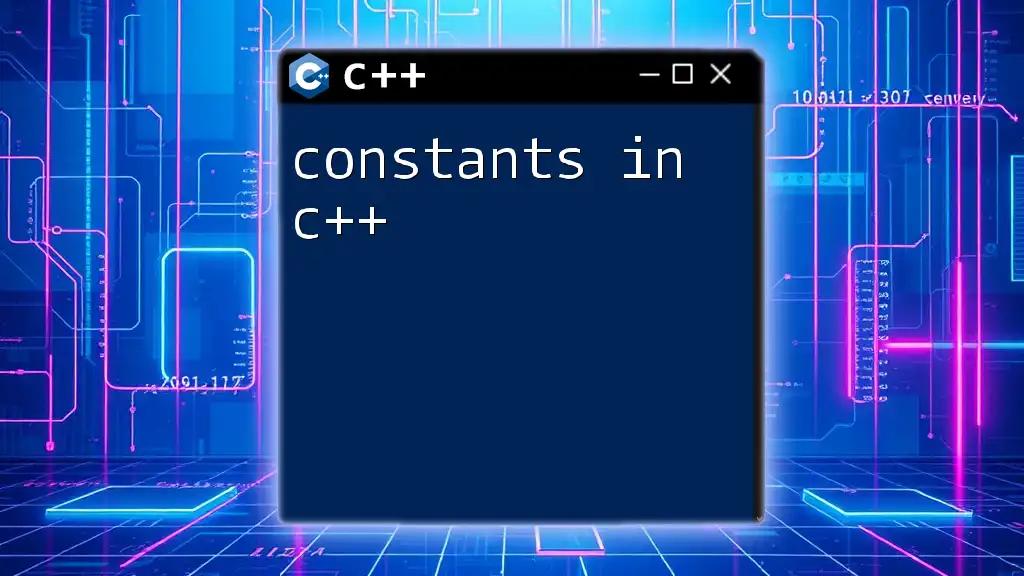
Additional Resources
Further Reading
To deepen your understanding, consider exploring books and articles dedicated to C++ programming, focusing specifically on object-oriented principles and resource management.
Community and Support
Participate in forums and online communities where you can ask questions, share experiences, and learn more about constructors and other C++ features.