The `sort` function in C++ is used to arrange the elements of a container in a specified order, typically in ascending order, using the standard library's algorithm facilities.
Here’s a simple code snippet demonstrating the use of the `sort` function with a vector:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 2, 9, 1, 5, 6};
std::sort(numbers.begin(), numbers.end());
for (int n : numbers) {
std::cout << n << " ";
}
return 0;
}
Understanding the Sort Function in C++
What is the Sort Function?
The sort function in C++ is a powerful utility provided by the Standard Library for organizing collections of data. It allows programmers to easily sort arrays and other containers in ascending or descending order. This function is extremely versatile and is a cornerstone for many algorithms that rely on ordered data.
Sorting is a foundational operation in computer science, essential for data analysis, searching algorithms, and improving program efficiency. Understanding how to utilize the sort function effectively will enable you to handle data more proficiently in your applications.
How Sorting Works
The sort function typically implements an efficient sorting algorithm, such as quicksort, heapsort, or introsort, which has an average time complexity of O(n log n). Here, n is the number of elements to be sorted. The specific algorithm can vary, but the goal remains consistent: to arrange data in a specific order, enhancing both accessibility and usability.
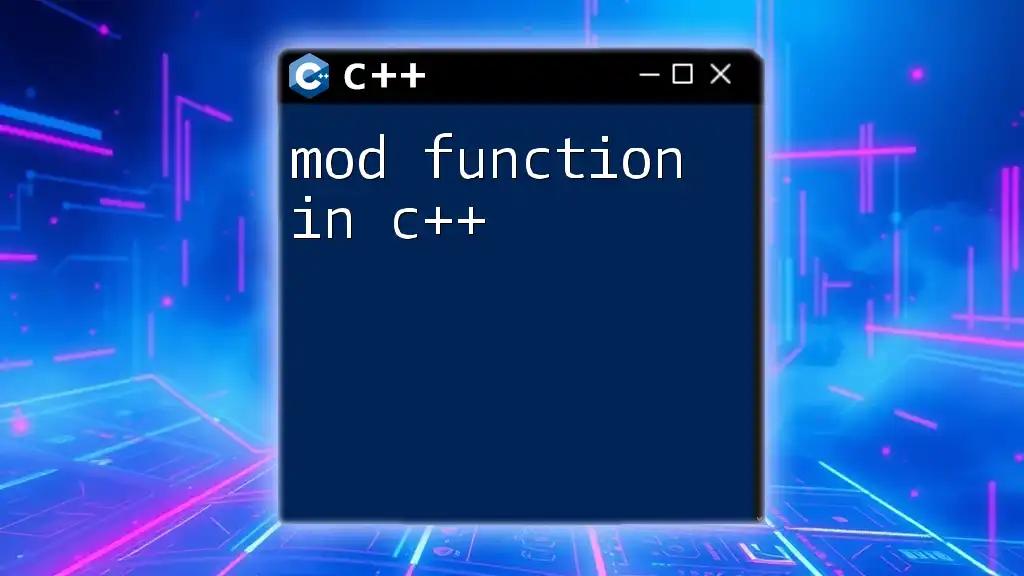
Using the Sort Function in C++
Syntax of the Sort Function
The syntax for using the sort function in C++ is straightforward:
std::sort(begin_iterator, end_iterator, comparison_function);
- begin_iterator: The starting point of the range to be sorted.
- end_iterator: The endpoint of the range to be sorted, not included in sorting.
- comparison_function: (optional) This is a custom function that defines how to compare the elements.
Including Necessary Libraries
To utilize the sort function, you must include the `<algorithm>` library at the beginning of your program:
#include <algorithm>
This library contains the declaration and definitions required for the sort function and other useful algorithms.
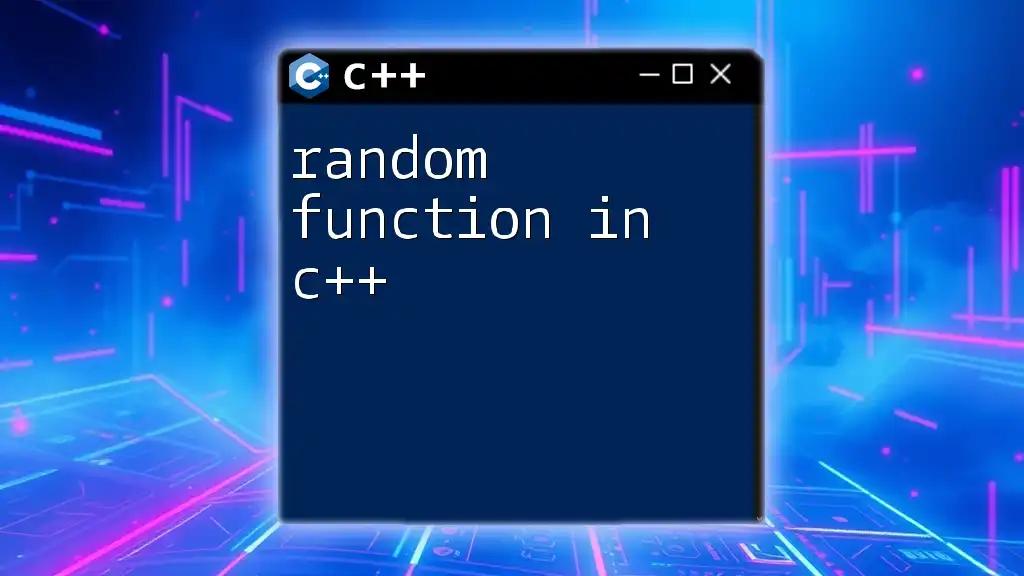
Example of the Sort Function
Sorting a Simple Array
A basic demonstration highlights sorting a standard array in ascending order. Consider the following example:
#include <iostream>
#include <algorithm>
int main() {
int arr[] = {5, 3, 4, 2, 1};
int n = sizeof(arr)/sizeof(arr[0]);
std::sort(arr, arr + n);
for(int i = 0; i < n; i++)
std::cout << arr[i] << " ";
return 0;
}
In this code:
- We define an array and calculate its size.
- The sort function is called with the array's begin and end pointers.
- Finally, we iterate through the sorted array to display the result.
Sorting Vectors
The sort function in C++ is equally effective when applied to vectors. The following example demonstrates this:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {5, 3, 4, 2, 1};
std::sort(vec.begin(), vec.end());
for(auto &v : vec)
std::cout << v << " ";
return 0;
}
Here, we utilize the `vector<int>` container. The sort function is applied directly to its begin and end iterators, making it a seamless process. The output will be a sorted vector displayed on the console.
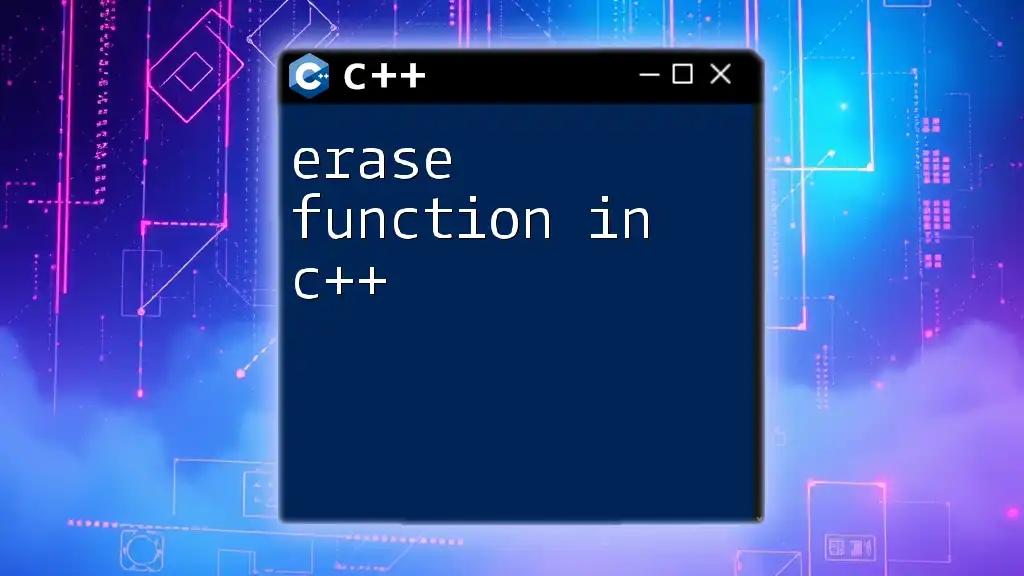
Custom Sorting Criteria
Using Comparison Functions
In some cases, you may wish to sort your data differently than the default ascending order. Here’s how to create a custom comparison function:
#include <iostream>
#include <vector>
#include <algorithm>
bool compare(int a, int b) {
return a > b; // For descending order
}
int main() {
std::vector<int> vec = {5, 3, 4, 2, 1};
std::sort(vec.begin(), vec.end(), compare);
for(auto &v : vec)
std::cout << v << " ";
return 0;
}
Lambda Expressions in Sorting
C++ allows for a more modern approach using lambda expressions. These can be particularly useful for defining inline comparison logic. Consider the following example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {5, 3, 4, 2, 1};
std::sort(vec.begin(), vec.end(), [](int a, int b) {
return a < b; // Ascending order
});
for(auto &v : vec)
std::cout << v << " ";
return 0;
}
In this case, the custom sorting logic is defined directly within the sort function call. This not only saves space but also enhances readability by keeping the logic close to where it is applied.
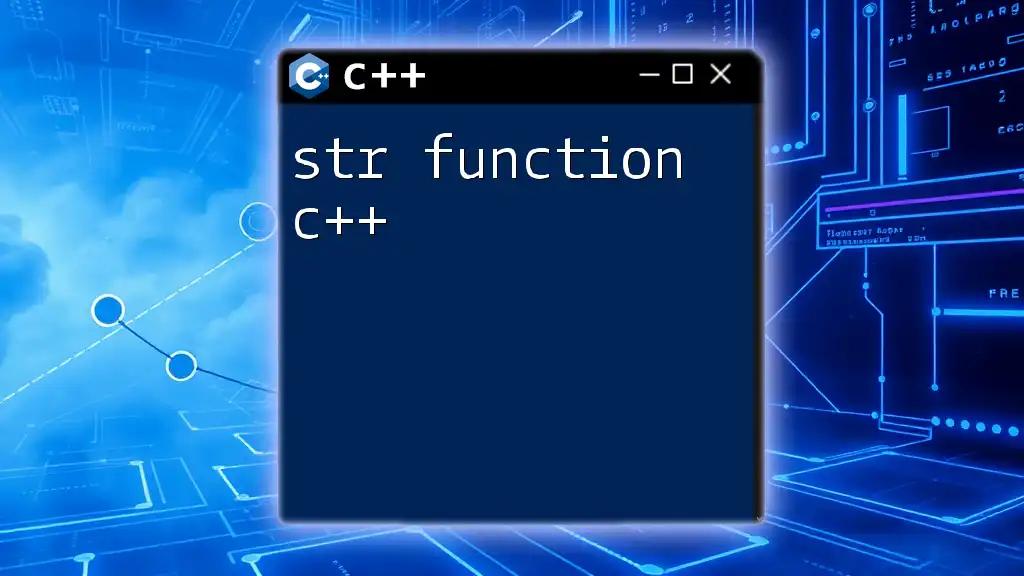
Edge Cases and Performance Considerations
Empty Arrays and Vectors
When dealing with the sort function in C++, you should be aware of how it behaves with empty arrays or vectors. Sorting an empty container does nothing, but it’s essential to ensure that your code can handle this scenario without assumptions.
Large Data Sets
When sorting large datasets, consider the implications on performance. The sort function is optimized for average cases, but for very large datasets, you might explore other sorting algorithms like merge sort, which can have better performance in specific conditions.
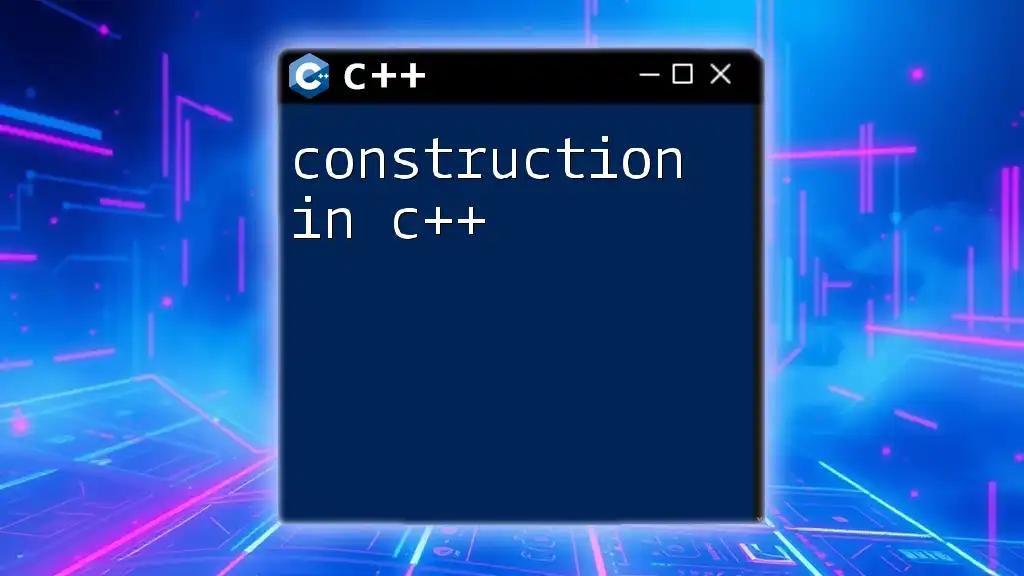
Conclusion
Recap of the Sort Function in C++
The sort function in C++ is an invaluable tool for organizing data quickly and effectively. It can handle arrays and vectors with ease, and supports customization through comparison functions and lambda expressions. Understanding this function will enhance your programming capabilities, allowing you to achieve more complex data handling processes with minimal effort.
Further Learning Resources
To dive deeper into sorting techniques, check the official C++ documentation or explore additional resources dedicated to algorithms. Engaging in hands-on practice with various sorting scenarios will solidify your understanding of this essential function.
Get Started with Sorting Now!
Begin incorporating the sort function in C++ into your projects and see how it can streamline your data manipulation processes. Happy coding!