Hash functions in C++ are algorithms that convert input data into a fixed-size string of characters, which is typically a sequence of numbers and letters, helping in data retrieval and ensuring data integrity. Here's a simple example using the Standard Template Library (STL) hash function:
#include <iostream>
#include <string>
#include <functional>
int main() {
std::string data = "Hello, C++!";
std::hash<std::string> hashFunction;
size_t hashValue = hashFunction(data);
std::cout << "Hash value of \"" << data << "\": " << hashValue << std::endl;
return 0;
}
What is a Hash Function?
A hash function is a specialized algorithm that transforms input data of arbitrary size into a fixed-size output, often referred to as a hash value or hash code. It is a fundamental concept in computer science and has various applications ranging from data integrity verification to efficient data retrieval mechanisms.
Importance of Hashing in Computer Science
Hash functions play a crucial role in many computer science fields. For instance, they are integral to data structure implementations such as hash tables, where they enable O(1) average time complexity for insertions, deletions, and look-ups. Additionally, hash functions are pivotal in cryptography, where they ensure data security and message integrity.
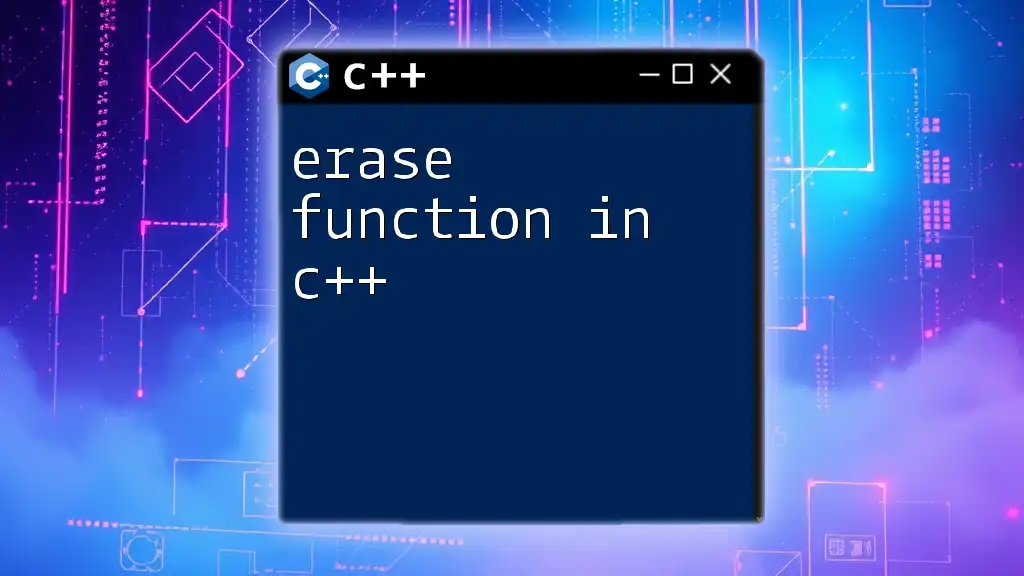
Overview of Hash Functions in C++
In C++, hash functions are used extensively in Standard Template Library (STL) containers, particularly `std::unordered_map` and `std::unordered_set`. By providing a hash function, you can efficiently store and retrieve data based on keys, reducing the complexity of these operations through effective indexing.
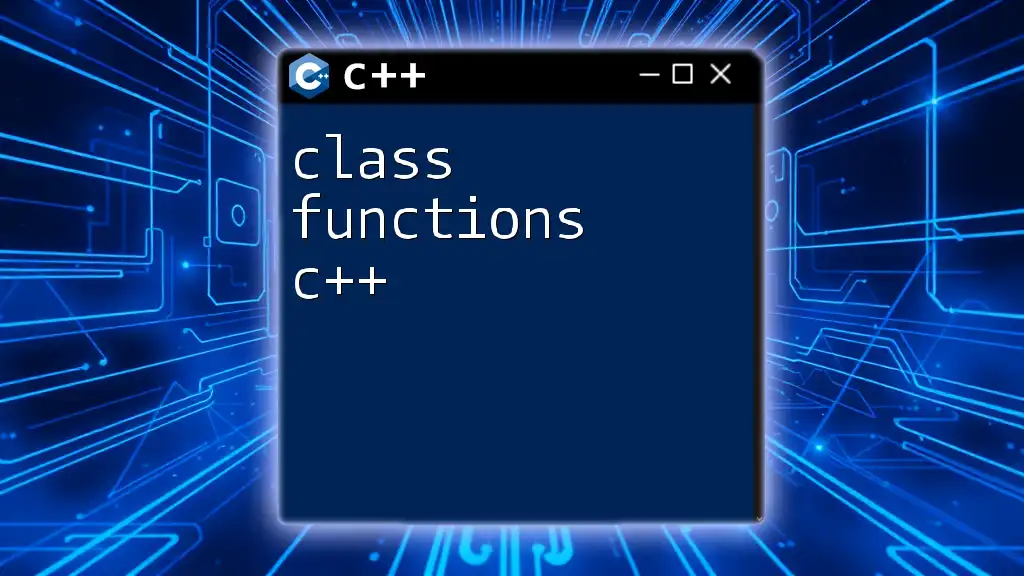
Understanding C++ Hash Function
Definition and Characteristics of a C++ Hash Function
A C++ hash function needs to satisfy several characteristics:
- Determinism: The same input must always produce the same hash value.
- Uniform Distribution: The hash values should be evenly distributed across the output space to minimize collisions.
- Efficiency: It should compute the hash in a short amount of time.
- Collision Resistance: It should minimize, but not entirely eliminate, the chances of different inputs producing the same hash value.
Common Uses of Hash Functions in C++
Hash functions find their utility in various scenarios:
- Data Retrieval in Containers: Using hash functions within data structures like hash tables allows faster access based on keys.
- Data Integrity Verification: Hashing techniques are employed for checksums to ensure data integrity during transmission.
- Cryptographic Applications: Hash functions are crucial for hashing passwords and other sensitive data, providing an additional layer of security.
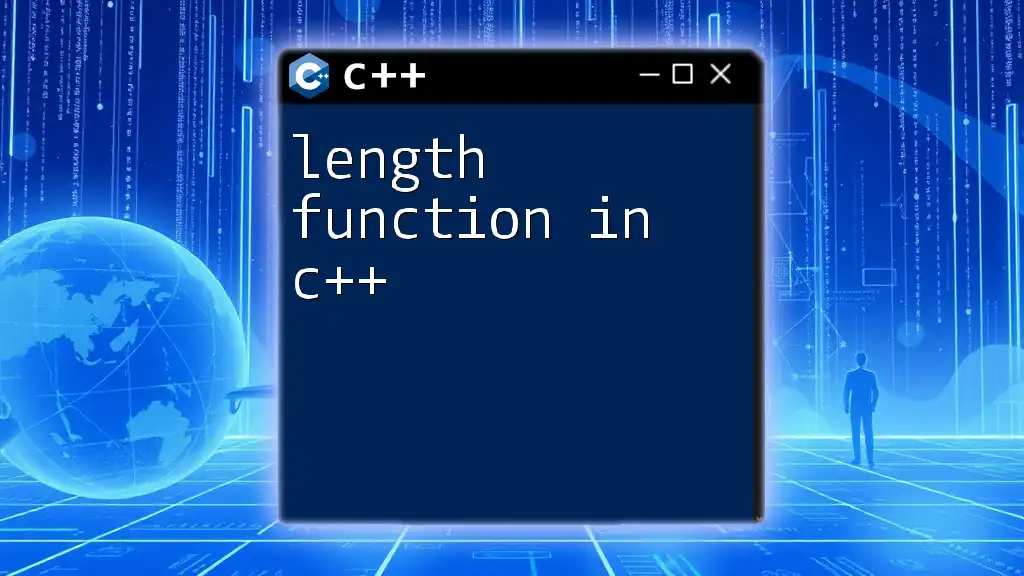
C++ Hash Algorithms
Common Hash Algorithms
Several hashing algorithms have been developed, which can provide varying levels of security and performance, notably:
- MD5: Once widely used, now considered insecure due to vulnerabilities.
- SHA-1: More secure than MD5 but still susceptible to certain attacks.
- SHA-256: A part of the SHA-2 family, it is currently one of the strongest hashing algorithms.
Implementing Hash Algorithms in C++
To utilize hash algorithms in C++, you can employ libraries such as OpenSSL.
Example: Using SHA-256 from OpenSSL
Here’s a practical implementation of SHA-256:
#include <openssl/sha.h>
#include <iostream>
#include <iomanip>
std::string sha256(const std::string str) {
unsigned char hash[SHA256_DIGEST_LENGTH];
SHA256_CTX sha256;
SHA256_Init(&sha256);
SHA256_Update(&sha256, str.c_str(), str.size());
SHA256_Final(hash, &sha256);
std::ostringstream oss;
for (const auto &byte : hash) {
oss << std::setw(2) << std::setfill('0') << std::hex << (int)byte;
}
return oss.str();
}
This code initializes the SHA-256 context, processes the input string, and outputs the hash in hexadecimal format.
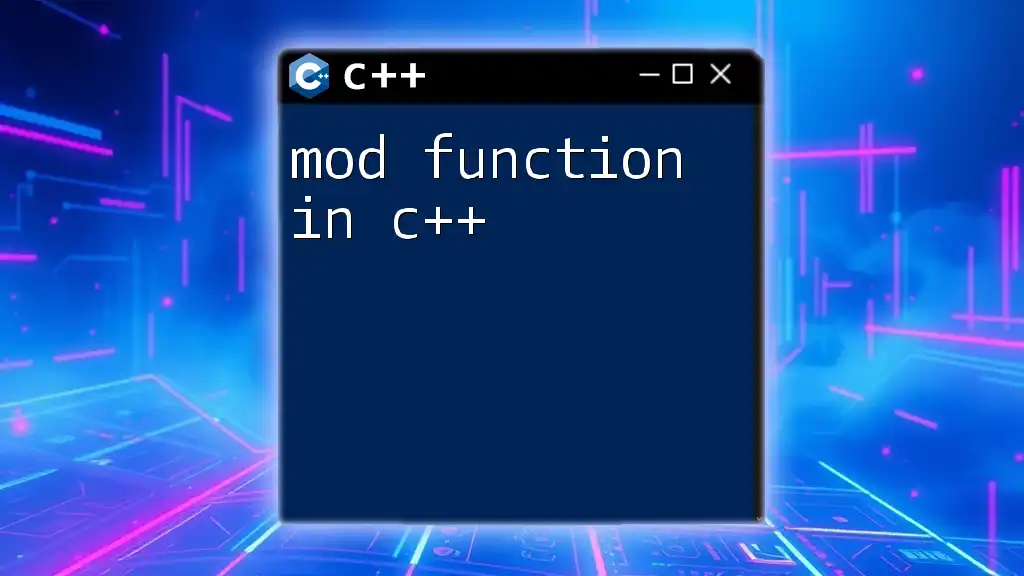
Creating a Custom Hash Function in C++
The Importance of Custom Hash Functions
While the standard hash functions are adequate for many applications, custom hash functions become necessary in specific contexts. Creating a tailored hashing function can enhance performance and improve the efficiency of data retrieval, particularly when dealing with custom data types.
Implementing a Custom Hash Function
Here’s a step-by-step guide to creating a simple custom hash function using a struct.
Example: Hash Function for Custom Struct
Suppose you have a data structure representing a point in a 2D space:
struct Point {
int x, y;
};
struct PointHash {
std::size_t operator()(const Point& p) const {
return std::hash<int>()(p.x) ^ std::hash<int>()(p.y);
}
};
In this code, the custom hash function combines the hash values of `x` and `y` coordinates to produce a unique hash for each `Point` instance. This allows you to use `Point` as keys in hash-based containers like `std::unordered_map`.
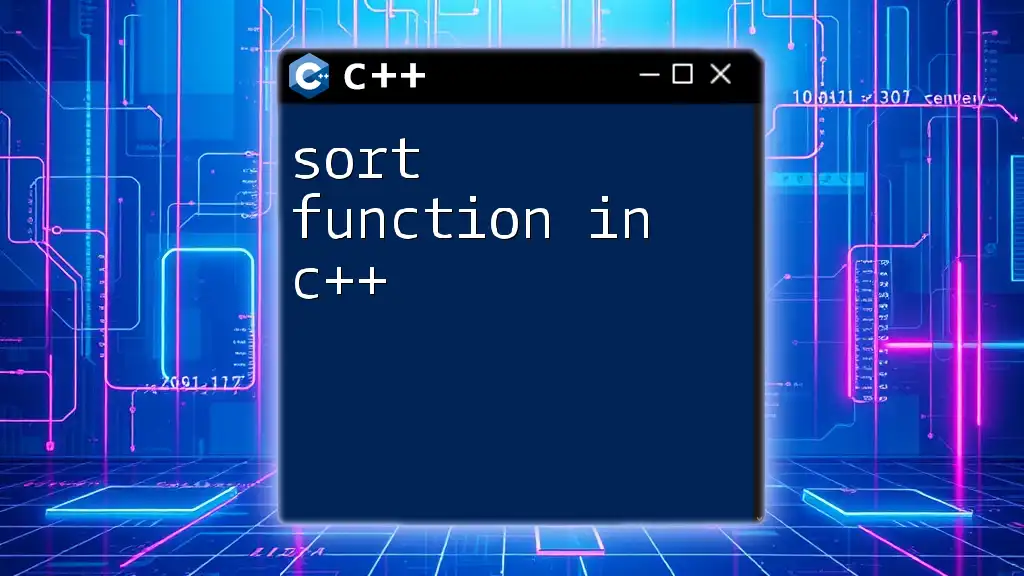
Collision Handling in Hash Functions
Understanding Hash Collisions
A hash collision occurs when two different inputs produce the same hash value. While collisions are virtually unavoidable due to the pigeonhole principle (more possible inputs than unique hash values), effective handling of collisions is essential for maintaining performance.
Collision Resolution Strategies
There are two primary strategies for managing hash collisions:
-
Separate Chaining: Each bucket in the hash table holds a list of entries. When a collision occurs, the new entry is simply added to the linked list for that bucket.
-
Open Addressing: Instead of using lists, this method finds the next available slot in the table upon collision.
Example: Simple Implementation of Open Addressing
Here’s a basic structure defining a hash table using open addressing:
const int TABLE_SIZE = 10;
struct HashTable {
std::vector<int> table[TABLE_SIZE];
void insert(int key);
// Additional methods for searching and deleting would go here
};
In this example, each index in the table represents a slot for storing the hashed values, with methods to handle insertions and lookups.
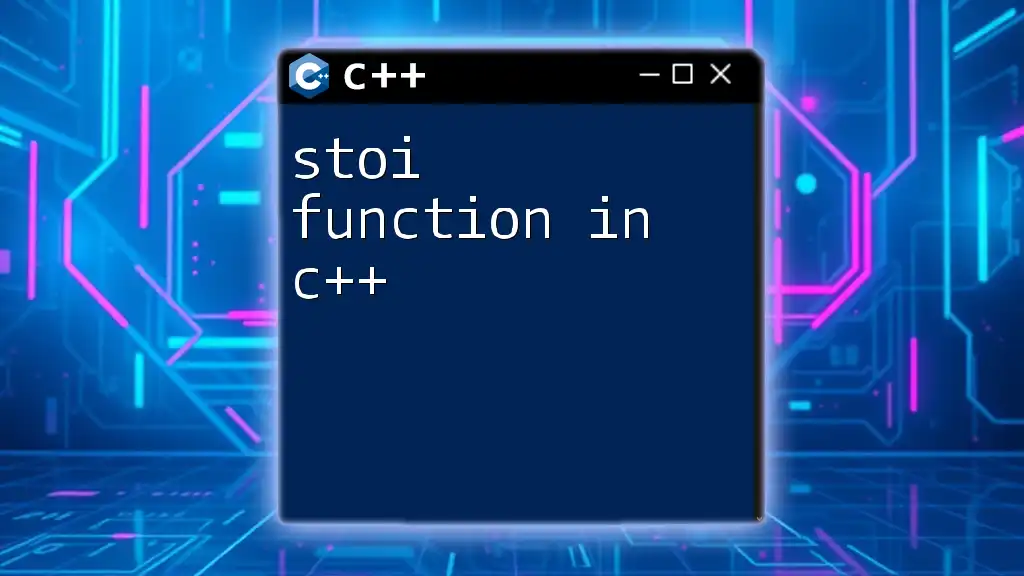
Best Practices for Using Hash Functions in C++
Choosing the Right Hash Function
When selecting a hash function, consider:
- Speed: Look for fast computation methods, especially for applications involving high volumes of data.
- Security: If your application involves sensitive data, opt for cryptographic hash functions to bolster security.
Testing for Hash Function Quality
It is important to assess the quality of your hash function using metrics like distribution, speed, and collision rate. Regular testing and analysis can help maintain optimal performance and adapt to new data patterns.
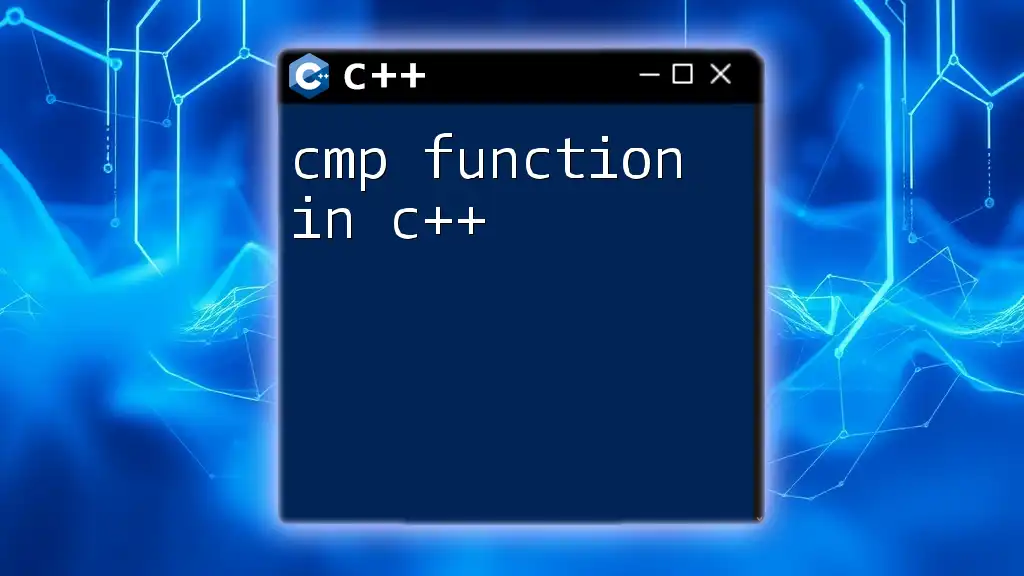
Conclusion
In this comprehensive guide, we explored the concept of hash functions in C++, delving into their definition, common uses, implementation of established algorithms, and creation of custom hash functions. While the handling of collisions and choosing the right hash strategy are crucial, understanding the foundational principles of hashing can enhance your development skills and improve application performance.
Stay curious and proactive in your learning journey! As you delve further into C++, employing effective hash functions will undoubtedly enhance your coding prowess and application efficiency.