The `rand()` function in C++ generates pseudo-random integers, and it can be used in conjunction with `srand()` to seed the random number generator for varied outputs each time the program runs.
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
srand(static_cast<unsigned int>(time(0))); // Seed the random number generator
int randomNum = rand(); // Generate a random number
std::cout << "Random number: " << randomNum << std::endl;
return 0;
}
Understanding `rand()` in C++
What is `rand()`?
The `rand()` function in C++ is a built-in function that generates pseudo-random integers. The randomness of these integers is generated using an algorithm that is initialized using a seed value. By default, `rand()` produces the same sequence of numbers each time the program runs unless you introduce some variation through seeding.
Syntax of `rand()`
The syntax of the `rand()` function is straightforward. It does not take any parameters and returns an `int` representing a pseudo-random number. Here's a simple invocation:
int randomValue = rand();
The default range of numbers returned by `rand()` is from 0 to `RAND_MAX`, which is a constant defined in `<cstdlib>`. Its value is typically at least 32767.

Random Number Generation in C++
How to Use `rand()` in C++
To make effective use of the `rand()` function, it’s crucial to understand how to call it properly. Here’s how you can implement the `rand()` function in a basic C++ program:
#include <iostream>
#include <cstdlib>
int main() {
std::cout << "Random Number: " << rand() << std::endl;
return 0;
}
In this example, the program outputs a random number every time it is run. However, without seeding, the sequence of random numbers will remain constant across runs, which limits the usefulness of randomization.
Seeding the Random Number Generator
To achieve unique sequences of random numbers, it's essential to seed the random number generator using the `srand()` function. The typical approach is to seed it with the current time, ensuring that the seed value changes with each execution.
#include <iostream>
#include <cstdlib>
#include <ctime> // For time()
int main() {
srand(static_cast<unsigned int>(time(0))); // Seed with current time
std::cout << "Random Number: " << rand() << std::endl;
return 0;
}
In this code, `srand()` is called once before invoking `rand()`, seeding the generator with the current time. This way, every program execution results in a different sequence of random numbers.
Generating Random Numbers within a Range
While `rand()` is useful, it can be more effective when you want random numbers within a specific range. To do this, you can use a simple formula to map the output of `rand()` to your desired range.
The formula to generate a random integer between `min` and `max` is as follows:
int randomInRange = min + (rand() % (max - min + 1));
Here’s an example generating a random number between 1 and 100:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
srand(static_cast<unsigned int>(time(0)));
int min = 1, max = 100;
int randomValue = min + (rand() % (max - min + 1));
std::cout << "Random Number between " << min << " and " << max << ": " << randomValue << std::endl;
return 0;
}
This code snippet demonstrates how you can control the range of your random numbers, making `rand()` much more versatile for various applications.
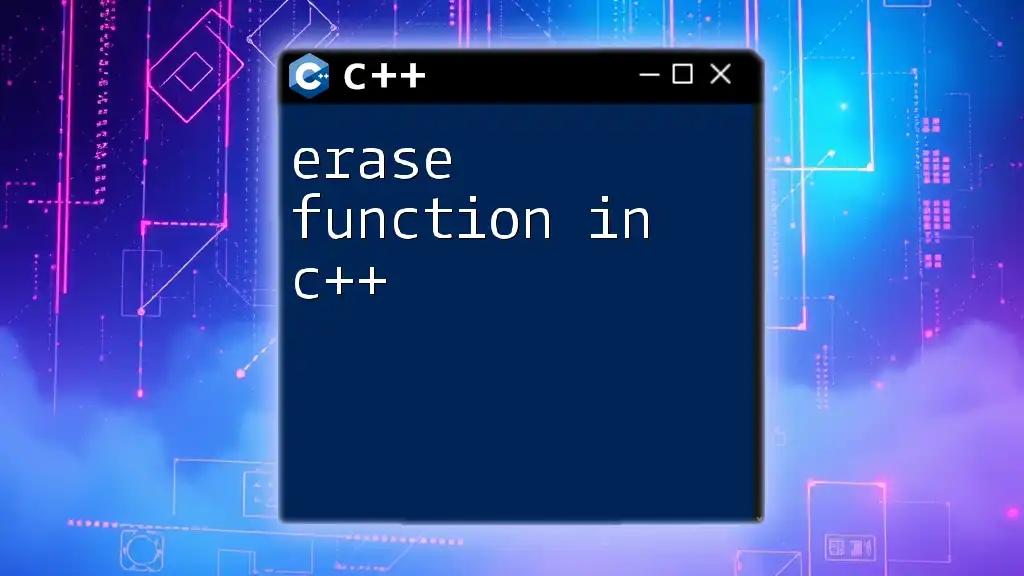
Alternatives to `rand()` in C++
Introduction to `<random>` Library
The `<random>` library, introduced in C++11, provides better and more flexible random number generation facilities than `rand()`. It allows finer control over the type of distribution and the quality of randomness. Unlike `rand()`, which uses a fixed algorithm and configuration, the `<random>` library supports various generators and distributions, offering greater robustness for applications requiring randomness.
Using `std::default_random_engine`
To use the `<random>` library, you can implement `std::default_random_engine`, which provides a random number generator that can be seeded for varying output.
Here’s an example:
#include <iostream>
#include <random>
int main() {
std::random_device rd; // Obtain a random number from hardware
std::default_random_engine eng(rd()); // Seed the generator
std::uniform_int_distribution<int> distr(1, 100); // Define the range
std::cout << "Random Number: " << distr(eng) << std::endl;
return 0;
}
In this example, a random device is used to obtain a seed from the hardware, and numbers are then drawn uniformly from the distribution defined between 1 and 100.
Exploring Different Distributions
The C++ `<random>` library offers several types of distributions allowing for various statistical models. A couple of notable examples include:
- Uniform Distribution: Generates real-valued numbers uniformly distributed in a specified range.
- Normal Distribution: Generates real numbers with a mean and standard deviation.
Here’s how you can implement a uniform real distribution:
std::uniform_real_distribution<float> distr(0.0f, 1.0f);
std::cout << "Random Float: " << distr(eng) << std::endl;
This provides a random float within the range of 0.0 to 1.0, showcasing the flexibility and accuracy provided by the `<random>` library compared to `rand()`.
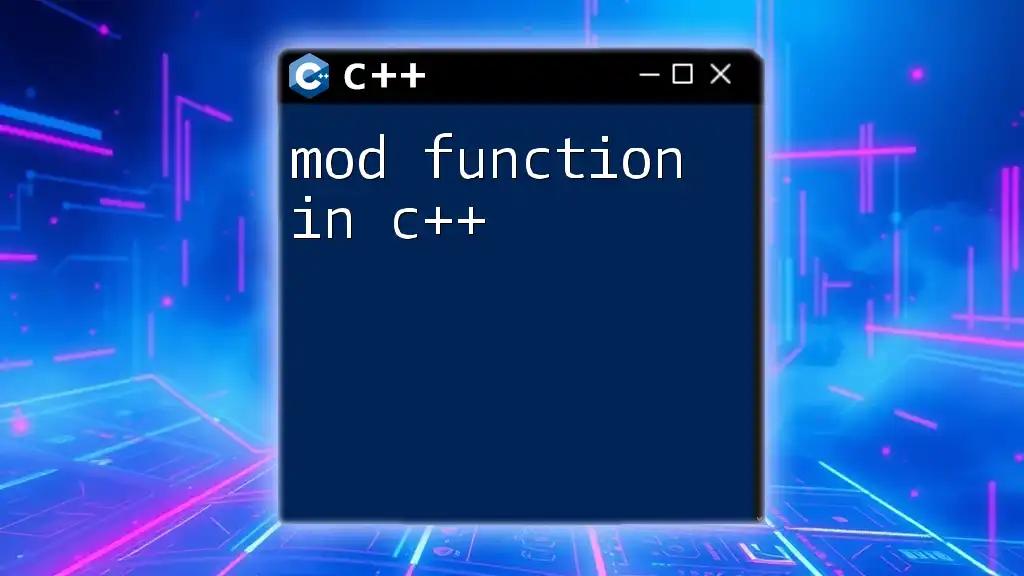
Common Use Cases of Random Functions in C++
Games and Simulations
Randomness plays a pivotal role in making games fun and unpredictable. For instance, when rolling a dice in a game, you'd want the outcome to be random. Here’s a simple dice roll simulation using `rand()`:
int rollDice() {
return 1 + (rand() % 6);
}
Whenever `rollDice()` is called, it will return a random number between 1 and 6, simulating a dice roll effectively.
Data Sampling and Tests
Random functions are also essential in statistical sampling methods where datasets need to be explored or validated. For example, you might need to pick random samples from large datasets for quality control tests or experimental simulations, employing random functions to ensure fairness and accuracy.
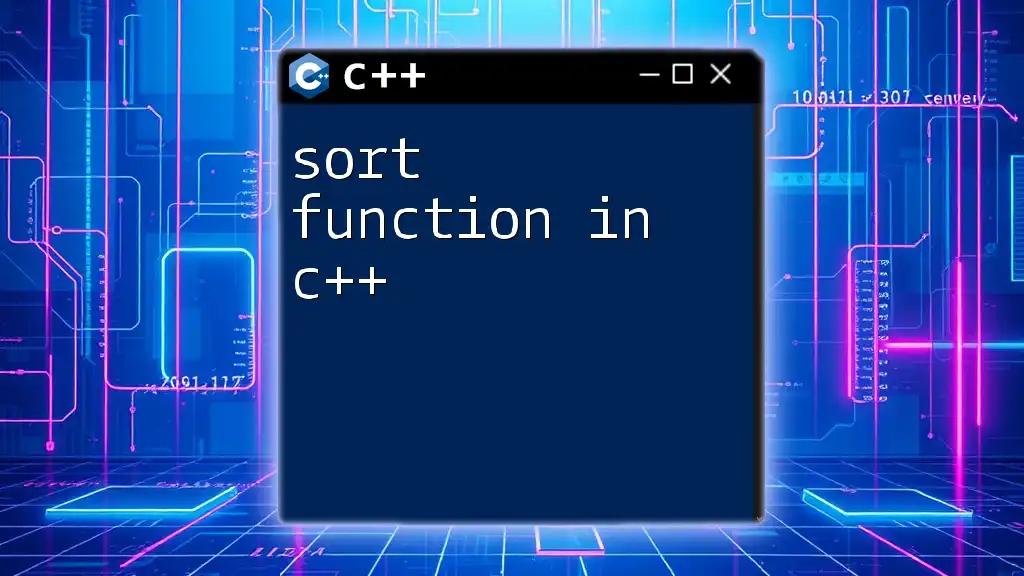
Tips for Effective Use of Random Functions in C++
Avoiding Common Pitfalls
When using the random function in C++, there are common pitfalls to avoid:
- Not Seeding: Failing to seed the generator leads to the same sequence of numbers upon each execution, which can render the randomness ineffective.
- Over-Reliance on `rand()`: Using `rand()` might suffice for simple applications, but for contexts requiring high-quality randomness (like cryptography), one should avoid it.
Best Practices for Randomization
To ensure effective usage of random functions in C++, consider the following best practices:
- Always seed the random number generator before first use.
- Prefer the `<random>` library for complex randomization needs.
- Understand the different distributions and choose appropriately based on your application needs.

Conclusion
The random function in C++ is a powerful tool that can add a layer of complexity and realism to your applications, whether in games, simulations, or data analysis. By understanding how to utilize `rand()` and the `<random>` library, you can create effective, random-based solutions tailored to your requirements. Experiment with the examples provided to deepen your understanding and mastery of random number generation in C++.