The `length()` function in C++ is used to return the number of characters in a string object, providing an efficient way to determine its size.
Here's an example of how to use the `length()` function in C++:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, World!";
std::cout << "Length of the string: " << myString.length() << std::endl;
return 0;
}
Understanding the Concept of Length in C++
In programming, length refers to the number of elements in a collection or string. Understanding how to calculate and manipulate lengths is fundamental in C++. This concept spans various data types, including arrays, strings, and vectors, each having unique methods for measuring length.
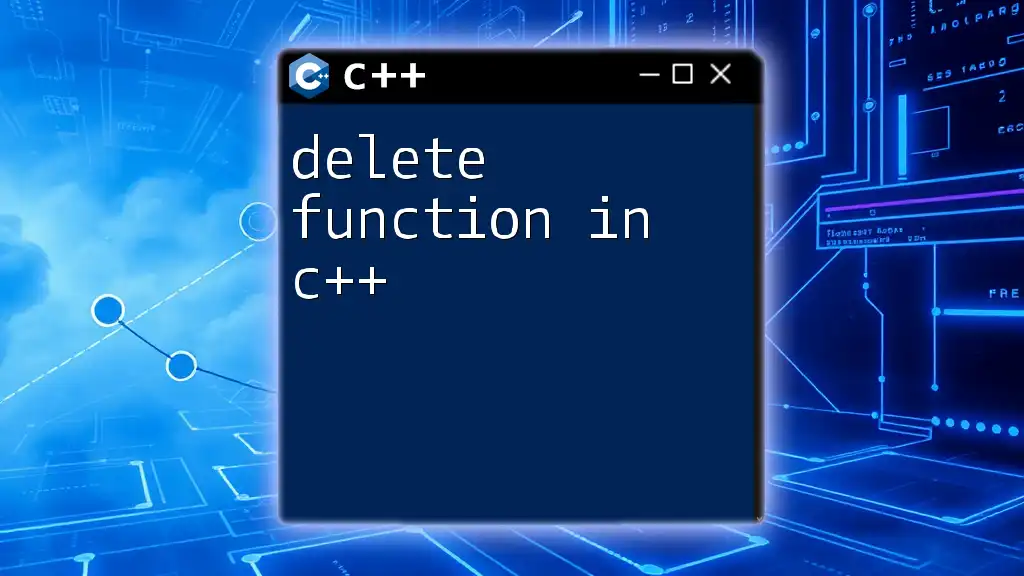
The Length Function for Strings
The std::string::length() Method
In C++, the `std::string` class provides a straightforward way to handle strings. One of its key methods is `length()`, which returns the number of characters in the string, excluding the null terminator.
Syntax:
size_t length() const noexcept;
Here’s an example to illustrate its usage:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, world!";
std::cout << "Length of the string: " << myString.length() << std::endl;
return 0;
}
In this example, the output would be:
Length of the string: 13
This example shows how `length()` calculates the number of characters in `myString`, effectively giving us the string's total count.
The std::string::size() Method
Interestingly, `std::string` also provides a `size()` method, which is synonymous with `length()`. Both methods achieve identical results, offering developers flexibility in choice.
Consider this snippet:
std::cout << "Size of the string: " << myString.size() << std::endl;
Although there's no significant difference, some developers prefer `size()`, as it resonates more naturally with other container classes in C++.
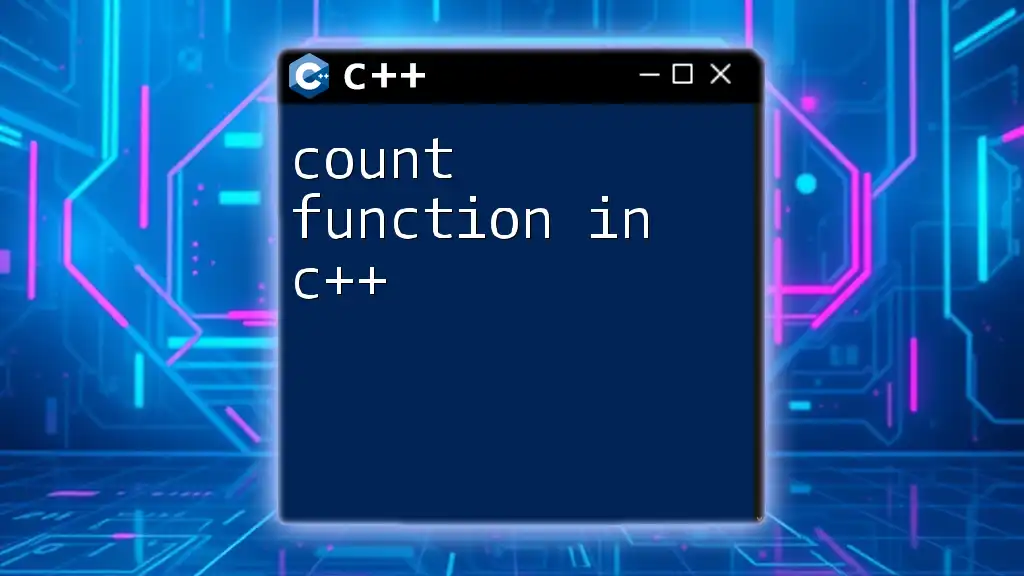
The Length Function for C-Style Strings
Understanding C-Style Strings
C-Style strings are char arrays terminated by a null character. Unlike `std::string`, they do not inherently know their length, requiring functions like `strlen()` to determine it.
Importantly, using C-Style strings can lead to risks, including buffer overflows and dangling pointers. Here’s how you measure length using `strlen()`:
#include <iostream>
#include <cstring>
int main() {
const char* cString = "Hello, C!";
std::cout << "Length of the C string: " << strlen(cString) << std::endl;
return 0;
}
The output:
Length of the C string: 10
This highlights that `strlen()` counts characters up to, but not including, the null terminator. Always remember to use this function cautiously, as lack of proper null termination can lead to undefined behavior.
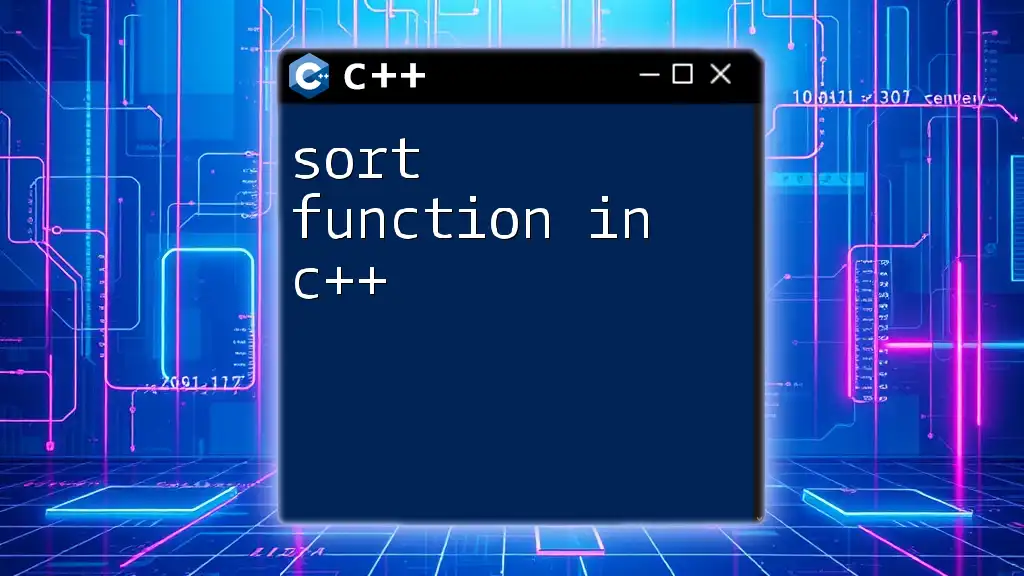
The Length Function for Other Data Structures
The length() Function in Vectors
Another vital area is the use of the length function with vectors. Vectors manage dynamic arrays and come with their own set of utility functions, most notably `size()`, which calculates the number of elements:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
std::cout << "Length of the vector: " << myVector.size() << std::endl;
return 0;
}
Here, the output would be:
Length of the vector: 5
In this context, `size()` serves as the length function for the vector, providing a simple way to determine how many elements are held.
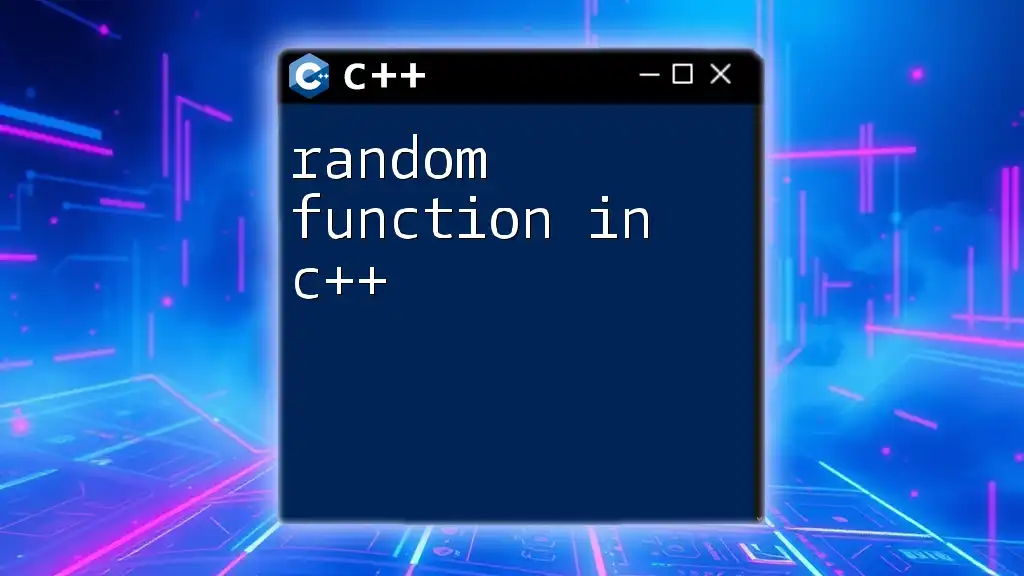
Performance Considerations
When working with the length function in C++, understanding performance is crucial. The complexity of length calculations varies by data type:
- For std::string: Both `length()` and `size()` operate in O(1) time, as they directly access a stored count of characters.
- For C-Style strings: Using `strlen()` operates in O(n) time, as it requires iterating through the string until it finds the null terminator.
- For vectors: The `size()` function also runs in O(1) time, leveraging already stored size information.
Knowing these complexities helps developers choose the appropriate functions based on their performance needs.
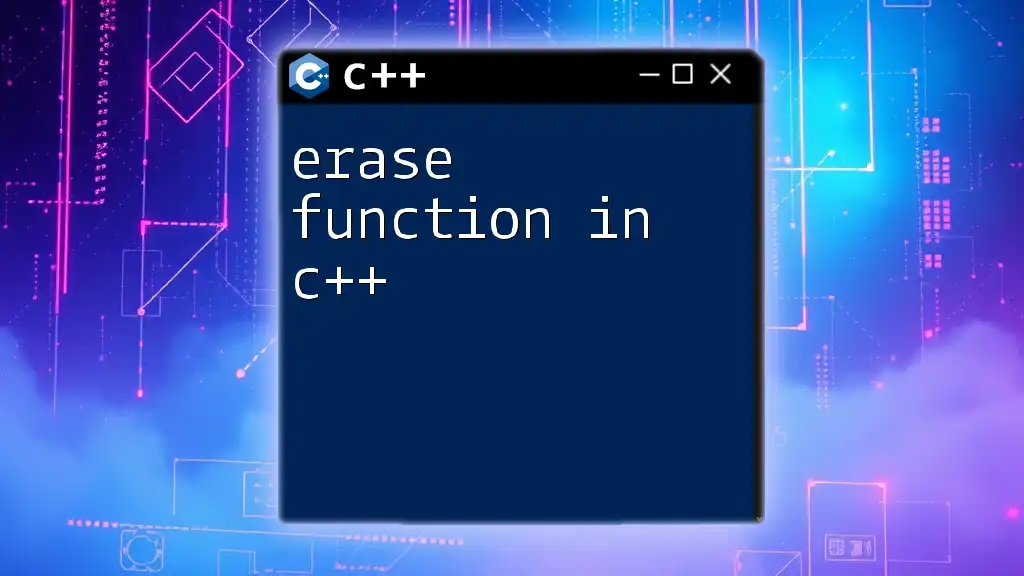
Common Mistakes and How to Avoid Them
- Using strlen() on uninitialized C-Style strings: Always ensure your strings are properly null-terminated.
- Confusing size() and length() in std::string: Remember that they are interchangeable, but consistency in usage is key for readability.
- Misestimating vector size: Remember that vectors automatically manage their size, so you often don't need to worry about length unless working with raw arrays.
To avoid these missteps, continual practice and consulting documentation is advised when working with the length function in C++.
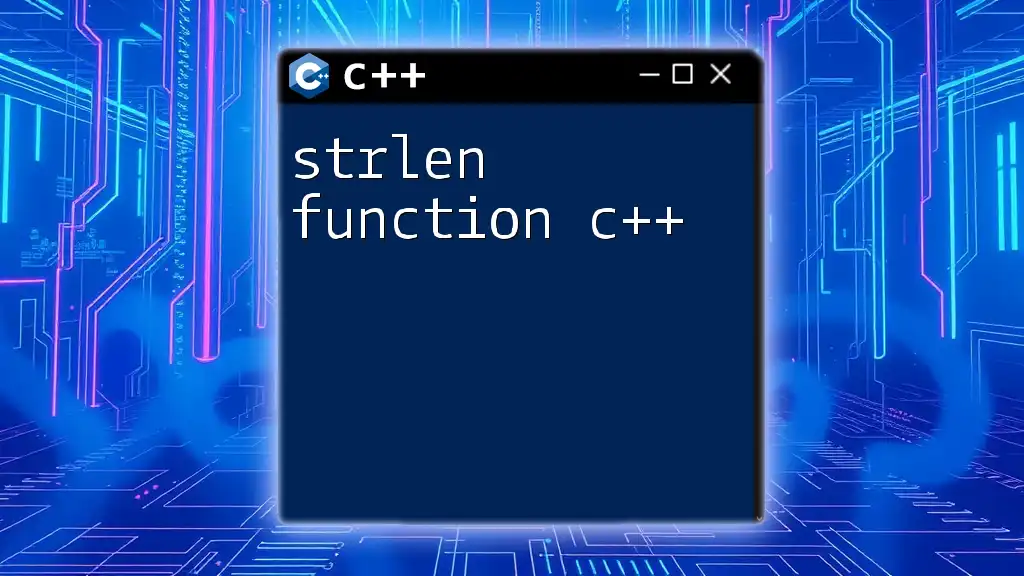
Conclusion
Understanding the length function in C++ is fundamental for effective programming. From manipulating strings to handling vectors, mastering these concepts empowers you to write more efficient and effective C++ code. With practice and the right resources, you can confidently navigate length calculations and their implications in various programming scenarios.