The `get` function in C++ typically refers to a method for retrieving input or accessing data, and is often used to read characters or strings from input streams.
Here's a simple example using `std::getline` to get a line of input from the user:
#include <iostream>
#include <string>
int main() {
std::string userInput;
std::cout << "Enter a line of text: ";
std::getline(std::cin, userInput); // Using getline to get a line of input
std::cout << "You entered: " << userInput << std::endl;
return 0;
}
Understanding the `get()` Function
What is the `get()` Function?
The `get` function in C++ is primarily used for input, allowing you to read characters from an input stream. This function is part of the standard input/output stream library and is an essential tool when it comes to handling character input with precision. Unlike other input functions that can skip whitespace, `get()` reads the input character by character, making it particularly useful for situations where precise control over the input is needed.
Syntax of the `get()` Function
The syntax of the `get()` function can vary depending on what you are trying to read. Here are the basic forms:
std::istream& get(char& ch);
std::istream& get(char* s, std::streamsize n);
std::istream& get(std::istream& is);
- `get(char& ch)`: Reads a single character from the stream and stores it in the variable `ch`.
- `get(char* s, std::streamsize n)`: Reads characters into a character array `s`, stopping after `n` characters or when a newline is encountered.
- `get(std::istream& is)`: Reads characters from the provided input stream.
Understanding the different signatures of the `get()` function allows you to select the version that best fits your input needs.
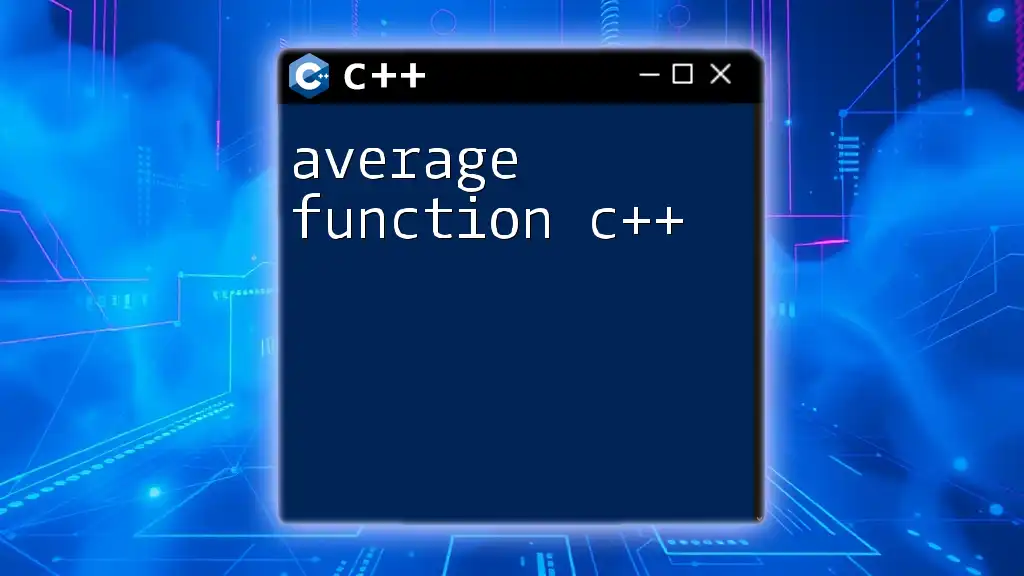
How to Use the `get()` Function
Reading a Single Character
You can use `get()` to retrieve a single character from the standard input. This simple operation is useful in various applications, such as validating user input.
Example Code Snippet
#include <iostream>
int main() {
char ch;
std::cout << "Enter a character: ";
std::cin.get(ch);
std::cout << "You entered: " << ch << std::endl;
return 0;
}
In this snippet, the program prompts the user to enter a character. The `get()` function captures the input character (including any whitespace) and then displays it back to the user. This is especially useful when you want to differentiate between space characters and other valid inputs.
Reading a String (Character Array)
If you want to read a string (or a sequence of characters) into a character array, `get()` comes in handy as well.
Example Code Snippet
#include <iostream>
int main() {
char str[100];
std::cout << "Enter a string: ";
std::cin.get(str, 100);
std::cout << "You entered: " << str << std::endl;
return 0;
}
Here, the program will read a string of characters up to a maximum of 99 characters, stopping if a newline character is encountered. The importance of this function is in its ability to manage input safely—ensuring that a buffer overflow doesn't occur by setting a limit.
Dealing with Newline Characters
One common situation programmers face is managing unwanted newline characters that `cin` might leave unread in the buffer.
Example Code Snippet
#include <iostream>
int main() {
char str[100];
std::cout << "Enter a string (includes newline): ";
std::cin.get(str, 100);
std::cin.ignore(); // Ignore the newline character left in the buffer
std::cout << "Wow, you entered: " << str << std::endl;
return 0;
}
In this example, after using `get()` to read the string, we call `ignore()` to clean up the newline that may be left in the input buffer. This is especially important if you are planning to read additional inputs using different functions that require a clean buffer.
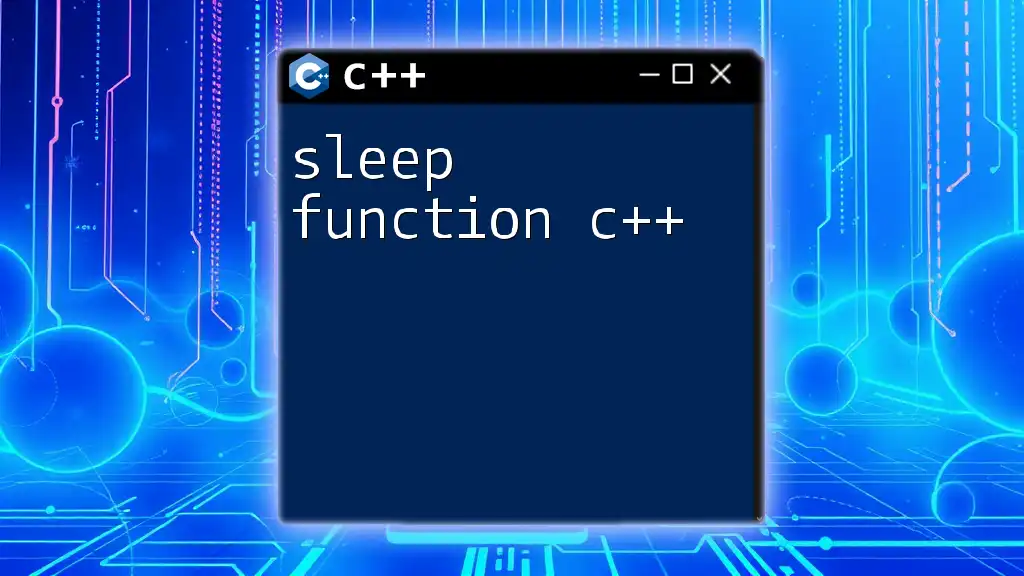
Advanced Usage of the `get()` Function
Reading Multiple Characters
You can use `get()` to read a string of multiple characters efficiently. By leveraging this capability, you can read input with more freedom and control.
Example Code Snippet
#include <iostream>
int main() {
char buffer[100];
std::cout << "Enter a few characters: ";
std::cin.get(buffer, 100);
std::cout << "You entered: " << buffer << std::endl;
return 0;
}
In this example, the program allows the user to enter a line of text. If you input more than 99 characters, the excess characters are ignored (up to the limit defined), which can help in managing user expectations by preventing overflow while keeping feedback immediate.
Using String Streams with `get()`
Another advanced scenario involves using `get()` with string streams, providing a bridge between file input and memory.
Example Code Snippet
#include <iostream>
#include <sstream>
int main() {
std::stringstream ss("This is a test");
char ch;
while (ss.get(ch)) {
std::cout << ch << " ";
}
return 0;
}
In this snippet, `get()` is being used with a `stringstream`. This allows you to read from a string as if it were an input stream, giving you greater flexibility in how data is processed within your programs. It can be particularly useful for parsing text or transforming input data.
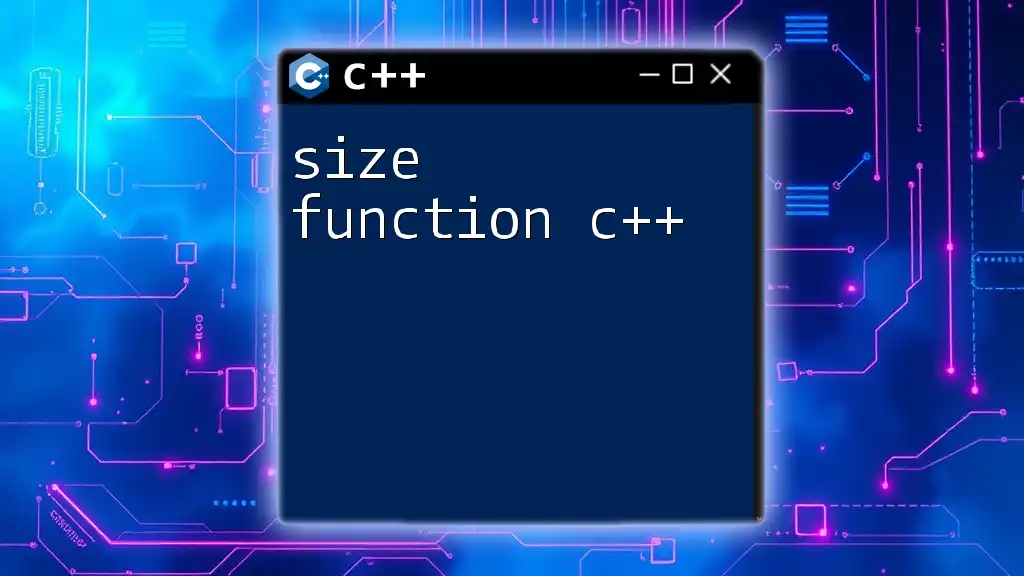
Common Errors and Troubleshooting
Common Issues When Using `get()`
While using the `get()` function, some common pitfalls may arise. For instance:
- Not accounting for buffer size: If you do not specify the buffer size correctly, you might face buffer overflow issues.
- Ignoring newlines: Failing to clear the input buffer after reading can lead to unexpected behaviors in subsequent input queries.
Debugging Suggestions
If `get()` isn’t working as expected:
- Check if you have set the correct buffer size.
- Use `cin.ignore()` to eliminate any leftover newlines before further input instructions.
- Ensure that you’re understanding the difference between reading into a character array versus a single character.
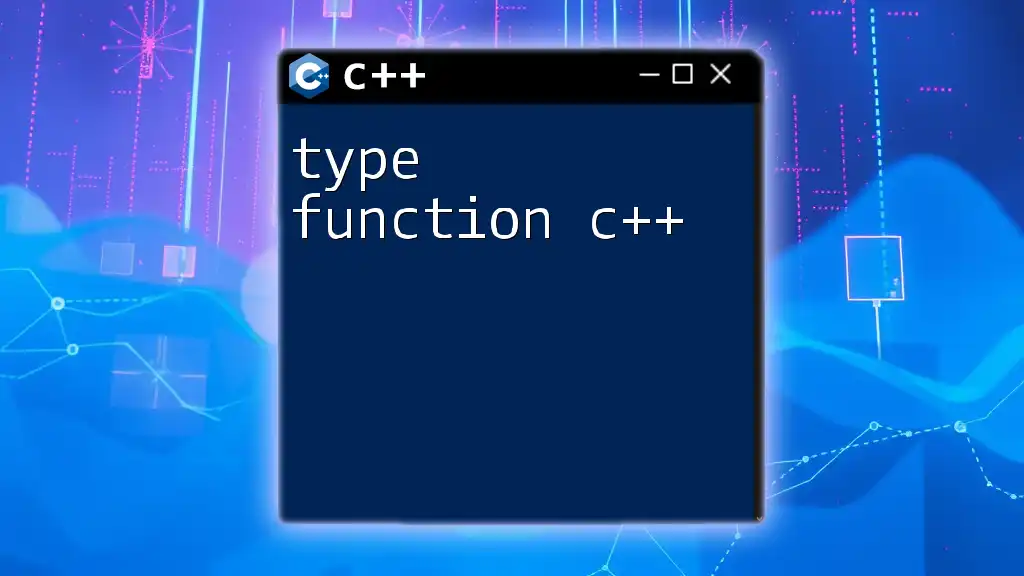
Conclusion
Understanding the `get function c++" is essential for anyone looking to handle input accurately in their C++ applications. By grasping how to read characters or strings, manage buffer sizes, and utilize stream-based techniques, you can enhance your control over user input dramatically. As you continue to explore C++, practicing these functionalities can help solidify your skills for real-world programming challenges.
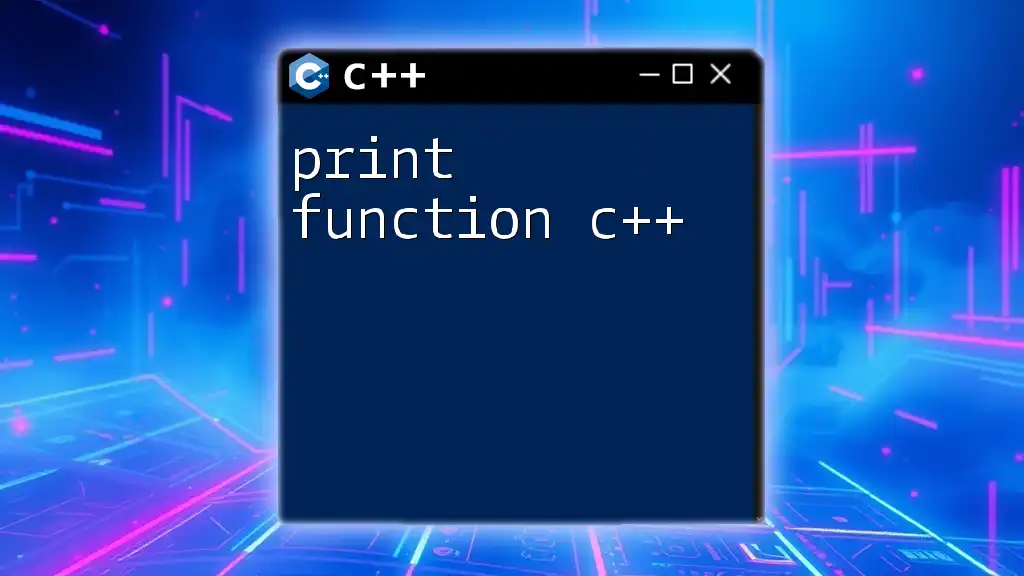
Additional Resources
To deepen your understanding of the `get()` function and its applications in C++, consider exploring additional readings, such as programming books, online tutorials, and structured courses that detail the rich capabilities of stream handling in C++.