The `strlen` function in C++ is used to determine the length of a string (excluding the null terminator), and it is part of the C Standard Library.
Here’s a simple code snippet demonstrating its usage:
#include <iostream>
#include <cstring>
int main() {
const char* str = "Hello, World!";
std::cout << "Length of the string: " << strlen(str) << std::endl;
return 0;
}
What is `strlen`?
The `strlen` function is a standard library function in C++ used to determine the length of a C-style string (an array of characters terminated by a null character). Understanding the `strlen` function is vital for string manipulation as it helps in tasks such as memory allocation and processing character arrays.
Brief Overview of C++ Strings
C++ provides two primary ways of handling strings: C-style strings and C++ strings (std::string).
- C-style strings are arrays of characters that end with a null character (`'\0'`). They are simple and form the foundation for many string operations.
- C++ strings (std::string) are more versatile, allowing dynamic sizing and providing numerous methods for string manipulation without needing to worry about null termination.
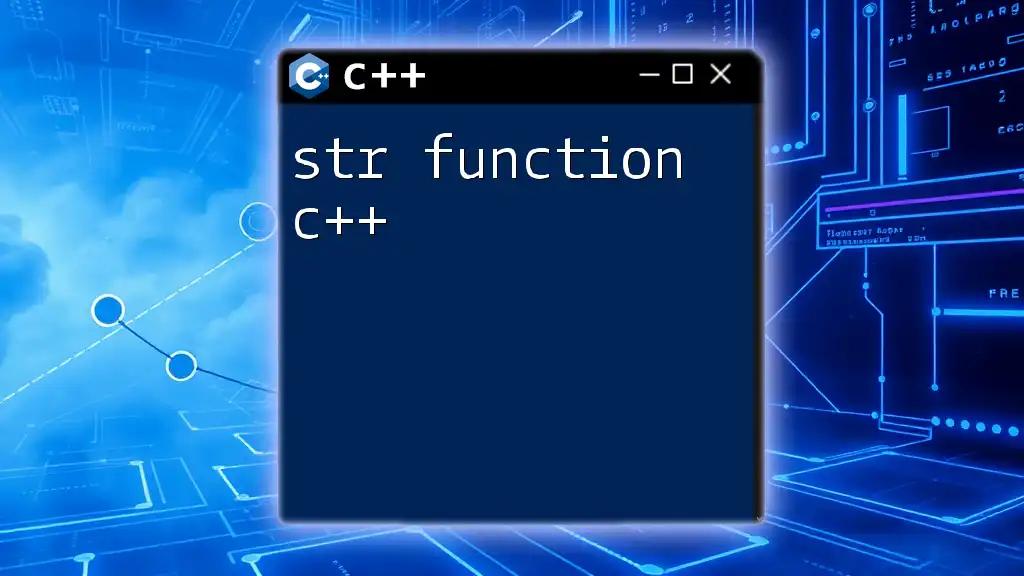
How to Use `strlen` in C++
Basic Syntax of `strlen`
The syntax of the `strlen` function is as follows:
size_t strlen(const char* str);
- Parameters: The function takes a single parameter, a pointer to a null-terminated character string.
- Return Value: It returns the length of the string, excluding the null terminator.
Including Necessary Libraries
To use the `strlen` function, you must include the `<cstring>` header in your program. Here's an example of the necessary headers to include:
#include <iostream>
#include <cstring>

Examples of Using `strlen` in C++
Basic Example of Using `strlen`
Here’s a straightforward example to demonstrate the use of `strlen`:
const char* myString = "Hello, World!";
size_t length = strlen(myString);
std::cout << "Length of myString: " << length << std::endl;
In this code, `myString` holds the value "Hello, World!" and `strlen` counts the number of characters, resulting in an output of 13.
Using `strlen` with Empty Strings
An empty string is a common edge case. Let’s look at how `strlen` handles it:
const char* emptyString = "";
std::cout << "Length of emptyString: " << strlen(emptyString) << std::endl; // Output: 0
In this instance, since there are no characters in `emptyString`, the output will be 0. This serves as a reminder to consider how edge cases can impact your string operations.
Using `strlen` with Special Characters
The `strlen` function counts all characters, including special ones. For example:
const char* specialChars = "Hello, World!\n";
std::cout << "Length of specialChars: " << strlen(specialChars) << std::endl;
The output will include all characters, showing the total count as 14. This demonstrates how `strlen` treats spaces, punctuation, and newline characters just like any other character.
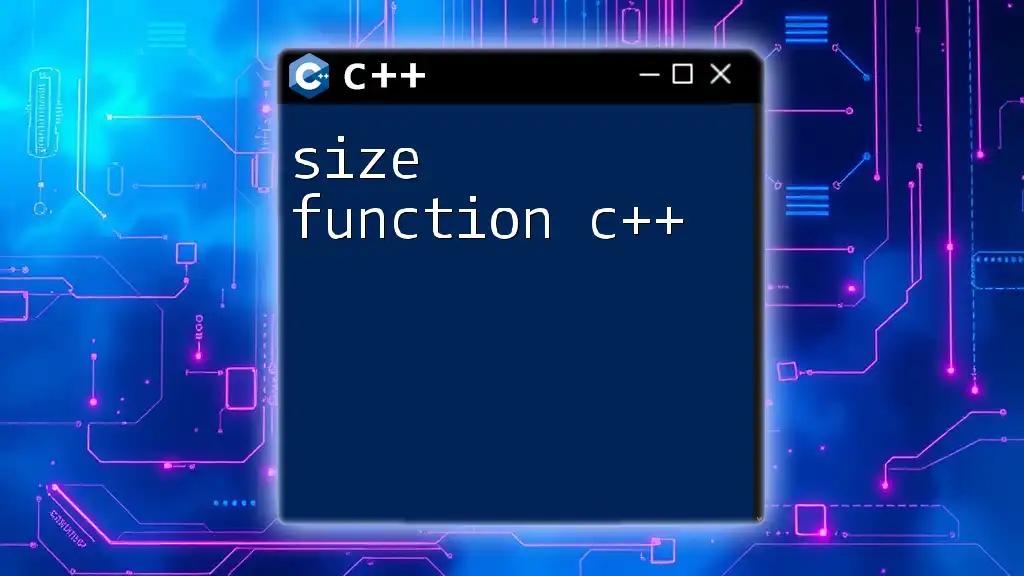
Performance Considerations
Time Complexity of `strlen`
The `strlen` function iterates through the string until it reaches the null terminator. This results in a time complexity of O(n), where n is the length of the string. Thus, for very long strings, it can impact performance in applications that frequently calculate string lengths.
Common Mistakes and Errors
Developers often encounter common pitfalls when using `strlen`.
- Not null-terminating strings: Failing to properly terminate a string can lead to undefined behavior since `strlen` relies on finding a null character.
- Passing non-C-style strings: Passing `std::string` directly to `strlen` will not work as expected. Here’s an example that illustrates this mistake:
std::string cppString = "Hello";
// Incorrect usage of strlen
size_t length = strlen(cppString.c_str()); // Correct approach to convert to C-style
The `c_str()` method is necessary to convert the `std::string` to a C-style string, which is compatible with `strlen`.

Alternatives to `strlen` in C++
Using `std::string::length()` Method
In C++, it's often more efficient to use the `length()` method from the `std::string` class instead of `strlen`, especially when dealing with C++ strings.
Here’s a comparison between `strlen` and `std::string::length()`:
std::string cppString = "Hello, World!";
std::cout << "Length of cppString: " << cppString.length() << std::endl;
This will yield the same length as `strlen`, but without the hassle of handling null-termination explicitly.
When to Use `strlen` vs `std::string::length()`
Using `strlen` is appropriate when working with legacy code or in scenarios where performance is critical with C-style strings. However, for most C++ applications, leveraging `std::string` and its `length()` method is recommended, as it enhances safety and reduces errors related to memory management.

Conclusion
In summary, the `strlen function in C++ is an essential tool for measuring the length of C-style strings. It plays a crucial role in string manipulation, yet comes with its own set of considerations regarding performance and safety. Whether you choose to use `strlen` or the `std::string::length()` method, understanding these functions will empower you to effectively handle string-related tasks in your C++ programming endeavors. Embrace the journey of mastering string functions and elevate your C++ skills!