The sum function in C++ takes two integers as arguments and returns their sum, allowing for quick arithmetic operations.
Here’s a simple code snippet demonstrating a sum function:
#include <iostream>
using namespace std;
int sum(int a, int b) {
return a + b;
}
int main() {
cout << "The sum is: " << sum(5, 7) << endl; // Outputs: The sum is: 12
return 0;
}
Understanding Functions in C++
In C++, a function is a block of code that performs a specific task and can be reused throughout your program. Functions enhance code modularity and readability by breaking down complex tasks into manageable parts. There are two main types of functions in C++: built-in and user-defined. Built-in functions are provided by the C++ standard library, whereas user-defined functions allow programmers to create custom operations tailored to their specific needs.
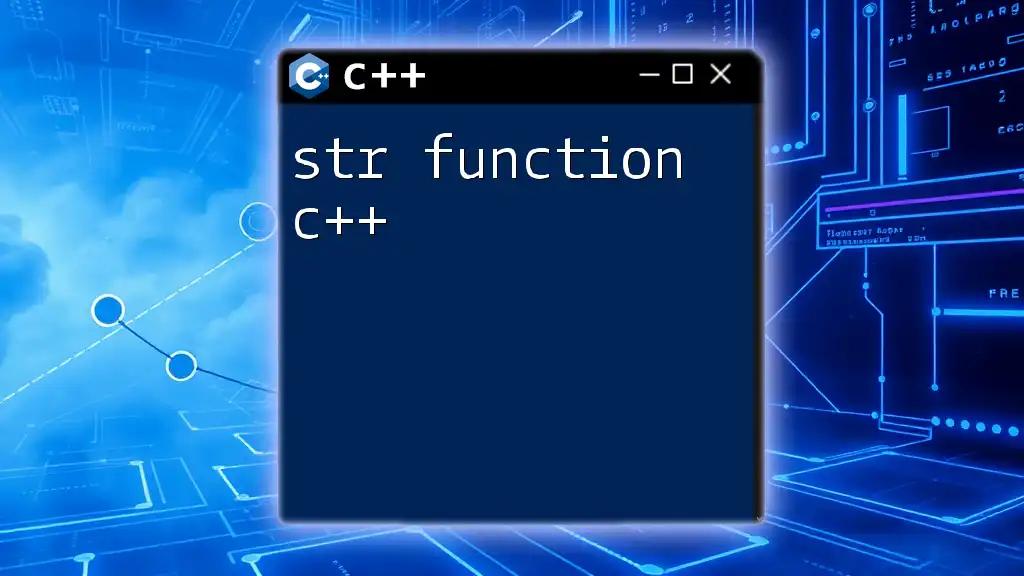
The Basics of Creating a Sum Function
Creating a sum function in C++ is straightforward. Let’s break down the syntax involved in defining a typical function:
- Return Type: The type of value the function will return. For a sum function, this is typically `int` (for integers).
- Function Name: A descriptive name indicating what the function does, like `sum`.
- Parameters: Inputs that the function can accept. These can help make the function more flexible.
- Body: The actual code that performs the calculation or operation.
Here’s a simple implementation of a sum function:
int sum(int a, int b) {
return a + b;
}
In this example:
- The return type is `int`, signifying that the function returns an integer.
- The function `sum` takes two parameters: `int a` and `int b`, which are then added together in the body of the function.
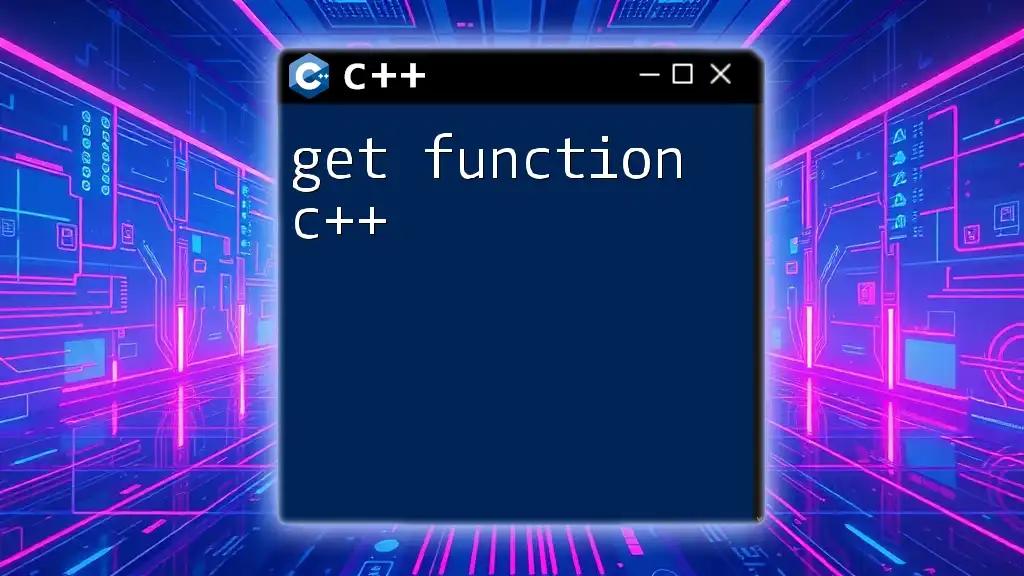
Different Ways to Implement a Sum Function
Using Parameters
At its simplest, the sum function can take two integer parameters and return their sum. This method is direct and works well when you know the number of inputs in advance:
int sum(int a, int b) {
return a + b;
}
Using Arrays
In many practical applications, you may need to sum multiple values stored in an array. Here’s how you could implement such a function:
int sumArray(int arr[], int size) {
int total = 0;
for(int i = 0; i < size; i++) {
total += arr[i];
}
return total;
}
In this code:
- The function `sumArray` accepts an array and its size.
- A simple for loop iterates through the array, adding each element to `total`.
- It then returns the computed total. This approach is particularly useful when dealing with dynamic sets of data.
Using Variadic Functions
C++ also supports variadic functions, which allow you to pass a variable number of arguments to a function. Here's an example of how you can implement a variadic sum function:
#include <cstdarg>
int sum(int count, ...) {
va_list args;
va_start(args, count);
int total = 0;
for (int i = 0; i < count; i++) {
total += va_arg(args, int);
}
va_end(args);
return total;
}
In this example:
- The `sum` function accepts an integer representing how many values will follow.
- The `va_list` type is used to handle the variable arguments with functions like `va_start` and `va_end` to iterate through each argument.
- This method is flexible, making it a good choice when the number of inputs is unpredictable.
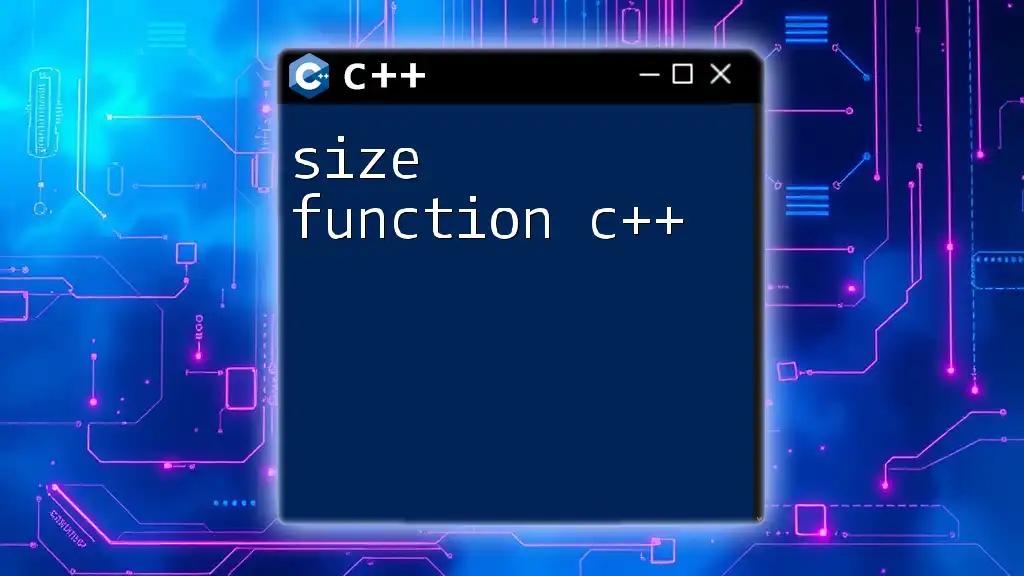
Modern C++ Approaches
Using STL (Standard Template Library)
The C++ Standard Template Library (STL) offers powerful tools for manipulating collections of data, including summing elements. One common approach is to use `std::accumulate` from the `<numeric>` header. Here is how you can sum a vector:
#include <vector>
#include <numeric>
int sumVector(const std::vector<int>& vec) {
return std::accumulate(vec.begin(), vec.end(), 0);
}
This code:
- Uses `std::accumulate`, which takes three arguments: the beginning of the vector, the end, and the initial value (0 in this case).
- It efficiently calculates the sum of all elements in the vector, showcasing the power of the C++ STL.
Using Lambda Expressions
Lambda functions provide a modern, concise way to write functions inline. Here’s how you can utilize a lambda expression to calculate the sum:
#include <algorithm>
#include <vector>
int sumWithLambda(const std::vector<int>& vec) {
return std::accumulate(vec.begin(), vec.end(), 0, [](int a, int b) {
return a + b;
});
}
In this example:
- `std::accumulate` is again used, but now with a lambda that defines how two items should be summed.
- This approach can add a layer of flexibility, allowing for easy changes to the summation logic without altering the function's signature.

Common Use Cases for Sum Functions
Sum functions are versatile and applicable in various domains, such as:
- Financial calculations: Summing expenses to calculate budgets or total sales.
- Statistical analysis: Computing totals for scores or measurements to derive averages or other statistical measures.
- Data processing: Aggressively aggregating values during data transformation tasks.
By using sum functions effectively, developers can streamline their code and make complex calculations manageable.
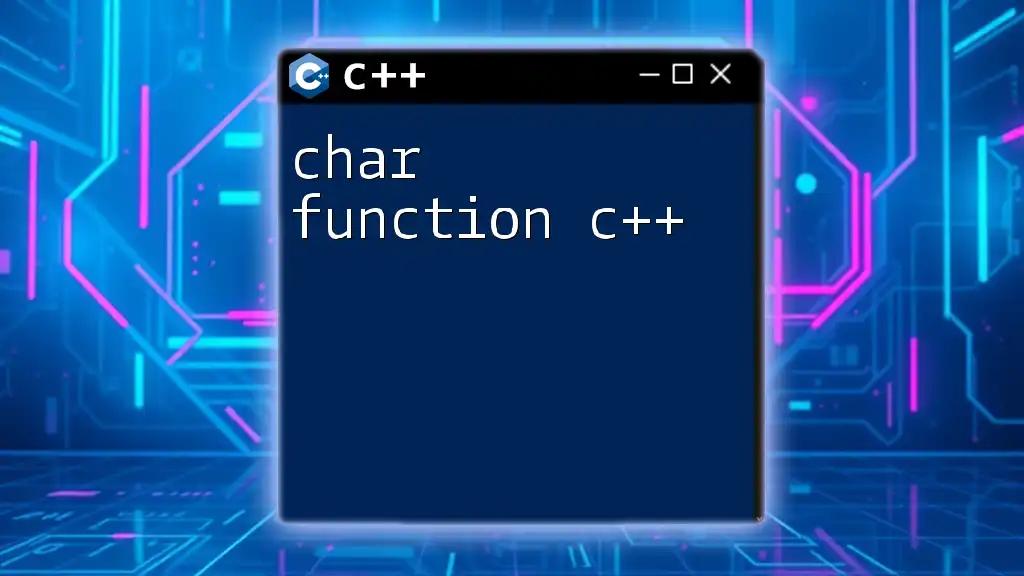
Performance Considerations
When choosing an implementation for the sum function, consider:
- Simplicity vs. Performance: While simple implementations are easier to understand, more complex solutions (like using STL) can offer better performance and readability for large datasets.
- Memory Usage: Variadic functions can be more memory-intensive, depending on how they are structured, while STL functions can optimize memory allocation and computational efficiency.
Selecting the appropriate method for your needs allows you to balance performance with usability, ensuring efficient coding practices.

Conclusion
The sum function in C++ is a critical tool for performing addition operations efficiently. By mastering the different ways to implement this function—from basic parameter passing to using modern C++ practices like STL and lambda functions—you can enhance your programming skills significantly. Start experimenting with these implementations to solidify your understanding and improve your coding efficiency in C++.